import numpy as np
import scipy as sp
import pandas as pd
import matplotlib.pyplot as plt
import pyreadstat
from IPython.display import display
from tangles.convenience import Survey, SurveyTangles
from tangles.convenience.survey_tangles_visualization import plot_tangle_search_tree
pd.set_option('display.max_rows', None)
pd.set_option('display.max_columns', None)
pd.set_option('display.max_colwidth', None)
vscrollbar = {'selector': '',
'props': ' height: 500px; overflow-y: scroll;display: inline-block;'}
hscrollbar = {'selector': '',
'props': ' height: 500px; overflow-x: scroll;display: inline-block;'}
def styled_df(df, scrollable: bool = True):
style = df.style.set_properties(**{'border': '1px black solid !important'})
if scrollable:
style = style.set_table_styles([vscrollbar, hscrollbar])
return style
Survey Tangles#
This notebook shows a small example of a tangle search. The purpose of this notebook is to introduce some facilities provided by the tangle library and to showcase recommended practices for the tangle analysis of survey data.
The survey we want to investigate is the European Value Study (EVS) from 2017: “The European Values Study (EVS) is a large-scale, cross-national, repeated cross-sectional survey research programme on basic human values. It provides insights into the ideas, beliefs, preferences, attitudes, values and opinions of citizens all over Europe” (from the EVS Website).
Due to license restrictions, we cannot share a copy of the survey data in our repository, but you can register and download the data for free at GESIS. (Please download the SPSS version of the EVS Integrated Dataset, create a folder called ‘data’ that lies next to this notebook and extract the downloaded ZIP file into this folder. If you downloaded the correct file, it should be called ZA7500_v5-0-0.sav).
1. Preparing, Cleaning and Selecting Data#
The tangle library provides special data types for survey data to make the tangle analysis for this type of data as convenient as possible. In this section, we provide a quick overview of the Survey Tangles Interface and show the steps needed to prepare survey data for tangles.
We first have to read the data. If the file was exported from a professional statistics tool, like SPSS or Stata, it is usually best to use the package pyreadstat
. The package allows to extract metadata from the file and store it in a dictionary-like object.
The quality of this metadata varies greatly with the tools used to export the data and the workflow preferences of the user. Therefore, it is often necessary to post-process the metadata for the tangle analysis. Nevertheless, using the metadata from SPSS-files often is a good starting point.
Files from SPSS usually contain a lot of information about missing data. It is highly recommended to use this information by setting the flag user_missing
of read_sav
to True
:
df, meta = pyreadstat.read_sav("./data/ZA7500_v5-0-0.sav", user_missing=True)
The tangle library provides a data type Survey
that manages survey data and its corresponding metadata. The main job of Survey
objects is to make sure that the data and its metadata do not go out of sync.
In the next cell, we create such a Survey
object and set the metadata afterward:
complete_survey = Survey(df)
complete_survey.set_pyreadstat_meta_data(meta, type_of_scale_variables='numeric', suppress_check_var_warning=True)
For the library to work properly, it is crucial to have meaningful metadata stored in the Survey
object. For the tangle analysis, the type of the variables associated to the questions is the most important kind of metadata. The library contains functions to automatically create binary features or separations from survey questions if their types are known.
The best way to turn a question into a set of associated features depends on the type of the variable corresponding to the question. The library supports three types:
Nominal variables: Variables that take one of a finite set of possible values. An example of a question best represented by a nominal variable is asking a respondent for its favorite ice cream. Such a question typically allows the user to choose one of a fixed set of options.
Ordinal variables: Like nominal variables, ordinal variables take one of a finite number of values, but these categories can be ordered in a meaningful way. An example would be a question asking about the number of days per week the respondent eats ice cream, with possible answers once, two times, more than two times but not every day and every day.
Numeric variables: These are variables that potentially can take arbitrary numeric values, for example a question asking for the amount of money spent on ice cream per year.
The assignment of a type to a variable is not always unambiguous. This is especially the case because there are different ways to classify survey data variables. For example, SPSS contains a variable type scale, that describes variables on interval scale or ratio scale. Depending on the type of scale, the use of certain statistical tools and techniques can be appropriate or inappropriate. In our context, the scale type might overlap the numeric and the ordinal type. It is not uncommon to see survey metadata where every variable has the type scale
.
Fortunately, to analyse tangles of a survey, we usually do not have to go into these statistical details. The type of a variable is only used to automatically find a good way to create binary features (or separations) from the questions. For this purpose, the library contains a generic feature factory that is easily customizable. A description of the details is out of the scope of this document. Here, we only list the currently implemented default ways to create features:
Nominal variables: If a nominal question has possible answers \(a_1, \dots, a_k\), the dataset is partitioned into \(k\) parts, the \(i\)-th containing the subset of all respondents that gave the answer \(a_i\).
Ordinal variables: If a question has possible answers \(a_1 < \dots < a_k\), the dataset is split at \(k-1\) thresholds \(a_2,\dots, a_k\) and the \(i\)-th feature contains all respondents that gave an answer greater or equal to \(a_{i+1}\).
Numeric variables: The range of a numeric variable is split into \(k\) parts using \(k-1\) equally spaced thresholds between the minimum and maximum value found in the set of all answers. Then, the \(i\)-th feature is the subset of respondents that gave an answer greater or equal to the \(i\)-th threshold.
As noted earlier, every survey contains the raw data set and information about the variables, i.e. the columns of the table. We can inspect information stored for a table column with the help of the function variable_info()
. Let’s have a look at the metadata found in the SPSS file:
styled_df(complete_survey.variable_info())
id | name | type | label | valid_values | invalid_values | is_usable | num_valid_answers | num_unique_answers | |
---|---|---|---|---|---|---|---|---|---|
studyno | 0 | studyno | numeric | GESIS study number | {} | {} | False | 59438 | 1 |
version | 1 | version | nominal | GESIS archive version | {} | {} | False | 59438 | 1 |
doi | 2 | doi | nominal | Digital Object Identifier | {} | {} | False | 59438 | 1 |
studynoc | 3 | studynoc | numeric | GESIS study number (national datasets) | {} | {} | True | 59438 | 36 |
versionc | 4 | versionc | nominal | GESIS archive version (national datasets) | {} | {} | False | 59438 | 5 |
id_cocas | 5 | id_cocas | numeric | unified respondent number | {} | {} | True | 59438 | 59438 |
caseno | 6 | caseno | numeric | original respondent number | {} | {} | True | 59438 | 19504 |
year | 7 | year | numeric | survey year | {2017.0: '2017', 2018.0: '2018', 2019.0: '2019', 2020.0: '2020', 2021.0: '2021'} | {} | True | 59438 | 5 |
fw_start | 8 | fw_start | numeric | year/month of start-fieldwork | {} | {} | True | 59438 | 19 |
fw_end | 9 | fw_end | numeric | year/month of end-fieldwork | {} | {} | True | 59438 | 19 |
country | 10 | country | numeric | country code (ISO 3166-1 numeric code) | {8.0: 'Albania', 31.0: 'Azerbaijan', 40.0: 'Austria', 51.0: 'Armenia', 70.0: 'Bosnia and Herzegovina', 100.0: 'Bulgaria', 112.0: 'Belarus', 191.0: 'Croatia', 203.0: 'Czechia', 208.0: 'Denmark', 233.0: 'Estonia', 246.0: 'Finland', 250.0: 'France', 268.0: 'Georgia', 276.0: 'Germany', 300.0: 'Greece', 348.0: 'Hungary', 352.0: 'Iceland', 380.0: 'Italy', 428.0: 'Latvia', 440.0: 'Lithuania', 499.0: 'Montenegro', 528.0: 'Netherlands', 578.0: 'Norway', 616.0: 'Poland', 620.0: 'Portugal', 642.0: 'Romania', 643.0: 'Russia', 688.0: 'Serbia', 703.0: 'Slovakia', 705.0: 'Slovenia', 724.0: 'Spain', 752.0: 'Sweden', 756.0: 'Switzerland', 804.0: 'Ukraine', 807.0: 'North Macedonia', 826.0: 'Great Britain'} | {} | True | 59438 | 36 |
c_abrv | 11 | c_abrv | nominal | country abbreviation (ISO 3166-1 Alpha-2 code) | {} | {} | False | 59438 | 36 |
cntry_y | 12 | cntry_y | numeric | country and year of FW (ISO 3166-1 numeric code) | {82018.0: 'Albania (2018)', 312018.0: 'Azerbaijan (2018)', 402018.0: 'Austria (2018)', 512018.0: 'Armenia (2018)', 702019.0: 'Bosnia and Herzegovina (2019)', 1002017.0: 'Bulgaria (2017)', 1122018.0: 'Belarus (2018)', 1912017.0: 'Croatia (2017)', 2032017.0: 'Czechia (2017)', 2082017.0: 'Denmark (2017)', 2332018.0: 'Estonia (2018)', 2462017.0: 'Finland (2017)', 2462018.0: 'Finland (2018)', 2502018.0: 'France (2018)', 2682018.0: 'Georgia (2018)', 2762017.0: 'Germany (2017)', 3002019.0: 'Greece (2019)', 3482018.0: 'Hungary (2018)', 3522017.0: 'Iceland (2017)', 3802018.0: 'Italy (2018)', 4282021.0: 'Latvia (2021)', 4402018.0: 'Lithuania (2018)', 4992019.0: 'Montenegro (2019)', 5282017.0: 'Netherlands (2017)', 5782018.0: 'Norway (2018)', 6162017.0: 'Poland (2017)', 6202020.0: 'Portugal (2020)', 6422018.0: 'Romania (2018)', 6422019.0: 'Romania (2019)', 6432017.0: 'Russia (2017)', 6882018.0: 'Serbia (2018)', 7032017.0: 'Slovakia (2017)', 7052017.0: 'Slovenia (2017)', 7242017.0: 'Spain (2017)', 7522017.0: 'Sweden (2017)', 7562017.0: 'Switzerland (2017)', 8042020.0: 'Ukraine (2020)', 8072019.0: 'North Macedonia (2019)', 8262018.0: 'Great Britain (2018)'} | {} | True | 59438 | 36 |
mode | 13 | mode | numeric | mode of data collection | {1.0: 'CAPI', 2.0: 'PAPI', 3.0: 'CAWI', 4.0: 'Mail', 5.0: 'CATI'} | {} | True | 59438 | 5 |
mm_select_sample | 14 | mm_select_sample | nominal | FLAG MIXED-MODE: subsamples on mode variables | {1.0: 'interviewer-administered (CAPI PAPI CATI)', 2.0: 'self-administered full-length questionnaire: original question order (CAWI Mail)', 3.0: 'self-administered full-length questionnaire: modified question order (CAWI Mail)', 4.0: 'self-administered matrix: with follow-up (CAWI Mail)', 5.0: 'self-administered matrix: follow-up non response (CAWI Mail)', 6.0: 'self-administered matrix: first survey only (CAWI Mail - DE)', 7.0: 'break-off (less than 50% valid answers)'} | {} | True | 59438 | 4 |
mm_mixed_mode | 15 | mm_mixed_mode | nominal | MATRIX DESIGN EXPERIMENT: mixed-mode/matrix design | {1.0: 'mixed-mode: full questionnaire', 2.0: 'mixed-mode: full (mod. order) questionnaire', 3.0: 'mixed-mode: matrix and follow-up', 4.0: 'mixed-mode: matrix only', 5.0: 'mixed-mode: not applied'} | {} | True | 59438 | 4 |
mm_mode_fu | 16 | mm_mode_fu | numeric | MATRIX DESIGN EXPERIMENT: mode of data collection (follow-up) | {1.0: 'CAWI (follow-up)', 2.0: 'MAIL (follow-up)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -3.0: 'not applicable'} | True | 3851 | 2 |
mm_matrix_group | 17 | mm_matrix_group | nominal | MATRIX DESIGN EXPERIMENT: matrix group/variable block | {1.0: ' [A B Core] [C D]', 2.0: ' [A C Core[ [B D]', 3.0: ' [A D Core] [B C]', 4.0: ' [B C Core] [A D]', 5.0: ' [B D Core] [A C]', 6.0: ' [C D Core] [A B]', 7.0: 'mixed mode: full questionnaire', 8.0: 'mixed mode: full (modified order) questionnaire', 11.0: '[A B Core]', 21.0: '[A C Core]', 31.0: '[A D Core]', 41.0: '[B C Core]', 51.0: '[B D Core]', 61.0: '[C D Core]'} | {-3.0: 'not applicable'} | True | 8346 | 8 |
mm_original_matrix_design_IS | 18 | mm_original_matrix_design_IS | nominal | IS MATRIX DESIGN EXPERIMENT: original matrix design assigned | {1.0: 'Matrix design assigned', 2.0: 'Full-length questionnaire assigned'} | {-4.0: 'item not included', -3.0: 'not applicable'} | True | 709 | 2 |
mm_original_matrix_group_IS | 19 | mm_original_matrix_group_IS | nominal | IS MATRIX DESIGN EXPERIMENT: original matrix group assigned | {1.0: 'Matrix design assigned: group 1', 2.0: 'Matrix design assigned: group 2', 3.0: 'Matrix design assigned: group 3', 4.0: 'Matrix design assigned: group 4', 5.0: 'Matrix design assigned: group 5', 6.0: 'Matrix design assigned: group 6', 7.0: 'Full-length questionnaire assigned'} | {-4.0: 'item not included', -3.0: 'not applicable'} | True | 709 | 7 |
mm_fw_start_fu | 20 | mm_fw_start_fu | numeric | MATRIX DESIGN EXPERIMENT: year/month of start-fieldwork (matrix design) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable'} | True | 3379 | 2 |
mm_fw_end_fu | 21 | mm_fw_end_fu | numeric | MATRIX DESIGN EXPERIMENT: year/month of end-fieldwork (matrix design) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable'} | True | 3379 | 3 |
mm_year_fu | 22 | mm_year_fu | numeric | MATRIX DESIGN EXPERIMENT: survey year (follow-up) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable'} | True | 3379 | 2 |
mr_detailed_mode_DE | 23 | mr_detailed_mode_DE | numeric | DE RESPONSIVE DESIGN EXPERIMENT: survey mode CAPI/CAWI/MAIL | {1.0: 'CAPI (base sample)', 2.0: 'CAPI (base follow-up)', 3.0: 'CAPI (sample increase)', 4.0: 'CAWI matrix (phase 1)', 5.0: 'CAWI matrix (phase 2)', 6.0: 'Mail matrix (phase 1)', 7.0: 'Mail matrix (phase 2)', 8.0: 'CAWI full length', 9.0: 'Mail full length'} | {-10.0: 'multi_referencing', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -5.0: 'other missings', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 676 | 2 |
mr_contact_mode_DE | 24 | mr_contact_mode_DE | numeric | DE RESPONSIVE DESIGN EXPERIMENT: contact mode CAWI/MAIL | {1.0: 'sequential', 2.0: 'simultaneous'} | {-10.0: 'multi_referencing', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -5.0: 'other missings', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | False | 676 | 1 |
mr_incentive_DE | 25 | mr_incentive_DE | numeric | DE RESPONSIVE DESIGN EXPERIMENT: incentive in Euros CAPI/CAWI/MAIL | {1.0: '10 EUR postpaid', 2.0: '5 EUR prepaid', 3.0: '20 EUR postpaid'} | {-10.0: 'multi_referencing', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -5.0: 'other missings', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | False | 676 | 1 |
fduplicate | 26 | fduplicate | nominal | FLAG duplication of cases: merging integrated/matrix design dataset | {0.0: 'no duplication of case after merging', 1.0: 'duplication of case after merging'} | {} | True | 59438 | 2 |
fmissings | 27 | fmissings | nominal | flag: complete/incomplete case | {0.0: 'full/complete case', 1.0: 'incomplete/partial case', 2.0: 'Break-off (Matrix Design)'} | {} | True | 59438 | 2 |
v1 | 28 | v1 | numeric | how important in your life: work (Q1A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58727 | 4 |
v2 | 29 | v2 | numeric | how important in your life: family (Q1B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59221 | 4 |
v3 | 30 | v3 | numeric | how important in your life: friends and acquaintances (Q1C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59215 | 4 |
v4 | 31 | v4 | numeric | how important in your life: leisure time (Q1D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59032 | 4 |
v5 | 32 | v5 | numeric | how important in your life: politics (Q1E) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58764 | 4 |
v6 | 33 | v6 | numeric | how important in your life: religion (Q1F) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58622 | 4 |
v7 | 34 | v7 | numeric | taking all things together how happy are you (Q2) | {1.0: 'very happy', 2.0: 'quite happy', 3.0: 'not very happy', 4.0: 'not at all happy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58707 | 4 |
v8 | 35 | v8 | numeric | describe your state of health these days (Q3) | {1.0: 'very good', 2.0: 'good', 3.0: 'fair', 4.0: 'poor', 5.0: 'very poor'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59304 | 5 |
v9 | 36 | v9 | numeric | do you belong to: religious organization (Q4A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58333 | 2 |
v10 | 37 | v10 | numeric | do you belong to: cultural activities (Q4B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58284 | 2 |
v11 | 38 | v11 | numeric | do you belong to: trade unions (Q4C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58269 | 2 |
v12 | 39 | v12 | numeric | do you belong to: political parties/groups (Q4D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58243 | 2 |
v13 | 40 | v13 | numeric | do you belong to: environment, ecology, animal rights (Q4E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58271 | 2 |
v14 | 41 | v14 | numeric | do you belong to: professional associations (Q4F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58223 | 2 |
v15 | 42 | v15 | numeric | do you belong to: sports/recreation (Q4G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58374 | 2 |
v16 | 43 | v16 | numeric | do you belong to: charitable/humanitarian organization (Q4H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58259 | 2 |
v17 | 44 | v17 | numeric | do you belong to: consumer organization (Q4I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58181 | 2 |
v18 | 45 | v18 | numeric | do you belong to: self-help group, mutual aid group (Q4J) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58176 | 2 |
v19 | 46 | v19 | numeric | do you belong to: other groups (Q4K) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57728 | 2 |
f20 | 47 | f20 | numeric | FLAG inconsistencies: do you belong to - none (spontaneous) (Q4) | {0.0: 'consistent', 1.0: 'inconsistent: respondent mentioned at least one item and none of these', 2.0: 'inconsistent: respondent is coded DK or NA for at least one item, and mentioned none of these', 3.0: 'inconsistent: none of these is coded DK or NA, although it is a spontaneous answer category (design error)'} | {-4.0: 'item not included'} | True | 53834 | 4 |
v20 | 48 | v20 | numeric | do you belong to: none (spontaneous) (Q4) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53185 | 2 |
v20a | 49 | v20a | nominal | do you belong to: I dont know (MQ: to complete question) (Q4) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 26602 | 2 |
v20b | 50 | v20b | nominal | do you belong to: no answer (MQ: to complete question) (Q4) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 26337 | 2 |
v21 | 51 | v21 | numeric | did you do voluntary work in the last 6 months (Q5) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58986 | 2 |
v22 | 52 | v22 | numeric | dont like as neighbours: people of different race (Q6A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57084 | 2 |
v23 | 53 | v23 | numeric | dont like as neighbours: heavy drinkers (Q6B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58075 | 2 |
v24 | 54 | v24 | numeric | dont like as neighbours: immigrants/foreign workers (Q6C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56520 | 2 |
f24_IT | 55 | f24_IT | nominal | IT FLAG EXPERIMENT (item wording modified) : experimental condition item v24 (Q6C) | {1.0: 'version 1 - dont like as neighbours: immigrants/foreign workers (item v24)', 2.0: 'version 2 - dont like as neighbours: foreign workers (item v24a_IT)', 3.0: 'version 3 - dont like as neighbours: immigrants (item v24b_IT)'} | {-4.0: 'item not included', -2.0: 'no answer', -1.0: 'dont know'} | True | 2277 | 3 |
v24a_IT | 56 | v24a_IT | nominal | IT EXPERIMENT (item wording modified): dont like as neighbours: foreign workers (Q6C: version 2) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-4.0: 'item not included', -2.0: 'no answer', -1.0: 'dont know'} | True | 303 | 2 |
v24b_IT | 57 | v24b_IT | nominal | IT EXPERIMENT (item wording modified): dont like as neighbours: immigrants (Q6C: version 3) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-4.0: 'item not included', -2.0: 'no answer', -1.0: 'dont know'} | True | 335 | 2 |
v25 | 58 | v25 | numeric | dont like as neighbours: drug addicts (Q6D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58247 | 2 |
v26 | 59 | v26 | numeric | dont like as neighbours: homosexuals (Q6E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57513 | 2 |
v27 | 60 | v27 | numeric | dont like as neighbours: Christians (optional in countries with Christian majority) (Q6F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49003 | 2 |
v28 | 61 | v28 | numeric | dont like as neighbours: Muslims (optional in countries with Muslim majority) (Q6G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55587 | 2 |
v29 | 62 | v29 | numeric | dont like as neighbours: Jews (optional) (Q6H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57061 | 2 |
v30 | 63 | v30 | numeric | dont like as neighbours: Gypsies (optional) (Q6I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57288 | 2 |
f30a | 64 | f30a | nominal | FLAG inconsistencies: dont like as neighbours - none of these (Q6) | {0.0: 'consistent', 1.0: 'inconsistent: respondent mentioned at least one item and none of these', 2.0: 'inconsistent: respondent is coded DK or NA for at least one item, and mentioned none of these', 3.0: 'inconsistent: none of these is coded DK or NA, although it is a spontaneous answer category (design error)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included'} | True | 19276 | 4 |
v30a | 65 | v30a | numeric | dont like as neighbours: wouldn't mind having any of these (Q6) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 17871 | 2 |
v30b | 66 | v30b | nominal | dont like as neighbours: I dont know (MQ: to complete question) (Q6) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 18369 | 2 |
v30c | 67 | v30c | nominal | dont like as neighbours: no answer (MQ: to complete question) (Q6) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 18823 | 2 |
v31 | 68 | v31 | numeric | people can be trusted/can't be too careful (Q7) | {1.0: 'most people can be trusted', 2.0: 'can not be too careful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58083 | 2 |
v32 | 69 | v32 | numeric | how much you trust: your family (Q8A) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59178 | 4 |
v33 | 70 | v33 | numeric | how much you trust: people in your neighborhood (Q8B) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58616 | 4 |
v34 | 71 | v34 | numeric | how much you trust: people you know personally (Q8C) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58981 | 4 |
v35 | 72 | v35 | numeric | how much you trust: people you meet for the first time (Q8D) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57969 | 4 |
v36 | 73 | v36 | numeric | how much you trust: people of another religion (Q8E) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54785 | 4 |
v37 | 74 | v37 | numeric | how much you trust: people of another nationality (Q8F) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55087 | 4 |
v38 | 75 | v38 | numeric | how much control over your life (Q9) | {1.0: 'none at all', 10.0: 'a great deal'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58550 | 10 |
v39 | 76 | v39 | numeric | how satisfied are you with your life (Q10) | {1.0: 'dissatisfied', 10.0: 'satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59122 | 10 |
v40 | 77 | v40 | numeric | important in a job: good pay (Q11A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58740 | 2 |
v41 | 78 | v41 | numeric | important in a job: good hours (Q11B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58538 | 2 |
v42 | 79 | v42 | numeric | important in a job: opportunity to use initiative (Q11C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57902 | 2 |
v43 | 80 | v43 | numeric | important in a job: generous holidays (Q11D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58007 | 2 |
v44 | 81 | v44 | numeric | important in a job: achieving something (Q11E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58218 | 2 |
v45 | 82 | v45 | numeric | important in a job: responsible job (Q11F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57965 | 2 |
f45a | 83 | f45a | numeric | FLAG inconsistencies: important in a job - none of these (spontaneous) (Q11) | {0.0: 'consistent', 1.0: 'inconsistent: respondent mentioned at least one item and none of these', 2.0: 'iconsistent: respondent is coded DK or NA for at least one item, and mentioned none of these', 3.0: 'inconsistent: none of these is coded DK or NA, although it is a spontaneous answer category (design error)'} | {-8.0: 'follow-up non response', -4.0: 'item not included'} | True | 55646 | 4 |
v45a | 84 | v45a | numeric | important in a job: none of these (spontaneous) (Q11) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55039 | 2 |
v45b | 85 | v45b | nominal | important in a job: I dont know (MQ: to complete question) (Q11) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 26626 | 2 |
v45c | 86 | v45c | nominal | important in a job: no answer (MQ: to complete question) (Q11) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27351 | 2 |
v46 | 87 | v46 | numeric | job needed to develop talents (Q12A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58777 | 5 |
f46_IT | 88 | f46_IT | nominal | IT FLAG EXPERIMENT: (answer cat. modified) experimental condition items v46 to v50 (Q12) | {1.0: "version 1 - d'accordo/contrario (agree/against)", 2.0: "version 2 - d'accordo/in disaccordo (agree/disagree)"} | {-4.0: 'item not included', -2.0: 'no answer', -1.0: 'dont know'} | True | 2277 | 2 |
v47 | 89 | v47 | numeric | humiliating receiving money without working (Q12B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58515 | 5 |
v48 | 90 | v48 | numeric | people turn lazy not working (Q12C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58687 | 5 |
v49 | 91 | v49 | numeric | work is a duty towards society (Q12D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58714 | 5 |
v50 | 92 | v50 | numeric | work comes always first (Q12E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58820 | 5 |
v51 | 93 | v51 | numeric | do you belong to a religious denomination (Q13) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58984 | 2 |
v52 | 94 | v52 | nominal | which religious denomination do you belong to (Q13a) (harmonized) | {1.0: 'Roman catholic', 2.0: 'Protestant', 3.0: 'Free church/Non-conformist/Evangelical', 4.0: 'Jew', 5.0: 'Muslim', 6.0: 'Hindu', 7.0: 'Buddhist', 8.0: 'Orthodox', 9.0: 'Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 41037 | 9 |
v52_cs | 95 | v52_cs | numeric | which religious denomination do you belong to (Q13a) (ISO 3166-1, country-specific) | {801.0: ' AL: Muslim (Sunni)', 802.0: ' AL: Muslim (Bekrathi)', 803.0: ' AL: Roman Catholics', 804.0: ' AL: Orthodox Christians', 805.0: ' AL: Evangelicals', 806.0: ' AL: Protestant', 807.0: ' AL: Mormon', 808.0: " AL: Jehovah's Witnesses", 809.0: ' AL: Hindu', 810.0: ' AL: Bahá’ís', 811.0: ' AL: Jewish', 812.0: ' AL: Buddhist', 813.0: ' AL: Other, please specify (Write in)', 3101.0: ' AZ: Muslim', 3102.0: ' AZ: Orthodox', 3103.0: ' AZ: Jewish', 3104.0: ' AZ: Catholic', 3105.0: " AZ: Baha'i", 3106.0: ' AZ: Protestant', 3130.0: ' AZ: \xa0Other, please specify (Write in)', 4001.0: ' AT: Roman Catholic', 4002.0: ' AT: Greek Catholic', 4003.0: ' AT: Armenic Catholic', 4004.0: ' AT: Other Roman Catholic', 4005.0: ' AT: Evangelic Church in Austria', 4006.0: ' AT: Evangelic Church of the Augsburg Confession', 4007.0: ' AT: Evangelic Church of the Helvetic Confession', 4008.0: ' AT: Uniting Churches (e.g. Greek Catholic)', 4009.0: ' AT: Other Evangelic Church', 4010.0: ' AT: Free-church', 4011.0: ' AT: Greek Oriental Church', 4012.0: ' AT: Russian Orthodox Church of Saint Nicholas', 4013.0: ' AT: Polish Orthodox Church', 4014.0: ' AT: Rumanian Greek Oriental Church Community of the Holy Resurrection', 4015.0: ' AT: Serbian Orthodox Church Community of Saint Sava', 4016.0: ' AT: Bulgarian Orthodox Church Community of Saint Ivan Rilski', 4017.0: ' AT: Other Orthodox Greek Oriental Church Community', 4018.0: ' AT: The Church of Jesus Christ of Latter-day Saints (Mormons)', 4019.0: "AT: Jehovah's Witnesses", 4020.0: ' AT: Old catholic', 4021.0: ' AT: Other Christian churches', 4022.0: ' AT: Jewish', 4023.0: ' AT: Alevism', 4024.0: ' AT: Shia', 4025.0: ' AT: Sunni', 4026.0: ' AT: Islam', 4027.0: ' AT: Other Islamic Community of Faith', 4028.0: ' AT: Hindu', 4029.0: 'AT: Buddhist', 4030.0: ' AT: Other religion', 4031.0: ' AT: Other, please specify (Write in)', 5101.0: 'AM: Armenian Apostolic Church', 5102.0: 'AM: Orthodox', 5103.0: 'AM: Catholic', 5104.0: 'AM: Protestant', 5105.0: 'AM: Muslim (Islam)', 5106.0: 'AM: Yazidi', 5116.0: 'AM: Other, please specify (Write in)', 7001.0: 'BA: Roman Catholic', 7002.0: 'BA: Serbian Orthodox', 7003.0: 'BA: Islam', 7004.0: 'BA: Jews', 7005.0: 'BA: Evangelic', 7006.0: 'BA: Adventist', 7007.0: 'BA: Baptist', 7008.0: "BA: Jehovah's witnesses", 7030.0: 'BA: Other, please specify (Write in)', 10001.0: 'BG: Orthodox', 10002.0: 'BG: Muslim', 10003.0: 'BG: Catholic', 10004.0: 'BG: Protestant', 10005.0: 'BG: Jew', 10006.0: 'BG: Buddhist', 10030.0: 'BG: \xa0Other, please specify (Write in)', 11201.0: 'BY: Orthodox Church', 11202.0: 'BY: Christians of Evangelical Belief (Pentecostals)', 11203.0: 'BY: Roman Catholic Church', 11204.0: 'BY: Evangelical Christian Baptists', 11205.0: 'BY: Seventh-Day Adventists', 11206.0: 'BY: Christians of the Whole Gospel', 11207.0: 'BY: Old Believers Church', 11208.0: 'BY: Judaic religion (Judaism)', 11209.0: 'BY: Lutheran Church', 11210.0: "BY: Jehovah's Witnesses", 11211.0: 'BY: Moslem religion (Islam)', 11212.0: 'BY: New Apostolic Church', 11213.0: 'BY: Greek Catholic Church (uniats)', 11214.0: 'BY: Other, please specify (Write in)', 19101.0: ' HR: The Roman Catholic Church', 19102.0: ' HR: The Greek-Catholic Church', 19103.0: ' HR: The Serbian Orthodox Church', 19104.0: ' HR: The Jewish Community of Zagreb', 19105.0: ' HR: The Evangelic Church', 19106.0: ' HR: The evangelical Church in Croatia (Pentecostal)', 19107.0: ' HR: Union of Baptists Churches in the Republic of Croatian', 19108.0: ' HR: Croatian – Slovenian Conference of the Seventh – Day Adventists Church', 19109.0: ' HR: Jehovah’s Witnesses', 19110.0: ' HR: Muslim (Islamic Community in Croatia)', 19116.0: ' HR: \xa0Other, please specify (Write in)', 20301.0: 'CZ: Roman Catholic', 20302.0: 'CZ: Greek-Catholic', 20303.0: 'CZ: Evangelical A.V. (Lutheran)', 20304.0: 'CZ: Evangelical Czech Brethren', 20305.0: 'CZ: Czechoslovak Hussite', 20306.0: 'CZ: Jewish', 20307.0: 'CZ: Muslim', 20308.0: 'CZ: Orthodox', 20309.0: "CZ: Jehovah's Witnesses", 20310.0: 'CZ: Other Christian', 20311.0: 'CZ: Other than Christian', 20312.0: 'CZ: Other', 20801.0: ' DK: National Church (protestant/lutheran)', 20802.0: ' DK: Islamic', 20803.0: ' DK: Buddhist', 20804.0: ' DK: Hindu', 20805.0: ' DK: Jewish', 20806.0: ' DK: Old Norse', 20807.0: ' DK: Other', 23301.0: 'EE: Lutheran', 23302.0: 'EE: Orthodox (Russian, Moscow)', 23303.0: 'EE: Orthodox (Constantinople)', 23304.0: 'EE: Catholic', 23305.0: 'EE: Judaist', 23306.0: 'EE: Muslim', 23307.0: 'EE: \xa0Other, please specify (Write in)', 24601.0: ' FI: \xa0Evangelical Lutheran Church', 24602.0: ' FI: Orthodox Church', 24603.0: ' FI: Roman Catholic Church', 24604.0: ' FI: Pentecostal Church', 24605.0: ' FI: Free Church', 24606.0: "FI: Jehovah's Witnesses", 24607.0: ' FI: Judaism', 24608.0: ' FI: Islam', 24610.0: ' FI: Other, please specify (Write in)', 25001.0: ' FR: Catholic', 25002.0: ' FR: Protestant', 25003.0: ' FR: Evangelical / free church', 25004.0: ' FR: Jew', 25005.0: ' FR: Muslim', 25006.0: ' FR: Hindu', 25007.0: ' FR: Buddhist', 25008.0: ' FR: Orthodox', 25016.0: ' FR: Other, please specify (Write in)', 25088.0: ' FR: don’t know (spontaneous)', 25099.0: ' FR: no answer (spontaneous)', 26801.0: 'GE: Orthodox', 26802.0: 'GE: Catholic', 26803.0: 'GE: Gregorian/Armenian Apostolic', 26804.0: 'GE: Protestant', 26805.0: 'GE: Jewish', 26806.0: 'GE: Muslim', 26807.0: "GE: Jehovah's Witnesses", 26808.0: 'GE: Sun Cult', 26809.0: 'GE: Baptist', 26816.0: 'GE: Other, please specify (Write in)', 27601.0: 'DE: Roman Catholic Church', 27602.0: 'DE: Protestant Church without Free Churches', 27603.0: 'DE: Protestant Free Churches', 27604.0: 'DE: Greek-Orthodox Church', 27605.0: 'DE: Russian-Orthodox Church', 27606.0: 'DE: Muslim', 27607.0: 'DE: Other religious denomination, please specify (Write in)', 34801.0: ' HU: Roman Catholic', 34802.0: ' HU: Greek Catholic, Armenian Catholic', 34803.0: ' HU: Calvinist', 34804.0: ' HU: Lutheran', 34805.0: ' HU: Baptist', 34806.0: ' HU: Orthodox', 34807.0: ' HU: Jewish', 34808.0: ' HU: Faith Church', 34809.0: ' HU: Witnesses of Jehova', 34810.0: ' HU: Krishna Consciousness', 34815.0: ' HU: \xa0Other, please specify (Write in)', 35201.0: 'IS: The Evangelical Lutheran Church of Iceland\xa0', 35202.0: 'IS: Catholic church\xa0', 35203.0: 'IS: The Independent Congregation', 35204.0: 'IS: The Free Lutheran Church in Reykjavik', 35205.0: 'IS: The Free Lutheran Church in Hafnarfjörður', 35206.0: 'IS: The Pentecostal Assembly\xa0', 35207.0: "IS: Jehova's Witnesses\xa0", 35208.0: "IS: The Bahá'í Community", 35209.0: 'IS: The Asatru Association', 35210.0: 'IS: Smárakirkja', 35211.0: 'IS: The Way', 35212.0: 'IS: Buddhist', 35213.0: 'IS: The Muslim Association of Iceland\xa0', 35214.0: 'IS: Other, which?', 35215.0: 'IS: Orthodox', 35216.0: 'IS: Sidmennt (the Icelandic Ethical Humanist Association)', 35217.0: 'IS: Seventh day Adventists', 35218.0: 'IS: Zuism', 35219.0: 'IS: Other Christian', 35220.0: 'IS: Other Non Christian', 38001.0: ' IT: Catholic', 38002.0: ' IT: Protestant', 38003.0: ' IT: Orthodox', 38004.0: " IT: Other Christian Religions (Jeovah's Withnesses, Mormons, etc.)", 38005.0: ' IT: Jewish', 38006.0: ' IT: Muslim', 38007.0: 'IT: Hindu', 38008.0: ' IT: Buddhist', 38030.0: ' IT: Other, Non-Christian Religion', 42801.0: ' LV: Catholic', 42802.0: ' LV: Lutheran', 42803.0: ' LV: Orthodox', 42804.0: ' LV: Baptist', 42805.0: ' LV: Old believers', 42806.0: ' LV: Pentecostal church', 42807.0: ' LV: Seventh day adventist', 42808.0: ' LV: Methodist church', 42809.0: ' LV: Muslim', 42810.0: ' LV: Buddhist', 42811.0: ' LV: Jew', 42816.0: ' LV: Other, please specify (Write in)', 44001.0: ' LT: Catholic', 44002.0: ' LT: Russian Orthodox', 44003.0: ' LT: Old Believer', 44004.0: ' LT: Evangelical Lutheran', 44005.0: ' LT: Evangelical Reformed', 44006.0: ' LT: Other Evangelical (e.g. Baptists, Methodists)', 44007.0: ' LT: Jew', 44008.0: ' LT: Muslim', 44016.0: ' LT: \xa0Other, please specify (Write in)', 49901.0: ' ME: Serbian Orthodox Church', 49902.0: ' ME: Montenegrin Orthodox Church', 49903.0: ' ME: Muslim', 49904.0: ' ME: Catholic', 49905.0: ' ME: Protestant', 49906.0: ' ME: Judaism', 49907.0: ' ME: Eastern religions and beliefs', 49916.0: ' ME: Other, please specify (Write in)', 49977.0: ' ME: not applicable', 49988.0: ' ME: don’t know (spontaneous)', 49999.0: ' ME: no answer (spontaneous)', 52801.0: 'NL: Roman Catholic', 52802.0: 'NL: Protestant Church Netherlands', 52803.0: 'NL: Protestant Church Netherlands (Reformed)', 52804.0: 'NL: Protestant Church Netherlands (Lutheran)', 52805.0: 'NL: Protestant Church Netherlands (not specified)', 52806.0: 'NL: Other Protestants', 52807.0: 'NL: Muslim', 52808.0: 'NL: Jewish', 52809.0: 'NL: Hindu', 52810.0: 'NL: Buddhism', 52811.0: 'NL: Other, please specify (Write in)', 57801.0: ' NO: The Church of Norway', 57802.0: ' NO: The Roman Catholic Church', 57803.0: ' NO: The Orthodox church, Russian, Greek, other', 57804.0: ' NO: Judaism', 57805.0: ' NO: Islam', 57806.0: 'NO: Hindu', 57807.0: 'NO: Buddhist', 57808.0: ' NO: Free churches (Protestant denominations outside The Church of Norway)', 57809.0: ' NO: \xa0Other, please specify (Write in)', 61601.0: 'PL: Roman-catholic', 61602.0: 'PL: Orhodox', 61603.0: 'PL: Jehovah’s Witnesses', 61604.0: 'PL: Protestant-mainstream', 61605.0: 'PL: Evangelic - other', 61606.0: 'PL: Greek-catholic', 61607.0: 'PL: Old-catholic Mariavite Church', 61608.0: 'PL: Judaism', 61609.0: 'PL: Islam', 61610.0: 'PL: Buddism', 61611.0: 'PL: Other, please specify (Write in)', 62001.0: ' PT: Catholic', 62002.0: ' PT: Protestant', 62003.0: ' PT: Eastern Orthodox', 62004.0: ' PT: Other Christian (specify)', 62005.0: ' PT: Jewish', 62006.0: ' PT: Islamic/Muslim', 62007.0: ' PT: Eastern religions', 62008.0: ' PT: Other non-Christian (specify)', 62009.0: ' PT: Evangelical', 62010.0: " PT: Jehovah's witness", 64201.0: ' RO: Roman Catholic Church', 64202.0: ' RO: Protestant Church', 64203.0: ' RO: Orthodox Church', 64204.0: ' RO: Jew', 64205.0: ' RO: Muslims', 64206.0: ' RO: Hindu', 64207.0: ' RO: Budhist', 64208.0: ' RO: Greek Catholic Church', 64209.0: " RO: New protestant Churches (Adventist, Baptist, Jehovah's Whitnesses etc.)", 64216.0: ' RO: \xa0Other, please specify (Write in)', 64301.0: ' RU: Orthodox Christian', 64302.0: ' RU: Catholicism', 64303.0: ' RU: Protestantism', 64304.0: ' RU: Islam', 64305.0: ' RU: Judaism', 64306.0: 'RU: Buddhist', 64307.0: 'RU: Hindu', 64316.0: ' RU: Other, please specify (Write in)', 68801.0: ' RS: Serbian Orthodox', 68802.0: ' RS: Roman Catholic', 68803.0: ' RS: Islam', 68804.0: ' RS: Jews', 68805.0: ' RS: Protestant', 68806.0: ' RS: Evangelic', 68807.0: ' RS: Slovakian evangelic', 68808.0: ' RS: Adventist', 68809.0: " RS: Jehovah's witnesses", 68810.0: ' RS: Baptist', 68830.0: ' RS: \xa0Other, please specify (Write in)', 70301.0: 'SK: Roman catholic', 70302.0: 'SK: Lutheran', 70303.0: 'SK: Greek Catholic', 70304.0: 'SK: Calvinist (Reform protestant)', 70305.0: 'SK: Orthodox', 70306.0: "GE: Jehovah's Witnesses", 70307.0: 'SK: Jew', 70308.0: 'SK: Other protestant (which one?)', 70309.0: 'SK: Other Christian, excepting protestant (which one?)', 70310.0: 'SK: Muslim', 70311.0: 'SK: Buddhist', 70312.0: 'SK: Hindu', 70316.0: 'SK: Other, please specify (Write in)', 70501.0: ' SI: Roman Catholic', 70502.0: ' SI: Evangelical', 70503.0: ' SI: Orthodox', 70504.0: ' SI: Other Christian', 70505.0: ' SI: Islamic', 70506.0: ' SI: Jewish', 70507.0: ' SI: Hinduistic', 70508.0: ' SI: Buddhist', 70509.0: ' SI: Other eastern', 70510.0: ' SI: Other, please specify (Write in)', 72401.0: 'ES: Christian – no denomination', 72402.0: 'ES: Roman Catholic', 72403.0: 'ES: Greek or Russian Orthodox', 72404.0: 'ES: Protestant (no further detail)', 72405.0: 'ES: Church of England / Anglican', 72406.0: 'ES: Baptist', 72407.0: 'ES: Methodist', 72408.0: 'ES: Presbyterian / Church of Scotland', 72409.0: 'ES: United Reform Church / Congregational', 72410.0: 'ES: Free Presbyterian', 72411.0: 'ES: Hindu', 72412.0: 'ES: Buddhist', 72413.0: 'ES: Jewish', 72414.0: 'ES: Islam / Muslim', 72416.0: 'ES: Other, please specify (Write in)', 75201.0: ' SE: Swedish church', 75202.0: ' SE: Catholic church', 75203.0: ' SE: An orthodox church or congregation', 75204.0: ' SE: Any independent church, protestant', 75205.0: ' SE: Jewish congregation', 75206.0: ' SE: Islamic community', 75207.0: ' SE: Hinduistic community', 75208.0: ' SE: Buddhistic community', 75216.0: ' SE: \xa0Other, please specify (Write in)', 75601.0: 'CH: Protestant reformed', 75602.0: 'CH: Free evangelical churches', 75603.0: 'CH: Roman Catholic', 75604.0: 'CH: Christian Catholic', 75605.0: 'CH: Christian Orthodox', 75606.0: 'CH: Jewish', 75607.0: 'CH: Islamic', 75608.0: 'CH: Buddhist', 75609.0: 'CH: Hindu', 75616.0: 'CH: Other, please specify (Write in)', 80401.0: ' UA: Orthodox Church of Ukraine (Metropolitan Epiphaniy)', 80402.0: ' UA: Ukrainian Orthodox Church (Metropolitan Onufriy)', 80403.0: ' UA: Ukrainian Greek Catholic Church', 80404.0: ' UA: Roman Catholic Church', 80405.0: ' UA: Evangelical Christian Baptist Church', 80406.0: ' UA: Christian Evangelicalism (Pentecostalism)', 80407.0: ' UA: Seventh-day Adventist Church', 80408.0: ' UA: Evangelicalism', 80409.0: ' UA: Charismatics', 80410.0: " UA: Jehovah's Witnesses", 80411.0: ' UA: Judaism', 80412.0: ' UA: Islam', 80413.0: ' UA: Buddhism', 80414.0: ' UA: Hinduism', 80415.0: ' UA: \xa0Other, please specify (Write in)', 80701.0: 'MK: Christianity - Orthodox', 80702.0: 'MK: Christianity - Catholicism', 80703.0: 'MK: Christianity - Protestantism Please specify', 80704.0: 'MK: Islam - (IRC - Islamic religious community in Republic of Macedonia)', 80705.0: 'MK: Judaism', 80706.0: 'MK: Small religious communities', 80716.0: 'MK: \xa0Other, please specify (Write in)', 82601.0: ' GB: Christian - no denomination', 82602.0: ' GB: Roman Catholic', 82603.0: ' GB: Church of England/Anglican', 82604.0: ' GB: Baptist', 82605.0: ' GB: Methodist', 82606.0: ' GB: Presbyterian/Church of Scotland', 82607.0: ' GB: Free Presbyterian', 82608.0: ' GB: Brethren', 82609.0: ' GB: United Reform Church (URC)/Congregational', 82610.0: ' GB: Other Protestant (WRITE IN)', 82611.0: ' GB: Other Christian (WRITE IN)', 82612.0: ' GB: Hindu', 82613.0: ' GB: Jewish', 82614.0: ' GB: Islam/Muslim', 82615.0: ' GB: Sikh', 82616.0: ' GB: Buddhist', 82617.0: ' GB: Other non-Christian (WRITE IN)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 41036 | 299 |
v53 | 96 | v53 | numeric | did you belong to a religious denomination (Q14) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 18919 | 2 |
v54 | 97 | v54 | numeric | how often attend religious services (Q15) | {1.0: 'more than once week', 2.0: 'once a week', 3.0: 'once a month', 4.0: 'only on specific holy days', 5.0: 'once a year', 6.0: 'less often', 7.0: 'never, practically never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58851 | 7 |
v55 | 98 | v55 | numeric | how often attend religious services 12 years old (Q16) | {1.0: 'more than once week', 2.0: 'once a week', 3.0: 'once a month', 4.0: 'only on specific holy days', 5.0: 'once a year', 6.0: 'less often', 7.0: 'never, practically never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57551 | 7 |
v56 | 99 | v56 | numeric | are you a religious person (Q17) | {1.0: 'a religious person', 2.0: 'not a religious person', 3.0: 'a convinced atheist'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57082 | 3 |
v57 | 100 | v57 | numeric | do you believe in: God (Q18A) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55894 | 2 |
v58 | 101 | v58 | numeric | do you believe in: life after death (Q18B) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51186 | 2 |
v59 | 102 | v59 | numeric | do you believe in: hell (Q18C) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51868 | 2 |
v60 | 103 | v60 | numeric | do you believe in: heaven (Q18D) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51899 | 2 |
v61 | 104 | v61 | numeric | do you believe in: re-incarnation (Q19) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52299 | 2 |
v62 | 105 | v62 | numeric | which statement closest to your beliefs (Q20) | {1.0: 'personal God', 2.0: 'spirit or life force', 3.0: "don't know what to think", 4.0: 'no spirit, God or life force'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57497 | 4 |
v63 | 106 | v63 | numeric | how important is God in your life (Q21) | {1.0: 'not at all important', 10.0: 'very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57947 | 10 |
v64 | 107 | v64 | numeric | how often do you pray outside religious services (Q22) | {1.0: 'every day', 2.0: 'more than once week', 3.0: 'once a week', 4.0: 'at least once a month', 5.0: 'several times a year', 6.0: 'less often', 7.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57882 | 7 |
v65 | 108 | v65 | numeric | important in marriage: faithfulness (Q23A) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59138 | 3 |
v66 | 109 | v66 | numeric | important in marriage: adequate income (Q23B) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59078 | 3 |
v67 | 110 | v67 | numeric | important in marriage: good housing (Q23C) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59121 | 3 |
v68 | 111 | v68 | numeric | important in marriage: share household chores (Q23D) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58887 | 3 |
v69 | 112 | v69 | numeric | important in marriage: children (Q23E) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58676 | 3 |
v70 | 113 | v70 | numeric | important in marriage: time for friends and personal hobbies (Q23F) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58814 | 3 |
v71 | 114 | v71 | numeric | marriage is outdated (Q24) | {1.0: 'agree', 2.0: 'disagree'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57349 | 2 |
v72 | 115 | v72 | numeric | child suffers with working mother (Q25A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58080 | 4 |
v72_DE | 116 | v72_DE | numeric | DE MODIFIED (additional middle cat): child suffers with working mother (Q25A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v73 | 117 | v73 | numeric | women really want home and children (Q25B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57241 | 4 |
v73_DE | 118 | v73_DE | numeric | DE MODIFIED (additional middle cat): women really want home and children (Q25B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v74 | 119 | v74 | numeric | family life suffers when woman has full-time job (Q25C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58055 | 4 |
v74_DE | 120 | v74_DE | numeric | DE MODIFIED (additional middle cat): family life suffers when woman has full-time job (Q25C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v75 | 121 | v75 | numeric | man's job is to earn money; woman's job is to look after home and family (Q25D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58466 | 4 |
v75_DE | 122 | v75_DE | numeric | DE MODIFIED (additional middle cat): man's job is to earn money; woman's job is to look after home and family (Q25D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v76 | 123 | v76 | numeric | men make better political leaders than women (Q25E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56994 | 4 |
v76_DE | 124 | v76_DE | numeric | DE MODIFIED (additional middle cat): men make better political leaders than women (Q25E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v77 | 125 | v77 | numeric | university education more important for a boy than for a girl (Q25F) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58183 | 4 |
v77_DE | 126 | v77_DE | numeric | DE MODIFIED (additional middle cat): university education more important for a boy than for a girl (Q25F) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v78 | 127 | v78 | numeric | men make better business executives than women (Q25G) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57158 | 4 |
v78_DE | 128 | v78_DE | numeric | DE MODIFIED (additional middle cat): men make better business executives than women (Q25G) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v79 | 129 | v79 | numeric | one of main goals in life has been to make my parents proud (Q25H) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56622 | 4 |
v79_DE | 130 | v79_DE | numeric | DE MODIFIED (additional middle cat): one of main goals in life has been to make my parents proud (Q25H) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree (additional category)', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 0 | 0 |
v80 | 131 | v80 | numeric | jobs are scarce: giving...(nation) priority (Q26A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58633 | 5 |
v81 | 132 | v81 | numeric | jobs are scarce: giving men priority (Q26B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58695 | 5 |
v82 | 133 | v82 | numeric | homosexual couples - as good parents as other couples (Q27A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54764 | 5 |
v83 | 134 | v83 | numeric | duty towards society to have children (Q27B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58491 | 5 |
v84 | 135 | v84 | numeric | It is childs duty to provide long-term care for parents (Q27C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58678 | 5 |
f85 | 136 | f85 | nominal | FLAG inconsistencies: learn children at home: more than five (Q28) | {0.0: 'consistent', 1.0: 'inconsistent'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'Item not included'} | True | 59438 | 2 |
v85 | 137 | v85 | numeric | learn children at home: good manners (Q28A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58798 | 2 |
v86 | 138 | v86 | numeric | learn children at home: independence (Q28B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58627 | 2 |
v87 | 139 | v87 | numeric | learn children at home: hard work (Q28C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58708 | 2 |
v88 | 140 | v88 | numeric | learn children at home: feeling of responsibility (Q28D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58729 | 2 |
v89 | 141 | v89 | numeric | learn children at home: imagination (Q28E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58160 | 2 |
v90 | 142 | v90 | numeric | learn children at home: tolerance and respect (Q28F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58560 | 2 |
v91 | 143 | v91 | numeric | learn children at home: thrift, saving money and things (Q28G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58432 | 2 |
v92 | 144 | v92 | numeric | learn children at home: determination, perseverance (Q28H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58376 | 2 |
v93 | 145 | v93 | numeric | learn children at home: religious faith (Q28I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58124 | 2 |
v94 | 146 | v94 | numeric | learn children at home: unselfishness (Q28J) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58190 | 2 |
v95 | 147 | v95 | numeric | learn children at home: obedience (Q28K) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58189 | 2 |
f96 | 148 | f96 | numeric | FLAG inconsistencies: learn children at home - none (spontaneous) (Q28) | {0.0: 'consistent', 1.0: 'inconsistent: respondent mentioned at least one item and none of these', 2.0: 'inconsistent: respondent is coded DK or NA for at least one item, and mentioned none of these', 3.0: 'inconsistent: none of these is coded DK or NA, although it is a spontaneous answer category (design error)'} | {-8.0: 'follow-up non response', -4.0: 'item not included'} | True | 54275 | 4 |
v96 | 149 | v96 | numeric | learn children at home: none (spontaneous) (Q28) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53671 | 2 |
v96a | 150 | v96a | nominal | learn children at home: I dont know (MQ: to complete question) (Q28) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 32113 | 2 |
v96b | 151 | v96b | nominal | learn children at home: no answer (MQ: to complete question) (Q28) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 32838 | 2 |
v97 | 152 | v97 | numeric | how interested are you in politics (Q29) | {1.0: 'very interested', 2.0: 'somewhat interested', 3.0: 'not very interested', 4.0: 'not at all interested'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59132 | 4 |
v98 | 153 | v98 | numeric | political action: signing a petition (Q30A) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57002 | 3 |
v99 | 154 | v99 | numeric | political action: joining in boycotts (Q30B) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55875 | 3 |
v100 | 155 | v100 | numeric | political action: attending lawful demonstrations (Q30C) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56622 | 3 |
v101 | 156 | v101 | numeric | political action: joining unofficial strikes (Q30D) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55474 | 3 |
v102 | 157 | v102 | numeric | political view: left-right (Q31) | {1.0: 'the left', 10.0: 'the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47723 | 10 |
v103 | 158 | v103 | numeric | individual vs. state responsibility for providing (Q32A) | {1.0: 'individual responsibility', 10.0: 'state responsibility'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58214 | 10 |
v104 | 159 | v104 | numeric | take any job vs. right to refuse job when unemployed (Q32B) | {1.0: 'unemployed take any job', 10.0: 'unemployed right to refuse a job'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58066 | 10 |
v105 | 160 | v105 | numeric | competition is good vs. harmful (Q32C) | {1.0: 'competition good', 10.0: 'competition harmful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57516 | 10 |
v106 | 161 | v106 | numeric | equalize incomes vs. incentives for individual effort (Q32D) | {1.0: 'incomes more equal', 10.0: 'incentives to individual efforts'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58103 | 10 |
v107 | 162 | v107 | numeric | private vs. government ownership business (Q32E) | {1.0: 'private ownership increased', 10.0: 'government ownership increased'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54991 | 10 |
f108 | 163 | f108 | nominal | FLAG inconsistencies: aims of country (Q33/Q33a) | {0.0: 'consistent', 1.0: 'inconsistent'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included'} | True | 59248 | 2 |
v108 | 164 | v108 | numeric | aims of this country: most important (Q33) | {1.0: 'high level of economic growth', 2.0: 'strong defense forces', 3.0: 'more say at their jobs/ in communities', 4.0: 'make cities/countryside more beautiful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57503 | 4 |
v109 | 165 | v109 | numeric | aims of this country: 2nd most important (Q33a) | {1.0: 'high level of economic growth', 2.0: 'strong defense forces', 3.0: 'more say at their jobs/ in communities', 4.0: 'make cities/countryside more beautiful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56342 | 4 |
f110 | 166 | f110 | nominal | FLAG inconsistencies: aims of respondent (Q34/Q35) | {0.0: 'consistent', 1.0: 'inconsistent'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included'} | True | 59321 | 2 |
v110 | 167 | v110 | numeric | aims of respondent: most important (Q34) | {1.0: 'maintaining order in nation', 2.0: 'more say in important government decisions', 3.0: 'fighting rising prices', 4.0: 'protect freedom of speech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58066 | 4 |
v111 | 168 | v111 | numeric | aims of respondent: 2nd most important (Q35) | {1.0: 'maintaining order in nation', 2.0: 'more say in important government decisions', 3.0: 'fighting rising prices', 4.0: 'protect freedom of speech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57178 | 4 |
v111_4 | 169 | v111_4 | nominal | post-materialist index 4-item (constructed) | {1.0: 'Materialist', 2.0: 'Mixed', 3.0: 'Postmaterialist'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57053 | 3 |
v112 | 170 | v112 | numeric | are you willing to fight for country (Q36) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52718 | 2 |
f112_SE | 171 | f112_SE | nominal | SE MODIFIED QUESTION: are you willing to fight for country (Q36) | {1.0: "Wording of Q36 using the term 'strida för' (fight for)", 2.0: "Wording of Q36 using the term 'försvara' (defend)"} | {-4.0: 'item not included'} | True | 1194 | 2 |
v113 | 172 | v113 | numeric | good/bad: decrease work importance (Q37A) | {1.0: 'good', 2.0: 'bad', 3.0: "don't mind"} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56028 | 3 |
v114 | 173 | v114 | numeric | good/bad: greater respect for authority (Q37B) | {1.0: 'good', 2.0: 'bad', 3.0: "don't mind"} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55280 | 3 |
v115 | 174 | v115 | numeric | how much confidence in: church (Q38A) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57455 | 4 |
v116 | 175 | v116 | numeric | how much confidence in: armed forces (Q38B) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56006 | 4 |
v117 | 176 | v117 | numeric | how much confidence in: education system (Q38C) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58159 | 4 |
v118 | 177 | v118 | numeric | how much confidence in: the press (Q38D) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58072 | 4 |
v119 | 178 | v119 | numeric | how much confidence in: trade unions (Q38E) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54191 | 4 |
v120 | 179 | v120 | numeric | how much confidence in: the police (Q38F) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58495 | 4 |
v121 | 180 | v121 | numeric | how much confidence in: parliament (Q38G) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57366 | 4 |
v122 | 181 | v122 | numeric | how much confidence in: civil service (Q38H) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57228 | 4 |
v123 | 182 | v123 | numeric | how much confidence in: social security system (Q38I) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57294 | 4 |
v124 | 183 | v124 | numeric | how much confidence in: European Union (Q38J) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55705 | 4 |
v125 | 184 | v125 | numeric | how much confidence in: United Nations Organization (Q38K) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54168 | 4 |
v126 | 185 | v126 | numeric | how much confidence in: health care system (Q38L) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58585 | 4 |
v127 | 186 | v127 | numeric | how much confidence in: justice system (Q38M) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57092 | 4 |
v128 | 187 | v128 | numeric | how much confidence in: major companies (Q38N) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54645 | 4 |
v129 | 188 | v129 | numeric | how much confidence in: environmental organizations (Q38O) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55582 | 4 |
v130 | 189 | v130 | numeric | how much confidence in: political parties (Q38P) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56884 | 4 |
v131 | 190 | v131 | numeric | how much confidence in: government (Q38Q) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57506 | 4 |
v132 | 191 | v132 | numeric | how much confidence in: social media (Q38R) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54341 | 4 |
v133 | 192 | v133 | numeric | democracy: governments tax the rich and subsidize the poor (Q39A) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52980 | 11 |
v133_11c | 193 | v133_11c | numeric | MODIFIED (cat. 0 displayed): democracy: governments tax the rich and subsidize the poor (Q39A) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3283 | 11 |
v134 | 194 | v134 | numeric | democracy: religious authorities interpret the laws (Q39B) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51892 | 11 |
v134_11c | 195 | v134_11c | numeric | MODIFIED (cat. 0 displayed): democracy: religious authorities interpret the laws (Q39B) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3227 | 11 |
v135 | 196 | v135 | numeric | democracy: people choose their leaders in free elections (Q39C) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54015 | 11 |
v135_11c | 197 | v135_11c | numeric | MODIFIED (cat. 0 displayed): democracy: people choose their leaders in free elections (Q39C) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3368 | 11 |
v136 | 198 | v136 | numeric | democracy: people receive state aid for unemployment (Q39D) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53977 | 11 |
v136_11c | 199 | v136_11c | numeric | MODIFIED (cat. 0 displayed): democracy: people receive state aid for unemployment (Q39D) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3387 | 11 |
v137 | 200 | v137 | numeric | democracy: the army takes over when government is incompetent (Q39E) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51896 | 11 |
v137_11c | 201 | v137_11c | numeric | MODIFIED (cat. 0 displayed): democracy: the army takes over when government is incompetent (Q39E) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3271 | 11 |
v138 | 202 | v138 | numeric | democracy: civil rights protect people from state oppression (Q39F) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52504 | 11 |
v138_11c | 203 | v138_11c | numeric | MODIFIED (cat. 0 displayed): democracy: civil rights protect people from state oppression (Q39F) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3323 | 11 |
v139 | 204 | v139 | numeric | democracy: the state makes people’s incomes equal (Q39G) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53050 | 11 |
v139_11c | 205 | v139_11c | numeric | MODIFIED (cat. 0 displayed): democracy: the state makes people’s incomes equal (Q39G) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3328 | 11 |
v140 | 206 | v140 | numeric | democracy: people obey their rulers (Q39H) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53068 | 11 |
v140_11c | 207 | v140_11c | numeric | MODIFIED (cat. 0 displayed): democracy: people obey their rulers (Q39H) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3326 | 11 |
v141 | 208 | v141 | numeric | democracy: women have the same rights as men (Q39I) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54426 | 11 |
v141_11c | 209 | v141_11c | numeric | MODIFIED (cat. 0 displayed): democracy: women have the same rights as men (Q39I) | {0.0: 'it is against democracy', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3440 | 11 |
v142 | 210 | v142 | numeric | importance of democracy (Q40) | {1.0: 'not at all important', 10.0: 'absolutely important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58125 | 10 |
v143 | 211 | v143 | numeric | democracy in own country (Q41) | {1.0: 'not at all democratic', 10.0: 'completely democratic'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57214 | 10 |
v144 | 212 | v144 | numeric | satisfaction political system (Q42) | {1.0: 'not satisfied at all', 10.0: 'completely satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57530 | 10 |
v145 | 213 | v145 | numeric | political system: strong leader (Q43A) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55374 | 4 |
v146 | 214 | v146 | numeric | political system: experts making decisions (Q43B) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54313 | 4 |
v147 | 215 | v147 | numeric | political system: the army ruling (Q43C) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55409 | 4 |
v148 | 216 | v148 | numeric | political system: democratic (Q43D) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56245 | 4 |
v149 | 217 | v149 | numeric | do you justify: claiming state benefits (Q44A) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58240 | 10 |
v150 | 218 | v150 | numeric | do you justify: cheating on tax (Q44B) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58511 | 10 |
v151 | 219 | v151 | numeric | do you justify: taking soft drugs (Q44C) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58442 | 10 |
v152 | 220 | v152 | numeric | do you justify: accepting a bribe (Q44D) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58723 | 10 |
v153 | 221 | v153 | numeric | do you justify: homosexuality (Q44E) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56952 | 10 |
v154 | 222 | v154 | numeric | do you justify: abortion (Q44F) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57277 | 10 |
v155 | 223 | v155 | numeric | do you justify: divorce (Q44G) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57903 | 10 |
v156 | 224 | v156 | numeric | do you justify: euthanasia (Q44H) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56185 | 10 |
v157 | 225 | v157 | numeric | do you justify: suicide (Q44I) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56705 | 10 |
v158 | 226 | v158 | numeric | do you justify: having casual sex (Q44J) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57033 | 10 |
v159 | 227 | v159 | numeric | do you justify: avoiding a fare on public transport (Q44K) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58559 | 10 |
v160 | 228 | v160 | numeric | do you justify: prostitution (Q44L) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57709 | 10 |
v161 | 229 | v161 | numeric | do you justify: artificial insemination or in-vitro fertilization (Q44M) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56800 | 10 |
v162 | 230 | v162 | numeric | do you justify: political violence (Q44N) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58009 | 10 |
v163 | 231 | v163 | numeric | do you justify: death penalty (Q44O) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57123 | 10 |
v164 | 232 | v164 | numeric | how close do you feel: to own town/city (Q45A) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58942 | 4 |
v165 | 233 | v165 | numeric | how close do you feel: to your [county, region, district] (Q45B) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58786 | 4 |
v166 | 234 | v166 | numeric | how close do you feel: to [country] (Q45C) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58857 | 4 |
v167 | 235 | v167 | numeric | how close do you feel: to [continent] (Q45D) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58110 | 4 |
v168 | 236 | v168 | numeric | how close do you feel: to world (Q45E) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57393 | 4 |
v169 | 237 | v169 | numeric | do you have... [country's] nationality (Q46) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59318 | 2 |
v170 | 238 | v170 | numeric | how proud are you to be a ... [country] citizen (Q47) | {1.0: 'very proud', 2.0: 'quite proud', 3.0: 'not very proud', 4.0: 'not at all proud'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55885 | 4 |
v171 | 239 | v171 | numeric | vote in elections: local level (Q48A) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 57525 | 3 |
v172 | 240 | v172 | numeric | vote in elections: national level (Q48B) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 56952 | 3 |
v173 | 241 | v173 | numeric | vote in elections: European level (Q48C) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 34242 | 3 |
v174_LR | 242 | v174_LR | nominal | political party: appeals to you most: left/right scale (Q49) | {1.0: 'left', 2.0: '', 3.0: '', 4.0: '', 5.0: '', 6.0: '', 7.0: '', 8.0: '', 9.0: '', 10.0: 'right', 44.0: 'not classifiable'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 40448 | 11 |
v174_cs | 243 | v174_cs | numeric | which political party appeals to you most (Q49) (ISO 3166-1, country-specific) | {801.0: ' AL: Democratic Party, PD', 802.0: ' AL: Socialist Party', 803.0: ' AL: Socialist Movement for Integration', 804.0: ' AL: The Human Rights Union Party', 805.0: ' AL: Christian Democrats', 806.0: ' AL: Republican Party', 807.0: ' AL: Social Democracy Party', 808.0: ' AL: Movement of Legality Party', 809.0: ' AL: National Front Party', 810.0: ' AL: New Democrat Party', 811.0: ' AL: Justice, Integration and Unity Party', 812.0: ' AL: Challenge for Albania', 813.0: ' AL: Social Democratic Party', 814.0: ' AL: Environmental Party', 815.0: ' AL: Albanian Democratic Union Party', 816.0: ' AL: Liberal Democratic Union Party', 817.0: ' AL: Agrarian Environmentalist Party', 818.0: " AL: Albanian Workers' Party", 819.0: ' AL: Communist Party of Albania', 820.0: " AL: The People's Alliance Party", 821.0: ' AL: Equal List, LIBRA', 822.0: ' AL: Green Party', 823.0: ' AL: Albanian Republican Union Party', 824.0: ' AL: Moderate Socialist Party', 825.0: ' AL: Party of People with Disabilities', 826.0: ' AL: Other, please specify (WRITE IN)', 866.0: ' AL: No [no other] party appeals to me', 877.0: ' AL: not applicable', 888.0: ' AL: don’t know (spontaneous)', 899.0: ' AL: no answer (spontaneous)', 3101.0: ' AZ: New Azerbaijan Party', 3102.0: ' AZ: Musavat/Equality Party', 3103.0: ' AZ: Azerbaijan Democratic Party', 3104.0: ' AZ: Popular Front Party', 3105.0: ' AZ: National Independence Party', 3106.0: ' AZ: Azerbaijan Liberal Party', 3107.0: ' AZ: Social-Democratic Party', 3108.0: ' AZ: Communist Party', 3109.0: ' AZ: Justice Party', 3110.0: ' AZ: Civic Solidarity Party', 3111.0: ' AZ: Motherland Party', 3112.0: ' AZ: Azerbaijan Party of Hope', 3113.0: ' AZ: Social Prosperity Party', 3114.0: ' AZ: Azerbaijan Party of Democratic Reform', 3115.0: ' AZ: Whole Azerbaijan Popular Front Party', 3116.0: ' AZ: Great Order Party', 3117.0: ' AZ: United Communist Party', 3118.0: ' AZ: Party of Unity', 3119.0: ' AZ: Modern Musavat Party', 3131.0: ' AZ: Other, please specify (WRITE IN)', 3166.0: ' AZ: No [, no other] party appeals to me (spontaneous)', 4001.0: 'AT: Social Democratic Party of Austria - SPÖ', 4002.0: "AT: List Kurz / Austrian People's Party - ÖVP", 4003.0: 'AT: Freedom Party of Austria - FPÖ', 4004.0: 'AT: The Greens - The Green Alternative', 4005.0: 'AT: The new Austria - NEOS', 4006.0: 'AT: List Peter Pilz', 4026.0: 'AT: Other, please specify (WRITE IN)', 4066.0: 'AT: No [no other] party appeals to me (spontaneous)', 5101.0: 'AM: Armenian Revolutionary Federation', 5102.0: 'AM: Armenian Communist Party', 5103.0: "AM: 'Exit' (Way out) Party Union", 5104.0: 'AM: Free Democrats Party', 5105.0: 'AM: Democratic Party of Armenia', 5106.0: 'AM: Heritage Party', 5107.0: 'AM: Armenian National Congress (former All-Armenian Movement)', 5108.0: 'AM: People’s Party of Armenia', 5109.0: 'AM: Tsarukyan Block (Prosperous Armenia Party)', 5110.0: 'AM: Republican Party of Armenia', 5111.0: "AM: 'Apricot`s country' Party", 5112.0: 'AM: Armenian Renaissance Party (Former Rule of Law)', 5126.0: 'AM: Other, please specify (WRITE IN)', 5166.0: 'AM: No [no other] party appeals to me (spontaneous)', 7001.0: 'BA: Bosnian Party', 7002.0: 'BA: Democratic Front', 7003.0: 'BA: Croatian Democratic Union - 1990', 7004.0: 'BA: Croatian Democratic Union', 7005.0: 'BA: Croatian republican party', 7006.0: 'BA: Croatian Party of Rights', 7007.0: 'BA: Liberal Democratic Party', 7008.0: 'BA: People and Justice', 7009.0: 'BA: Our Party', 7010.0: 'BA: Party of Democratic Progress', 7011.0: 'BA: Movement of Democratic Action', 7012.0: 'BA: The Alliance of Independent Social Democrats', 7013.0: 'BA: Union for a better future of BiH', 7014.0: 'BA: Social-Democratic Party', 7015.0: 'BA: Serbian Democratic Party', 7016.0: "BA: Democratic People's Alliance", 7017.0: 'BA: Party of Democratic Action', 7018.0: 'BA: National Democratic Movement', 7019.0: 'BA: Independent Bloc', 7031.0: 'BA: Other, please specify (WRITE IN)', 7066.0: 'BA: No [,no other] party appeals to me', 10001.0: 'BG: Citizens for European Development of Bulgaria', 10002.0: 'BG: Bulgarian Socialist Party (BSP)', 10003.0: 'BG: Movement for Rights and Freedoms (MRF)', 10004.0: 'BG: Attack', 10005.0: 'BG: Union of Democratic Forces', 10006.0: 'BG: Democrats for a Strong Bulgaria', 10007.0: 'BG: VMRO – Bulgarian National Movement', 10008.0: 'BG: Political Party VOLYA', 10009.0: 'BG: Alternative for Bulgarian Revival', 10010.0: 'BG: Movement 21', 10011.0: 'BG: DOST Democrats for Responsibility, Freedom, Tolerance', 10012.0: 'BG: Movement Bulgaria of the Citizens', 10013.0: 'BG: Party The Greens', 10014.0: 'BG: Yes, Bulgaria', 10015.0: 'BG: National Front for the Salvation of Bulgaria', 10026.0: 'BG: Other, please specify (WRITE IN)', 10066.0: 'BG: No [no other] party appeals to me (spontaneous)', 11201.0: 'BY: Liberal Democratic Party', 11202.0: 'BY: Belarusian Social Sports Party', 11203.0: 'BY: Belarusian Party “The Greens” (“Zyalyoniya”)', 11204.0: 'BY: Social-Democratic Party of Peoples Concord (Narodnai Zgody)', 11205.0: 'BY: Belarusian Agrarian Party', 11206.0: 'BY: Republican Party', 11207.0: 'BY: Conservative Christian Party – BNF', 11208.0: 'BY: Party BNF', 11209.0: 'BY: Republican Party of Labor and Justice', 11210.0: "BY: Belarusian Party of the Left 'Fair World'", 11211.0: 'BY: United Civic Party', 11212.0: 'BY: Belarusian Patriotic Party', 11213.0: 'BY: Party “Belarusian Social-Democratic Assembly” (Hramada)', 11214.0: 'BY: Belarusian Social-Democratic Party (Assembly) (Hramada)', 11215.0: 'BY: Communist Party of Belarus', 11216.0: 'BY: Supporters of the political policy of the President of the Republic of Belarus A.G.\xa0Lukashenko', 11226.0: 'BY: Other, please specify (WRITE IN)', 11266.0: 'BY: No [,no other] party appeals to me (spontaneous)', 19101.0: 'HR: Bandić Milan 365', 19102.0: 'HR: Croatian democratic union', 19103.0: "HR: Croatian people's party", 19104.0: 'HR: Croatian peasant party', 19105.0: 'HR: Croatian social liberal party', 19106.0: 'HR: Croatian party of rights', 19107.0: 'HR: Croatian party of pensioners', 19108.0: 'HR: Croatian democratic alliance of Slavonia and Baranja', 19109.0: 'HR: Istrian democratic assembly', 19110.0: 'HR: Smart', 19111.0: 'HR: Gorski-Kotar alliance', 19112.0: 'HR: Independent democratic Serbian party', 19113.0: 'HR: Bridge of Independent Lists', 19114.0: 'HR: Social democratic party of Croatia', 19115.0: 'HR: Human shield', 19126.0: 'HR: Other, please specify (WRITE IN)', 19166.0: 'HR: No [, no other] party appeals to me (spontaneous)', 20301.0: 'CZ: ANO', 20302.0: 'CZ: ČSSD (Czech Social Democratic Party)', 20303.0: 'CZ: KSČM (Communist Party of Bohemia and Moravia)', 20304.0: 'CZ: ODS (Civic Democratic Party)', 20305.0: 'CZ: KDU-ČSL (Christian Democratic Party - Czechoslovak Peoples´Party)', 20306.0: 'CZ: TOP 09', 20307.0: 'CZ: Piráti (Pirates)', 20308.0: 'CZ: SPD (Freedom and Direct Democracy- Tomio Okamura)', 20309.0: 'CZ: SZ (Green Party)', 20310.0: 'CZ: STAN (Mayors and Independents)', 20311.0: 'CZ: Úsvit-NK (Dawn- National Coalition)', 20312.0: 'CZ: Svobodní (Party of Free Citizens)', 20313.0: 'CZ: SPO (Party for the Rights of Citizens)', 20314.0: 'CZ: Realisté (Realists)', 20315.0: 'CZ: DSSS (Worker´s Party of Social Justice)', 20316.0: 'CZ: Other', 20366.0: 'CZ: No [no other] party appeals to me (spontaneous)', 20801.0: ' DK: The Social Democrats', 20802.0: ' DK: Danish Social Liberal Party', 20803.0: ' DK: Conservative Peoples Party', 20804.0: ' DK: Socialist Peoples Party', 20805.0: ' DK: Danish Peoples Party', 20806.0: ' DK: The New Right', 20807.0: ' DK: Venstre, Denmarks Liberal Party', 20808.0: ' DK: Liberal Alliance', 20809.0: ' DK: Red-Green Alliance', 20810.0: ' DK: The Alternative', 20811.0: ' DK: Blank vote', 20866.0: ' DK: No [no other] party appeals to me', 23301.0: 'EE: Estonian Centre Party', 23302.0: 'EE: Estonian Reform Party', 23303.0: 'EE: Social Democratic Party', 23304.0: 'EE: Union of Pro Patria and Res Publica', 23305.0: 'EE: Estonian Greens', 23306.0: 'EE: Estonian Conseravative Peoples party', 23307.0: 'EE: Estonian Free Party', 23331.0: 'EE: Other, please specify (WRITE IN)', 23366.0: 'EE: No [no other] party appeals to me (spontaneous)', 24601.0: ' FI: Center party', 24602.0: ' FI: National Coalition Party', 24603.0: ' FI: Social democratic party', 24604.0: ' FI: Left alliance', 24605.0: ' FI: Green league', 24606.0: ' FI: Christian democrats', 24607.0: " FI: Swedish People's Party in Finland", 24608.0: ' FI: Finns Party', 24609.0: ' FI: Other, please specify (WRITE IN)', 24666.0: ' FI: No [no other] party appeals to me', 25001.0: " FR: Left Wing Extremist Parties (New Anticapitalist Party, Workers' Struggle, Independent Workers' Party)", 25002.0: ' FR: Unsubmissive France', 25003.0: ' FR: Communist Party', 25004.0: ' FR: Socialist Party', 25005.0: " FR: Other Left Wing Parties (Radical Leftist Party, Republicain's and Citizen's Movement", 25006.0: ' FR: Europe Ecology – The Greens', 25007.0: ' FR: Other Environmentalist Parties', 25008.0: ' FR: The Republic Onwards', 25009.0: ' FR: Democratic Movement', 25010.0: ' FR: Union of Democrats and Independents', 25011.0: ' FR: Act – The constructive right', 25012.0: ' FR: The Republicans', 25013.0: ' FR: France Arise', 25014.0: ' FR: National Front', 25015.0: ' FR: Other Right Wing Extremist Parties (The Patriots, National Republican Movement)', 25026.0: ' FR: Other, please specify (WRITE IN)', 25066.0: ' FR: No [no other] party appeals to me (spontaneous)', 26801.0: 'GE: United National Movement', 26802.0: 'GE: Georgian Labor Party', 26803.0: 'GE: New Rightists Party', 26804.0: 'GE: Republican Party of Georgia', 26805.0: 'GE: Industry Will Save Georgia', 26806.0: 'GE: United Democratic Movement', 26807.0: 'GE: Alliance of Patriots of Georgia', 26808.0: 'GE: European Georgia', 26809.0: 'GE: Free Democrats', 26810.0: 'GE: Georgian Dream - Democratic Georgia', 26811.0: 'GE: National Forum', 26812.0: 'GE: State for the People', 26813.0: 'GE: New Political Center Girchi', 26814.0: 'GE: New Georgia', 26815.0: 'GE: Development Party', 26826.0: 'GE: Other', 26866.0: 'GE: No [no other] party appeals to me (spontaneous)', 27601.0: 'DE: Christian Democratic Party/Christian Social Union', 27602.0: 'DE: German Social-Democratic Party', 27603.0: 'DE: German Liberal Party', 27604.0: 'DE: The Green Party', 27605.0: 'DE: The Left', 27606.0: 'DE: Alternative for Germany', 27607.0: 'DE: Other, please specify (WRITE IN)', 27666.0: 'DE: No [no other] party appeals to me (spontaneous)', 34801.0: 'HU: Hungarian Socialist Party (MSZP)', 34802.0: 'HU: Fidesz', 34803.0: "HU: Christian Democratic People's Party (KDNP)", 34804.0: 'HU: Movement for a Better Hungary (Jobbik)', 34805.0: 'HU: Politics Can Be Different (LMP)', 34806.0: 'HU: Democratic Coalition (DK)', 34807.0: 'HU: Together – Party for a New Era (Együtt)', 34808.0: 'HU: Dialogue for Hungary (PM)', 34809.0: 'HU: Momentum Movement (Momentum)', 34810.0: 'HU: Hungarian Liberal Party (Liberálisok)', 34811.0: 'HU: Hungarian Two-tailed Dog Party (MKKP)', 34812.0: "HU: Hungarian Workers' Party (Munkáspárt)", 34826.0: 'HU: Other, please specify (WRITE IN)', 34866.0: 'HU: No [no other] party appeals to me (spontaneous)', 35201.0: 'IS: The Independence Party', 35202.0: 'IS: The Progressive Party', 35203.0: 'IS: The Social Democratic Alliance', 35204.0: 'IS: The\xa0Left-Green Movement\xa0', 35205.0: 'IS: Bright Future', 35206.0: 'IS: Pirate Party', 35207.0: 'IS: Reform Party', 35208.0: 'IS: Dawn - The organization of justice, fairness and democracy', 35209.0: "IS: The People's Front of Iceland", 35210.0: 'IS: The\xa0Icelandic National Front', 35211.0: "IS: The People's Party\xa0", 35212.0: 'IS: The Humanist Party', 35216.0: 'IS: Centre Party', 35226.0: 'IS: Other, please specify (WRITE IN)', 35266.0: 'IS: No (no other) party appeals to me', 38001.0: ' IT: Communist Party', 38002.0: ' IT: Power to the People!', 38003.0: ' IT: Free and Equal', 38004.0: ' IT: Italy Europe Together', 38005.0: ' IT: Democratic Party', 38006.0: ' IT: More Europe', 38007.0: ' IT: Popular Civic Lorenzin', 38008.0: ' IT: SVP - PATT', 38009.0: ' IT: Five Star Movement', 38010.0: ' IT: Us with Italy - UDC (Union of the Centre)', 38011.0: ' IT: The People of the Family', 38012.0: ' IT: Go Italy', 38013.0: ' IT: League', 38014.0: ' IT: Brothers of Italy with Giorgia Meloni', 38015.0: ' IT: CasaPound Italy', 38016.0: ' IT: Italy to the Italians', 38031.0: ' IT: Other, please specify (WRITE IN)', 38066.0: ' IT: No [no other] party appeals to me (spontaneous)', 42801.0: ' LV: Harmony', 42802.0: ' LV: KPV LV', 42803.0: ' LV: New Conservative Party', 42804.0: ' LV: Development/For!', 42805.0: ' LV: National Alliance', 42806.0: ' LV: Union of Greens and Farmers', 42807.0: ' LV: New Unity', 42808.0: ' LV: Latvian Association of Regions', 42809.0: ' LV: Russian Union', 42810.0: ' LV: Progressives', 42816.0: ' LV: Other, please specify (WRITE IN)', 42866.0: ' LV: No party appeals to me (spontaneous)', 44001.0: ' LT: Labour Party', 44002.0: ' LT: Lithuanian Centre Party', 44003.0: ' LT: Lithuanian Freedom Union (Liberals)', 44004.0: ' LT: Electoral Action of Poles – Christian Families Aliance', 44005.0: ' LT: Liberal Union of Lithuanian Republic', 44006.0: ' LT: Lithuanian Social Democratic Party', 44007.0: ' LT: Lithuanian Farmers and Greens Union', 44008.0: " LT: Party 'Order and Justice'", 44009.0: " LT: Political Party 'List of Lithuania'", 44010.0: ' LT: Homeland Union: Lithuanian Christian Democrats', 44011.0: ' LT: Lithuanian Green Party', 44012.0: ' LT: Other, please specify (WRITE IN)', 44066.0: ' LT: No [, no other] party appeals to me', 49901.0: ' ME: Democratic party of socialists', 49902.0: ' ME: Socialdemocratic party', 49903.0: ' ME: Socialdemocrats of Montenegro', 49904.0: " ME: Socialist people's party", 49905.0: ' ME: Democratic front', 49906.0: ' ME: Positive Montenegro', 49907.0: ' ME: Liberal party of Montenegro', 49908.0: ' ME: DEMOS', 49909.0: ' ME: United Montenegro', 49910.0: ' ME: Democratic Montenegro', 49911.0: ' ME: United reform action', 49912.0: ' ME: Montenegrin (party)', 49913.0: " ME: Bosniak's party", 49914.0: ' ME: Democratic alliance in Montenegro', 49915.0: ' ME: Democratic union of of Albanians', 49916.0: ' ME: Albanian Alternative', 49917.0: ' ME: FORCA', 49918.0: ' ME: Croatian civic initiative', 49919.0: ' ME: True Montenegro', 49926.0: ' ME: Other, please specify (WRITE IN)', 49966.0: ' ME: No [, no other] party appeals to me', 52801.0: "NL: People's Party for Freedom and Democracy", 52802.0: 'NL: Party for Freedom', 52803.0: 'NL: Christian Democratic Appeal', 52804.0: 'NL: Democrats 66', 52805.0: 'NL: GreenLeft', 52806.0: 'NL: Socialist Party', 52807.0: 'NL: Labour Party', 52808.0: 'NL: Christian Union', 52809.0: 'NL: Party for the Animals', 52810.0: 'NL: 50Plus', 52811.0: 'NL: Reformed Political Party', 52812.0: 'NL: Denk', 52813.0: 'NL: Forum for Democracy', 52814.0: 'NL: Other, please specify (WRITE IN)', 52866.0: 'NL: No [no other] party appeals to me (spontaneous)', 57801.0: ' NO: Labour Party', 57802.0: ' NO: Democrats in Norway', 57803.0: ' NO: Progress Party', 57804.0: ' NO: Conservative Party', 57805.0: ' NO: Christian Democratic Party', 57806.0: ' NO: Coastal Party', 57807.0: ' NO: Green Party', 57808.0: ' NO: The Christians', 57809.0: " NO: Pensioners' Party", 57810.0: ' NO: Red Party', 57811.0: ' NO: Centre Party', 57812.0: ' NO: Socialist Left Party', 57813.0: ' NO: Liberal Party', 57814.0: ' NO: Other, please specify (WRITE IN)', 57866.0: ' NO: No party appeals to me (spontanious)', 61601.0: 'PL: Law and Justice', 61602.0: "PL: Citizens' Platform", 61603.0: "PL: Kukiz'15", 61604.0: 'PL: Modern', 61605.0: "PL: Polish Peasants' Party", 61606.0: 'PL: Democratic Left Alliance', 61607.0: 'PL: Liberty (Korwin)', 61608.0: 'PL: Together Party', 61609.0: 'PL: Other, please specify (WRITE IN)', 61666.0: 'PL: No (no other) party appeals to me', 62001.0: ' PT: Alternative Socialist Movement', 62002.0: ' PT: Left Block', 62003.0: ' PT: Portuguese Communist Party', 62004.0: ' PT: FREE', 62005.0: ' PT: Communist Party of Portuguese Workers/Reorganizing Movement of the Portuguese Proletariat', 62006.0: ' PT: Popular Monarchic Party', 62007.0: ' PT: Socialist Party', 62008.0: ' PT: People–Animals–Nature', 62009.0: ' PT: Social Democratic Party', 62010.0: ' PT: Social Democratic Centre - Popular Party', 62011.0: ' PT: Enough', 62012.0: ' PT: Together for the People', 62013.0: ' PT: Liberal Initiative', 62014.0: ' PT: We, the Citizens', 62015.0: ' PT: National Renewal Party (PNR)', 62016.0: ' PT: Democratic Republican Party', 62017.0: ' PT: Workers Party of Socialist Unity', 62018.0: ' PT: Liberal Democratic Party', 62019.0: ' PT: United Party of Retirees and Pensionists', 62020.0: ' PT: Alliance', 62021.0: ' PT: Citizenship and Christian Democracy', 62022.0: ' PT: Portuguese Labour Party (PTP)', 62023.0: ' PT: Earth Party', 62024.0: ' PT: Other', 62066.0: ' PT: No party appeals to me (spontaneous)', 64201.0: ' RO: The Social-Democratic Party', 64202.0: ' RO: National Liberal Party', 64203.0: " RO: 'Save Romania' Union", 64204.0: ' RO: Democratic Union of Hungarians from Romania', 64205.0: ' RO: Great Romania Party', 64206.0: " RO: 'Aliance of Liberals and Democrats' Party", 64207.0: ' RO: Popular Movement Party', 64226.0: ' RO: Other, please specify (WRITE IN)', 64266.0: ' RO: No [no other] party appeals to me', 64301.0: 'RU: Rodina', 64302.0: 'RU: Communists of Russia', 64303.0: 'RU: Russian Party of Pensioners for Social Justice', 64304.0: 'RU: United Russia', 64305.0: 'RU: Greens', 64306.0: 'RU: Civic platform', 64307.0: 'RU: Liberal Democratic Party of Russia (LDPR)', 64308.0: "RU: People's Freedom Party (PARNAS)", 64309.0: 'RU: Party of Growth', 64310.0: "RU: Citizens' Force (Civilian power)", 64311.0: 'RU: Yabloko', 64312.0: 'RU: Communist Party of Russian Federation (CPRF)', 64313.0: 'RU: Patriots of Russia', 64314.0: 'RU: Just Russia', 64326.0: 'RU: Other, please specify (WRITE IN)', 64366.0: 'RU: No [no other] party appeals to me (spontaneous)', 68801.0: ' RS: Serbian Progressive Party (SNS)', 68802.0: ' RS: Democratic Party (DS)', 68803.0: ' RS: Movement of Free Citizens (PSG)', 68804.0: " RS: People's Party (NS)", 68805.0: ' RS: Democratic Party of Serbia (DSS)', 68806.0: ' RS: Serbian Radical Party (SRS)', 68807.0: ' RS: Social Democratic Party (SDS)', 68808.0: ' RS: New Party (NS)', 68809.0: ' RS: Liberal Democratic Party (LDP)', 68810.0: ' RS: Socialist Party of Serbia (SPS)', 68811.0: ' RS: Dveri', 68812.0: " RS: It's enough (DJB)", 68813.0: ' RS: Party of United Pensioners of Serbia (PUPS)', 68814.0: ' RS: United Serbia (JS)', 68815.0: ' RS: Alliance of Vojvodina Hungarians (SVM)', 68816.0: ' RS: League of Social Democrats of Vojvodina (LSV)', 68817.0: ' RS: Party for Democratic Action (PDD)', 68818.0: ' RS: Roma Party (RP)', 68819.0: ' RS: Party for Democratic Action (SDA)', 68820.0: ' RS: Social Democratic Party of Serbia (SDPS)', 68821.0: ' RS: Democratic League of Croats in Vojvodina (DSHV)', 68831.0: ' RS: Other, please specify (WRITE IN)', 68866.0: ' RS: No [no other] party appeals to me', 70301.0: 'SK: Communist Party of Slovakia', 70302.0: "SK: Kotleba - People's Party Our Slovakia", 70303.0: 'SK: Christian Democratic Movement', 70304.0: 'SK: MOST - HÍD', 70305.0: 'SK: Ordinary People and Independent Personalities', 70306.0: 'SK: NETWORK', 70307.0: 'SK: Freedom and Solidarity', 70308.0: 'SK: Slovak National Party', 70309.0: 'SK: We Are Family - Boris Kollár', 70310.0: 'SK: DIRECTION - Social Democracy', 70311.0: 'SK: The Party of the Hungarian Community', 70312.0: 'SK: Slovak Green Party', 70313.0: 'SK:Other, please specify (WRITE IN)', 70366.0: 'SK: No [no other] party appeals to me (spontaneous)', 70501.0: ' SI: SMC - Party of Modern Center', 70502.0: ' SI: SDS - Slovenian democratic party', 70503.0: ' SI: DESUS - Democratic party of slovenian pensioners', 70504.0: ' SI: SD - Social democrats', 70505.0: ' SI: ZL - United Left', 70506.0: " SI: NSi - New Slovenia - Christian people's party", 70507.0: ' SI: ZAAB - Alliance of Alenka Bratusek', 70508.0: " SI: SLS - Slovene people's party", 70509.0: ' SI: PS - Positive Slovenia', 70510.0: ' SI: SNS - Slovenian national party', 70511.0: 'SI: Other, please specify (WRITE IN)', 70566.0: 'SI: No [no other] party appeals to me (spontaneous)', 72401.0: "ES: Spanish Workers' Socialist Party", 72402.0: 'ES: Popular Party', 72403.0: 'ES: United Left', 72404.0: 'ES: Initiative For Catalonia', 72405.0: 'ES: We Can', 72406.0: 'ES: We can together', 72407.0: 'ES: In Tide', 72408.0: 'ES: Compromise', 72409.0: 'ES: Citizens', 72410.0: 'ES: Catalan Democratic Party', 72411.0: 'ES: Republican Party of Catalonia', 72412.0: 'ES: Basque Nationalist Party', 72413.0: 'ES: Basque Country United', 72414.0: 'ES: Union of Navarrese People', 72415.0: 'ES: Yes to the Future', 72416.0: 'ES: Canary Coalition', 72417.0: 'ES: New Canary Islands', 72418.0: 'ES: Galician Nationalist Block', 72419.0: 'ES: Aragonese Assembly', 72420.0: 'ES: Aragonese Party', 72421.0: 'ES: Asturias Forum', 72426.0: 'ES: Other', 72466.0: 'ES: No [no other] party appeals to me (spontaneous)', 75201.0: ' SE: Social democratic party', 75202.0: ' SE: Moderate party', 75203.0: ' SE: Sweden democrats', 75204.0: ' SE: Center party', 75205.0: ' SE: Liberals', 75206.0: ' SE: Left wing party', 75207.0: ' SE: Green party', 75208.0: ' SE: Christian Democratic Party', 75231.0: ' SE: Other, please specify (WRITE IN)', 75266.0: ' SE: No [no other] party appeals to me (spontaneous)', 75601.0: 'CH: The Liberals (Merge from Radicals and Liberals)', 75602.0: 'CH: Christian Democratic Party', 75603.0: 'CH: Social Democratic Party (socialist)', 75604.0: "CH: Swiss People's Party", 75605.0: 'CH: Conservative Democratic Party', 75606.0: 'CH: Movement of the Citizens of French-speaking Switzerland', 75607.0: 'CH: Swiss Labour Party', 75608.0: 'CH: Green Party', 75609.0: 'CH: Green Liberal Party', 75610.0: 'CH: Pirate Party', 75611.0: 'CH: Federal Democratic Union', 75612.0: "CH: Evangelical People's Party", 75613.0: 'CH: Ticino League', 75614.0: 'CH: The alternative Left', 75626.0: 'CH:Other, please specify (WRITE IN)', 75666.0: 'CH: No (no other) party appeals to me', 80401.0: ' UA: Party «Sluha narodu»', 80402.0: ' UA: Party «Opozytsiina platforma – Za zhyttia»', 80403.0: ' UA: All-Ukrainian association «Batkivshchyna»', 80404.0: ' UA: Party «Yevropeiska solidarnist»', 80405.0: ' UA: Party «Holos»', 80406.0: ' UA: Oleh Liashko’s Radical party', 80407.0: ' UA: Party «Syla i chest»', 80408.0: ' UA: Partiia «Opozytsiinyi blok»', 80409.0: ' UA: Party «Hroisman’s Ukrainian Strategy»', 80410.0: ' UA: Shariy’s Party', 80411.0: ' UA: All-Ukrainian association «Svoboda»', 80412.0: ' UA: Other, please specify (WRITE IN)', 80466.0: ' UA: No party appeals to me (spontaneous)', 80701.0: 'MK: IMRO - DPMNU Internal Macedonian Revolutionary Organization - Democratic Party for Macedonian National Unity', 80702.0: 'MK: Civil Option For Macedonia', 80703.0: 'MK: Movement Besa', 80704.0: 'MK: Democratic Renewal of Macedonia', 80705.0: 'MK: DPA (Democratic Party of Albanians)', 80706.0: 'MK: DPSM (Democratic Party of Serbs in Macedonia)', 80707.0: 'MK: DPTM (Democratic Party of Turcs In Macedonia)', 80708.0: 'MK: DUI (Democratic Unioun for Integration)', 80709.0: 'MK: “Alliance of Albanians” Coalition (DR-ADP, UNITETI, NDP)', 80710.0: 'MK: LDP (Liberal Democratic Party)', 80711.0: 'MK: NSDP (New Social-Democratic Party)', 80712.0: 'MK: PDT (Party for Movement of the Turks in Macedonia)', 80713.0: 'MK: PEF (Party for European Future)', 80714.0: 'MK: POPGM (Party of United Pensioners and Citizens of Macedonia)', 80715.0: 'MK: RAM (Roma Aliance of Macedonia)', 80716.0: 'MK: SDAM (Social-Democratic Aliance of Macedonia)', 80717.0: 'MK: SPM (Socialistic Party of Macedonia)', 80718.0: 'MK: SPM (Serbian Party of Macedonia)', 80719.0: 'MK: Levica', 80731.0: 'MK: Other, please specify (WRITE IN):', 80766.0: 'MK: No (other) party appeals to me (spontaneous)', 82601.0: ' GB: Conservative', 82602.0: ' GB: Labour', 82603.0: ' GB: Liberal Democrat', 82604.0: ' GB: Scottish National Party', 82605.0: ' GB: Plaid Cymru', 82606.0: ' GB: Green Party', 82607.0: ' GB: UK Independence Party (UKIP)', 82608.0: ' GB: British National Party (BNP)/ National Front', 82609.0: ' GB: Other party (WRITE IN)', 82666.0: ' GB: No [no other] party appeals to me (spontaneous)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47229 | 508 |
v175_LR | 244 | v175_LR | nominal | political party: another appeals to you: left/right scale (Q49a) | {1.0: 'left', 2.0: '', 3.0: '', 4.0: '', 5.0: '', 6.0: '', 7.0: '', 8.0: '', 9.0: '', 10.0: 'right', 44.0: 'not classifiable'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 23004 | 11 |
v175_cs | 245 | v175_cs | numeric | is there another party that appeals to you (Q49a) (ISO 3166-1, country-specific) | {801.0: ' AL: Democratic Party, PD', 802.0: ' AL: Socialist Party', 803.0: ' AL: Socialist Movement for Integration', 804.0: ' AL: The Human Rights Union Party', 805.0: ' AL: Christian Democrats', 806.0: ' AL: Republican Party', 807.0: ' AL: Social Democracy Party', 808.0: ' AL: Movement of Legality Party', 809.0: ' AL: National Front Party', 810.0: ' AL: New Democrat Party', 811.0: ' AL: Justice, Integration and Unity Party', 812.0: ' AL: Challenge for Albania', 813.0: ' AL: Social Democratic Party', 814.0: ' AL: Environmental Party', 815.0: ' AL: Albanian Democratic Union Party', 816.0: ' AL: Liberal Democratic Union Party', 817.0: ' AL: Agrarian Environmentalist Party', 818.0: " AL: Albanian Workers' Party", 819.0: ' AL: Communist Party of Albania', 820.0: " AL: The People's Alliance Party", 821.0: ' AL: Equal List, LIBRA', 822.0: ' AL: Green Party', 823.0: ' AL: Albanian Republican Union Party', 824.0: ' AL: Moderate Socialist Party', 825.0: ' AL: Party of People with Disabilities', 826.0: ' AL: Other, please specify (WRITE IN)', 866.0: ' AL: No [no other] party appeals to me', 877.0: ' AL: not applicable', 888.0: ' AL: don’t know (spontaneous)', 899.0: ' AL: no answer (spontaneous)', 3101.0: ' AZ: New Azerbaijan Party', 3102.0: ' AZ: Musavat/Equality Party', 3103.0: ' AZ: Azerbaijan Democratic Party', 3104.0: ' AZ: Popular Front Party', 3105.0: ' AZ: National Independence Party', 3106.0: ' AZ: Azerbaijan Liberal Party', 3107.0: ' AZ: Social-Democratic Party', 3108.0: ' AZ: Communist Party', 3109.0: ' AZ: Justice Party', 3110.0: ' AZ: Civic Solidarity Party', 3111.0: ' AZ: Motherland Party', 3112.0: ' AZ: Azerbaijan Party of Hope', 3113.0: ' AZ: Social Prosperity Party', 3114.0: ' AZ: Azerbaijan Party of Democratic Reform', 3115.0: ' AZ: Whole Azerbaijan Popular Front Party', 3116.0: ' AZ: Great Order Party', 3117.0: ' AZ: United Communist Party', 3118.0: ' AZ: Party of Unity', 3119.0: ' AZ: Modern Musavat Party', 3131.0: ' AZ: Other, please specify (WRITE IN)', 3166.0: ' AZ: No [, no other] party appeals to me (spontaneous)', 4001.0: 'AT: Social Democratic Party of Austria - SPÖ', 4002.0: "AT: List Kurz / Austrian People's Party - ÖVP", 4003.0: 'AT: Freedom Party of Austria - FPÖ', 4004.0: 'AT: The Greens - The Green Alternative', 4005.0: 'AT: The new Austria - NEOS', 4006.0: 'AT: List Peter Pilz', 4026.0: 'AT: Other, please specify (WRITE IN)', 4066.0: 'AT: No [no other] party appeals to me (spontaneous)', 5101.0: 'AM: Armenian Revolutionary Federation', 5102.0: 'AM: Armenian Communist Party', 5103.0: "AM: 'Exit' (Way out) Party Union", 5104.0: 'AM: Free Democrats Party', 5105.0: 'AM: Democratic Party of Armenia', 5106.0: 'AM: Heritage Party', 5107.0: 'AM: Armenian National Congress (former All-Armenian Movement)', 5108.0: 'AM: People’s Party of Armenia', 5109.0: 'AM: Tsarukyan Block (Prosperous Armenia Party)', 5110.0: 'AM: Republican Party of Armenia', 5111.0: "AM: 'Apricot`s country' Party", 5112.0: 'AM: Armenian Renaissance Party (Former Rule of Law)', 5126.0: 'AM: Other, please specify (WRITE IN)', 5166.0: 'AM: No [no other] party appeals to me (spontaneous)', 7001.0: 'BA: Bosnian Party', 7002.0: 'BA: Democratic Front', 7003.0: 'BA: Croatian Democratic Union - 1990', 7004.0: 'BA: Croatian Democratic Union', 7005.0: 'BA: Croatian republican party', 7006.0: 'BA: Croatian Party of Rights', 7007.0: 'BA: Liberal Democratic Party', 7008.0: 'BA: People and Justice', 7009.0: 'BA: Our Party', 7010.0: 'BA: Party of Democratic Progress', 7011.0: 'BA: Movement of Democratic Action', 7012.0: 'BA: The Alliance of Independent Social Democrats', 7013.0: 'BA: Union for a better future of BiH', 7014.0: 'BA: Social-Democratic Party', 7015.0: 'BA: Serbian Democratic Party', 7016.0: "BA: Democratic People's Alliance", 7017.0: 'BA: Party of Democratic Action', 7018.0: 'BA: National Democratic Movement', 7019.0: 'BA: Independent Bloc', 7031.0: 'BA: Other, please specify (WRITE IN)', 7066.0: 'BA: No [,no other] party appeals to me', 10001.0: 'BG: Citizens for European Development of Bulgaria', 10002.0: 'BG: Bulgarian Socialist Party (BSP)', 10003.0: 'BG: Movement for Rights and Freedoms (MRF)', 10004.0: 'BG: Attack', 10005.0: 'BG: Union of Democratic Forces', 10006.0: 'BG: Democrats for a Strong Bulgaria', 10007.0: 'BG: VMRO – Bulgarian National Movement', 10008.0: 'BG: Political Party VOLYA', 10009.0: 'BG: Alternative for Bulgarian Revival', 10010.0: 'BG: Movement 21', 10011.0: 'BG: DOST Democrats for Responsibility, Freedom, Tolerance', 10012.0: 'BG: Movement Bulgaria of the Citizens', 10013.0: 'BG: Party The Greens', 10014.0: 'BG: Yes, Bulgaria', 10015.0: 'BG: National Front for the Salvation of Bulgaria', 10026.0: 'BG: Other, please specify (WRITE IN)', 10066.0: 'BG: No [no other] party appeals to me (spontaneous)', 11201.0: 'BY: Liberal Democratic Party', 11202.0: 'BY: Belarusian Social Sports Party', 11203.0: 'BY: Belarusian Party “The Greens” (“Zyalyoniya”)', 11204.0: 'BY: Social-Democratic Party of Peoples Concord (Narodnai Zgody)', 11205.0: 'BY: Belarusian Agrarian Party', 11206.0: 'BY: Republican Party', 11207.0: 'BY: Conservative Christian Party – BNF', 11208.0: 'BY: Party BNF', 11209.0: 'BY: Republican Party of Labor and Justice', 11210.0: "BY: Belarusian Party of the Left 'Fair World'", 11211.0: 'BY: United Civic Party', 11212.0: 'BY: Belarusian Patriotic Party', 11213.0: 'BY: Party “Belarusian Social-Democratic Assembly” (Hramada)', 11214.0: 'BY: Belarusian Social-Democratic Party (Assembly) (Hramada)', 11215.0: 'BY: Communist Party of Belarus', 11216.0: 'BY: Supporters of the political policy of the President of the Republic of Belarus A.G.\xa0Lukashenko', 11226.0: 'BY: Other, please specify (WRITE IN)', 11266.0: 'BY: No [,no other] party appeals to me (spontaneous)', 19101.0: 'HR: Bandić Milan 365', 19102.0: 'HR: Croatian democratic union', 19103.0: "HR: Croatian people's party", 19104.0: 'HR: Croatian peasant party', 19105.0: 'HR: Croatian social liberal party', 19106.0: 'HR: Croatian party of rights', 19107.0: 'HR: Croatian party of pensioners', 19108.0: 'HR: Croatian democratic alliance of Slavonia and Baranja', 19109.0: 'HR: Istrian democratic assembly', 19110.0: 'HR: Smart', 19111.0: 'HR: Gorski-Kotar alliance', 19112.0: 'HR: Independent democratic Serbian party', 19113.0: 'HR: Bridge of Independent Lists', 19114.0: 'HR: Social democratic party of Croatia', 19115.0: 'HR: Human shield', 19126.0: 'HR: Other, please specify (WRITE IN)', 19166.0: 'HR: No [, no other] party appeals to me (spontaneous)', 20301.0: 'CZ: ANO', 20302.0: 'CZ: ČSSD (Czech Social Democratic Party)', 20303.0: 'CZ: KSČM (Communist Party of Bohemia and Moravia)', 20304.0: 'CZ: ODS (Civic Democratic Party)', 20305.0: 'CZ: KDU-ČSL (Christian Democratic Party - Czechoslovak Peoples´Party)', 20306.0: 'CZ: TOP 09', 20307.0: 'CZ: Piráti (Pirates)', 20308.0: 'CZ: SPD (Freedom and Direct Democracy- Tomio Okamura)', 20309.0: 'CZ: SZ (Green Party)', 20310.0: 'CZ: STAN (Mayors and Independents)', 20311.0: 'CZ: Úsvit-NK (Dawn- National Coalition)', 20312.0: 'CZ: Svobodní (Party of Free Citizens)', 20313.0: 'CZ: SPO (Party for the Rights of Citizens)', 20314.0: 'CZ: Realisté (Realists)', 20315.0: 'CZ: DSSS (Worker´s Party of Social Justice)', 20316.0: 'CZ: Other', 20366.0: 'CZ: No [no other] party appeals to me (spontaneous)', 20801.0: ' DK: The Social Democrats', 20802.0: ' DK: Danish Social Liberal Party', 20803.0: ' DK: Conservative Peoples Party', 20804.0: ' DK: Socialist Peoples Party', 20805.0: ' DK: Danish Peoples Party', 20806.0: ' DK: The New Right', 20807.0: ' DK: Venstre, Denmarks Liberal Party', 20808.0: ' DK: Liberal Alliance', 20809.0: ' DK: Red-Green Alliance', 20810.0: ' DK: The Alternative', 20811.0: ' DK: Blank vote', 20866.0: ' DK: No [no other] party appeals to me', 23301.0: 'EE: Estonian Centre Party', 23302.0: 'EE: Estonian Reform Party', 23303.0: 'EE: Social Democratic Party', 23304.0: 'EE: Union of Pro Patria and Res Publica', 23305.0: 'EE: Estonian Greens', 23306.0: 'EE: Estonian Conseravative Peoples party', 23307.0: 'EE: Estonian Free Party', 23331.0: 'EE: Other, please specify (WRITE IN)', 23366.0: 'EE: No [no other] party appeals to me (spontaneous)', 24601.0: ' FI: Center party', 24602.0: ' FI: National Coalition Party', 24603.0: ' FI: Social democratic party', 24604.0: ' FI: Left alliance', 24605.0: ' FI: Green league', 24606.0: ' FI: Christian democrats', 24607.0: " FI: Swedish People's Party in Finland", 24608.0: ' FI: Finns Party', 24609.0: ' FI: Other, please specify (WRITE IN)', 24666.0: ' FI: No [no other] party appeals to me', 25001.0: " FR: Left Wing Extremist Parties (New Anticapitalist Party, Workers' Struggle, Independent Workers' Party)", 25002.0: ' FR: Unsubmissive France', 25003.0: ' FR: Communist Party', 25004.0: ' FR: Socialist Party', 25005.0: " FR: Other Left Wing Parties (Radical Leftist Party, Republicain's and Citizen's Movement", 25006.0: ' FR: Europe Ecology – The Greens', 25007.0: ' FR: Other Environmentalist Parties', 25008.0: ' FR: The Republic Onwards', 25009.0: ' FR: Democratic Movement', 25010.0: ' FR: Union of Democrats and Independents', 25011.0: ' FR: Act – The constructive right', 25012.0: ' FR: The Republicans', 25013.0: ' FR: France Arise', 25014.0: ' FR: National Front', 25015.0: ' FR: Other Right Wing Extremist Parties (The Patriots, National Republican Movement)', 25026.0: ' FR: Other, please specify (WRITE IN)', 25066.0: ' FR: No [no other] party appeals to me (spontaneous)', 26801.0: 'GE: United National Movement', 26802.0: 'GE: Georgian Labor Party', 26803.0: 'GE: New Rightists Party', 26804.0: 'GE: Republican Party of Georgia', 26805.0: 'GE: Industry Will Save Georgia', 26806.0: 'GE: United Democratic Movement', 26807.0: 'GE: Alliance of Patriots of Georgia', 26808.0: 'GE: European Georgia', 26809.0: 'GE: Free Democrats', 26810.0: 'GE: Georgian Dream - Democratic Georgia', 26811.0: 'GE: National Forum', 26812.0: 'GE: State for the People', 26813.0: 'GE: New Political Center Girchi', 26814.0: 'GE: New Georgia', 26815.0: 'GE: Development Party', 26826.0: 'GE: Other', 26866.0: 'GE: No [no other] party appeals to me (spontaneous)', 27601.0: 'DE: Christian Democratic Party/Christian Social Union', 27602.0: 'DE: German Social-Democratic Party', 27603.0: 'DE: German Liberal Party', 27604.0: 'DE: The Green Party', 27605.0: 'DE: The Left', 27606.0: 'DE: Alternative for Germany', 27607.0: 'DE: Other, please specify (WRITE IN)', 27666.0: 'DE: No [no other] party appeals to me (spontaneous)', 34801.0: 'HU: Hungarian Socialist Party (MSZP)', 34802.0: 'HU: Fidesz', 34803.0: "HU: Christian Democratic People's Party (KDNP)", 34804.0: 'HU: Movement for a Better Hungary (Jobbik)', 34805.0: 'HU: Politics Can Be Different (LMP)', 34806.0: 'HU: Democratic Coalition (DK)', 34807.0: 'HU: Together – Party for a New Era (Együtt)', 34808.0: 'HU: Dialogue for Hungary (PM)', 34809.0: 'HU: Momentum Movement (Momentum)', 34810.0: 'HU: Hungarian Liberal Party (Liberálisok)', 34811.0: 'HU: Hungarian Two-tailed Dog Party (MKKP)', 34812.0: "HU: Hungarian Workers' Party (Munkáspárt)", 34826.0: 'HU: Other, please specify (WRITE IN)', 34866.0: 'HU: No [no other] party appeals to me (spontaneous)', 35201.0: 'IS: The Independence Party', 35202.0: 'IS: The Progressive Party', 35203.0: 'IS: The Social Democratic Alliance', 35204.0: 'IS: The\xa0Left-Green Movement\xa0', 35205.0: 'IS: Bright Future', 35206.0: 'IS: Pirate Party', 35207.0: 'IS: Reform Party', 35208.0: 'IS: Dawn - The organization of justice, fairness and democracy', 35209.0: "IS: The People's Front of Iceland", 35210.0: 'IS: The\xa0Icelandic National Front', 35211.0: "IS: The People's Party\xa0", 35212.0: 'IS: The Humanist Party', 35216.0: 'IS: Centre Party', 35226.0: 'IS: Other, please specify (WRITE IN)', 35266.0: 'IS: No (no other) party appeals to me', 38001.0: ' IT: Communist Party', 38002.0: ' IT: Power to the People!', 38003.0: ' IT: Free and Equal', 38004.0: ' IT: Italy Europe Together', 38005.0: ' IT: Democratic Party', 38006.0: ' IT: More Europe', 38007.0: ' IT: Popular Civic Lorenzin', 38008.0: ' IT: SVP - PATT', 38009.0: ' IT: Five Star Movement', 38010.0: ' IT: Us with Italy - UDC (Union of the Centre)', 38011.0: ' IT: The People of the Family', 38012.0: ' IT: Go Italy', 38013.0: ' IT: League', 38014.0: ' IT: Brothers of Italy with Giorgia Meloni', 38015.0: ' IT: CasaPound Italy', 38016.0: ' IT: Italy to the Italians', 38031.0: ' IT: Other, please specify (WRITE IN)', 38066.0: ' IT: No [no other] party appeals to me (spontaneous)', 42801.0: ' LV: Harmony', 42802.0: ' LV: KPV LV', 42803.0: ' LV: New Conservative Party', 42804.0: ' LV: Development/For!', 42805.0: ' LV: National Alliance', 42806.0: ' LV: Union of Greens and Farmers', 42807.0: ' LV: New Unity', 42808.0: ' LV: Latvian Association of Regions', 42809.0: ' LV: Russian Union', 42810.0: ' LV: Progressives', 42816.0: ' LV: Other, please specify (WRITE IN)', 42866.0: ' LV: No party appeals to me (spontaneous)', 44001.0: ' LT: Labour Party', 44002.0: ' LT: Lithuanian Centre Party', 44003.0: ' LT: Lithuanian Freedom Union (Liberals)', 44004.0: ' LT: Electoral Action of Poles – Christian Families Aliance', 44005.0: ' LT: Liberal Union of Lithuanian Republic', 44006.0: ' LT: Lithuanian Social Democratic Party', 44007.0: ' LT: Lithuanian Farmers and Greens Union', 44008.0: " LT: Party 'Order and Justice'", 44009.0: " LT: Political Party 'List of Lithuania'", 44010.0: ' LT: Homeland Union: Lithuanian Christian Democrats', 44011.0: ' LT: Lithuanian Green Party', 44012.0: ' LT: Other, please specify (WRITE IN)', 44066.0: ' LT: No [, no other] party appeals to me', 49901.0: ' ME: Democratic party of socialists', 49902.0: ' ME: Socialdemocratic party', 49903.0: ' ME: Socialdemocrats of Montenegro', 49904.0: " ME: Socialist people's party", 49905.0: ' ME: Democratic front', 49906.0: ' ME: Positive Montenegro', 49907.0: ' ME: Liberal party of Montenegro', 49908.0: ' ME: DEMOS', 49909.0: ' ME: United Montenegro', 49910.0: ' ME: Democratic Montenegro', 49911.0: ' ME: United reform action', 49912.0: ' ME: Montenegrin (party)', 49913.0: " ME: Bosniak's party", 49914.0: ' ME: Democratic alliance in Montenegro', 49915.0: ' ME: Democratic union of of Albanians', 49916.0: ' ME: Albanian Alternative', 49917.0: ' ME: FORCA', 49918.0: ' ME: Croatian civic initiative', 49919.0: ' ME: True Montenegro', 49926.0: ' ME: Other, please specify (WRITE IN)', 49966.0: ' ME: No [, no other] party appeals to me', 52801.0: "NL: People's Party for Freedom and Democracy", 52802.0: 'NL: Party for Freedom', 52803.0: 'NL: Christian Democratic Appeal', 52804.0: 'NL: Democrats 66', 52805.0: 'NL: GreenLeft', 52806.0: 'NL: Socialist Party', 52807.0: 'NL: Labour Party', 52808.0: 'NL: Christian Union', 52809.0: 'NL: Party for the Animals', 52810.0: 'NL: 50Plus', 52811.0: 'NL: Reformed Political Party', 52812.0: 'NL: Denk', 52813.0: 'NL: Forum for Democracy', 52814.0: 'NL: Other, please specify (WRITE IN)', 52866.0: 'NL: No [no other] party appeals to me (spontaneous)', 57801.0: ' NO: Labour Party', 57802.0: ' NO: Democrats in Norway', 57803.0: ' NO: Progress Party', 57804.0: ' NO: Conservative Party', 57805.0: ' NO: Christian Democratic Party', 57806.0: ' NO: Coastal Party', 57807.0: ' NO: Green Party', 57808.0: ' NO: The Christians', 57809.0: " NO: Pensioners' Party", 57810.0: ' NO: Red Party', 57811.0: ' NO: Centre Party', 57812.0: ' NO: Socialist Left Party', 57813.0: ' NO: Liberal Party', 57814.0: ' NO: Other, please specify (WRITE IN)', 57866.0: ' NO: No party appeals to me (spontanious)', 61601.0: 'PL: Law and Justice', 61602.0: "PL: Citizens' Platform", 61603.0: "PL: Kukiz'15", 61604.0: 'PL: Modern', 61605.0: "PL: Polish Peasants' Party", 61606.0: 'PL: Democratic Left Alliance', 61607.0: 'PL: Liberty (Korwin)', 61608.0: 'PL: Together Party', 61609.0: 'PL: Other, please specify (WRITE IN)', 61666.0: 'PL: No (no other) party appeals to me', 62001.0: ' PT: Alternative Socialist Movement', 62002.0: ' PT: Left Block', 62003.0: ' PT: Portuguese Communist Party', 62004.0: ' PT: FREE', 62005.0: ' PT: Communist Party of Portuguese Workers/Reorganizing Movement of the Portuguese Proletariat', 62006.0: ' PT: Popular Monarchic Party', 62007.0: ' PT: Socialist Party', 62008.0: ' PT: People–Animals–Nature', 62009.0: ' PT: Social Democratic Party', 62010.0: ' PT: Social Democratic Centre - Popular Party', 62011.0: ' PT: Enough', 62012.0: ' PT: Together for the People', 62013.0: ' PT: Liberal Initiative', 62014.0: ' PT: We, the Citizens', 62015.0: ' PT: National Renewal Party (PNR)', 62016.0: ' PT: Democratic Republican Party', 62017.0: ' PT: Workers Party of Socialist Unity', 62018.0: ' PT: Liberal Democratic Party', 62019.0: ' PT: United Party of Retirees and Pensionists', 62020.0: ' PT: Alliance', 62021.0: ' PT: Citizenship and Christian Democracy', 62022.0: ' PT: Portuguese Labour Party (PTP)', 62023.0: ' PT: Earth Party', 62024.0: ' PT: Other', 62066.0: ' PT: No party appeals to me (spontaneous)', 64201.0: ' RO: The Social-Democratic Party', 64202.0: ' RO: National Liberal Party', 64203.0: " RO: 'Save Romania' Union", 64204.0: ' RO: Democratic Union of Hungarians from Romania', 64205.0: ' RO: Great Romania Party', 64206.0: " RO: 'Aliance of Liberals and Democrats' Party", 64207.0: ' RO: Popular Movement Party', 64226.0: ' RO: Other, please specify (WRITE IN)', 64266.0: ' RO: No [no other] party appeals to me', 64301.0: 'RU: Rodina', 64302.0: 'RU: Communists of Russia', 64303.0: 'RU: Russian Party of Pensioners for Social Justice', 64304.0: 'RU: United Russia', 64305.0: 'RU: Greens', 64306.0: 'RU: Civic platform', 64307.0: 'RU: Liberal Democratic Party of Russia (LDPR)', 64308.0: "RU: People's Freedom Party (PARNAS)", 64309.0: 'RU: Party of Growth', 64310.0: "RU: Citizens' Force (Civilian power)", 64311.0: 'RU: Yabloko', 64312.0: 'RU: Communist Party of Russian Federation (CPRF)', 64313.0: 'RU: Patriots of Russia', 64314.0: 'RU: Just Russia', 64326.0: 'RU: Other, please specify (WRITE IN)', 64366.0: 'RU: No [no other] party appeals to me (spontaneous)', 68801.0: ' RS: Serbian Progressive Party (SNS)', 68802.0: ' RS: Democratic Party (DS)', 68803.0: ' RS: Movement of Free Citizens (PSG)', 68804.0: " RS: People's Party (NS)", 68805.0: ' RS: Democratic Party of Serbia (DSS)', 68806.0: ' RS: Serbian Radical Party (SRS)', 68807.0: ' RS: Social Democratic Party (SDS)', 68808.0: ' RS: New Party (NS)', 68809.0: ' RS: Liberal Democratic Party (LDP)', 68810.0: ' RS: Socialist Party of Serbia (SPS)', 68811.0: ' RS: Dveri', 68812.0: " RS: It's enough (DJB)", 68813.0: ' RS: Party of United Pensioners of Serbia (PUPS)', 68814.0: ' RS: United Serbia (JS)', 68815.0: ' RS: Alliance of Vojvodina Hungarians (SVM)', 68816.0: ' RS: League of Social Democrats of Vojvodina (LSV)', 68817.0: ' RS: Party for Democratic Action (PDD)', 68818.0: ' RS: Roma Party (RP)', 68819.0: ' RS: Party for Democratic Action (SDA)', 68820.0: ' RS: Social Democratic Party of Serbia (SDPS)', 68821.0: ' RS: Democratic League of Croats in Vojvodina (DSHV)', 68831.0: ' RS: Other, please specify (WRITE IN)', 68866.0: ' RS: No [no other] party appeals to me', 70301.0: 'SK: Communist Party of Slovakia', 70302.0: "SK: Kotleba - People's Party Our Slovakia", 70303.0: 'SK: Christian Democratic Movement', 70304.0: 'SK: MOST - HÍD', 70305.0: 'SK: Ordinary People and Independent Personalities', 70306.0: 'SK: NETWORK', 70307.0: 'SK: Freedom and Solidarity', 70308.0: 'SK: Slovak National Party', 70309.0: 'SK: We Are Family - Boris Kollár', 70310.0: 'SK: DIRECTION - Social Democracy', 70311.0: 'SK: The Party of the Hungarian Community', 70312.0: 'SK: Slovak Green Party', 70313.0: 'SK:Other, please specify (WRITE IN)', 70366.0: 'SK: No [no other] party appeals to me (spontaneous)', 70501.0: ' SI: SMC - Party of Modern Center', 70502.0: ' SI: SDS - Slovenian democratic party', 70503.0: ' SI: DESUS - Democratic party of slovenian pensioners', 70504.0: ' SI: SD - Social democrats', 70505.0: ' SI: ZL - United Left', 70506.0: " SI: NSi - New Slovenia - Christian people's party", 70507.0: ' SI: ZAAB - Alliance of Alenka Bratusek', 70508.0: " SI: SLS - Slovene people's party", 70509.0: ' SI: PS - Positive Slovenia', 70510.0: ' SI: SNS - Slovenian national party', 70511.0: 'SI: Other, please specify (WRITE IN)', 70566.0: 'SI: No [no other] party appeals to me (spontaneous)', 72401.0: "ES: Spanish Workers' Socialist Party", 72402.0: 'ES: Popular Party', 72403.0: 'ES: United Left', 72404.0: 'ES: Initiative For Catalonia', 72405.0: 'ES: We Can', 72406.0: 'ES: We can together', 72407.0: 'ES: In Tide', 72408.0: 'ES: Compromise', 72409.0: 'ES: Citizens', 72410.0: 'ES: Catalan Democratic Party', 72411.0: 'ES: Republican Party of Catalonia', 72412.0: 'ES: Basque Nationalist Party', 72413.0: 'ES: Basque Country United', 72414.0: 'ES: Union of Navarrese People', 72415.0: 'ES: Yes to the Future', 72416.0: 'ES: Canary Coalition', 72417.0: 'ES: New Canary Islands', 72418.0: 'ES: Galician Nationalist Block', 72419.0: 'ES: Aragonese Assembly', 72420.0: 'ES: Aragonese Party', 72421.0: 'ES: Asturias Forum', 72426.0: 'ES: Other', 72466.0: 'ES: No [no other] party appeals to me (spontaneous)', 75201.0: ' SE: Social democratic party', 75202.0: ' SE: Moderate party', 75203.0: ' SE: Sweden democrats', 75204.0: ' SE: Center party', 75205.0: ' SE: Liberals', 75206.0: ' SE: Left wing party', 75207.0: ' SE: Green party', 75208.0: ' SE: Christian Democratic Party', 75231.0: ' SE: Other, please specify (WRITE IN)', 75266.0: ' SE: No [no other] party appeals to me (spontaneous)', 75601.0: 'CH: The Liberals (Merge from Radicals and Liberals)', 75602.0: 'CH: Christian Democratic Party', 75603.0: 'CH: Social Democratic Party (socialist)', 75604.0: "CH: Swiss People's Party", 75605.0: 'CH: Conservative Democratic Party', 75606.0: 'CH: Movement of the Citizens of French-speaking Switzerland', 75607.0: 'CH: Swiss Labour Party', 75608.0: 'CH: Green Party', 75609.0: 'CH: Green Liberal Party', 75610.0: 'CH: Pirate Party', 75611.0: 'CH: Federal Democratic Union', 75612.0: "CH: Evangelical People's Party", 75613.0: 'CH: Ticino League', 75614.0: 'CH: The alternative Left', 75626.0: 'CH:Other, please specify (WRITE IN)', 75666.0: 'CH: No (no other) party appeals to me', 80401.0: ' UA: Party «Sluha narodu»', 80402.0: ' UA: Party «Opozytsiina platforma – Za zhyttia»', 80403.0: ' UA: All-Ukrainian association «Batkivshchyna»', 80404.0: ' UA: Party «Yevropeiska solidarnist»', 80405.0: ' UA: Party «Holos»', 80406.0: ' UA: Oleh Liashko’s Radical party', 80407.0: ' UA: Party «Syla i chest»', 80408.0: ' UA: Partiia «Opozytsiinyi blok»', 80409.0: ' UA: Party «Hroisman’s Ukrainian Strategy»', 80410.0: ' UA: Shariy’s Party', 80411.0: ' UA: All-Ukrainian association «Svoboda»', 80412.0: ' UA: Other, please specify (WRITE IN)', 80466.0: ' UA: No party appeals to me (spontaneous)', 80701.0: 'MK: IMRO - DPMNU Internal Macedonian Revolutionary Organization - Democratic Party for Macedonian National Unity', 80702.0: 'MK: Civil Option For Macedonia', 80703.0: 'MK: Movement Besa', 80704.0: 'MK: Democratic Renewal of Macedonia', 80705.0: 'MK: DPA (Democratic Party of Albanians)', 80706.0: 'MK: DPSM (Democratic Party of Serbs in Macedonia)', 80707.0: 'MK: DPTM (Democratic Party of Turcs In Macedonia)', 80708.0: 'MK: DUI (Democratic Unioun for Integration)', 80709.0: 'MK: “Alliance of Albanians” Coalition (DR-ADP, UNITETI, NDP)', 80710.0: 'MK: LDP (Liberal Democratic Party)', 80711.0: 'MK: NSDP (New Social-Democratic Party)', 80712.0: 'MK: PDT (Party for Movement of the Turks in Macedonia)', 80713.0: 'MK: PEF (Party for European Future)', 80714.0: 'MK: POPGM (Party of United Pensioners and Citizens of Macedonia)', 80715.0: 'MK: RAM (Roma Aliance of Macedonia)', 80716.0: 'MK: SDAM (Social-Democratic Aliance of Macedonia)', 80717.0: 'MK: SPM (Socialistic Party of Macedonia)', 80718.0: 'MK: SPM (Serbian Party of Macedonia)', 80719.0: 'MK: Levica', 80731.0: 'MK: Other, please specify (WRITE IN):', 80766.0: 'MK: No (other) party appeals to me (spontaneous)', 82601.0: ' GB: Conservative', 82602.0: ' GB: Labour', 82603.0: ' GB: Liberal Democrat', 82604.0: ' GB: Scottish National Party', 82605.0: ' GB: Plaid Cymru', 82606.0: ' GB: Green Party', 82607.0: ' GB: UK Independence Party (UKIP)', 82608.0: ' GB: British National Party (BNP)/ National Front', 82609.0: ' GB: Other party (WRITE IN)', 82666.0: ' GB: No [no other] party appeals to me (spontaneous)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35218 | 498 |
v176 | 246 | v176 | numeric | how often in country's elections: votes are counted fairly (Q50A) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52270 | 4 |
v176_DK | 247 | v176_DK | numeric | DK MODIFIED (cat 4 dropped): how often in country's elections: votes are counted fairly (Q50A) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1618 | 3 |
v177 | 248 | v177 | numeric | how often in country's elections: opposition candidates are prevented from running (Q50B) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47740 | 4 |
v177_DK | 249 | v177_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: opposition candidates are prevented from running (Q50B) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1469 | 3 |
v178 | 250 | v178 | numeric | how often in country's elections: TV news favors the governing party (Q50C) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51081 | 4 |
v178_DK | 251 | v178_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: TV news favors the governing party (Q50C) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1460 | 3 |
v179 | 252 | v179 | numeric | how often in country's elections: voters are bribed (Q50D) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49897 | 4 |
v179_DK | 253 | v179_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: voters are bribed (Q50D) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1473 | 3 |
v180 | 254 | v180 | numeric | how often in country's elections: journalists provide fair coverage of elections (Q50E) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51685 | 4 |
v180_DK | 255 | v180_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: journalists provide fair coverage of elections (Q50E) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1571 | 3 |
v181 | 256 | v181 | numeric | how often in country's elections: election officials are fair (Q50F) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50664 | 4 |
v181_DK | 257 | v181_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: election officials are fair (Q50F) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1551 | 3 |
v182 | 258 | v182 | numeric | how often in country's elections: rich people buy elections (Q50G) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 48126 | 4 |
v182_DK | 259 | v182_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: rich people buy elections (Q50G) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1459 | 3 |
v183 | 260 | v183 | numeric | how often in country's elections: voters are threatened with violence at the polls (Q50H) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49341 | 4 |
v183_DK | 261 | v183_DK | numeric | DK MODIFIED (cat. 4 dropped): how often in country's elections: voters are threatened with violence at the polls (Q50H) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1534 | 3 |
v184 | 262 | v184 | numeric | immigrants: impact on the development of [your country] (Q51) | {1.0: 'very bad', 2.0: 'quite bad', 3.0: 'neither good, nor bad', 4.0: 'quite good', 5.0: 'very good'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56958 | 5 |
v185 | 263 | v185 | numeric | immigrants take away jobs from [nationality] (Q52A) | {1.0: 'take away', 10.0: 'do not take away'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57389 | 10 |
v186 | 264 | v186 | numeric | immigrants increase crime problems (Q52B) | {1.0: 'make it worse', 10.0: 'do not make it worse'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56679 | 10 |
v187 | 265 | v187 | numeric | immigrants are a strain on welfare system (Q52C) | {1.0: 'are a strain', 10.0: 'are not a strain'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56578 | 10 |
v188 | 266 | v188 | numeric | better if immigrants maintain/not maintain own customs (Q52D) | {1.0: 'maintain distinct customs and traditions', 10.0: 'do not maintain distinct customs and traditions'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55706 | 10 |
v189 | 267 | v189 | numeric | important: to have been born in [country] (Q53A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58850 | 4 |
v190 | 268 | v190 | numeric | important: to respect [country nationality] political institutions and laws (Q53B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58850 | 4 |
v191 | 269 | v191 | numeric | important: to have [country nationality] ancestry (Q53C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58728 | 4 |
v192 | 270 | v192 | numeric | important: to be able to speak [national language] (Q53D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59034 | 4 |
v193 | 271 | v193 | numeric | important: to share [national] culture (Q53E) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58811 | 4 |
v194 | 272 | v194 | numeric | important: to be born in Europe (Q54A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57741 | 4 |
v195 | 273 | v195 | numeric | important: to have European ancestry (Q54B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57562 | 4 |
v196 | 274 | v196 | numeric | important: to be a Christian (Q54C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57302 | 4 |
v197 | 275 | v197 | numeric | important: to share European culture (Q54D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57614 | 4 |
v198 | 276 | v198 | numeric | European Union enlargement (Q55) | {1.0: 'should go further', 10.0: 'has gone too far'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54139 | 10 |
v199 | 277 | v199 | numeric | environment: giving part of income (Q56A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57664 | 5 |
f199_IT | 278 | f199_IT | nominal | IT FLAG EXPERIMENT: (answer cat. modified) experimental condition items v199 to v203 (Q56) | {1.0: "version 1 - d'accordo/contrario (agree/against)", 2.0: "version 2 - d'accordo/in disaccordo (agree/disagree)"} | {-4.0: 'item not included', -2.0: 'no answer', -1.0: 'dont know'} | True | 2277 | 2 |
v200 | 279 | v200 | numeric | environment: too difficult for me to do much (Q56B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58012 | 5 |
v201 | 280 | v201 | numeric | environment: there are more important things to do (Q56C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58091 | 5 |
v202 | 281 | v202 | numeric | environment: no point unless others do the same (Q56D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58042 | 5 |
v203 | 282 | v203 | numeric | environment: environmental threats are exaggerated (Q56E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56348 | 5 |
v204 | 283 | v204 | numeric | protecting environment vs. economic growth (Q57) | {1.0: 'protecting the environment priority, even if slower economic growth and loss of jobs', 2.0: 'economic growth and creating jobs priority, even if environment suffers'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 3.0: 'other answer (code if volunteered only!)'} | True | 52224 | 2 |
v205 | 284 | v205 | numeric | government: public area under video surveillance (Q58A) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57923 | 4 |
v206 | 285 | v206 | numeric | government: monitor all information exchanged on the internet (Q58B) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57022 | 4 |
v207 | 286 | v207 | numeric | government: collect information about anyone without their knowledge (Q58C) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57470 | 4 |
v208 | 287 | v208 | numeric | how often do you follow politics: on television (Q59A) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59128 | 5 |
v209 | 288 | v209 | numeric | how often do you follow politics: on the radio (Q59B) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58924 | 5 |
v210 | 289 | v210 | numeric | how often do you follow politics: in the daily papers (Q59C) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58929 | 5 |
v211 | 290 | v211 | numeric | how often do you follow politics: on social media (Q59D) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58647 | 5 |
v212 | 291 | v212 | numeric | are you concerned with: people neighbourhood (Q60A) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58883 | 5 |
v213 | 292 | v213 | numeric | are you concerned with: people own region (Q60B) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58781 | 5 |
v214 | 293 | v214 | numeric | are you concerned with: fellow countrymen (Q60C) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58704 | 5 |
v215 | 294 | v215 | numeric | are you concerned with: Europeans (Q60D) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58212 | 5 |
v216 | 295 | v216 | numeric | are you concerned with: humankind (Q60E) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58144 | 5 |
v217 | 296 | v217 | numeric | are you concerned with: elderly people (Q61A) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59034 | 5 |
v218 | 297 | v218 | numeric | are you concerned with: unemployed people (Q61B) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58797 | 5 |
v219 | 298 | v219 | numeric | are you concerned with: immigrants (Q61C) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58379 | 5 |
v220 | 299 | v220 | numeric | are you concerned with: sick and disabled (Q61D) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58912 | 5 |
v221 | 300 | v221 | numeric | important: eliminating income inequalities (Q62A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57320 | 4 |
v221_DK | 301 | v221_DK | numeric | DK MODIFIED (cat. 4 dropped): important: eliminating income inequalities (Q62A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 384 | 3 |
v222 | 302 | v222 | numeric | important: basic needs for all (Q62B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58247 | 4 |
v222_DK | 303 | v222_DK | numeric | DK MODIFIED (cat. 4 dropped): important: basic needs for all (Q62B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 400 | 3 |
v223 | 304 | v223 | numeric | important: recognizing people on merits (Q62C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57866 | 4 |
v223_DK | 305 | v223_DK | numeric | DK MODIFIED (cat. 4 dropped): important: recognizing people on merits (Q62C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 381 | 3 |
v224 | 306 | v224 | numeric | important: protecting against terrorism (Q62D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58215 | 4 |
v224_DK | 307 | v224_DK | numeric | DK MODIFIED (cat. 4 dropped): important: protecting against terrorism (Q62D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 404 | 3 |
v225 | 308 | v225 | numeric | sex respondent (Q63) | {1.0: 'male', 2.0: 'female'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59412 | 2 |
v226 | 309 | v226 | numeric | year of birth respondent (Q64) | {1937.0: '1937 and before'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 67 |
age | 310 | age | numeric | age: respondent (constructed) (Q64) | {82.0: '82 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 65 |
age_r | 311 | age_r | nominal | age recoded (6 intervals) (Q64) | {1.0: '15-24 years', 2.0: '25-34 years', 3.0: '35-44 years', 4.0: '45-54 years', 5.0: '55-64 years', 6.0: '65 and more years'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 6 |
age_r2 | 312 | age_r2 | nominal | age recoded (3 intervals) (Q64) | {1.0: '15-29 years', 2.0: '30-49 years', 3.0: '50 and more years'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 3 |
age_r3 | 313 | age_r3 | nominal | age recoded (7 intervals) (Q64) | {1.0: '18-24 years', 2.0: '25-34 years', 3.0: '35-44 years', 4.0: '45-54 years', 5.0: '55-64 years', 6.0: '65-74 years', 7.0: '75 and more years'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 7 |
v227 | 314 | v227 | numeric | respondent born in [country] (Q65) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59367 | 2 |
v228b | 315 | v228b | nominal | respondents country of birth (ISO 3166-1/3 alpha code) (Q66) | {'005': 'M49 code: South America', '011': 'M49 code: Western Africa', '013': 'M49 code: Central America', '014': 'M49 code: Eastern Africa', '015': 'M49 code: Northern Africa', '017': 'M49 code: Middle Africa', '018': 'M49 code: Southern Africa', '029': 'M49 code: Caribbean', '030': 'M49 code: Eastern Asia', '034': 'M49 code: Southern Asia', '035': 'M49 code: South-Eastern Asia', '039': 'M49 code: Southern Europe', '053': 'M49 code: Australia and New Zealand', '054': 'M49 code: Melanesia', '061': 'M49 code: Polynesia', '143': 'M49 code: Central Asia', '145': 'M49 code: Western Asia', '154': 'M49 code: Northern Europe', 'AD': 'Andorra', 'AE': 'United Arab Emirates', 'AF': 'Afghanistan', 'AG': 'Antigua and Barbuda', 'AI': 'Anguilla', 'AL': 'Albania', 'AM': 'Armenia', 'AO': 'Angola', 'AQ': 'Antarctica', 'AR': 'Argentina', 'AS': 'American Samoa', 'AT': 'Austria', 'AU': 'Australia', 'AW': 'Aruba', 'AX': 'Åland Islands', 'AZ': 'Azerbaijan', 'BA': 'Bosnia and Herzegovina', 'BB': 'Barbados', 'BD': 'Bangladesh', 'BE': 'Belgium', 'BF': 'Burkina Faso', 'BG': 'Bulgaria', 'BH': 'Bahrain', 'BI': 'Burundi', 'BJ': 'Benin', 'BL': 'Saint Barthélemy', 'BM': 'Bermuda', 'BN': 'Brunei Darussalam', 'BO': 'Bolivia (Plurinational State of)', 'BQ': 'Bonaire, Sint Eustatius and Saba', 'BR': 'Brazil', 'BS': 'Bahamas', 'BT': 'Bhutan', 'BV': 'Bouvet Island', 'BW': 'Botswana', 'BY': 'Belarus', 'BZ': 'Belize', 'CA': 'Canada', 'CC': 'Cocos (Keeling) Islands', 'CD': 'Congo (Democratic Republic of the)', 'CF': 'Central African Republic', 'CG': 'Congo', 'CH': 'Switzerland', 'CI': 'Côte d´Ivoire', 'CK': 'Cook Islands', 'CL': 'Chile', 'CM': 'Cameroon', 'CN': 'China', 'CO': 'Colombia', 'CR': 'Costa Rica', 'CSHH': 'Czechoslovakia (former country)', 'CSXX': 'Serbia and Montenegro (former country)', 'CU': 'Cuba', 'CV': 'Cabo Verde', 'CW': 'Curaçao', 'CX': 'Christmas Island', 'CY': 'Cyprus', 'CY-TCC': 'Northern Cyprus', 'CZ': 'Czechia', 'DDDE': 'German Democratic Republic (former country)', 'DE': 'Germany', 'DJ': 'Djibouti', 'DK': 'Denmark', 'DM': 'Dominica', 'DO': 'Dominican Republic', 'DZ': 'Algeria', 'EC': 'Ecuador', 'EE': 'Estonia', 'EG': 'Egypt', 'EH': 'Western Sahara', 'ER': 'Eritrea', 'ES': 'Spain', 'ET': 'Ethiopia', 'FI': 'Finland', 'FJ': 'Fiji', 'FK': 'Falkland Islands (Malvinas)', 'FM': 'Micronesia (Federated States of)', 'FO': 'Faroe Islands', 'FR': 'France', 'GA': 'Gabon', 'GB': 'United Kingdom of Great Britain and Northern Ireland', 'GD': 'Grenada', 'GE': 'Georgia', 'GF': 'French Guiana', 'GG': 'Guernsey', 'GH': 'Ghana', 'GI': 'Gibraltar', 'GL': 'Greenland', 'GM': 'Gambia', 'GN': 'Guinea', 'GP': 'Guadeloupe', 'GQ': 'Equatorial Guinea', 'GR': 'Greece', 'GS': 'South Georgia and the South Sandwich Islands', 'GT': 'Guatemala', 'GU': 'Guam', 'GW': 'Guinea-Bissau', 'GY': 'Guyana', 'HK': 'Hong Kong', 'HM': 'Heard Island and McDonald Islands', 'HN': 'Honduras', 'HR': 'Croatia', 'HT': 'Haiti', 'HU': 'Hungary', 'ID': 'Indonesia', 'IE': 'Ireland', 'IL': 'Israel', 'IM': 'Isle of Man', 'IN': 'India', 'IO': 'British Indian Ocean Territory', 'IQ': 'Iraq', 'IR': 'Iran (Islamic Republic of)', 'IS': 'Iceland', 'IT': 'Italy', 'JE': 'Jersey', 'JM': 'Jamaica', 'JO': 'Jordan', 'JP': 'Japan', 'KE': 'Kenya', 'KG': 'Kyrgyzstan', 'KH': 'Cambodia', 'KI': 'Kiribati', 'KM': 'Comoros', 'KN': 'Saint Kitts and Nevis', 'KP': 'Korea (Democratic People´s Republic of)', 'KR': 'Korea (Republic of)', 'KW': 'Kuwait', 'KY': 'Cayman Islands', 'KZ': 'Kazakhstan', 'LA': 'Lao People´s Democratic Republic', 'LB': 'Lebanon', 'LC': 'Saint Lucia', 'LI': 'Liechtenstein', 'LK': 'Sri Lanka', 'LR': 'Liberia', 'LS': 'Lesotho', 'LT': 'Lithuania', 'LU': 'Luxembourg', 'LV': 'Latvia', 'LY': 'Libya', 'MA': 'Morocco', 'MC': 'Monaco', 'MD': 'Moldova (Republic of)', 'ME': 'Montenegro', 'MF': 'Saint Martin (French part)', 'MG': 'Madagascar', 'MH': 'Marshall Islands', 'MK': 'North Macedonia', 'ML': 'Mali', 'MM': 'Myanmar', 'MN': 'Mongolia', 'MO': 'Macao', 'MP': 'Northern Mariana Islands', 'MQ': 'Martinique', 'MR': 'Mauritania', 'MS': 'Montserrat', 'MT': 'Malta', 'MU': 'Mauritius', 'MV': 'Maldives', 'MW': 'Malawi', 'MX': 'Mexico', 'MY': 'Malaysia', 'MZ': 'Mozambique', 'NA': 'Namibia', 'NC': 'New Caledonia', 'NE': 'Niger', 'NF': 'Norfolk Island', 'NG': 'Nigeria', 'NI': 'Nicaragua', 'NL': 'Netherlands', 'NO': 'Norway', 'NP': 'Nepal', 'NR': 'Nauru', 'NU': 'Niue', 'NZ': 'New Zealand', 'OM': 'Oman', 'PA': 'Panama', 'PE': 'Peru', 'PF': 'French Polynesia', 'PG': 'Papua New Guinea', 'PH': 'Philippines', 'PK': 'Pakistan', 'PL': 'Poland', 'PM': 'Saint Pierre and Miquelon', 'PN': 'Pitcairn', 'PR': 'Puerto Rico', 'PS': 'Palestine, State of', 'PT': 'Portugal', 'PW': 'Palau', 'PY': 'Paraguay', 'QA': 'Qatar', 'RE': 'Réunion', 'RO': 'Romania', 'RS': 'Serbia', 'RU': 'Russian Federation', 'RW': 'Rwanda', 'SA': 'Saudi Arabia', 'SB': 'Solomon Islands', 'SC': 'Seychelles', 'SD': 'Sudan', 'SE': 'Sweden', 'SG': 'Singapore', 'SH': 'Saint Helena, Ascension and Tristan da Cunha', 'SI': 'Slovenia', 'SJ': 'Svalbard and Jan Mayen', 'SK': 'Slovakia', 'SL': 'Sierra Leone', 'SM': 'San Marino', 'SN': 'Senegal', 'SO': 'Somalia', 'SR': 'Suriname', 'SS': 'South Sudan', 'ST': 'Sao Tome and Principe', 'SUHH': 'U.S.S.R. (former country)', 'SV': 'El Salvador', 'SX': 'Sint Maarten (Dutch part)', 'SY': 'Syrian Arab Republic', 'SZ': 'Swaziland', 'TC': 'Turks and Caicos Islands', 'TD': 'Chad', 'TF': 'French Southern Territories', 'TG': 'Togo', 'TH': 'Thailand', 'TJ': 'Tajikistan', 'TK': 'Tokelau', 'TL': 'Timor-Leste', 'TM': 'Turkmenistan', 'TN': 'Tunisia', 'TO': 'Tonga', 'TR': 'Turkey', 'TT': 'Trinidad and Tobago', 'TV': 'Tuvalu', 'TW': 'Taiwan, Province of China[a]', 'TZ': 'Tanzania, United Republic of', 'UA': 'Ukraine', 'UG': 'Uganda', 'UM': 'United States Minor Outlying Islands', 'US': 'United States of America', 'UY': 'Uruguay', 'UZ': 'Uzbekistan', 'VA': 'Holy See', 'VC': 'Saint Vincent and the Grenadines', 'VE': 'Venezuela (Bolivarian Republic of)', 'VG': 'Virgin Islands (British)', 'VI': 'Virgin Islands (U.S.)', 'VN': 'Viet Nam', 'VU': 'Vanuatu', 'WF': 'Wallis and Futuna', 'WS': 'Samoa', 'XK': 'Kosovo', 'XXX': 'Other', 'YE': 'Yemen', 'YT': 'Mayotte', 'YUCS': 'Yugoslavia (former country)', 'ZA': 'South Africa', 'ZM': 'Zambia', 'ZW': 'Zimbabwe'} | {'-1': "don't know/no answer", '-3': 'not applicable', '-5': 'other missing'} | True | 4221 | 94 |
v228b_r | 316 | v228b_r | nominal | respondents country of birth (ISO 3166-1 numeric code) (Q66) | {4.0: ' Afghanistan', 5.0: 'M49 code: South America', 8.0: ' Albania', 10.0: ' Antarctica', 11.0: 'M49 code: Western Africa', 12.0: ' Algeria', 13.0: 'M49 code: Central America', 14.0: 'M49 code: Eastern Africa', 15.0: 'M49 code: Northern Africa', 16.0: ' American Samoa', 17.0: 'M49 code: Middle Africa', 18.0: 'M49 code: Southern Africa', 20.0: ' Andorra', 24.0: ' Angola', 28.0: ' Antigua and Barbuda', 29.0: 'M49 code: Caribbean', 30.0: 'M49 code: Eastern Asia', 31.0: ' Azerbaijan', 32.0: ' Argentina', 34.0: 'M49 code: Southern Asia', 35.0: 'M49 code: South-Eastern Asia', 36.0: ' Australia', 39.0: 'M49 code: Southern Europe', 40.0: ' Austria', 44.0: ' Bahamas', 48.0: ' Bahrain', 50.0: ' Bangladesh', 51.0: ' Armenia', 52.0: ' Barbados', 53.0: 'M49 code: Australia and New Zealand', 54.0: 'M49 code: Melanesia', 56.0: ' Belgium', 60.0: ' Bermuda', 61.0: 'M49 code: Polynesia', 64.0: ' Bhutan', 68.0: ' Bolivia, Plurinational State of', 70.0: ' Bosnia and Herzegovina', 72.0: ' Botswana', 74.0: ' Bouvet Island', 76.0: ' Brazil', 84.0: ' Belize', 86.0: ' British Indian Ocean Territory', 90.0: ' Solomon Islands', 92.0: ' Virgin Islands, British', 96.0: ' Brunei Darussalam', 100.0: ' Bulgaria', 104.0: ' Myanmar', 108.0: ' Burundi', 112.0: ' Belarus', 116.0: ' Cambodia', 120.0: ' Cameroon', 124.0: ' Canada', 132.0: ' Cabo Verde', 136.0: ' Cayman Islands', 140.0: ' Central African Republic', 143.0: 'M49 code: Central Asia', 144.0: ' Sri Lanka', 145.0: 'M49 code: Western Asia', 148.0: ' Chad', 152.0: ' Chile', 154.0: 'M49 code: Northern Europe', 156.0: ' China', 158.0: ' Taiwan, Province of China', 162.0: ' Christmas Island', 166.0: ' Cocos (Keeling) Islands', 170.0: ' Colombia', 174.0: ' Comoros', 175.0: ' Mayotte', 178.0: ' Congo', 180.0: ' Congo, the Democratic Republic of the', 184.0: ' Cook Islands', 188.0: ' Costa Rica', 191.0: ' Croatia', 192.0: ' Cuba', 196.0: ' Cyprus', 200.0: ' Czechoslovakia (former country)', 203.0: ' Czechia', 204.0: ' Benin', 208.0: ' Denmark', 212.0: ' Dominica', 214.0: ' Dominican Republic', 218.0: ' Ecuador', 222.0: ' El Salvador', 226.0: ' Equatorial Guinea', 231.0: ' Ethiopia', 232.0: ' Eritrea', 233.0: ' Estonia', 234.0: ' Faroe Islands', 238.0: ' Falkland Islands (Malvinas)', 239.0: ' South Georgia and the South Sandwich Islands', 242.0: ' Fiji', 246.0: ' Finland', 248.0: ' Åland Islands', 250.0: ' France', 254.0: ' French Guiana', 258.0: ' French Polynesia', 260.0: ' French Southern Territories', 262.0: ' Djibouti', 266.0: ' Gabon', 268.0: ' Georgia', 270.0: ' Gambia', 275.0: ' Palestine, State of', 276.0: ' Germany', 278.0: 'German Democratic Republic (former country)', 288.0: ' Ghana', 292.0: ' Gibraltar', 296.0: ' Kiribati', 300.0: ' Greece', 304.0: ' Greenland', 308.0: ' Grenada', 312.0: ' Guadeloupe', 316.0: ' Guam', 320.0: ' Guatemala', 324.0: ' Guinea', 328.0: ' Guyana', 332.0: ' Haiti', 334.0: ' Heard Island and McDonald Islands', 336.0: ' Holy See', 340.0: ' Honduras', 344.0: ' Hong Kong', 348.0: ' Hungary', 352.0: ' Iceland', 356.0: ' India', 360.0: ' Indonesia', 364.0: ' Iran, Islamic Republic of', 368.0: ' Iraq', 372.0: ' Ireland', 376.0: ' Israel', 380.0: ' Italy', 384.0: " Côte d'Ivoire", 388.0: ' Jamaica', 392.0: ' Japan', 398.0: ' Kazakhstan', 400.0: ' Jordan', 404.0: ' Kenya', 408.0: " Korea, Democratic People's Republic of", 410.0: ' Korea, Republic of', 414.0: ' Kuwait', 417.0: ' Kyrgyzstan', 418.0: " Lao People's Democratic Republic", 422.0: ' Lebanon', 426.0: ' Lesotho', 428.0: ' Latvia', 430.0: ' Liberia', 434.0: ' Libya', 438.0: ' Liechtenstein', 440.0: ' Lithuania', 442.0: ' Luxembourg', 446.0: ' Macao', 450.0: ' Madagascar', 454.0: ' Malawi', 458.0: ' Malaysia', 462.0: ' Maldives', 466.0: ' Mali', 470.0: ' Malta', 474.0: ' Martinique', 478.0: ' Mauritania', 480.0: ' Mauritius', 484.0: ' Mexico', 492.0: ' Monaco', 496.0: ' Mongolia', 498.0: ' Moldova, Republic of', 499.0: ' Montenegro', 500.0: ' Montserrat', 504.0: ' Morocco', 508.0: ' Mozambique', 512.0: ' Oman', 516.0: ' Namibia', 520.0: ' Nauru', 524.0: ' Nepal', 528.0: ' Netherlands', 531.0: ' Curaçao', 533.0: ' Aruba', 534.0: ' Sint Maarten (Dutch part)', 535.0: ' Bonaire, Sint Eustatius and Saba', 540.0: ' New Caledonia', 548.0: ' Vanuatu', 554.0: ' New Zealand', 558.0: ' Nicaragua', 562.0: ' Niger', 566.0: ' Nigeria', 570.0: ' Niue', 574.0: ' Norfolk Island', 578.0: ' Norway', 580.0: ' Northern Mariana Islands', 581.0: ' United States Minor Outlying Islands', 583.0: ' Micronesia, Federated States of', 584.0: ' Marshall Islands', 585.0: ' Palau', 586.0: ' Pakistan', 591.0: ' Panama', 598.0: ' Papua New Guinea', 600.0: ' Paraguay', 604.0: ' Peru', 608.0: ' Philippines', 612.0: ' Pitcairn', 616.0: ' Poland', 620.0: ' Portugal', 624.0: ' Guinea-Bissau', 626.0: ' Timor-Leste', 630.0: ' Puerto Rico', 634.0: ' Qatar', 638.0: ' Réunion', 642.0: ' Romania', 643.0: ' Russian Federation', 646.0: ' Rwanda', 652.0: ' Saint Barthélemy', 654.0: ' Saint Helena, Ascension and Tristan da Cunha', 659.0: ' Saint Kitts and Nevis', 660.0: ' Anguilla', 662.0: ' Saint Lucia', 663.0: ' Saint Martin (French part)', 666.0: ' Saint Pierre and Miquelon', 670.0: ' Saint Vincent and the Grenadines', 674.0: ' San Marino', 678.0: ' Sao Tome and Principe', 682.0: ' Saudi Arabia', 686.0: ' Senegal', 688.0: ' Serbia', 690.0: ' Seychelles', 694.0: ' Sierra Leone', 702.0: ' Singapore', 703.0: ' Slovak Republic', 704.0: ' Viet Nam', 705.0: ' Slovenia', 706.0: ' Somalia', 710.0: ' South Africa', 716.0: ' Zimbabwe', 724.0: ' Spain', 728.0: ' South Sudan', 729.0: ' Sudan', 732.0: ' Western Sahara', 740.0: ' Suriname', 744.0: ' Svalbard and Jan Mayen', 748.0: ' Swaziland', 752.0: ' Sweden', 756.0: ' Switzerland', 760.0: ' Syrian Arab Republic', 762.0: ' Tajikistan', 764.0: ' Thailand', 768.0: ' Togo', 772.0: ' Tokelau', 776.0: ' Tonga', 780.0: ' Trinidad and Tobago', 784.0: ' United Arab Emirates', 788.0: ' Tunisia', 792.0: ' Turkey', 795.0: ' Turkmenistan', 796.0: ' Turks and Caicos Islands', 798.0: ' Tuvalu', 800.0: ' Uganda', 804.0: ' Ukraine', 807.0: ' North Macedonia', 810.0: ' U.S.S.R. (former country)', 818.0: ' Egypt', 826.0: ' United Kingdom of Great Britain and Northern Ireland', 831.0: ' Guernsey', 832.0: ' Jersey', 833.0: ' Isle of Man', 834.0: ' Tanzania, United Republic of', 840.0: ' United States of America', 850.0: ' Virgin Islands, U.S.', 854.0: ' Burkina Faso', 858.0: ' Uruguay', 860.0: ' Uzbekistan', 862.0: ' Venezuela, Bolivarian Republic of', 876.0: ' Wallis and Futuna', 882.0: ' Samoa', 887.0: ' Yemen', 890.0: ' Yugoslavia (former country)', 891.0: 'Serbia and Montenegro (former country)', 894.0: ' Zambia', 915.0: ' Kosovo', 1111.0: ' Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'NaN', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 4220 | 94 |
v229 | 317 | v229 | numeric | year in which respondent came to live in [country] (Q67) | {1941.0: '1941 and before'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 4116 | 79 |
v230 | 318 | v230 | numeric | father born in [country] (Q68) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59042 | 2 |
v231b | 319 | v231b | nominal | fathers country of birth (ISO 3166-1/3 Alpha code) (Q69) | {'005': 'M49 code: South America', '011': 'M49 code: Western Africa', '013': 'M49 code: Central America', '014': 'M49 code: Eastern Africa', '015': 'M49 code: Northern Africa', '017': 'M49 code: Middle Africa', '018': 'M49 code: Southern Africa', '029': 'M49 code: Caribbean', '030': 'M49 code: Eastern Asia', '034': 'M49 code: Southern Asia', '035': 'M49 code: South-Eastern Asia', '039': 'M49 code: Southern Europe', '053': 'M49 code: Australia and New Zealand', '054': 'M49 code: Melanesia', '061': 'M49 code: Polynesia', '143': 'M49 code: Central Asia', '145': 'M49 code: Western Asia', '154': 'M49 code: Northern Europe', 'AD': 'Andorra', 'AE': 'United Arab Emirates', 'AF': 'Afghanistan', 'AG': 'Antigua and Barbuda', 'AI': 'Anguilla', 'AL': 'Albania', 'AM': 'Armenia', 'AO': 'Angola', 'AQ': 'Antarctica', 'AR': 'Argentina', 'AS': 'American Samoa', 'AT': 'Austria', 'AU': 'Australia', 'AW': 'Aruba', 'AX': 'Åland Islands', 'AZ': 'Azerbaijan', 'BA': 'Bosnia and Herzegovina', 'BB': 'Barbados', 'BD': 'Bangladesh', 'BE': 'Belgium', 'BF': 'Burkina Faso', 'BG': 'Bulgaria', 'BH': 'Bahrain', 'BI': 'Burundi', 'BJ': 'Benin', 'BL': 'Saint Barthélemy', 'BM': 'Bermuda', 'BN': 'Brunei Darussalam', 'BO': 'Bolivia (Plurinational State of)', 'BQ': 'Bonaire, Sint Eustatius and Saba', 'BR': 'Brazil', 'BS': 'Bahamas', 'BT': 'Bhutan', 'BV': 'Bouvet Island', 'BW': 'Botswana', 'BY': 'Belarus', 'BZ': 'Belize', 'CA': 'Canada', 'CC': 'Cocos (Keeling) Islands', 'CD': 'Congo (Democratic Republic of the)', 'CF': 'Central African Republic', 'CG': 'Congo', 'CH': 'Switzerland', 'CI': 'Côte d´Ivoire', 'CK': 'Cook Islands', 'CL': 'Chile', 'CM': 'Cameroon', 'CN': 'China', 'CO': 'Colombia', 'CR': 'Costa Rica', 'CSHH': 'Czechoslovakia (former country)', 'CSXX': 'Serbia and Montenegro (former country)', 'CU': 'Cuba', 'CV': 'Cabo Verde', 'CW': 'Curaçao', 'CX': 'Christmas Island', 'CY': 'Cyprus', 'CY-TCC': 'Northern Cyprus', 'CZ': 'Czechia', 'DDDE': 'German Democratic Republic (former country)', 'DE': 'Germany', 'DJ': 'Djibouti', 'DK': 'Denmark', 'DM': 'Dominica', 'DO': 'Dominican Republic', 'DZ': 'Algeria', 'EC': 'Ecuador', 'EE': 'Estonia', 'EG': 'Egypt', 'EH': 'Western Sahara', 'ER': 'Eritrea', 'ES': 'Spain', 'ET': 'Ethiopia', 'FI': 'Finland', 'FJ': 'Fiji', 'FK': 'Falkland Islands (Malvinas)', 'FM': 'Micronesia (Federated States of)', 'FO': 'Faroe Islands', 'FR': 'France', 'GA': 'Gabon', 'GB': 'United Kingdom of Great Britain and Northern Ireland', 'GD': 'Grenada', 'GE': 'Georgia', 'GF': 'French Guiana', 'GG': 'Guernsey', 'GH': 'Ghana', 'GI': 'Gibraltar', 'GL': 'Greenland', 'GM': 'Gambia', 'GN': 'Guinea', 'GP': 'Guadeloupe', 'GQ': 'Equatorial Guinea', 'GR': 'Greece', 'GS': 'South Georgia and the South Sandwich Islands', 'GT': 'Guatemala', 'GU': 'Guam', 'GW': 'Guinea-Bissau', 'GY': 'Guyana', 'HK': 'Hong Kong', 'HM': 'Heard Island and McDonald Islands', 'HN': 'Honduras', 'HR': 'Croatia', 'HT': 'Haiti', 'HU': 'Hungary', 'ID': 'Indonesia', 'IE': 'Ireland', 'IL': 'Israel', 'IM': 'Isle of Man', 'IN': 'India', 'IO': 'British Indian Ocean Territory', 'IQ': 'Iraq', 'IR': 'Iran (Islamic Republic of)', 'IS': 'Iceland', 'IT': 'Italy', 'JE': 'Jersey', 'JM': 'Jamaica', 'JO': 'Jordan', 'JP': 'Japan', 'KE': 'Kenya', 'KG': 'Kyrgyzstan', 'KH': 'Cambodia', 'KI': 'Kiribati', 'KM': 'Comoros', 'KN': 'Saint Kitts and Nevis', 'KP': 'Korea (Democratic People´s Republic of)', 'KR': 'Korea (Republic of)', 'KW': 'Kuwait', 'KY': 'Cayman Islands', 'KZ': 'Kazakhstan', 'LA': 'Lao People´s Democratic Republic', 'LB': 'Lebanon', 'LC': 'Saint Lucia', 'LI': 'Liechtenstein', 'LK': 'Sri Lanka', 'LR': 'Liberia', 'LS': 'Lesotho', 'LT': 'Lithuania', 'LU': 'Luxembourg', 'LV': 'Latvia', 'LY': 'Libya', 'MA': 'Morocco', 'MC': 'Monaco', 'MD': 'Moldova (Republic of)', 'ME': 'Montenegro', 'MF': 'Saint Martin (French part)', 'MG': 'Madagascar', 'MH': 'Marshall Islands', 'MK': 'North Macedonia', 'ML': 'Mali', 'MM': 'Myanmar', 'MN': 'Mongolia', 'MO': 'Macao', 'MP': 'Northern Mariana Islands', 'MQ': 'Martinique', 'MR': 'Mauritania', 'MS': 'Montserrat', 'MT': 'Malta', 'MU': 'Mauritius', 'MV': 'Maldives', 'MW': 'Malawi', 'MX': 'Mexico', 'MY': 'Malaysia', 'MZ': 'Mozambique', 'NA': 'Namibia', 'NC': 'New Caledonia', 'NE': 'Niger', 'NF': 'Norfolk Island', 'NG': 'Nigeria', 'NI': 'Nicaragua', 'NL': 'Netherlands', 'NO': 'Norway', 'NP': 'Nepal', 'NR': 'Nauru', 'NU': 'Niue', 'NZ': 'New Zealand', 'OM': 'Oman', 'PA': 'Panama', 'PE': 'Peru', 'PF': 'French Polynesia', 'PG': 'Papua New Guinea', 'PH': 'Philippines', 'PK': 'Pakistan', 'PL': 'Poland', 'PM': 'Saint Pierre and Miquelon', 'PN': 'Pitcairn', 'PR': 'Puerto Rico', 'PS': 'Palestine, State of', 'PT': 'Portugal', 'PW': 'Palau', 'PY': 'Paraguay', 'QA': 'Qatar', 'RE': 'Réunion', 'RO': 'Romania', 'RS': 'Serbia', 'RU': 'Russian Federation', 'RW': 'Rwanda', 'SA': 'Saudi Arabia', 'SB': 'Solomon Islands', 'SC': 'Seychelles', 'SD': 'Sudan', 'SE': 'Sweden', 'SG': 'Singapore', 'SH': 'Saint Helena, Ascension and Tristan da Cunha', 'SI': 'Slovenia', 'SJ': 'Svalbard and Jan Mayen', 'SK': 'Slovakia', 'SL': 'Sierra Leone', 'SM': 'San Marino', 'SN': 'Senegal', 'SO': 'Somalia', 'SR': 'Suriname', 'SS': 'South Sudan', 'ST': 'Sao Tome and Principe', 'SUHH': 'U.S.S.R. (former country)', 'SV': 'El Salvador', 'SX': 'Sint Maarten (Dutch part)', 'SY': 'Syrian Arab Republic', 'SZ': 'Swaziland', 'TC': 'Turks and Caicos Islands', 'TD': 'Chad', 'TF': 'French Southern Territories', 'TG': 'Togo', 'TH': 'Thailand', 'TJ': 'Tajikistan', 'TK': 'Tokelau', 'TL': 'Timor-Leste', 'TM': 'Turkmenistan', 'TN': 'Tunisia', 'TO': 'Tonga', 'TR': 'Turkey', 'TT': 'Trinidad and Tobago', 'TV': 'Tuvalu', 'TW': 'Taiwan, Province of China[a]', 'TZ': 'Tanzania, United Republic of', 'UA': 'Ukraine', 'UG': 'Uganda', 'UM': 'United States Minor Outlying Islands', 'US': 'United States of America', 'UY': 'Uruguay', 'UZ': 'Uzbekistan', 'VA': 'Holy See', 'VC': 'Saint Vincent and the Grenadines', 'VE': 'Venezuela (Bolivarian Republic of)', 'VG': 'Virgin Islands (British)', 'VI': 'Virgin Islands (U.S.)', 'VN': 'Viet Nam', 'VU': 'Vanuatu', 'WF': 'Wallis and Futuna', 'WS': 'Samoa', 'XK': 'Kosovo', 'XXX': 'Other', 'YE': 'Yemen', 'YT': 'Mayotte', 'YUCS': 'Yugoslavia (former country)', 'ZA': 'South Africa', 'ZM': 'Zambia', 'ZW': 'Zimbabwe'} | {'-1': "don't know/no answer", '-3': 'not applicable', '-5': 'other missing'} | True | 6117 | 96 |
v231b_r | 320 | v231b_r | nominal | fathers country of birth (ISO 3166-1 numeric code) (Q69) | {4.0: ' Afghanistan', 5.0: 'M49 code: South America', 8.0: ' Albania', 10.0: ' Antarctica', 11.0: 'M49 code: Western Africa', 12.0: ' Algeria', 13.0: 'M49 code: Central America', 14.0: 'M49 code: Eastern Africa', 15.0: 'M49 code: Northern Africa', 16.0: ' American Samoa', 17.0: 'M49 code: Middle Africa', 18.0: 'M49 code: Southern Africa', 20.0: ' Andorra', 24.0: ' Angola', 28.0: ' Antigua and Barbuda', 29.0: 'M49 code: Caribbean', 30.0: 'M49 code: Eastern Asia', 31.0: ' Azerbaijan', 32.0: ' Argentina', 34.0: 'M49 code: Southern Asia', 35.0: 'M49 code: South-Eastern Asia', 36.0: ' Australia', 39.0: 'M49 code: Southern Europe', 40.0: ' Austria', 44.0: ' Bahamas', 48.0: ' Bahrain', 50.0: ' Bangladesh', 51.0: ' Armenia', 52.0: ' Barbados', 53.0: 'M49 code: Australia and New Zealand', 54.0: 'M49 code: Melanesia', 56.0: ' Belgium', 60.0: ' Bermuda', 61.0: 'M49 code: Polynesia', 64.0: ' Bhutan', 68.0: ' Bolivia, Plurinational State of', 70.0: ' Bosnia and Herzegovina', 72.0: ' Botswana', 74.0: ' Bouvet Island', 76.0: ' Brazil', 84.0: ' Belize', 86.0: ' British Indian Ocean Territory', 90.0: ' Solomon Islands', 92.0: ' Virgin Islands, British', 96.0: ' Brunei Darussalam', 100.0: ' Bulgaria', 104.0: ' Myanmar', 108.0: ' Burundi', 112.0: ' Belarus', 116.0: ' Cambodia', 120.0: ' Cameroon', 124.0: ' Canada', 132.0: ' Cabo Verde', 136.0: ' Cayman Islands', 140.0: ' Central African Republic', 143.0: 'M49 code: Central Asia', 144.0: ' Sri Lanka', 145.0: 'M49 code: Western Asia', 148.0: ' Chad', 152.0: ' Chile', 154.0: 'M49 code: Northern Europe', 156.0: ' China', 158.0: ' Taiwan, Province of China', 162.0: ' Christmas Island', 166.0: ' Cocos (Keeling) Islands', 170.0: ' Colombia', 174.0: ' Comoros', 175.0: ' Mayotte', 178.0: ' Congo', 180.0: ' Congo, the Democratic Republic of the', 184.0: ' Cook Islands', 188.0: ' Costa Rica', 191.0: ' Croatia', 192.0: ' Cuba', 196.0: ' Cyprus', 200.0: ' Czechoslovakia (former country)', 203.0: ' Czechia', 204.0: ' Benin', 208.0: ' Denmark', 212.0: ' Dominica', 214.0: ' Dominican Republic', 218.0: ' Ecuador', 222.0: ' El Salvador', 226.0: ' Equatorial Guinea', 231.0: ' Ethiopia', 232.0: ' Eritrea', 233.0: ' Estonia', 234.0: ' Faroe Islands', 238.0: ' Falkland Islands (Malvinas)', 239.0: ' South Georgia and the South Sandwich Islands', 242.0: ' Fiji', 246.0: ' Finland', 248.0: ' Åland Islands', 250.0: ' France', 254.0: ' French Guiana', 258.0: ' French Polynesia', 260.0: ' French Southern Territories', 262.0: ' Djibouti', 266.0: ' Gabon', 268.0: ' Georgia', 270.0: ' Gambia', 275.0: ' Palestine, State of', 276.0: ' Germany', 278.0: 'German Democratic Republic (former country)', 288.0: ' Ghana', 292.0: ' Gibraltar', 296.0: ' Kiribati', 300.0: ' Greece', 304.0: ' Greenland', 308.0: ' Grenada', 312.0: ' Guadeloupe', 316.0: ' Guam', 320.0: ' Guatemala', 324.0: ' Guinea', 328.0: ' Guyana', 332.0: ' Haiti', 334.0: ' Heard Island and McDonald Islands', 336.0: ' Holy See', 340.0: ' Honduras', 344.0: ' Hong Kong', 348.0: ' Hungary', 352.0: ' Iceland', 356.0: ' India', 360.0: ' Indonesia', 364.0: ' Iran, Islamic Republic of', 368.0: ' Iraq', 372.0: ' Ireland', 376.0: ' Israel', 380.0: ' Italy', 384.0: " Côte d'Ivoire", 388.0: ' Jamaica', 392.0: ' Japan', 398.0: ' Kazakhstan', 400.0: ' Jordan', 404.0: ' Kenya', 408.0: " Korea, Democratic People's Republic of", 410.0: ' Korea, Republic of', 414.0: ' Kuwait', 417.0: ' Kyrgyzstan', 418.0: " Lao People's Democratic Republic", 422.0: ' Lebanon', 426.0: ' Lesotho', 428.0: ' Latvia', 430.0: ' Liberia', 434.0: ' Libya', 438.0: ' Liechtenstein', 440.0: ' Lithuania', 442.0: ' Luxembourg', 446.0: ' Macao', 450.0: ' Madagascar', 454.0: ' Malawi', 458.0: ' Malaysia', 462.0: ' Maldives', 466.0: ' Mali', 470.0: ' Malta', 474.0: ' Martinique', 478.0: ' Mauritania', 480.0: ' Mauritius', 484.0: ' Mexico', 492.0: ' Monaco', 496.0: ' Mongolia', 498.0: ' Moldova, Republic of', 499.0: ' Montenegro', 500.0: ' Montserrat', 504.0: ' Morocco', 508.0: ' Mozambique', 512.0: ' Oman', 516.0: ' Namibia', 520.0: ' Nauru', 524.0: ' Nepal', 528.0: ' Netherlands', 531.0: ' Curaçao', 533.0: ' Aruba', 534.0: ' Sint Maarten (Dutch part)', 535.0: ' Bonaire, Sint Eustatius and Saba', 540.0: ' New Caledonia', 548.0: ' Vanuatu', 554.0: ' New Zealand', 558.0: ' Nicaragua', 562.0: ' Niger', 566.0: ' Nigeria', 570.0: ' Niue', 574.0: ' Norfolk Island', 578.0: ' Norway', 580.0: ' Northern Mariana Islands', 581.0: ' United States Minor Outlying Islands', 583.0: ' Micronesia, Federated States of', 584.0: ' Marshall Islands', 585.0: ' Palau', 586.0: ' Pakistan', 591.0: ' Panama', 598.0: ' Papua New Guinea', 600.0: ' Paraguay', 604.0: ' Peru', 608.0: ' Philippines', 612.0: ' Pitcairn', 616.0: ' Poland', 620.0: ' Portugal', 624.0: ' Guinea-Bissau', 626.0: ' Timor-Leste', 630.0: ' Puerto Rico', 634.0: ' Qatar', 638.0: ' Réunion', 642.0: ' Romania', 643.0: ' Russian Federation', 646.0: ' Rwanda', 652.0: ' Saint Barthélemy', 654.0: ' Saint Helena, Ascension and Tristan da Cunha', 659.0: ' Saint Kitts and Nevis', 660.0: ' Anguilla', 662.0: ' Saint Lucia', 663.0: ' Saint Martin (French part)', 666.0: ' Saint Pierre and Miquelon', 670.0: ' Saint Vincent and the Grenadines', 674.0: ' San Marino', 678.0: ' Sao Tome and Principe', 682.0: ' Saudi Arabia', 686.0: ' Senegal', 688.0: ' Serbia', 690.0: ' Seychelles', 694.0: ' Sierra Leone', 702.0: ' Singapore', 703.0: ' Slovak Republic', 704.0: ' Viet Nam', 705.0: ' Slovenia', 706.0: ' Somalia', 710.0: ' South Africa', 716.0: ' Zimbabwe', 724.0: ' Spain', 728.0: ' South Sudan', 729.0: ' Sudan', 732.0: ' Western Sahara', 740.0: ' Suriname', 744.0: ' Svalbard and Jan Mayen', 748.0: ' Swaziland', 752.0: ' Sweden', 756.0: ' Switzerland', 760.0: ' Syrian Arab Republic', 762.0: ' Tajikistan', 764.0: ' Thailand', 768.0: ' Togo', 772.0: ' Tokelau', 776.0: ' Tonga', 780.0: ' Trinidad and Tobago', 784.0: ' United Arab Emirates', 788.0: ' Tunisia', 792.0: ' Turkey', 795.0: ' Turkmenistan', 796.0: ' Turks and Caicos Islands', 798.0: ' Tuvalu', 800.0: ' Uganda', 804.0: ' Ukraine', 807.0: ' North Macedonia', 810.0: ' U.S.S.R. (former country)', 818.0: ' Egypt', 826.0: ' United Kingdom of Great Britain and Northern Ireland', 831.0: ' Guernsey', 832.0: ' Jersey', 833.0: ' Isle of Man', 834.0: ' Tanzania, United Republic of', 840.0: ' United States of America', 850.0: ' Virgin Islands, U.S.', 854.0: ' Burkina Faso', 858.0: ' Uruguay', 860.0: ' Uzbekistan', 862.0: ' Venezuela, Bolivarian Republic of', 876.0: ' Wallis and Futuna', 882.0: ' Samoa', 887.0: ' Yemen', 890.0: ' Yugoslavia (former country)', 891.0: 'Serbia and Montenegro (former country)', 894.0: ' Zambia', 915.0: ' Kosovo', 1111.0: ' Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'NaN', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 6117 | 96 |
v232 | 321 | v232 | numeric | mother born in [country] (Q70) | {1.0: 'yes', 2.0: 'no'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59218 | 2 |
v233b | 322 | v233b | nominal | mothers country of birth (ISO 3166-1/3 Alpha code) (Q71) | {'005': 'M49 code: South America', '011': 'M49 code: Western Africa', '013': 'M49 code: Central America', '014': 'M49 code: Eastern Africa', '015': 'M49 code: Northern Africa', '017': 'M49 code: Middle Africa', '018': 'M49 code: Southern Africa', '029': 'M49 code: Caribbean', '030': 'M49 code: Eastern Asia', '034': 'M49 code: Southern Asia', '035': 'M49 code: South-Eastern Asia', '039': 'M49 code: Southern Europe', '053': 'M49 code: Australia and New Zealand', '054': 'M49 code: Melanesia', '061': 'M49 code: Polynesia', '143': 'M49 code: Central Asia', '145': 'M49 code: Western Asia', '154': 'M49 code: Northern Europe', 'AD': 'Andorra', 'AE': 'United Arab Emirates', 'AF': 'Afghanistan', 'AG': 'Antigua and Barbuda', 'AI': 'Anguilla', 'AL': 'Albania', 'AM': 'Armenia', 'AO': 'Angola', 'AQ': 'Antarctica', 'AR': 'Argentina', 'AS': 'American Samoa', 'AT': 'Austria', 'AU': 'Australia', 'AW': 'Aruba', 'AX': 'Åland Islands', 'AZ': 'Azerbaijan', 'BA': 'Bosnia and Herzegovina', 'BB': 'Barbados', 'BD': 'Bangladesh', 'BE': 'Belgium', 'BF': 'Burkina Faso', 'BG': 'Bulgaria', 'BH': 'Bahrain', 'BI': 'Burundi', 'BJ': 'Benin', 'BL': 'Saint Barthélemy', 'BM': 'Bermuda', 'BN': 'Brunei Darussalam', 'BO': 'Bolivia (Plurinational State of)', 'BQ': 'Bonaire, Sint Eustatius and Saba', 'BR': 'Brazil', 'BS': 'Bahamas', 'BT': 'Bhutan', 'BV': 'Bouvet Island', 'BW': 'Botswana', 'BY': 'Belarus', 'BZ': 'Belize', 'CA': 'Canada', 'CC': 'Cocos (Keeling) Islands', 'CD': 'Congo (Democratic Republic of the)', 'CF': 'Central African Republic', 'CG': 'Congo', 'CH': 'Switzerland', 'CI': 'Côte d´Ivoire', 'CK': 'Cook Islands', 'CL': 'Chile', 'CM': 'Cameroon', 'CN': 'China', 'CO': 'Colombia', 'CR': 'Costa Rica', 'CSHH': 'Czechoslovakia (former country)', 'CSXX': 'Serbia and Montenegro (former country)', 'CU': 'Cuba', 'CV': 'Cabo Verde', 'CW': 'Curaçao', 'CX': 'Christmas Island', 'CY': 'Cyprus', 'CY-TCC': 'Northern Cyprus', 'CZ': 'Czechia', 'DDDE': 'German Democratic Republic (former country)', 'DE': 'Germany', 'DJ': 'Djibouti', 'DK': 'Denmark', 'DM': 'Dominica', 'DO': 'Dominican Republic', 'DZ': 'Algeria', 'EC': 'Ecuador', 'EE': 'Estonia', 'EG': 'Egypt', 'EH': 'Western Sahara', 'ER': 'Eritrea', 'ES': 'Spain', 'ET': 'Ethiopia', 'FI': 'Finland', 'FJ': 'Fiji', 'FK': 'Falkland Islands (Malvinas)', 'FM': 'Micronesia (Federated States of)', 'FO': 'Faroe Islands', 'FR': 'France', 'GA': 'Gabon', 'GB': 'United Kingdom of Great Britain and Northern Ireland', 'GD': 'Grenada', 'GE': 'Georgia', 'GF': 'French Guiana', 'GG': 'Guernsey', 'GH': 'Ghana', 'GI': 'Gibraltar', 'GL': 'Greenland', 'GM': 'Gambia', 'GN': 'Guinea', 'GP': 'Guadeloupe', 'GQ': 'Equatorial Guinea', 'GR': 'Greece', 'GS': 'South Georgia and the South Sandwich Islands', 'GT': 'Guatemala', 'GU': 'Guam', 'GW': 'Guinea-Bissau', 'GY': 'Guyana', 'HK': 'Hong Kong', 'HM': 'Heard Island and McDonald Islands', 'HN': 'Honduras', 'HR': 'Croatia', 'HT': 'Haiti', 'HU': 'Hungary', 'ID': 'Indonesia', 'IE': 'Ireland', 'IL': 'Israel', 'IM': 'Isle of Man', 'IN': 'India', 'IO': 'British Indian Ocean Territory', 'IQ': 'Iraq', 'IR': 'Iran (Islamic Republic of)', 'IS': 'Iceland', 'IT': 'Italy', 'JE': 'Jersey', 'JM': 'Jamaica', 'JO': 'Jordan', 'JP': 'Japan', 'KE': 'Kenya', 'KG': 'Kyrgyzstan', 'KH': 'Cambodia', 'KI': 'Kiribati', 'KM': 'Comoros', 'KN': 'Saint Kitts and Nevis', 'KP': 'Korea (Democratic People´s Republic of)', 'KR': 'Korea (Republic of)', 'KW': 'Kuwait', 'KY': 'Cayman Islands', 'KZ': 'Kazakhstan', 'LA': 'Lao People´s Democratic Republic', 'LB': 'Lebanon', 'LC': 'Saint Lucia', 'LI': 'Liechtenstein', 'LK': 'Sri Lanka', 'LR': 'Liberia', 'LS': 'Lesotho', 'LT': 'Lithuania', 'LU': 'Luxembourg', 'LV': 'Latvia', 'LY': 'Libya', 'MA': 'Morocco', 'MC': 'Monaco', 'MD': 'Moldova (Republic of)', 'ME': 'Montenegro', 'MF': 'Saint Martin (French part)', 'MG': 'Madagascar', 'MH': 'Marshall Islands', 'MK': 'North Macedonia', 'ML': 'Mali', 'MM': 'Myanmar', 'MN': 'Mongolia', 'MO': 'Macao', 'MP': 'Northern Mariana Islands', 'MQ': 'Martinique', 'MR': 'Mauritania', 'MS': 'Montserrat', 'MT': 'Malta', 'MU': 'Mauritius', 'MV': 'Maldives', 'MW': 'Malawi', 'MX': 'Mexico', 'MY': 'Malaysia', 'MZ': 'Mozambique', 'NA': 'Namibia', 'NC': 'New Caledonia', 'NE': 'Niger', 'NF': 'Norfolk Island', 'NG': 'Nigeria', 'NI': 'Nicaragua', 'NL': 'Netherlands', 'NO': 'Norway', 'NP': 'Nepal', 'NR': 'Nauru', 'NU': 'Niue', 'NZ': 'New Zealand', 'OM': 'Oman', 'PA': 'Panama', 'PE': 'Peru', 'PF': 'French Polynesia', 'PG': 'Papua New Guinea', 'PH': 'Philippines', 'PK': 'Pakistan', 'PL': 'Poland', 'PM': 'Saint Pierre and Miquelon', 'PN': 'Pitcairn', 'PR': 'Puerto Rico', 'PS': 'Palestine, State of', 'PT': 'Portugal', 'PW': 'Palau', 'PY': 'Paraguay', 'QA': 'Qatar', 'RE': 'Réunion', 'RO': 'Romania', 'RS': 'Serbia', 'RU': 'Russian Federation', 'RW': 'Rwanda', 'SA': 'Saudi Arabia', 'SB': 'Solomon Islands', 'SC': 'Seychelles', 'SD': 'Sudan', 'SE': 'Sweden', 'SG': 'Singapore', 'SH': 'Saint Helena, Ascension and Tristan da Cunha', 'SI': 'Slovenia', 'SJ': 'Svalbard and Jan Mayen', 'SK': 'Slovakia', 'SL': 'Sierra Leone', 'SM': 'San Marino', 'SN': 'Senegal', 'SO': 'Somalia', 'SR': 'Suriname', 'SS': 'South Sudan', 'ST': 'Sao Tome and Principe', 'SUHH': 'U.S.S.R. (former country)', 'SV': 'El Salvador', 'SX': 'Sint Maarten (Dutch part)', 'SY': 'Syrian Arab Republic', 'SZ': 'Swaziland', 'TC': 'Turks and Caicos Islands', 'TD': 'Chad', 'TF': 'French Southern Territories', 'TG': 'Togo', 'TH': 'Thailand', 'TJ': 'Tajikistan', 'TK': 'Tokelau', 'TL': 'Timor-Leste', 'TM': 'Turkmenistan', 'TN': 'Tunisia', 'TO': 'Tonga', 'TR': 'Turkey', 'TT': 'Trinidad and Tobago', 'TV': 'Tuvalu', 'TW': 'Taiwan, Province of China[a]', 'TZ': 'Tanzania, United Republic of', 'UA': 'Ukraine', 'UG': 'Uganda', 'UM': 'United States Minor Outlying Islands', 'US': 'United States of America', 'UY': 'Uruguay', 'UZ': 'Uzbekistan', 'VA': 'Holy See', 'VC': 'Saint Vincent and the Grenadines', 'VE': 'Venezuela (Bolivarian Republic of)', 'VG': 'Virgin Islands (British)', 'VI': 'Virgin Islands (U.S.)', 'VN': 'Viet Nam', 'VU': 'Vanuatu', 'WF': 'Wallis and Futuna', 'WS': 'Samoa', 'XK': 'Kosovo', 'XXX': 'Other', 'YE': 'Yemen', 'YT': 'Mayotte', 'YUCS': 'Yugoslavia (former country)', 'ZA': 'South Africa', 'ZM': 'Zambia', 'ZW': 'Zimbabwe'} | {'-1': "don't know/no answer", '-3': 'not applicable', '-5': 'other missing'} | True | 6121 | 98 |
v233b_r | 323 | v233b_r | nominal | mothers country of birth (ISO 3166-1 numeric code) (Q69) | {4.0: ' Afghanistan', 5.0: 'M49 code: South America', 8.0: ' Albania', 10.0: ' Antarctica', 11.0: 'M49 code: Western Africa', 12.0: ' Algeria', 13.0: 'M49 code: Central America', 14.0: 'M49 code: Eastern Africa', 15.0: 'M49 code: Northern Africa', 16.0: ' American Samoa', 17.0: 'M49 code: Middle Africa', 18.0: 'M49 code: Southern Africa', 20.0: ' Andorra', 24.0: ' Angola', 28.0: ' Antigua and Barbuda', 29.0: 'M49 code: Caribbean', 30.0: 'M49 code: Eastern Asia', 31.0: ' Azerbaijan', 32.0: ' Argentina', 34.0: 'M49 code: Southern Asia', 35.0: 'M49 code: South-Eastern Asia', 36.0: ' Australia', 39.0: 'M49 code: Southern Europe', 40.0: ' Austria', 44.0: ' Bahamas', 48.0: ' Bahrain', 50.0: ' Bangladesh', 51.0: ' Armenia', 52.0: ' Barbados', 53.0: 'M49 code: Australia and New Zealand', 54.0: 'M49 code: Melanesia', 56.0: ' Belgium', 60.0: ' Bermuda', 61.0: 'M49 code: Polynesia', 64.0: ' Bhutan', 68.0: ' Bolivia, Plurinational State of', 70.0: ' Bosnia and Herzegovina', 72.0: ' Botswana', 74.0: ' Bouvet Island', 76.0: ' Brazil', 84.0: ' Belize', 86.0: ' British Indian Ocean Territory', 90.0: ' Solomon Islands', 92.0: ' Virgin Islands, British', 96.0: ' Brunei Darussalam', 100.0: ' Bulgaria', 104.0: ' Myanmar', 108.0: ' Burundi', 112.0: ' Belarus', 116.0: ' Cambodia', 120.0: ' Cameroon', 124.0: ' Canada', 132.0: ' Cabo Verde', 136.0: ' Cayman Islands', 140.0: ' Central African Republic', 143.0: 'M49 code: Central Asia', 144.0: ' Sri Lanka', 145.0: 'M49 code: Western Asia', 148.0: ' Chad', 152.0: ' Chile', 154.0: 'M49 code: Northern Europe', 156.0: ' China', 158.0: ' Taiwan, Province of China', 162.0: ' Christmas Island', 166.0: ' Cocos (Keeling) Islands', 170.0: ' Colombia', 174.0: ' Comoros', 175.0: ' Mayotte', 178.0: ' Congo', 180.0: ' Congo, the Democratic Republic of the', 184.0: ' Cook Islands', 188.0: ' Costa Rica', 191.0: ' Croatia', 192.0: ' Cuba', 196.0: ' Cyprus', 200.0: ' Czechoslovakia (former country)', 203.0: ' Czechia', 204.0: ' Benin', 208.0: ' Denmark', 212.0: ' Dominica', 214.0: ' Dominican Republic', 218.0: ' Ecuador', 222.0: ' El Salvador', 226.0: ' Equatorial Guinea', 231.0: ' Ethiopia', 232.0: ' Eritrea', 233.0: ' Estonia', 234.0: ' Faroe Islands', 238.0: ' Falkland Islands (Malvinas)', 239.0: ' South Georgia and the South Sandwich Islands', 242.0: ' Fiji', 246.0: ' Finland', 248.0: ' Åland Islands', 250.0: ' France', 254.0: ' French Guiana', 258.0: ' French Polynesia', 260.0: ' French Southern Territories', 262.0: ' Djibouti', 266.0: ' Gabon', 268.0: ' Georgia', 270.0: ' Gambia', 275.0: ' Palestine, State of', 276.0: ' Germany', 278.0: 'German Democratic Republic (former country)', 288.0: ' Ghana', 292.0: ' Gibraltar', 296.0: ' Kiribati', 300.0: ' Greece', 304.0: ' Greenland', 308.0: ' Grenada', 312.0: ' Guadeloupe', 316.0: ' Guam', 320.0: ' Guatemala', 324.0: ' Guinea', 328.0: ' Guyana', 332.0: ' Haiti', 334.0: ' Heard Island and McDonald Islands', 336.0: ' Holy See', 340.0: ' Honduras', 344.0: ' Hong Kong', 348.0: ' Hungary', 352.0: ' Iceland', 356.0: ' India', 360.0: ' Indonesia', 364.0: ' Iran, Islamic Republic of', 368.0: ' Iraq', 372.0: ' Ireland', 376.0: ' Israel', 380.0: ' Italy', 384.0: " Côte d'Ivoire", 388.0: ' Jamaica', 392.0: ' Japan', 398.0: ' Kazakhstan', 400.0: ' Jordan', 404.0: ' Kenya', 408.0: " Korea, Democratic People's Republic of", 410.0: ' Korea, Republic of', 414.0: ' Kuwait', 417.0: ' Kyrgyzstan', 418.0: " Lao People's Democratic Republic", 422.0: ' Lebanon', 426.0: ' Lesotho', 428.0: ' Latvia', 430.0: ' Liberia', 434.0: ' Libya', 438.0: ' Liechtenstein', 440.0: ' Lithuania', 442.0: ' Luxembourg', 446.0: ' Macao', 450.0: ' Madagascar', 454.0: ' Malawi', 458.0: ' Malaysia', 462.0: ' Maldives', 466.0: ' Mali', 470.0: ' Malta', 474.0: ' Martinique', 478.0: ' Mauritania', 480.0: ' Mauritius', 484.0: ' Mexico', 492.0: ' Monaco', 496.0: ' Mongolia', 498.0: ' Moldova, Republic of', 499.0: ' Montenegro', 500.0: ' Montserrat', 504.0: ' Morocco', 508.0: ' Mozambique', 512.0: ' Oman', 516.0: ' Namibia', 520.0: ' Nauru', 524.0: ' Nepal', 528.0: ' Netherlands', 531.0: ' Curaçao', 533.0: ' Aruba', 534.0: ' Sint Maarten (Dutch part)', 535.0: ' Bonaire, Sint Eustatius and Saba', 540.0: ' New Caledonia', 548.0: ' Vanuatu', 554.0: ' New Zealand', 558.0: ' Nicaragua', 562.0: ' Niger', 566.0: ' Nigeria', 570.0: ' Niue', 574.0: ' Norfolk Island', 578.0: ' Norway', 580.0: ' Northern Mariana Islands', 581.0: ' United States Minor Outlying Islands', 583.0: ' Micronesia, Federated States of', 584.0: ' Marshall Islands', 585.0: ' Palau', 586.0: ' Pakistan', 591.0: ' Panama', 598.0: ' Papua New Guinea', 600.0: ' Paraguay', 604.0: ' Peru', 608.0: ' Philippines', 612.0: ' Pitcairn', 616.0: ' Poland', 620.0: ' Portugal', 624.0: ' Guinea-Bissau', 626.0: ' Timor-Leste', 630.0: ' Puerto Rico', 634.0: ' Qatar', 638.0: ' Réunion', 642.0: ' Romania', 643.0: ' Russian Federation', 646.0: ' Rwanda', 652.0: ' Saint Barthélemy', 654.0: ' Saint Helena, Ascension and Tristan da Cunha', 659.0: ' Saint Kitts and Nevis', 660.0: ' Anguilla', 662.0: ' Saint Lucia', 663.0: ' Saint Martin (French part)', 666.0: ' Saint Pierre and Miquelon', 670.0: ' Saint Vincent and the Grenadines', 674.0: ' San Marino', 678.0: ' Sao Tome and Principe', 682.0: ' Saudi Arabia', 686.0: ' Senegal', 688.0: ' Serbia', 690.0: ' Seychelles', 694.0: ' Sierra Leone', 702.0: ' Singapore', 703.0: ' Slovak Republic', 704.0: ' Viet Nam', 705.0: ' Slovenia', 706.0: ' Somalia', 710.0: ' South Africa', 716.0: ' Zimbabwe', 724.0: ' Spain', 728.0: ' South Sudan', 729.0: ' Sudan', 732.0: ' Western Sahara', 740.0: ' Suriname', 744.0: ' Svalbard and Jan Mayen', 748.0: ' Swaziland', 752.0: ' Sweden', 756.0: ' Switzerland', 760.0: ' Syrian Arab Republic', 762.0: ' Tajikistan', 764.0: ' Thailand', 768.0: ' Togo', 772.0: ' Tokelau', 776.0: ' Tonga', 780.0: ' Trinidad and Tobago', 784.0: ' United Arab Emirates', 788.0: ' Tunisia', 792.0: ' Turkey', 795.0: ' Turkmenistan', 796.0: ' Turks and Caicos Islands', 798.0: ' Tuvalu', 800.0: ' Uganda', 804.0: ' Ukraine', 807.0: ' North Macedonia', 810.0: ' U.S.S.R. (former country)', 818.0: ' Egypt', 826.0: ' United Kingdom of Great Britain and Northern Ireland', 831.0: ' Guernsey', 832.0: ' Jersey', 833.0: ' Isle of Man', 834.0: ' Tanzania, United Republic of', 840.0: ' United States of America', 850.0: ' Virgin Islands, U.S.', 854.0: ' Burkina Faso', 858.0: ' Uruguay', 860.0: ' Uzbekistan', 862.0: ' Venezuela, Bolivarian Republic of', 876.0: ' Wallis and Futuna', 882.0: ' Samoa', 887.0: ' Yemen', 890.0: ' Yugoslavia (former country)', 891.0: 'Serbia and Montenegro (former country)', 894.0: ' Zambia', 915.0: ' Kosovo', 1111.0: ' Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'NaN', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 6121 | 98 |
v234 | 324 | v234 | numeric | current legal marital status respondent (Q72) | {1.0: 'married', 2.0: 'registered partnership', 3.0: 'widowed', 4.0: 'divorced', 5.0: 'separated', 6.0: 'never married and never registered partnership'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59020 | 6 |
v235 | 325 | v235 | numeric | lived with partner before marriage (Q73) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 43987 | 2 |
v236 | 326 | v236 | numeric | living with partner (Q74) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27301 | 2 |
v237 | 327 | v237 | numeric | having steady relationship (Q75) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 19888 | 2 |
v238 | 328 | v238 | numeric | do you live with your parents/parents in law (Q76) | {1.0: 'no', 2.0: 'yes, own parent(s)', 3.0: 'yes, parent(s) in law', 4.0: 'yes, both own parent(s) and parent(s) in law'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59001 | 4 |
v239_r | 329 | v239_r | nominal | number of children in/outside HH (constructed) (Q77) | {0.0: 'no children', 5.0: '5 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58952 | 6 |
v239a | 330 | v239a | numeric | number of children in household (Q77A) | {4.0: '4 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58094 | 5 |
v239b | 331 | v239b | numeric | number of children outside household (Q77B) | {3.0: '3 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58114 | 4 |
v240 | 332 | v240 | numeric | number of people in household (Q78) | {1.0: 'I live alone', 6.0: '6 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58691 | 6 |
v241 | 333 | v241 | numeric | age of youngest person in household (Q79) | {80.0: '80 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 45983 | 81 |
v242 | 334 | v242 | numeric | age completed education respondent (Q80) | {7.0: '7 and younger', 70.0: '70 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 0.0: 'no formal education'} | True | 55834 | 64 |
v242_r | 335 | v242_r | nominal | What age did you complete your education (recoded in intervals) (Q80) | {1.0: '<=12 years', 2.0: '13 years', 3.0: '14 years', 4.0: '15 years', 5.0: '16 years', 6.0: '17 years', 7.0: '18 years', 8.0: '19 years', 9.0: '20 years', 10.0: '21+ years'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 0.0: 'no formal education'} | True | 55834 | 10 |
v243_edulvlb | 336 | v243_edulvlb | nominal | educational level respondent: ESS-edulvlb coding (Q81) | {0.0: 'Not completed ISCED 1', 113.0: 'Completed ISCED 1', 129.0: 'short vocational ISCED 2', 212.0: 'General/pre-vocational ISCED 2 with access to 32x', 213.0: 'General ISCED 2 with access to all ISCED 3', 221.0: 'Vocational ISCED 2 without access to ISCED 3', 222.0: 'Vocational ISCED 2 with access to 32x only', 223.0: 'Vocational ISCED 2 with access to all ISCED 3', 229.0: 'short vocational ISCED 3', 311.0: 'General ISCED 3 without access to tertiary', 312.0: 'General ISCED 3 with access to non-university tertiary only', 313.0: 'General ISCED 3 with access to university', 321.0: 'Vocational ISCED 3 without access to tertiary', 322.0: 'Vocational ISCED 3 with access to non-university tertiary only', 323.0: 'Vocational ISCED 3 with access to university', 412.0: 'General ISCED 4, with access to non-university tertiary only', 413.0: 'General ISCED 4 with access to all first degrees at ISCED 6/7', 421.0: 'Vocational ISCED 4 without access to tertiary', 422.0: 'Vocational ISCED 4, with access to non-university tertiary only', 423.0: 'Vocational ISCED 4, with access to university', 510.0: "University qualification below bachelor's degree", 520.0: "Non-university qualification below bachelor's degree", 610.0: "Non-university bachelor's degree", 620.0: "University bachelor's degree", 710.0: "Non-university master's degree", 720.0: "University master's degree", 800.0: 'Doctoral degree', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 28 |
v243_edulvlb_2 | 337 | v243_edulvlb_2 | nominal | educational level respondent: ESS-edulvlb coding two digits (Q81) | {0.0: 'Less than primary', 11.0: 'Primary: general education', 12.0: 'Primary: vocational programmes', 21.0: 'Lower secondary: general education', 22.0: 'Lower secondary: vocational programmes', 31.0: 'Upper secondary: general education', 32.0: 'Upper secondary: vocational programmes', 41.0: 'Post-secondary non tertiary: general education', 42.0: 'Post-secondary non tertiary: vocational programmes', 51.0: 'Short-cycle tertiary: general education', 52.0: 'Short-cycle tertiary: vocational programmes', 61.0: 'Bachelor or equivalent: general education', 62.0: 'Bachelor or equivalent: vocational programmes', 71.0: 'Master or equivalent: general education', 72.0: 'Master or equivalent: vocational programmes', 80.0: 'Doctoral or equivalent', 666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 17 |
v243_edulvlb_1 | 338 | v243_edulvlb_1 | nominal | educational level respondent: ESS-edulvlb coding one digit (Q81) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 10 |
v243_ISCED_3 | 339 | v243_ISCED_3 | numeric | educational level respondent: ISCED-code three digit (Q81) | {0.0: 'Less than primary', 100.0: 'Primary', 244.0: 'General lower secondary with direct access to upper secondary', 253.0: 'Vocational lower secondary without direct access to upper secondary', 254.0: 'Vocational lower secondary with direct access to upper secondary', 343.0: 'General upper secondary without direct access to first tertiary programmes', 344.0: 'General upper secondary with direct access to first tertiary programmes', 353.0: 'Vocational upper secondary without direct access to first tertiary programmes', 354.0: 'Vocational upper secondary with direct access to first tertiary programmes', 444.0: 'General post-secondary non-tertiary with direct access to first tertiary programmes', 453.0: 'Vocational post-secondary non-tertiary without direct access to first tertiary programmes', 454.0: 'Vocational post-secondary non-tertiary with direct access to first tertiary programmes', 540.0: 'General short-cycle tertiary', 550.0: 'Vocational short-cycle tertiary', 640.0: 'Academic Bachelor’s or equivalent level', 650.0: 'Professional Bachelor’s or equivalent level', 740.0: 'Academic Master’s or equivalent level', 750.0: 'Professional Master’s or equivalent level', 800.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 20 |
v243_ISCED_2 | 340 | v243_ISCED_2 | numeric | educational level respondent: ISCED-code two digit (Q81) | {0.0: 'Pre-primary education', 10.0: 'Primary education', 24.0: 'Lower secondary general education', 25.0: 'Lower secondary vocational education', 34.0: 'Upper secondary general education', 35.0: 'Upper secondary vocational education', 44.0: 'Post-secondary non-tertiary general education', 45.0: 'Post-secondary non-tertiary vocational education', 54.0: 'Short-cycle tertiary general education', 55.0: 'Short-cycle tertiary vocational education', 64.0: 'Bachelor’s or equivalent level, academic', 65.0: 'Bachelor’s or equivalent level, professional', 74.0: 'Master’s or equivalent level, academic', 75.0: 'Master’s or equivalent level, professional', 80.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 16 |
v243_ISCED_2b | 341 | v243_ISCED_2b | nominal | educational level respondent: ISCED-code two digit, second option (Q81) | {0.0: 'Less than primary', 10.0: 'Primary', 23.0: 'Lower secondary, no access to upper secondary education', 24.0: 'Lower secondary, access to upper secondary education', 33.0: 'Upper secondary, no access to upper secondary education', 34.0: 'Upper secondary, access to upper secondary education', 43.0: 'Post-secondary non-tertiary, no access to upper secondary education', 44.0: 'Post-secondary non-tertiary, access to upper secondary education', 50.0: 'Short-cycle tertiary education', 60.0: 'Bachelor’s or equivalent level', 70.0: 'Master or equivalent level', 80.0: 'Doctorate or equivalent level', 6666.0: 'Other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59050 | 13 |
v243_ISCED_1 | 342 | v243_ISCED_1 | nominal | educational level respondent: ISCED‐code one digit (Q81) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 10 |
v243_EISCED | 343 | v243_EISCED | numeric | educational level respondent: ES-ISCED coding (Q81) | {0.0: 'No formal or less than primary education', 1.0: 'I - Primary education', 2.0: 'II - Lower secondary (including vocational training that is not considered as completion of upper secondary education)', 3.0: 'IIIb - Upper secondary without access to higher education', 4.0: 'IIIa - Upper secondary with access to higher education', 5.0: 'IV - Post-secondary/advanced vocational education below bachelor’s degree level', 6.0: "V1 - Bachelor's level", 7.0: "V2 - Master's and higher level", 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59049 | 9 |
v243_ISCED97 | 344 | v243_ISCED97 | numeric | educational level respondent: ISCED97‐code one digit (Q81) | {0.0: ' 0 : Pre-primary education or none education', 1.0: ' 1 : Primary education or first stage of basic education', 2.0: ' 2 : Lower secondary or second stage of basic education', 3.0: ' 3 : (Upper) secondary education', 4.0: ' 4 : Post-secondary non-tertiary education', 5.0: ' 5 : First stage of tertiary education', 6.0: ' 6 : Second stage of tertiary education'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59049 | 8 |
v243_8cat | 345 | v243_8cat | numeric | educational level respondent: 8 categories (Q81) | {1.0: ' Inadequately completed elementary education', 2.0: ' Completed (compulsory) elementary education', 3.0: ' Incomplete secondary school: technical/vocational type', 4.0: ' Complete secondary school: technical/vocational type/secondary', 5.0: ' Incomplete secondary: university-preparatory type/secondary', 6.0: ' Complete secondary: university-preparatory type/full secondary', 7.0: ' Some university without degree/higher education - lower-level tertiary', 8.0: ' University with degree/higher education - upper-level tertiary'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59049 | 9 |
v243_r | 346 | v243_r | nominal | educational level respondent: recoded (Q81) | {1.0: 'lower', 2.0: 'medium', 3.0: 'higher', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59050 | 4 |
v243_cs | 347 | v243_cs | numeric | educational level respondent: country-specific, ISO 3166-1 (Q81) | {801.0: ' AL: Without school', 802.0: ' AL: Primary education', 803.0: ' AL: Lower secondary (Leaving certificate)', 804.0: ' AL: Upper secondary general (Maturity diploma)', 805.0: ' AL: Upper secondary vocational (2 years, certificate of high technical or vocational education)', 806.0: ' AL: Upper secondary vocational (over 2 years, Maturity diplome)', 807.0: ' AL: Post-secondary non tertiary', 808.0: ' AL: University diploma/Bachelor', 809.0: ' AL: Vocational/Professional Master', 810.0: ' AL: Post-University', 811.0: ' AL: Master of Arts', 812.0: ' AL: Master of Science', 813.0: ' AL: Long term specialization', 814.0: ' AL: Doctoral Degree', 877.0: ' AL: not applicable', 888.0: ' AL: don’t know (spontaneous)', 899.0: ' AL: no answer (spontaneous)', 3101.0: ' AZ: None', 3102.0: ' AZ: Primary education (1-4 years)', 3103.0: ' AZ: Incomplete general secondary (5-8 grades)', 3104.0: ' AZ: General secondary education (9 grades)', 3105.0: ' AZ: Complete secondary education (11 grades)', 3106.0: ' AZ: Primary vocational-professional education (Vocational school/lyceum) (1-3 years)', 3107.0: ' AZ: Secondary special education (Technical school/College) (2-4 years)', 3108.0: ' AZ: Bachelor degree (4 years)', 3109.0: ' AZ: Master degree (2 years)', 3110.0: ' AZ: Science degree (former Aspirantura/PhD): Doctor of Philosophy, Doctor of Science', 3188.0: ' AZ: don’t know (spontaneous)', 3199.0: ' AZ: no answer (spontaneous)', 4001.0: 'AT: Not completed', 4002.0: 'AT: Completed primary education', 4003.0: 'AT: Completed Lower Secundary Education, New Secundary School', 4004.0: 'AT: Completed Polytechnic School, or one-year Middle School', 4005.0: 'AT: Completed Academic Secondary School (AHS), elementary level', 4006.0: 'AT: Completed apprenticeship (final apprenticeship examination)', 4007.0: 'AT: Completed Vocational Middle School (at least 2 years, e.g. commercial school, professional school)', 4008.0: 'AT: Completed Higher School Certificate (matriculation, general qualification for university entrance)', 4009.0: 'AT: Completed degree in health or nursing care', 4010.0: 'AT: Completed Higher School Certificate in Vocational Higher School', 4011.0: 'AT: Completed advanced vocational training', 4012.0: 'AT: Completed pedagoical/educational/medical degree', 4013.0: "AT: Completed Bachelor's degree at a university of applied sciences", 4014.0: "AT: Completed Bachelor's degree at a university", 4015.0: "AT: Completed Master's degree at a university of applied sciences", 4016.0: "AT: Completed Master's degree at a university of applied sciences", 4017.0: 'AT: Completed post-graduate university courses (e.g. MBA)', 4018.0: 'AT: Completed doctorate/PhD', 4019.0: 'AT: other', 5101.0: 'AM: Never went to school', 5102.0: 'AM: Less than 4 years of education', 5103.0: 'AM: 4 years of primary education', 5104.0: 'AM: Certificate of lower secondary/ basic secondary education, 9 years', 5105.0: 'AM: Certificate of initial vocational education after lower /basic education', 5106.0: 'AM: Advanced vocational education after lower /basic education', 5107.0: 'AM: Certificate of upper secondary education, 12 years', 5108.0: 'AM: Certificate of initial vocational training after upper secondary education', 5109.0: 'AM: Advanced vocational education after upper secondary education', 5110.0: 'AM: Bachelor degree 4 years', 5111.0: 'AM: Specialist degree', 5112.0: 'AM: Master degree 2 years', 5113.0: 'AM: Phd and habilitation', 7001.0: 'BA: No school (up to three grades of elementary school)', 7002.0: 'BA: Unfinished elementary school (4 to 7 grades)', 7003.0: 'BA: Completed elementary school', 7004.0: 'BA: Secondary vocational school up to 1-2 years', 7005.0: 'BA: Secondary vocational school up to 3 years', 7006.0: 'BA: Technical and related secondary vocational schools up to 4 years or more', 7007.0: 'BA: High school', 7008.0: 'BA: Professional study up to 2-3 years', 7009.0: "BA: Undergraduate university study - for the academic title 'baccalaureus' (3-4 years); Art academy (undergraduate stud", 7010.0: 'BA: Specialist graduate professional study (4-5 years); Specialist in the profession', 7011.0: 'BA: Graduate university study (4-6 years) - old program', 7012.0: "BA: Master's degree (5 years); Art academy (graduate study)", 7013.0: "BA: Master's degree of Science or completed postgraduate specialist studies", 7014.0: 'BA: Postgraduate Doctorate of Science', 10001.0: 'BG: Incomplete primary education', 10002.0: 'BG: Primary education', 10003.0: 'BG: Basic education', 10004.0: 'BG: Secondary general education', 10005.0: 'BG: Secondary special education Language Schools, Mathematics and Natural Science', 10006.0: 'BG: Secondary special education Arts and Sports', 10007.0: 'BG: Secondary vocational education', 10008.0: 'BG: Semi-higher education', 10009.0: 'BG: College - Professional bachelor', 10010.0: 'BG: Higher education - Bachelor', 10011.0: 'BG: Higher education - Master', 10012.0: 'BG: Doctor of science', 11201.0: 'BY: No education', 11202.0: 'BY: Primary school', 11203.0: 'BY: Certificate of lower secondary general education', 11204.0: 'BY: Certificate of upper general secondary education', 11205.0: 'BY: 1-2 year vocational education after lower secondary education', 11206.0: 'BY: Vocational training with upper secondary general training in parallel', 11207.0: 'BY: Certificate of upper vocational education with upper secondary general education', 11208.0: "BY: Bachelor's Degree", 11209.0: "BY: Higher education, Specialist's Diploma", 11210.0: "BY: Master's degree", 11211.0: 'BY: PhD', 11212.0: 'BY: Habilitation', 19101.0: 'HR: No school (up to three grades of elementary school)', 19102.0: 'HR: Unfinished elementary school (4 to 7 grades)', 19103.0: 'HR: Completed elementary school', 19104.0: 'HR: Secondary vocational school up to 1-2 years', 19105.0: 'HR: Secondary vocational school up to 3 years', 19106.0: 'HR: Technical and related secondary vocational schools up to 4 years or more', 19107.0: 'HR: High school', 19108.0: 'HR: Professional study up to 2-3 years', 19109.0: "HR: Undergraduate university study; for academic title 'baccalaureus' (3-4 years); Art academy (undergraduate study)", 19110.0: 'HR: Specialist graduate professional study (4-5 years); Specialist in the profession', 19111.0: 'HR: Graduate university study (4-6 years) - old program', 19112.0: "HR: Master's degree (5 years); Art academy (graduate study)", 19113.0: "HR: Master's degree of Science or completed postgraduate specialist studies", 19114.0: 'HR: Postgraduate Doctorate of Science', 20301.0: 'CZ Incomplete elementary education, unfinished 1st level (less than 5 grades/years)', 20302.0: 'CZ: Incomplete basic education (5+ years of schooling, only primary school completed, SZŠ, ZZŠ, general school)', 20303.0: 'CZ: Basic education (bougeois school)', 20304.0: 'CZ: Secondary education with an apprenticeship certificate, Secondary education without school-leaving examination', 20305.0: 'CZ: Secondary education without leaving examination followed by further studies with final exam (teaching, retraining)', 20306.0: 'CZ: Education with GCSE, full secondary vocational education with GCSE', 20307.0: 'CZ: Secondary educ. with school-leaving exam followed by studies with final exam (post-gr., follow-up courses etc)', 20308.0: 'CZ: Secondary general education with school-leaving examination (grammar school)', 20309.0: 'CZ: Post-secondary education with diploma: Higher vocational school (DiS), 5th and 6th year of conservatory (discharge)', 20310.0: "CZ: Bachelor's degree", 20311.0: "CZ: Master's degree education (Mgr., Ing., Ing., MUDr., MDDr., MVDr., …)", 20312.0: 'CZ: Doctoral studies, postgraduate education (Ph.D., Th.D., CSc, ...)', 20801.0: ' DK: municipal primary and lower secondary school: 5th grade or lower', 20802.0: ' DK: municipal primary and lower secondary school: 6th grade to 8th grade', 20803.0: ' DK: municipal primary and lower secondary school: 9th grade to 10th grade', 20804.0: " DK: '(Upper) Secondary Education: Gymnasium, Higher Preparatory Examination (HF), Higher Commercial Examination Program", 20805.0: ' DK: Semiskilled worker courses and short vocational education (less than 2 years)', 20806.0: ' DK: Vocational and technical (upper) secondary education', 20807.0: ' DK: Short-cycle higher non-university programmes (2-3 years)', 20808.0: ' DK: medium-cycle university and non-university programmes, proffesional bachelor degree (3-4 years)', 20809.0: ' DK: long-cycle university programmes, 1 part - university bachelor degree', 20810.0: ' DK: long-cycle university programmes, 2. part Kandidat degree (Masters) (5-6 years)', 20811.0: ' DK: Licentiat (closed research education program)', 20812.0: ' DK: Research Education, PhD., Doctor', 20813.0: ' DK: other', 23301.0: 'EE: Without primary education (less than 4 grades)', 23302.0: 'EE: Completed primary education (4-6 grades)', 23303.0: 'EE: Vocational education without completed primary education', 23304.0: 'EE: Completed basic education (7-9 grades)', 23305.0: 'EE: Vocational secondary education after basic education (training programme under 2 years)', 23306.0: 'EE: Completed secondary education', 23307.0: 'EE: Vocational secondary education after basic education (training programme 2 years or more)', 23308.0: 'EE: Vocational secondary education with secondary education or vocational secondary education (tehnical education) afte', 23309.0: 'EE: Vocational-secondary education (tehnical education) after secondary education', 23310.0: 'EE: Vocational university after secondary education diploma (up to 2 years trainings, but not Bachelor of Arts)', 23311.0: 'EE: Higher vocational education diploma or Bachelor of Arts degree (3-4 years of trainings)', 23312.0: 'EE: University Bachelor of Arts degree (3-4 years of trainings', 23313.0: 'EE: Master of Arts from Vocational university', 23314.0: 'EE: Master of Arts\xa0(3+2, 4+2 or by 5+4 system, incl. integrated BA and MA), higher education started before 1992 (speci', 23315.0: "EE: Doctor's degree", 24601.0: ' FI: Less than primary school (grades 1-6) or less than comprehensive school', 24602.0: " FI: Grades 1-6 of comprehensive school, or primary school or people's school", 24603.0: ' FI: Grades 7-9 of comprehensive school, or middle school', 24604.0: ' FI: General upper secondary school, matriculation examination', 24605.0: ' FI: Vocational upper secondary qualification, further vocational qualification', 24606.0: ' FI: Both matriculation examination and vocational qualification', 24607.0: ' FI: Specialist vocational qualification', 24608.0: ' FI: Post-secondary level vocational qualification (old)', 24609.0: " FI: Bachelors' degree in universities of applied sciences (polytechnic)", 24610.0: " FI: Bachelors' degree in university", 24611.0: " FI: Master's degree in universities of applied sciences (polytechnic)", 24612.0: " FI: Master's degree in university", 24613.0: ' FI: Licentiate degree', 24614.0: ' FI: Doctoral degree', 24615.0: ' FI: other', 25001.0: ' FR: no schooling or not completed primary education', 25002.0: " FR: Completed primary education, without the final certificate (Certificat d'études primaires=an old qualification off", 25003.0: " FR: Completed primary education, with the Certificat d'études primaires", 25004.0: " FR: Secondary education 1st cycle, without the final certificate (Brevet élémentaire, Brevet d'étude du premier cycl", 25005.0: " FR: Secondary education 1st cycle, with the final certificate (Brevet élémentaire, Brevet d'étude du premier cycle,", 25006.0: ' FR: Secondary education 2nd cycle, without the final exam (Baccalauréat)', 25007.0: " FR: Certificate of vocational ability : Certificat d'Aptitude Professionnelle (CAP) or Brevet d'Etudes Professionnelles", 25008.0: ' FR: Vocational qualification in care (home care, children care, health care)', 25009.0: ' FR: Vocational Baccalaureat', 25010.0: ' FR: Technological Baccalaureat or equivalent diploma', 25011.0: ' FR: General Baccalaureat or equivalent diploma', 25012.0: ' FR: Certificate of ability in Law, Diploma allowing admission in tertiary education', 25013.0: ' FR: Qualifications of vocational secondary education in health and care institutions (like Moniteur éducateur and equi', 25014.0: ' FR: University education, 1st cycle Diploma in general tertiary education (2 years), classes for competitive entrance', 25015.0: ' FR: Diploma in Technological Studies , Diploma of Advanced Technician', 25016.0: ' FR: Qualification in paramedical and social sectors (social assistant, etc,)', 25017.0: ' FR: Vocational Bachelor (University education, 1st graduation and apprenticeship)', 25018.0: ' FR: Bachelor (University education, 2nd cycle, 1st year and apprenticeship)', 25019.0: ' FR: Engineer Degree', 25020.0: ' FR: Vocational Master Degree', 25021.0: ' FR: Intermediate Master degree (university education, 2nd cycle, 2nd year), qualification of primary and secondary educ', 25022.0: ' FR: Research Master Degree, qualification of university education teachers', 25023.0: ' FR: Qualifications of architects, veterinaries, notaries', 25024.0: ' FR: Diplôma from selective “grandes écoles”', 25025.0: ' FR: Doctorate in medecine, odontology, pharmacy', 25026.0: ' FR: Doctorate', 26801.0: 'GE: No education', 26802.0: 'GE: Less than 6 years primary education', 26803.0: 'GE: Primary education completed, 6 years', 26804.0: 'GE: Basic/ lower general education certificate, 9 years', 26805.0: 'GE: Upper secondary general education certificate', 26806.0: 'GE: Professional diploma level 1, 2, or 3 (Vocational Education certificate, before 2010)', 26807.0: 'GE: Professional diploma level 4/5 (Secondary Vocational Educ. Certificate pre-2010, Certified Diploma 2008-2010)', 26808.0: 'GE: Interim qualification, short cycle tertiary education', 26809.0: 'GE: Bachelor degree', 26810.0: 'GE: Master degree', 26811.0: 'GE: Specialist studies', 26812.0: 'GE: Doctoral degree', 34801.0: 'HU: Never attented school; 1-3 years of primary school or equivalent', 34802.0: 'HU: 4-7 years of primary school or equivalent', 34803.0: 'HU: Completed primary school or equivalent', 34804.0: 'HU: Vocational school', 34805.0: 'HU: Vocational training after the 10. grade', 34806.0: 'HU: Completed secondary vocational education with school-leaving exam', 34807.0: 'HU: Completed secondary general education (Gymnasium) with school-leaving exam', 34808.0: 'HU: Post-secondary, mon-tertiary vocational education and training (E.g. technical school)', 34809.0: 'HU: Short-cycle tertiary level vocational education and training (VET)', 34810.0: "HU: College degree or college bachelor's degree - BA/BSc", 34811.0: "HU: University bachelor's degree - BA/BSc", 34812.0: "HU: College master's degree - MA/MSc", 34813.0: "HU: University degree or university master's degree - MA/MSc", 34814.0: 'HU: Doctoral degree', 35201.0: 'IS: Less than primary education', 35202.0: 'IS: Primary education', 35203.0: 'IS: Elementary school, 14–15 years of age', 35204.0: 'IS: National test', 35205.0: 'IS: Elementary school, 15–16 years of age (compulsory education)', 35206.0: 'IS: Elementary school, 16–17 years of age', 35207.0: 'IS: Vocational education at the lower secondary level', 35208.0: 'IS: Post-secondary non-tertiary education', 35209.0: 'IS: Other post-secondary, non-tertiary vocational education', 35210.0: 'IS: Upper secondary art education, 2+ years', 35211.0: 'IS: Certified trades, school diploma', 35212.0: 'IS: Matriculation examination', 35213.0: 'IS: Upper secondary education', 35214.0: 'IS: Master of a certified trade', 35215.0: 'IS: Tertiary general education, 2–3 years', 35216.0: 'IS: Tertiary vocational education, 2–3 years', 35217.0: 'IS: Bachelor’s or equivalent level', 35218.0: 'IS: Master’s or equivalent level', 35219.0: 'IS: Doctoral or equivalent level', 38001.0: ' IT: No title', 38002.0: ' IT: Elementary school diploma', 38003.0: ' IT: Vocational training', 38004.0: ' IT: Middle school diploma', 38005.0: ' IT: Post-obligation vocational regional qualification', 38006.0: " IT: Vocational institute diploma (2 or 3 years), including art teacher diploma, teacher's training school diploma and n", 38007.0: ' IT: High school diploma (5 years), technical or vocational institute, including art institute five years diploma', 38008.0: ' IT: High school diploma (4 or 5 years), classical, scientific, linguistic or psyco-pedagogical, musical lyceum, includi', 38009.0: ' IT: Post-(high school) diploma specialization, post-(high school) diploma vocational regional qualification, certificat', 38010.0: ' IT: Former system academic diploma (2 or 3 years), including higher institute of physical education, social service and', 38011.0: ' IT: Tertiary education not academic diploma: Music Conservatory, Academy of Fine Arts and similar', 38012.0: ' IT: Three years or first level degree (bachelor)', 38013.0: ' IT: First level specialization degree', 38014.0: " IT: Former system degree, master's degree, single cycle degree", 38015.0: ' IT: Second level specialization degree', 38016.0: ' IT: Post-graduate specialization (1 or 2 years)', 38017.0: ' IT: Post-graduate specialization (3 or 4 years), including medical specialization', 38018.0: ' IT: Doctoral degree', 42801.0: ' LV: Has not attended school', 42802.0: ' LV: Incompete primary education ( 6 grades)', 42803.0: ' LV: General primary education', 42804.0: ' LV: Basic vocational education', 42805.0: ' LV: Vocational education (without secondary) (1 year)', 42806.0: ' LV: Vocational education (without secondary) (3 years)', 42807.0: ' LV: General secondary education', 42808.0: ' LV: Vocational secondary education', 42809.0: ' LV: Vocational (not higher) education after general secondary education', 42810.0: ' LV: 1st level professional/ vocational higher education (college)', 42811.0: " LV: Bachelor's degree (professional)", 42812.0: " LV: Bachelor's degree (academic)", 42813.0: " LV: Master's degree (professional)", 42814.0: " LV: Master's degree (academic)", 42815.0: ' LV: Higher education obtained during the Soviet era', 42816.0: ' LV: Doctoral degree', 44000.0: ' LT: Less than primary', 44001.0: ' LT: Primary', 44002.0: ' LT: Lower general vocational', 44003.0: ' LT: General/ lower secondary', 44004.0: ' LT: General vocational', 44005.0: ' LT: Lower vocational after general', 44006.0: ' LT: Vocational after general', 44007.0: ' LT: Secondary', 44008.0: ' LT: Vocational with secondary', 44009.0: ' LT: Vocational after secondary', 44010.0: ' LT: Upper general and secondary vocational', 44011.0: ' LT: Upper secondary vocational', 44012.0: ' LT: Higher non university', 44013.0: ' LT: Higher university education (BA)', 44014.0: ' LT: Higher university education (MA)', 44015.0: ' LT: Higher university integrated education (I and II stages - 6 years)', 44016.0: ' LT: Doctoral studies and diploma', 49901.0: ' ME: No education', 49902.0: ' ME: Completed 4 years of primary education', 49903.0: ' ME: Completed lower secondary education', 49904.0: ' ME: 1-2 years vocational training', 49905.0: ' ME: Completed high school lasting three years', 49906.0: ' ME: Certificate of upper secondary vocational education (4 years)', 49907.0: ' ME: Secondary general (grammar schools, 4 years)', 49908.0: ' ME: Vocational exam', 49909.0: ' ME: Primary level diploma (after two or three years of bachelor level pre-Bologne reform)', 49910.0: ' ME: Completed two years of higher education that provides basic certificate but not entrance to MA studies', 49911.0: ' ME: Vocational studies (in duration of three years)', 49912.0: ' ME: Bachelor studies in duration of 3 years', 49913.0: ' ME: Specialization studies or four year studies in pre-Bologne system', 49914.0: ' ME: Master studies in duration of one year; Integrated studies (five year studies); Specialization studies', 49915.0: ' ME: Magister studies, Studies of medicine (in duration of 6 years, in old system), Specialisation', 49916.0: ' ME: PhD', 52801.0: 'NL: No completed primary education', 52802.0: 'NL: Primary education', 52803.0: "NL: Pre-vocational program ('vmbo level 1, 2 or 3' or 'lbo') or comparable", 52804.0: "NL: Preparatory secondary vocational education ('mavo' (previous 'vmbo') or 'vmbo level 4') or comparable", 52805.0: 'NL: Intermediate vocational educational, level 1 (duration 2 year)', 52806.0: "NL: Senior general secondary school ('havo') or comparable", 52807.0: "NL: Pre-university education ('vwo' or gymnasium) or comparable", 52808.0: "NL: kmbo', 'meao', 'mts' or comparable (duration 2-3 years)", 52809.0: 'NL: Intermediate vocational education , levels 2 and 3 (duration 2-3 years)', 52810.0: 'NL: Intermediate vocational education , level 4 (duration 4 years)', 52811.0: "NL: Intermediate vocational education plus (for people that completed 'havo')", 52812.0: 'NL: First year of university, Bachelor (propaedeutics)', 52813.0: 'NL: Shorter higher vocational education, shorter University of Applied Sciences (duration 2 or 3 years)', 52814.0: "NL: Higher vocational education / University of Applied Sciences, Bachelor's degree or comparable", 52815.0: "NL: University, Bachelor's degree", 52816.0: "NL: Higher vocational education / University of Applied Sciences, Master's degree or comparable", 52817.0: "NL: University, Master's degree or comparable", 52818.0: 'NL: University, doctoral degree (promotion)', 57801.0: ' NO: Never attended an education programme', 57802.0: ' NO: Some early childhood education', 57803.0: ' NO: Some primary education (without level completion)', 57810.0: ' NO: Primary education', 57824.0: ' NO: Lower secondary education - general', 57825.0: ' NO: Lower secondary education - vocational', 57834.0: ' NO: Upper secondary education - general', 57835.0: ' NO: Upper secondary education - vocational', 57844.0: ' NO: Post-secondary non-tertiary education - general', 57845.0: ' NO: Post-secondary non-tertiary education - vocational', 57854.0: ' NO: Short-cycle tertiary education - general', 57855.0: ' NO: Short-cycle tertiary education - vocational', 57856.0: ' NO: Short-cycle tertiary education - orientation unspecified', 57864.0: ' NO: Bachelor’s or equivalent level - academic', 57865.0: ' NO: Bachelor’s or equivalent level - professional', 57866.0: ' NO: Bachelor’s or equivalent level - orientation unspecified', 57874.0: ' NO: Master’s or equivalent level - academic', 57875.0: ' NO: Master’s or equivalent level - professional', 57876.0: ' NO: Master’s or equivalent level - orientation unspecified', 57884.0: ' NO: Doctoral or equivalent level - academic', 57885.0: ' NO: Doctoral or equivalent level - professional', 57886.0: ' NO: Doctoral or equivalent level - orientation unspecified', 61601.0: 'PL: primary education not completed', 61602.0: 'PL: primary education (6 grades or 4 grades)', 61603.0: 'PL: primary education', 61604.0: 'PL: lower secondary', 61605.0: 'PL: basic vocational education in agriculture', 61606.0: 'PL: basic vocational education', 61607.0: 'PL: basic vocational education', 61608.0: 'PL: upper secondary education without A-level diploma', 61609.0: 'PL: upper secondary education with A-level diploma', 61610.0: 'PL: upper secondary vocational or technical education', 61611.0: 'PL: upper secondary vocational or technical education with A-level diploma', 61612.0: 'PL: diploma of technician or post secondary education', 61613.0: "PL: post secondary education in teachers' college", 61614.0: "PL: BA or engineer's diploma", 61615.0: "PL: MA or doctor's diploma", 61616.0: "PL: PhD, assistant professor, professor's title", 61617.0: 'PL: other', 62001.0: ' PT: None', 62002.0: ' PT: Basic Level 1 (until 4th grade, primary education)', 62003.0: ' PT: Basic level 2 (until 6th grade, 1st cycle of former comercial or industrial high school)', 62004.0: ' PT: Type 1 education and formation courses. Attribution of level 1 professional qualification diploma', 62005.0: ' PT: Basic Level 3 (Certificate of conclusion of one of the following grades: 9th grade; former 5th grade of high school', 62006.0: ' PT: Type 2 education and formation courses. Attribution of level 2 professional qualification diploma', 62007.0: ' PT: Types 3 and 4 education and formation courses. Attribution of level 2 professional qualification diploma', 62008.0: ' PT: Secondary Level - Scientific-Humanistic studies (certificate of conclusion of one of the following grades: 12th gra', 62009.0: ' PT: Secondary Level -technologic and artistic studies (visual and audiovisual arts, dance and music), professional cour', 62010.0: ' PT: Technologic specialisation course. Attribution of Thecnologic specialisation diploma', 62011.0: ' PT: Polytechnic superior level:3 years bacalaureat (primary school teachers, social service, agricultural manager); f', 62012.0: ' PT: Polytechnic superior level:3-4 years graduation', 62013.0: ' PT: University superior level: 3-4 years graduation: 4 years two-stage graduation', 62014.0: ' PT: Post-graduation: post-graduation specialisation without degree atribution, MBA', 62015.0: ' PT: University superior level: gradution with more than 4 years: 5 years two-stage graduation', 62016.0: ' PT: Master degree (inc. integrated master)', 62017.0: ' PT: PhD', 64200.0: ' RO: No school at all', 64201.0: ' RO: Unfinished primary school', 64202.0: ' RO: Finished primary school', 64203.0: ' RO: Unfinished gymnasia', 64204.0: ' RO: Graduated gymnasia', 64205.0: ' RO: Apprentice (complementary) school', 64206.0: ' RO: School of Arts and Crafts', 64207.0: ' RO: School of Arts and Crafts - year of completion', 64208.0: ' RO: Professional school, less than 2 years', 64209.0: ' RO: Professional school, 2-4 years', 64210.0: ' RO: Unfinished High School', 64211.0: ' RO: Completed Theoretical High School (Baccalaureate Diploma)', 64212.0: ' RO: Completed Technological / Industrial High School (Baccalaureate Diploma)', 64213.0: ' RO: Foreman school without Baccalaureate', 64214.0: ' RO: Foreman school with Baccalaureate', 64215.0: ' RO: Post-highschool without Baccalaureate', 64216.0: ' RO: Post-highschool with Baccalaureate', 64217.0: ' RO: Unfinished university studies', 64218.0: ' RO: Sub-engineers or College', 64219.0: ' RO: BA (graduated university): 3 years', 64220.0: ' RO: BA (graduated university): 4 years', 64221.0: ' RO: BA (graduated university): 5 years', 64222.0: ' RO: BA (graduated university): 6 years', 64223.0: ' RO: Master', 64224.0: ' RO: PhD', 64301.0: 'RU: Did not study in school or completed 1-2 grades on school only (incomplete primary school)', 64302.0: 'RU: Graduated 3-7 grades of secondary school without attestat on basic general education', 64303.0: 'RU: Attestat of basic general educ.–complete 7 grade (pre 1958); 8 gr.(1960-1980); 9 gr.(modern system); no profes. ed', 64304.0: 'RU: Completed general secondary educ. (10 grade by old system,11 grade by new), got attestat; no professional educ.', 64305.0: 'RU: Got primary professional educ.–grad. PTU,FSU,FSO, profes.-techn. lyceum; no attestat of compl. sec. gen. educ.(<2y', 64306.0: 'RU: Got primary professional educ.– grad. PTU, professional-techn. lyceum, with attestat of completed sec. educ.(1-3 y', 64307.0: 'RU: Got secondary professional education – graduated technikum, uchilishe, college (2-4 years of study)', 64308.0: 'RU: Got diploma of bachelor in college after 4 years of education on two-stage new education system', 64309.0: 'RU: Got diploma of master in college after additional 2 years of education on two-stage new education system', 64310.0: 'RU: Completed high education by 5-6-years system (diploma of specialist)', 64311.0: 'RU: Scientific degree (candidate, doctor of sciences)', 68801.0: ' RS: No education (under 3rd grade)', 68802.0: ' RS: Primary education lower cycle (4th – 7th grade)', 68803.0: ' RS: Primary education upper cycle (8th grade)', 68804.0: ' RS: Secondary vocational 3 yrs', 68805.0: ' RS: Secondary vocational 4 yrs', 68806.0: ' RS: Secondary general (grammar schools)', 68807.0: ' RS: 1-2 years vocational training', 68808.0: ' RS: Higher schools', 68809.0: ' RS: University: Graduate studies', 68810.0: ' RS: Postgraduate studies: Specialist studies', 68811.0: ' RS: Postgraduate studies: Magister studies (outdated)', 68812.0: ' RS: Postgraduate studies: Master studies', 68813.0: ' RS: PhD studies/ Doctor’s Degree', 70301.0: 'SK: Not completed first stage of elementary school (not completed primary level of education)', 70302.0: 'SK: Not completed second stage of elementary school (not completed lower secondary level of education)', 70303.0: 'SK: Completed lower secondary educ.(completed 8/9 y. of elementary school or courses to complete lower secondary educ.)', 70304.0: ' SK: Practical (girl) school, two-years training programmes, requalification courses without vocational certificate', 70305.0: 'SK: secondary vocational school or sec. voc. training institution or sec. voc. school without leaving exam (no maturita)', 70306.0: 'SK: Secondary vocational school with leaving exam (with maturita), secondary vocat. training institution (with maturita)', 70307.0: 'SK: Eight year grammar school - 4 year grammar school', 70308.0: 'SK: Complementary study', 70309.0: "SK: Complementary 'postgraduate' pedagogical study", 70310.0: "SK: 'postgraduate' vocational study", 70311.0: 'SK: Postgraduate specialised study with leaving certificate, Dance conservatory', 70312.0: 'SK: Secondary vocational schoool with leaving examination (maturita) - six year study', 70313.0: 'SK: Bachelors degree', 70314.0: "SK: Master degree (engineering study, 'small' doctorate)", 70315.0: 'SK: postgraduate pedagogical study, teaching certificate for university graduates', 70316.0: 'SK: PhD study, doctoral degree', 70501.0: 'SI: Without school education', 70502.0: 'SI: Incomplete primary education', 70503.0: 'SI: Primary education', 70504.0: 'SI: Lower or upper secondary vocational education', 70505.0: 'SI: Secondary vocational education', 70506.0: 'SI: Secondary general education', 70507.0: 'SI: Higher vocational education, post-secondary education (2 years)', 70508.0: 'SI: Higher vocational education - 1. Bologna level.', 70509.0: 'SI: Higher education university education', 70510.0: "SI: Bologna master's degree", 70511.0: 'SI: Specialization', 70512.0: "SI: Master's degree", 70513.0: 'SI: phD', 72401.0: 'ES: No studies', 72402.0: 'ES: Not completed primary studies', 72403.0: 'ES: Old Primary School (Before 1970)', 72404.0: 'ES: Up to the 5th course of EGB (Basic General Education). From 70s to 90s', 72405.0: 'ES: Up to the 6th course of Primary School', 72406.0: 'ES: Primary School in Music and Dance', 72407.0: 'ES: Elementary High School (Before 1970)', 72408.0: 'ES: Up to the 8th course of EGB (Basic General Education). From 70s to 90s', 72409.0: 'ES: Compulsory High School', 72410.0: 'ES: High School (From 70s to 90s)', 72411.0: 'ES: Pre-University Studies (before 90s)', 72412.0: 'ES: Not compulsory High School', 72413.0: 'ES: Vocational training (initiation)', 72414.0: 'ES: Vocational training (initiation) (old Law)', 72415.0: 'ES: Vocational training (first grade) (old Law)', 72416.0: 'ES: Vocational training (first grade)', 72417.0: 'ES: Vocational training (medium grade, technical in various professions such as administrative or mechanic )', 72418.0: 'ES: Vocational training (medium grade, arts and design)', 72419.0: 'ES: Vocational training (medium grade, music and dance)', 72420.0: 'ES: Vocational training (high grade, industrial) (old Law)', 72421.0: 'ES: Vocational training (high grade, general) (old Law)', 72422.0: 'ES: Vocational training (high grade, general)', 72423.0: 'ES: Vocational training (high grade in arts)', 72424.0: 'ES: Medium Tertiary studies (nursery, school teacher, social worker)', 72425.0: 'ES: Medium Tertiary studies ( building engineer, industrial or business expert)', 72426.0: 'ES: Long tertiary studies', 72427.0: 'ES: Phd', 72428.0: 'ES: Other (specify)', 75201.0: ' SE: not completed ISCED level 1', 75202.0: ' SE: ISCED 1, completed primary education', 75203.0: ' SE: Qualification from general ISCED 2A programmes, access to ISCED 3A general or all 3', 75204.0: ' SE: Old version of vocational high school', 75205.0: ' SE: Old and current version of high school education that gives access to higher education', 75206.0: ' SE: Old version of mainly vocational high school with limited access to higher education', 75207.0: ' SE: Vocational high school', 75208.0: ' SE: Technical high school, formerly 4 years at high school, currently 3 years at high school and 4th year at university', 75209.0: ' SE: Degree from one year of studies at university college.', 75210.0: ' SE: Vocational degree, qualification obtained after high school graduation, from vocational training institutes', 75211.0: ' SE: Vocational degree, obtained at university or university college, e.g. old professional nursing programmes', 75212.0: ' SE: Vocational degree, obtained after high school graduation, longer programme 2-3 years from vocational training insti', 75213.0: " SE: Bachelor's degree or professional degree from a university college", 75214.0: " SE: Bachelor's degree or professional degree from a university", 75215.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75216.0: ' SE: Masters degree from university college', 75217.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75218.0: ' SE: Masters degree from university', 75219.0: ' SE: Research degree, licantiate degree, a sort of half-way doctoral degree, old degree that is not often used', 75220.0: ' SE: Research degree, phd', 75601.0: 'CH: Incompleted primary school', 75602.0: 'CH: Primary school', 75603.0: 'CH: Secondary education (first stage)', 75604.0: 'CH: Additional year of secondary education, preparation for vocational training', 75605.0: 'CH: General training school (2-3 years)', 75606.0: 'CH: Baccalaureate preparing for university', 75607.0: 'CH: Baccalaureate for adults or apprenticeship after Baccalaureate', 75608.0: 'CH: Diploma for teaching in primary school or preprimary school', 75609.0: 'CH: Vocational baccalaureate', 75610.0: 'CH: Vocational baccalaureate for adults', 75611.0: 'CH: Elementary vocational training (enterprise and school, 1-2 year)', 75612.0: 'CH: Apprenticeship (vocational training, dual system, 3-4 years)', 75613.0: 'CH: Second vocational training (or apprenticeship as second education)', 75614.0: 'CH: Advanced vocational qualification (specialization exam, federal certif. or dipl. of advanced vocational training)', 75615.0: 'CH: Higher vocational training (high school diploma - technical, administration, health, social work, applied arts)', 75616.0: 'CH:Higher vocational training (diploma of some specific high schools withrecognition of tertiary level)', 75617.0: 'CH: University of applied science and pedagogical university (Bachelor)', 75618.0: 'CH: University of applied science and pedagogical university (Master)', 75619.0: 'CH: University diploma (intermediary level)', 75620.0: 'CH: University diploma and post-graduate (including technical) (Bachelor and short university degree)', 75621.0: 'CH: University diploma and post-graduate (including technical) (degree requiring more than 4 years)', 75622.0: 'CH: University diploma and post-graduate (including technical) (Master)', 75623.0: 'CH: Doctoral degree', 75624.0: 'CH: other', 80400.0: ' UA: Incomplete primary secondary education (less than 4 classes)', 80401.0: ' UA: Primary secondary education (1- 3(4) classes)', 80402.0: ' UA: Basic secondary education (certificate for 8(9) class)', 80403.0: ' UA: Technical-vocational education based on basic secondary education (no certificate of complete secondary education)', 80404.0: ' UA: Technical-vocational education based on of basic general secondary (certificate of general secondary education and', 80405.0: ' UA: Complete secondary education (certificate of general secondary education of 10(11) classes)', 80406.0: ' UA: Additional training training on the basis of general secondary education (vocational and general educational course', 80407.0: ' UA: Technical-vocational education on the basis of general secondary education', 80408.0: ' UA: Professional pre-higher education (diploma of junior specialist)', 80409.0: " UA: Basic higher education (bachelor's degree)", 80410.0: ' UA: Full higher education (specialist)', 80411.0: ' UA: Full higher education (Master or equivalent)', 80412.0: ' UA: Academic degree (candidate, doctor, PhD)', 80701.0: 'MK: Without education', 80702.0: 'MK: Completed primary education (4 years of primary school)', 80703.0: 'MK: Completed primary and lower secondary education', 80704.0: 'MK: Completed upper secondary education, vocational training - 2 years', 80705.0: 'MK: Completed upper secondary education, vocational training - 3 years', 80706.0: 'MK: Completed upper secondary education, vocational training - 4 years', 80707.0: 'MK: Completed upper secondary education, general (high school) - 4 years', 80708.0: 'MK: Completed further education - short cycle university education - 2 years', 80709.0: 'MK: Bachelor degree - 3 years', 80710.0: 'MK: Bachelor degree - 4 years', 80711.0: 'MK: Completed postgraduate studies, specialist studies - 1 year', 80712.0: 'MK: Completed postgraduate studies, specialist studies - 2 years', 80713.0: 'MK: Completed postgraduate studies, master studies - 1 year', 80714.0: 'MK: Completed postgraduate studies, master studies - 2 years', 80715.0: 'MK: Doctoral degree, completed doctorate - 5 years', 80716.0: 'MK: Doctoral degree, completed doctorate (PhD studies) - 3 years', 80788.0: 'MK: don’t know (spontaneous)', 80799.0: 'MK: no answer (spontaneous)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55120 | 535 |
v243_cs_DE1 | 348 | v243_cs_DE1 | numeric | DE COUNTRY-SPECIFIC: educational level respondent (Q81), SCHOOL | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', 27601.0: 'Primary school not completed', 27602.0: 'Primary school completed, secondary school not (yet) completed', 27603.0: 'Lower secondary schools (no access to general) or polytechnic secondary school (8th or 9th grade)', 27604.0: 'Lower secondary schools (access to general) or polytechnic secondary school (10th grade)', 27605.0: 'University of Applied Science entrance qualification (specialised vocational high schools etc.)', 27606.0: 'Grammar school (University entrance qualification)', 27607.0: 'Other, please specify: (write in)'} | {-4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 2133 | 7 |
v243_cs_DE2 | 349 | v243_cs_DE2 | numeric | DE COUNTRY-SPECIFIC: educational level respondent (Q81), STUDIES | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', 27601.0: 'No degree', 27602.0: 'Preliminary examination, preliminary diploma', 27603.0: 'College of public administration or University of Applied Sciences (diploma)', 27604.0: 'Vocational academy (diploma)', 27605.0: 'College of public administration or University of Applied Sciences (Bachelor)', 27606.0: 'Vocational academy (Bachelor)', 27607.0: 'University (Bachelor)', 27608.0: 'College of public administration or University of Applied Sciences (Master)', 27609.0: 'Vocational academy (Master)', 27610.0: 'University (diploma, Magister, state examaniation)', 27611.0: 'University (Master or postgraduate studies)', 27612.0: 'Doctoral studies', 27613.0: 'other, please specify: (write in)'} | {-4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 2016 | 13 |
v243_cs_DE3 | 350 | v243_cs_DE3 | numeric | DE COUNTRY-SPECIFIC: educational level respondent (Q81), VOCATIONAL | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', 27601.0: 'No degree', 27602.0: 'Pre-vocational training, qualification of short health sector programmes', 27603.0: 'Vocational training with diploma, but no Dual System (second cycle)', 27604.0: 'Medical assistant, nurse (qualification of health sector schools (2 and 3 years)', 27605.0: 'Qualification of civil servants for the medium level', 27606.0: 'Qualification in the Dual System (second cycle), industrial or agricultural sector', 27607.0: 'Qualification in the Dual System (second cycle), sales sector', 27608.0: 'Qualification of a vocational full-time school', 27609.0: 'Occupational qualification (second cycle) for students with occupational qualification or with university entrace qualif', 27610.0: "Master craftsmen's or Technician's qualification (or equal)", 27611.0: 'other, please specify: (write in)'} | {-4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 2048 | 11 |
v243_cs_GB1 | 351 | v243_cs_GB1 | numeric | GB COUNTRY-SPECIFIC: educational level respondent: Up to 2 or more A-levels or equivalent (Q81a) | {82601.0: '2 or more A-levels or equivalent', 82602.0: ' GNVQ or GSVQ Intermediate', 82603.0: ' Vocational GCSE or equivalent', 82604.0: ' 5 or more GCSEs A*-C or equivalent', 82605.0: ' 1-4 GCSEs A*-C or equivalent', 82606.0: ' Skills for life', 82607.0: ' None of these'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1776 | 7 |
v243_cs_GB2 | 352 | v243_cs_GB2 | numeric | GB COUNTRY-SPECIFIC: educational level respondent: Up to Ph.D or equivalent (Q81b) | {82601.0: ' Ph.D, D.Phil or equivalent', 82602.0: ' Masters Degree, M.Phil, Post-Graduate Diplomas and Certificates', 82603.0: ' 5 year University/CNAA first Degree (MB, BDS, BV etc)', 82604.0: ' 3-4 year University/CNAA first Degree (BA, BSc., BEd., BEng. Etc)', 82605.0: ' Nursing certificate, Teacher training, HE Diploma, Edexcel/BTEC/BEC/TEC/HND, City and Guilds, NVQ/SVQ - Leve', 82606.0: ' Foundation Degree (FdA, FdSc etc)', 82607.0: ' Edexcel/BTEC/BEC/TEC - Higher National Certificate (HNC) or equivalent', 82608.0: ' HE Access', 82609.0: ' Vocational A-level (AVCE), GCE Applied A-level, NVQ/SVQ Level 3, GNVQ/GSVQ Advanced, Edexcel/BTEC/BEC/TEC (G', 82610.0: ' (Modern) Apprenticeship, Advanced (Modern) Apprenticeship, SVQ/NVQ/Key Skills Level 1 and 2, City and Guild', 82611.0: ' None of these', 82612.0: ' Any other qualifications (specify)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1775 | 12 |
v244 | 353 | v244 | numeric | paid employment/no paid employment (Q82) | {1.0: '30h a week or more', 2.0: 'less then 30h a week', 3.0: 'self employed', 4.0: 'military service', 5.0: 'retired/pensioned', 6.0: 'homemaker not otherwise employed', 7.0: 'student', 8.0: 'unemployed', 9.0: 'disabled', 10.0: 'other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59027 | 10 |
v245 | 354 | v245 | numeric | employment/self-employment: last job (Q83) | {1.0: 'employed', 2.0: 'self-employed'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'never had a paid job'} | True | 21903 | 2 |
v246_ISCO_2 | 355 | v246_ISCO_2 | numeric | kind of job respondent - 2 digit ISCO08 code (Q84a) | {0.0: 'Armed Forces Occupations', 1.0: 'Commissioned Armed Forces Officers', 2.0: 'Non-commissioned Armed Forces Officers', 3.0: 'Armed Forces Occupations, Other Ranks', 10.0: 'ISCO 1-digit: Managers', 11.0: 'Chief Executives, Senior Officials and Legislators', 12.0: 'Administrative and Commercial Managers', 13.0: 'Production and Specialized Services Managers', 14.0: 'Hospitality, Retail and Other Services Managers', 20.0: 'ISCO 1-digit: Professionals', 21.0: 'Science and Engineering Professionals', 22.0: 'Health Professionals', 23.0: 'Teaching Professionals', 24.0: 'Business and Administration Professionals', 25.0: 'Information and Communications Technology Professionals', 26.0: 'Legal, Social and Cultural Professionals', 30.0: 'ISCO 1-digit: Technicians and Associate Professionals', 31.0: 'Science and Engineering Associate Professionals', 32.0: 'Health Associate Professionals', 33.0: 'Business and Administration Associate Professionals', 34.0: 'Legal, Social, Cultural and Related Associate Professionals', 35.0: 'Information and Communications Technicians', 40.0: 'ISCO 1-digit: Clerical Support Workers', 41.0: 'General and Keyboard Clerks', 42.0: 'Customer Services Clerks', 43.0: 'Numerical and Material Recording Clerks', 44.0: 'Other Clerical Support Workers', 50.0: 'ISCO 1-digit: Services and Sales Workers', 51.0: 'Personal Services Workers', 52.0: 'Sales Workers', 53.0: 'Personal Care Workers', 54.0: 'Protective Services Workers', 60.0: 'ISCO 1-digit: Skilled Agricultural, Forestry and Fishery Workers', 61.0: 'Market-oriented Skilled Agricultural Workers', 62.0: 'Market-oriented Skilled Forestry, Fishery and Hunting Workers', 63.0: 'Subsistence Farmers, Fishers, Hunters and Gatherers', 70.0: 'ISCO 1-digit: Craft and Related Trades Workers', 71.0: 'Building and Related Trades Workers (excluding Electricians)', 72.0: 'Metal, Machinery and Related Trades Workers', 73.0: 'Handicraft and Printing Workers', 74.0: 'Electrical and Electronics Trades Workers', 75.0: 'Food Processing, Woodworking, Garment and Other Craft and Related Trades Workers', 80.0: 'ISCO 1-digit: Plant and Machine Operators and Assemblers', 81.0: 'Stationary Plant and Machine Operators', 82.0: 'Assemblers', 83.0: 'Drivers and Mobile Plant Operators', 90.0: 'ISCO 1-digit: Elementary Occupations', 91.0: 'Cleaners and Helpers', 92.0: 'Agricultural, Forestry and Fishery Labourers', 93.0: 'Labourers in Mining, Construction, Manufacturing and Transport', 94.0: 'Food Preparation Assistants', 95.0: 'Street and Related Sales and Services Workers', 96.0: 'Refuse Workers and Other Elementary Workers', 99.0: 'ISCO could not be applied to response given'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51510 | 51 |
v246_SIOPS | 356 | v246_SIOPS | numeric | kind of job respondent - SIOPS08 code (Q84a) | {} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51280 | 63 |
v246_ISEI | 357 | v246_ISEI | numeric | kind of job respondent - ISEI08 code (Q84a) | {} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51280 | 77 |
v246_ESeC | 358 | v246_ESeC | nominal | kind of job respondent - ESEC08 code (Q84a) | {1.0: 'Large employers, higher mgrs/professionals', 2.0: 'Lower mgrs/professionals, higher supervisory/technicians', 3.0: 'Intermediate occupations', 4.0: 'Small employers and self-employed (non-agriculture)', 5.0: 'Small employers and self-employed (agriculture)', 6.0: 'Lower supervisors and technicians', 7.0: 'Lower sales and service', 8.0: 'Lower technical', 9.0: 'Routine', 99.0: 'ISCO could not be applied to response given'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey beak-off)', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51280 | 9 |
v246_egp | 359 | v246_egp | numeric | kind of job respondent - EGP11 (Q84a) | {1.0: 'I :Higher Controllers', 2.0: 'II :Lower Controllers', 3.0: 'IIIa:Routine Nonmanual', 4.0: 'IIIb:Lower Sales-Service', 5.0: 'IVa:Selfempl with empl', 6.0: 'IVb:Selfempl no empl', 7.0: 'V :Manual Supervisors', 8.0: 'VI :Skilled Worker', 9.0: 'VIIa:Unskilled Worker', 10.0: 'VIIb:Farm Labor', 11.0: 'IVc:Selfempl Farmer', 99999.0: 'ISCO could not be applied to response given'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 51257 | 11 |
v247 | 360 | v247 | numeric | respondent has/had how many employees (Q85) | {1.0: 'none', 2.0: '1-9', 3.0: '10-24', 4.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 5467 | 4 |
v248 | 361 | v248 | numeric | do/did you supervise someone (Q86) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 46098 | 2 |
v248a | 362 | v248a | numeric | how many people do/did you supervise (Q86a) | {1.0: '1-9', 2.0: '10-24', 3.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 12205 | 3 |
v249 | 363 | v249 | numeric | employment sector you work/-ed for (Q87) | {1.0: 'government or public institution', 2.0: 'private business or industry', 3.0: 'private non-profit organization'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 43442 | 3 |
v250 | 364 | v250 | numeric | partner/spouse born in [country] (Q88) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35914 | 2 |
v251b | 365 | v251b | nominal | spouse/partners country of birth (ISO 3166-1/3 Alpha code) (Q88a) | {'005': 'M49 code: South America', '011': 'M49 code: Western Africa', '013': 'M49 code: Central America', '014': 'M49 code: Eastern Africa', '015': 'M49 code: Northern Africa', '017': 'M49 code: Middle Africa', '018': 'M49 code: Southern Africa', '029': 'M49 code: Caribbean', '030': 'M49 code: Eastern Asia', '034': 'M49 code: Southern Asia', '035': 'M49 code: South-Eastern Asia', '039': 'M49 code: Southern Europe', '053': 'M49 code: Australia and New Zealand', '054': 'M49 code: Melanesia', '061': 'M49 code: Polynesia', '143': 'M49 code: Central Asia', '145': 'M49 code: Western Asia', '154': 'M49 code: Northern Europe', 'AD': 'Andorra', 'AE': 'United Arab Emirates', 'AF': 'Afghanistan', 'AG': 'Antigua and Barbuda', 'AI': 'Anguilla', 'AL': 'Albania', 'AM': 'Armenia', 'AO': 'Angola', 'AQ': 'Antarctica', 'AR': 'Argentina', 'AS': 'American Samoa', 'AT': 'Austria', 'AU': 'Australia', 'AW': 'Aruba', 'AX': 'Åland Islands', 'AZ': 'Azerbaijan', 'BA': 'Bosnia and Herzegovina', 'BB': 'Barbados', 'BD': 'Bangladesh', 'BE': 'Belgium', 'BF': 'Burkina Faso', 'BG': 'Bulgaria', 'BH': 'Bahrain', 'BI': 'Burundi', 'BJ': 'Benin', 'BL': 'Saint Barthélemy', 'BM': 'Bermuda', 'BN': 'Brunei Darussalam', 'BO': 'Bolivia (Plurinational State of)', 'BQ': 'Bonaire, Sint Eustatius and Saba', 'BR': 'Brazil', 'BS': 'Bahamas', 'BT': 'Bhutan', 'BV': 'Bouvet Island', 'BW': 'Botswana', 'BY': 'Belarus', 'BZ': 'Belize', 'CA': 'Canada', 'CC': 'Cocos (Keeling) Islands', 'CD': 'Congo (Democratic Republic of the)', 'CF': 'Central African Republic', 'CG': 'Congo', 'CH': 'Switzerland', 'CI': 'Côte d´Ivoire', 'CK': 'Cook Islands', 'CL': 'Chile', 'CM': 'Cameroon', 'CN': 'China', 'CO': 'Colombia', 'CR': 'Costa Rica', 'CSHH': 'Czechoslovakia (former country)', 'CSXX': 'Serbia and Montenegro (former country)', 'CU': 'Cuba', 'CV': 'Cabo Verde', 'CW': 'Curaçao', 'CX': 'Christmas Island', 'CY': 'Cyprus', 'CY-TCC': 'Northern Cyprus', 'CZ': 'Czechia', 'DDDE': 'German Democratic Republic (former country)', 'DE': 'Germany', 'DJ': 'Djibouti', 'DK': 'Denmark', 'DM': 'Dominica', 'DO': 'Dominican Republic', 'DZ': 'Algeria', 'EC': 'Ecuador', 'EE': 'Estonia', 'EG': 'Egypt', 'EH': 'Western Sahara', 'ER': 'Eritrea', 'ES': 'Spain', 'ET': 'Ethiopia', 'FI': 'Finland', 'FJ': 'Fiji', 'FK': 'Falkland Islands (Malvinas)', 'FM': 'Micronesia (Federated States of)', 'FO': 'Faroe Islands', 'FR': 'France', 'GA': 'Gabon', 'GB': 'United Kingdom of Great Britain and Northern Ireland', 'GD': 'Grenada', 'GE': 'Georgia', 'GF': 'French Guiana', 'GG': 'Guernsey', 'GH': 'Ghana', 'GI': 'Gibraltar', 'GL': 'Greenland', 'GM': 'Gambia', 'GN': 'Guinea', 'GP': 'Guadeloupe', 'GQ': 'Equatorial Guinea', 'GR': 'Greece', 'GS': 'South Georgia and the South Sandwich Islands', 'GT': 'Guatemala', 'GU': 'Guam', 'GW': 'Guinea-Bissau', 'GY': 'Guyana', 'HK': 'Hong Kong', 'HM': 'Heard Island and McDonald Islands', 'HN': 'Honduras', 'HR': 'Croatia', 'HT': 'Haiti', 'HU': 'Hungary', 'ID': 'Indonesia', 'IE': 'Ireland', 'IL': 'Israel', 'IM': 'Isle of Man', 'IN': 'India', 'IO': 'British Indian Ocean Territory', 'IQ': 'Iraq', 'IR': 'Iran (Islamic Republic of)', 'IS': 'Iceland', 'IT': 'Italy', 'JE': 'Jersey', 'JM': 'Jamaica', 'JO': 'Jordan', 'JP': 'Japan', 'KE': 'Kenya', 'KG': 'Kyrgyzstan', 'KH': 'Cambodia', 'KI': 'Kiribati', 'KM': 'Comoros', 'KN': 'Saint Kitts and Nevis', 'KP': 'Korea (Democratic People´s Republic of)', 'KR': 'Korea (Republic of)', 'KW': 'Kuwait', 'KY': 'Cayman Islands', 'KZ': 'Kazakhstan', 'LA': 'Lao People´s Democratic Republic', 'LB': 'Lebanon', 'LC': 'Saint Lucia', 'LI': 'Liechtenstein', 'LK': 'Sri Lanka', 'LR': 'Liberia', 'LS': 'Lesotho', 'LT': 'Lithuania', 'LU': 'Luxembourg', 'LV': 'Latvia', 'LY': 'Libya', 'MA': 'Morocco', 'MC': 'Monaco', 'MD': 'Moldova (Republic of)', 'ME': 'Montenegro', 'MF': 'Saint Martin (French part)', 'MG': 'Madagascar', 'MH': 'Marshall Islands', 'MK': 'North Macedonia', 'ML': 'Mali', 'MM': 'Myanmar', 'MN': 'Mongolia', 'MO': 'Macao', 'MP': 'Northern Mariana Islands', 'MQ': 'Martinique', 'MR': 'Mauritania', 'MS': 'Montserrat', 'MT': 'Malta', 'MU': 'Mauritius', 'MV': 'Maldives', 'MW': 'Malawi', 'MX': 'Mexico', 'MY': 'Malaysia', 'MZ': 'Mozambique', 'NA': 'Namibia', 'NC': 'New Caledonia', 'NE': 'Niger', 'NF': 'Norfolk Island', 'NG': 'Nigeria', 'NI': 'Nicaragua', 'NL': 'Netherlands', 'NO': 'Norway', 'NP': 'Nepal', 'NR': 'Nauru', 'NU': 'Niue', 'NZ': 'New Zealand', 'OM': 'Oman', 'PA': 'Panama', 'PE': 'Peru', 'PF': 'French Polynesia', 'PG': 'Papua New Guinea', 'PH': 'Philippines', 'PK': 'Pakistan', 'PL': 'Poland', 'PM': 'Saint Pierre and Miquelon', 'PN': 'Pitcairn', 'PR': 'Puerto Rico', 'PS': 'Palestine, State of', 'PT': 'Portugal', 'PW': 'Palau', 'PY': 'Paraguay', 'QA': 'Qatar', 'RE': 'Réunion', 'RO': 'Romania', 'RS': 'Serbia', 'RU': 'Russian Federation', 'RW': 'Rwanda', 'SA': 'Saudi Arabia', 'SB': 'Solomon Islands', 'SC': 'Seychelles', 'SD': 'Sudan', 'SE': 'Sweden', 'SG': 'Singapore', 'SH': 'Saint Helena, Ascension and Tristan da Cunha', 'SI': 'Slovenia', 'SJ': 'Svalbard and Jan Mayen', 'SK': 'Slovakia', 'SL': 'Sierra Leone', 'SM': 'San Marino', 'SN': 'Senegal', 'SO': 'Somalia', 'SR': 'Suriname', 'SS': 'South Sudan', 'ST': 'Sao Tome and Principe', 'SUHH': 'U.S.S.R. (former country)', 'SV': 'El Salvador', 'SX': 'Sint Maarten (Dutch part)', 'SY': 'Syrian Arab Republic', 'SZ': 'Swaziland', 'TC': 'Turks and Caicos Islands', 'TD': 'Chad', 'TF': 'French Southern Territories', 'TG': 'Togo', 'TH': 'Thailand', 'TJ': 'Tajikistan', 'TK': 'Tokelau', 'TL': 'Timor-Leste', 'TM': 'Turkmenistan', 'TN': 'Tunisia', 'TO': 'Tonga', 'TR': 'Turkey', 'TT': 'Trinidad and Tobago', 'TV': 'Tuvalu', 'TW': 'Taiwan, Province of China[a]', 'TZ': 'Tanzania, United Republic of', 'UA': 'Ukraine', 'UG': 'Uganda', 'UM': 'United States Minor Outlying Islands', 'US': 'United States of America', 'UY': 'Uruguay', 'UZ': 'Uzbekistan', 'VA': 'Holy See', 'VC': 'Saint Vincent and the Grenadines', 'VE': 'Venezuela (Bolivarian Republic of)', 'VG': 'Virgin Islands (British)', 'VI': 'Virgin Islands (U.S.)', 'VN': 'Viet Nam', 'VU': 'Vanuatu', 'WF': 'Wallis and Futuna', 'WS': 'Samoa', 'XK': 'Kosovo', 'XXX': 'Other', 'YE': 'Yemen', 'YT': 'Mayotte', 'YUCS': 'Yugoslavia (former country)', 'ZA': 'South Africa', 'ZM': 'Zambia', 'ZW': 'Zimbabwe'} | {'-1': "don't know/no answer", '-3': 'not applicable', '-5': 'other missing'} | True | 2708 | 97 |
v251b_r | 366 | v251b_r | nominal | spouse/partners country of birth (ISO 3166-1 numeric code) (Q88a) | {4.0: ' Afghanistan', 5.0: 'M49 code: South America', 8.0: ' Albania', 10.0: ' Antarctica', 11.0: 'M49 code: Western Africa', 12.0: ' Algeria', 13.0: 'M49 code: Central America', 14.0: 'M49 code: Eastern Africa', 15.0: 'M49 code: Northern Africa', 16.0: ' American Samoa', 17.0: 'M49 code: Middle Africa', 18.0: 'M49 code: Southern Africa', 20.0: ' Andorra', 24.0: ' Angola', 28.0: ' Antigua and Barbuda', 29.0: 'M49 code: Caribbean', 30.0: 'M49 code: Eastern Asia', 31.0: ' Azerbaijan', 32.0: ' Argentina', 34.0: 'M49 code: Southern Asia', 35.0: 'M49 code: South-Eastern Asia', 36.0: ' Australia', 39.0: 'M49 code: Southern Europe', 40.0: ' Austria', 44.0: ' Bahamas', 48.0: ' Bahrain', 50.0: ' Bangladesh', 51.0: ' Armenia', 52.0: ' Barbados', 53.0: 'M49 code: Australia and New Zealand', 54.0: 'M49 code: Melanesia', 56.0: ' Belgium', 60.0: ' Bermuda', 61.0: 'M49 code: Polynesia', 64.0: ' Bhutan', 68.0: ' Bolivia, Plurinational State of', 70.0: ' Bosnia and Herzegovina', 72.0: ' Botswana', 74.0: ' Bouvet Island', 76.0: ' Brazil', 84.0: ' Belize', 86.0: ' British Indian Ocean Territory', 90.0: ' Solomon Islands', 92.0: ' Virgin Islands, British', 96.0: ' Brunei Darussalam', 100.0: ' Bulgaria', 104.0: ' Myanmar', 108.0: ' Burundi', 112.0: ' Belarus', 116.0: ' Cambodia', 120.0: ' Cameroon', 124.0: ' Canada', 132.0: ' Cabo Verde', 136.0: ' Cayman Islands', 140.0: ' Central African Republic', 143.0: 'M49 code: Central Asia', 144.0: ' Sri Lanka', 145.0: 'M49 code: Western Asia', 148.0: ' Chad', 152.0: ' Chile', 154.0: 'M49 code: Northern Europe', 156.0: ' China', 158.0: ' Taiwan, Province of China', 162.0: ' Christmas Island', 166.0: ' Cocos (Keeling) Islands', 170.0: ' Colombia', 174.0: ' Comoros', 175.0: ' Mayotte', 178.0: ' Congo', 180.0: ' Congo, the Democratic Republic of the', 184.0: ' Cook Islands', 188.0: ' Costa Rica', 191.0: ' Croatia', 192.0: ' Cuba', 196.0: ' Cyprus', 200.0: ' Czechoslovakia (former country)', 203.0: ' Czechia', 204.0: ' Benin', 208.0: ' Denmark', 212.0: ' Dominica', 214.0: ' Dominican Republic', 218.0: ' Ecuador', 222.0: ' El Salvador', 226.0: ' Equatorial Guinea', 231.0: ' Ethiopia', 232.0: ' Eritrea', 233.0: ' Estonia', 234.0: ' Faroe Islands', 238.0: ' Falkland Islands (Malvinas)', 239.0: ' South Georgia and the South Sandwich Islands', 242.0: ' Fiji', 246.0: ' Finland', 248.0: ' Åland Islands', 250.0: ' France', 254.0: ' French Guiana', 258.0: ' French Polynesia', 260.0: ' French Southern Territories', 262.0: ' Djibouti', 266.0: ' Gabon', 268.0: ' Georgia', 270.0: ' Gambia', 275.0: ' Palestine, State of', 276.0: ' Germany', 278.0: 'German Democratic Republic (former country)', 288.0: ' Ghana', 292.0: ' Gibraltar', 296.0: ' Kiribati', 300.0: ' Greece', 304.0: ' Greenland', 308.0: ' Grenada', 312.0: ' Guadeloupe', 316.0: ' Guam', 320.0: ' Guatemala', 324.0: ' Guinea', 328.0: ' Guyana', 332.0: ' Haiti', 334.0: ' Heard Island and McDonald Islands', 336.0: ' Holy See', 340.0: ' Honduras', 344.0: ' Hong Kong', 348.0: ' Hungary', 352.0: ' Iceland', 356.0: ' India', 360.0: ' Indonesia', 364.0: ' Iran, Islamic Republic of', 368.0: ' Iraq', 372.0: ' Ireland', 376.0: ' Israel', 380.0: ' Italy', 384.0: " Côte d'Ivoire", 388.0: ' Jamaica', 392.0: ' Japan', 398.0: ' Kazakhstan', 400.0: ' Jordan', 404.0: ' Kenya', 408.0: " Korea, Democratic People's Republic of", 410.0: ' Korea, Republic of', 414.0: ' Kuwait', 417.0: ' Kyrgyzstan', 418.0: " Lao People's Democratic Republic", 422.0: ' Lebanon', 426.0: ' Lesotho', 428.0: ' Latvia', 430.0: ' Liberia', 434.0: ' Libya', 438.0: ' Liechtenstein', 440.0: ' Lithuania', 442.0: ' Luxembourg', 446.0: ' Macao', 450.0: ' Madagascar', 454.0: ' Malawi', 458.0: ' Malaysia', 462.0: ' Maldives', 466.0: ' Mali', 470.0: ' Malta', 474.0: ' Martinique', 478.0: ' Mauritania', 480.0: ' Mauritius', 484.0: ' Mexico', 492.0: ' Monaco', 496.0: ' Mongolia', 498.0: ' Moldova, Republic of', 499.0: ' Montenegro', 500.0: ' Montserrat', 504.0: ' Morocco', 508.0: ' Mozambique', 512.0: ' Oman', 516.0: ' Namibia', 520.0: ' Nauru', 524.0: ' Nepal', 528.0: ' Netherlands', 531.0: ' Curaçao', 533.0: ' Aruba', 534.0: ' Sint Maarten (Dutch part)', 535.0: ' Bonaire, Sint Eustatius and Saba', 540.0: ' New Caledonia', 548.0: ' Vanuatu', 554.0: ' New Zealand', 558.0: ' Nicaragua', 562.0: ' Niger', 566.0: ' Nigeria', 570.0: ' Niue', 574.0: ' Norfolk Island', 578.0: ' Norway', 580.0: ' Northern Mariana Islands', 581.0: ' United States Minor Outlying Islands', 583.0: ' Micronesia, Federated States of', 584.0: ' Marshall Islands', 585.0: ' Palau', 586.0: ' Pakistan', 591.0: ' Panama', 598.0: ' Papua New Guinea', 600.0: ' Paraguay', 604.0: ' Peru', 608.0: ' Philippines', 612.0: ' Pitcairn', 616.0: ' Poland', 620.0: ' Portugal', 624.0: ' Guinea-Bissau', 626.0: ' Timor-Leste', 630.0: ' Puerto Rico', 634.0: ' Qatar', 638.0: ' Réunion', 642.0: ' Romania', 643.0: ' Russian Federation', 646.0: ' Rwanda', 652.0: ' Saint Barthélemy', 654.0: ' Saint Helena, Ascension and Tristan da Cunha', 659.0: ' Saint Kitts and Nevis', 660.0: ' Anguilla', 662.0: ' Saint Lucia', 663.0: ' Saint Martin (French part)', 666.0: ' Saint Pierre and Miquelon', 670.0: ' Saint Vincent and the Grenadines', 674.0: ' San Marino', 678.0: ' Sao Tome and Principe', 682.0: ' Saudi Arabia', 686.0: ' Senegal', 688.0: ' Serbia', 690.0: ' Seychelles', 694.0: ' Sierra Leone', 702.0: ' Singapore', 703.0: ' Slovak Republic', 704.0: ' Viet Nam', 705.0: ' Slovenia', 706.0: ' Somalia', 710.0: ' South Africa', 716.0: ' Zimbabwe', 724.0: ' Spain', 728.0: ' South Sudan', 729.0: ' Sudan', 732.0: ' Western Sahara', 740.0: ' Suriname', 744.0: ' Svalbard and Jan Mayen', 748.0: ' Swaziland', 752.0: ' Sweden', 756.0: ' Switzerland', 760.0: ' Syrian Arab Republic', 762.0: ' Tajikistan', 764.0: ' Thailand', 768.0: ' Togo', 772.0: ' Tokelau', 776.0: ' Tonga', 780.0: ' Trinidad and Tobago', 784.0: ' United Arab Emirates', 788.0: ' Tunisia', 792.0: ' Turkey', 795.0: ' Turkmenistan', 796.0: ' Turks and Caicos Islands', 798.0: ' Tuvalu', 800.0: ' Uganda', 804.0: ' Ukraine', 807.0: ' North Macedonia', 810.0: ' U.S.S.R. (former country)', 818.0: ' Egypt', 826.0: ' United Kingdom of Great Britain and Northern Ireland', 831.0: ' Guernsey', 832.0: ' Jersey', 833.0: ' Isle of Man', 834.0: ' Tanzania, United Republic of', 840.0: ' United States of America', 850.0: ' Virgin Islands, U.S.', 854.0: ' Burkina Faso', 858.0: ' Uruguay', 860.0: ' Uzbekistan', 862.0: ' Venezuela, Bolivarian Republic of', 876.0: ' Wallis and Futuna', 882.0: ' Samoa', 887.0: ' Yemen', 890.0: ' Yugoslavia (former country)', 891.0: 'Serbia and Montenegro (former country)', 894.0: ' Zambia', 915.0: ' Kosovo', 1111.0: ' Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'NaN', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 2708 | 98 |
v252_edulvlb | 367 | v252_edulvlb | nominal | educational level spouse/partner: ESS-edulvlb coding (Q89) | {0.0: 'Not completed ISCED 1', 113.0: 'Completed ISCED 1', 129.0: 'short vocational ISCED 2', 212.0: 'General/pre-vocational ISCED 2 with access to 32x', 213.0: 'General ISCED 2 with access to all ISCED 3', 221.0: 'Vocational ISCED 2 without access to ISCED 3', 222.0: 'Vocational ISCED 2 with access to 32x only', 223.0: 'Vocational ISCED 2 with access to all ISCED 3', 229.0: 'short vocational ISCED 3', 311.0: 'General ISCED 3 without access to tertiary', 312.0: 'General ISCED 3 with access to non-university tertiary only', 313.0: 'General ISCED 3 with access to university', 321.0: 'Vocational ISCED 3 without access to tertiary', 322.0: 'Vocational ISCED 3 with access to non-university tertiary only', 323.0: 'Vocational ISCED 3 with access to university', 412.0: 'General ISCED 4, with access to non-university tertiary only', 413.0: 'General ISCED 4 with access to all first degrees at ISCED 6/7', 421.0: 'Vocational ISCED 4 without access to tertiary', 422.0: 'Vocational ISCED 4, with access to non-university tertiary only', 423.0: 'Vocational ISCED 4, with access to university', 510.0: "University qualification below bachelor's degree", 520.0: "Non-university qualification below bachelor's degree", 610.0: "Non-university bachelor's degree", 620.0: "University bachelor's degree", 710.0: "Non-university master's degree", 720.0: "University master's degree", 800.0: 'Doctoral degree', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35069 | 28 |
f252_edulvlb_CH | 368 | f252_edulvlb_CH | nominal | CH MODIFIED QUESTION (WEB_GERMAN): educational level spouse/partner: ESS-edulvlb coding (Q89) | {0.0: 'question wording corresponds to standard', 1.0: "2nd question part German WEB:'your' instead 'spouse/partner'.German:‘Ihr’/ 'your' same as for female partner"} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 2501 | 2 |
v252_edulvlb_2 | 369 | v252_edulvlb_2 | nominal | educational level spouse/partner: ESS-edulvlb coding two digits (Q89) | {0.0: 'Less than primary', 11.0: 'Primary: general education', 12.0: 'Primary: vocational programmes', 21.0: 'Lower secondary: general education', 22.0: 'Lower secondary: vocational programmes', 31.0: 'Upper secondary: general education', 32.0: 'Upper secondary: vocational programmes', 41.0: 'Post-secondary non tertiary: general education', 42.0: 'Post-secondary non tertiary: vocational programmes', 51.0: 'Short-cycle tertiary: general education', 52.0: 'Short-cycle tertiary: vocational programmes', 61.0: 'Bachelor or equivalent: general education', 62.0: 'Bachelor or equivalent: vocational programmes', 71.0: 'Master or equivalent: general education', 72.0: 'Master or equivalent: vocational programmes', 80.0: 'Doctoral or equivalent', 666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35069 | 17 |
v252_edulvlb_1 | 370 | v252_edulvlb_1 | nominal | educational level spouse/partner: ESS-edulvlb coding one digit (Q89) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35069 | 10 |
v252_ISCED_3 | 371 | v252_ISCED_3 | numeric | educational level spouse/partner: ISCED-code three digit (Q89) | {0.0: 'Less than primary', 100.0: 'Primary', 244.0: 'General lower secondary with direct access to upper secondary', 253.0: 'Vocational lower secondary without direct access to upper secondary', 254.0: 'Vocational lower secondary with direct access to upper secondary', 343.0: 'General upper secondary without direct access to first tertiary programmes', 344.0: 'General upper secondary with direct access to first tertiary programmes', 353.0: 'Vocational upper secondary without direct access to first tertiary programmes', 354.0: 'Vocational upper secondary with direct access to first tertiary programmes', 444.0: 'General post-secondary non-tertiary with direct access to first tertiary programmes', 453.0: 'Vocational post-secondary non-tertiary without direct access to first tertiary programmes', 454.0: 'Vocational post-secondary non-tertiary with direct access to first tertiary programmes', 540.0: 'General short-cycle tertiary', 550.0: 'Vocational short-cycle tertiary', 640.0: 'Academic Bachelor’s or equivalent level', 650.0: 'Professional Bachelor’s or equivalent level', 740.0: 'Academic Master’s or equivalent level', 750.0: 'Professional Master’s or equivalent level', 800.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35069 | 20 |
v252_ISCED_2 | 372 | v252_ISCED_2 | numeric | educational level spouse/partner: ISCED-code two digit (Q89) | {0.0: 'Pre-primary education', 10.0: 'Primary education', 24.0: 'Lower secondary general education', 25.0: 'Lower secondary vocational education', 34.0: 'Upper secondary general education', 35.0: 'Upper secondary vocational education', 44.0: 'Post-secondary non-tertiary general education', 45.0: 'Post-secondary non-tertiary vocational education', 54.0: 'Short-cycle tertiary general education', 55.0: 'Short-cycle tertiary vocational education', 64.0: 'Bachelor’s or equivalent level, academic', 65.0: 'Bachelor’s or equivalent level, professional', 74.0: 'Master’s or equivalent level, academic', 75.0: 'Master’s or equivalent level, professional', 80.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35069 | 16 |
v252_ISCED_2b | 373 | v252_ISCED_2b | nominal | educational level spouse/partner: ISCED-code two digit, second option (Q89) | {0.0: 'Less than primary', 10.0: 'Primary', 23.0: 'Lower secondary, no access to upper secondary education', 24.0: 'Lower secondary, access to upper secondary education', 33.0: 'Upper secondary, no access to upper secondary education', 34.0: 'Upper secondary, access to upper secondary education', 43.0: 'Post-secondary non-tertiary, no access to upper secondary education', 44.0: 'Post-secondary non-tertiary, access to upper secondary education', 50.0: 'Short-cycle tertiary education', 60.0: 'Bachelor’s or equivalent level', 70.0: 'Master or equivalent level', 80.0: 'Doctorate or equivalent level', 6666.0: 'Other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 35069 | 13 |
v252_ISCED_1 | 374 | v252_ISCED_1 | nominal | educational level spouse/partner: ISCED‐code one digit (Q89) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35820 | 10 |
v252_EISCED | 375 | v252_EISCED | numeric | educational level spouse/partner: ES-ISCED coding (Q89) | {0.0: 'No formal or less than primary education', 1.0: 'I - Primary education', 2.0: 'II - Lower secondary (including vocational training that is not considered as completion of upper secondary education)', 3.0: 'IIIb - Upper secondary without access to higher education', 4.0: 'IIIa - Upper secondary with access to higher education', 5.0: 'IV - Post-secondary/advanced vocational education below bachelor’s degree level', 6.0: "V1 - Bachelor's level", 7.0: "V2 - Master's and higher level", 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35069 | 9 |
v252_ISCED97 | 376 | v252_ISCED97 | numeric | educational level spouse/partner: ISCED97‐code one digit (Q89) | {0.0: ' 0 : Pre-primary education or none education', 1.0: ' 1 : Primary education or first stage of basic education', 2.0: ' 2 : Lower secondary or second stage of basic education', 3.0: ' 3 : (Upper) secondary education', 4.0: ' 4 : Post-secondary non-tertiary education', 5.0: ' 5 : First stage of tertiary education', 6.0: ' 6 : Second stage of tertiary education'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 35069 | 8 |
v252_8cat | 377 | v252_8cat | numeric | educational level spouse/partner: 8 categories (Q89) | {1.0: ' Inadequately completed elementary education', 2.0: ' Completed (compulsory) elementary education', 3.0: ' Incomplete secondary school: technical/vocational type', 4.0: ' Complete secondary school: technical/vocational type/secondary', 5.0: ' Incomplete secondary: university-preparatory type/secondary', 6.0: ' Complete secondary: university-preparatory type/full secondary', 7.0: ' Some university without degree/higher education - lower-level tertiary', 8.0: ' University with degree/higher education - upper-level tertiary'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 35069 | 9 |
v252_r | 378 | v252_r | nominal | educational level spouse/partner: recoded (Q89) | {1.0: 'lower', 2.0: 'medium', 3.0: 'higher', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35820 | 4 |
v252_cs | 379 | v252_cs | numeric | educational level spouse/partner: country-specific, ISO 3166-1 (Q89) | {801.0: ' AL: Without school', 802.0: ' AL: Primary education', 803.0: ' AL: Lower secondary (Leaving certificate)', 804.0: ' AL: Upper secondary general (Maturity diploma)', 805.0: ' AL: Upper secondary vocational (2 years, certificate of high technical or vocational education)', 806.0: ' AL: Upper secondary vocational (over 2 years, Maturity diplome)', 807.0: ' AL: Post-secondary non tertiary', 808.0: ' AL: University diploma/Bachelor', 809.0: ' AL: Vocational/Professional Master', 810.0: ' AL: Post-University', 811.0: ' AL: Master of Arts', 812.0: ' AL: Master of Science', 813.0: ' AL: Long term specialization', 814.0: ' AL: Doctoral Degree', 877.0: ' AL: not applicable', 888.0: ' AL: don’t know (spontaneous)', 899.0: ' AL: no answer (spontaneous)', 3101.0: ' AZ: None', 3102.0: ' AZ: Primary education (1-4 years)', 3103.0: ' AZ: Incomplete general secondary (5-8 grades)', 3104.0: ' AZ: General secondary education (9 grades)', 3105.0: ' AZ: Complete secondary education (11 grades)', 3106.0: ' AZ: Primary vocational-professional education (Vocational school/lyceum) (1-3 years)', 3107.0: ' AZ: Secondary special education (Technical school/College) (2-4 years)', 3108.0: ' AZ: Bachelor degree (4 years)', 3109.0: ' AZ: Master degree (2 years)', 3110.0: ' AZ: Science degree (former Aspirantura/PhD): Doctor of Philosophy, Doctor of Science', 3188.0: ' AZ: don’t know (spontaneous)', 3199.0: ' AZ: no answer (spontaneous)', 4001.0: 'AT: Not completed', 4002.0: 'AT: Completed primary education', 4003.0: 'AT: Completed Lower Secundary Education, New Secundary School', 4004.0: 'AT: Completed Polytechnic School, or one-year Middle School', 4005.0: 'AT: Completed Academic Secondary School (AHS), elementary level', 4006.0: 'AT: Completed apprenticeship (final apprenticeship examination)', 4007.0: 'AT: Completed Vocational Middle School (at least 2 years, e.g. commercial school, professional school)', 4008.0: 'AT: Completed Higher School Certificate (matriculation, general qualification for university entrance)', 4009.0: 'AT: Completed degree in health or nursing care', 4010.0: 'AT: Completed Higher School Certificate in Vocational Higher School', 4011.0: 'AT: Completed advanced vocational training', 4012.0: 'AT: Completed pedagoical/educational/medical degree', 4013.0: "AT: Completed Bachelor's degree at a university of applied sciences", 4014.0: "AT: Completed Bachelor's degree at a university", 4015.0: "AT: Completed Master's degree at a university of applied sciences", 4016.0: "AT: Completed Master's degree at a university of applied sciences", 4017.0: 'AT: Completed post-graduate university courses (e.g. MBA)', 4018.0: 'AT: Completed doctorate/PhD', 4019.0: 'AT: other', 5101.0: 'AM: Never went to school', 5102.0: 'AM: Less than 4 years of education', 5103.0: 'AM: 4 years of primary education', 5104.0: 'AM: Certificate of lower secondary/ basic secondary education, 9 years', 5105.0: 'AM: Certificate of initial vocational education after lower /basic education', 5106.0: 'AM: Advanced vocational education after lower /basic education', 5107.0: 'AM: Certificate of upper secondary education, 12 years', 5108.0: 'AM: Certificate of initial vocational training after upper secondary education', 5109.0: 'AM: Advanced vocational education after upper secondary education', 5110.0: 'AM: Bachelor degree 4 years', 5111.0: 'AM: Specialist degree', 5112.0: 'AM: Master degree 2 years', 5113.0: 'AM: Phd and habilitation', 7001.0: 'BA: No school (up to three grades of elementary school)', 7002.0: 'BA: Unfinished elementary school (4 to 7 grades)', 7003.0: 'BA: Completed elementary school', 7004.0: 'BA: Secondary vocational school up to 1-2 years', 7005.0: 'BA: Secondary vocational school up to 3 years', 7006.0: 'BA: Technical and related secondary vocational schools up to 4 years or more', 7007.0: 'BA: High school', 7008.0: 'BA: Professional study up to 2-3 years', 7009.0: "BA: Undergraduate university study - for the academic title 'baccalaureus' (3-4 years); Art academy (undergraduate stud", 7010.0: 'BA: Specialist graduate professional study (4-5 years); Specialist in the profession', 7011.0: 'BA: Graduate university study (4-6 years) - old program', 7012.0: "BA: Master's degree (5 years); Art academy (graduate study)", 7013.0: "BA: Master's degree of Science or completed postgraduate specialist studies", 7014.0: 'BA: Postgraduate Doctorate of Science', 10001.0: 'BG: Incomplete primary education', 10002.0: 'BG: Primary education', 10003.0: 'BG: Basic education', 10004.0: 'BG: Secondary general education', 10005.0: 'BG: Secondary special education Language Schools, Mathematics and Natural Science', 10006.0: 'BG: Secondary special education Arts and Sports', 10007.0: 'BG: Secondary vocational education', 10008.0: 'BG: Semi-higher education', 10009.0: 'BG: College - Professional bachelor', 10010.0: 'BG: Higher education - Bachelor', 10011.0: 'BG: Higher education - Master', 10012.0: 'BG: Doctor of science', 11201.0: 'BY: No education', 11202.0: 'BY: Primary school', 11203.0: 'BY: Certificate of lower secondary general education', 11204.0: 'BY: Certificate of upper general secondary education', 11205.0: 'BY: 1-2 year vocational education after lower secondary education', 11206.0: 'BY: Vocational training with upper secondary general training in parallel', 11207.0: 'BY: Certificate of upper vocational education with upper secondary general education', 11208.0: "BY: Bachelor's Degree", 11209.0: "BY: Higher education, Specialist's Diploma", 11210.0: "BY: Master's degree", 11211.0: 'BY: PhD', 11212.0: 'BY: Habilitation', 19101.0: 'HR: No school (up to three grades of elementary school)', 19102.0: 'HR: Unfinished elementary school (4 to 7 grades)', 19103.0: 'HR: Completed elementary school', 19104.0: 'HR: Secondary vocational school up to 1-2 years', 19105.0: 'HR: Secondary vocational school up to 3 years', 19106.0: 'HR: Technical and related secondary vocational schools up to 4 years or more', 19107.0: 'HR: High school', 19108.0: 'HR: Professional study up to 2-3 years', 19109.0: "HR: Undergraduate university study; for academic title 'baccalaureus' (3-4 years); Art academy (undergraduate study)", 19110.0: 'HR: Specialist graduate professional study (4-5 years); Specialist in the profession', 19111.0: 'HR: Graduate university study (4-6 years) - old program', 19112.0: "HR: Master's degree (5 years); Art academy (graduate study)", 19113.0: "HR: Master's degree of Science or completed postgraduate specialist studies", 19114.0: 'HR: Postgraduate Doctorate of Science', 20301.0: 'CZ Incomplete elementary education, unfinished 1st level (less than 5 grades/years)', 20302.0: 'CZ: Incomplete basic education (5+ years of schooling, only primary school completed, SZŠ, ZZŠ, general school)', 20303.0: 'CZ: Basic education (bougeois school)', 20304.0: 'CZ: Secondary education with an apprenticeship certificate, Secondary education without school-leaving examination', 20305.0: 'CZ: Secondary education without leaving examination followed by further studies with final exam (teaching, retraining)', 20306.0: 'CZ: Education with GCSE, full secondary vocational education with GCSE', 20307.0: 'CZ: Secondary educ. with school-leaving exam followed by studies with final exam (post-gr., follow-up courses etc)', 20308.0: 'CZ: Secondary general education with school-leaving examination (grammar school)', 20309.0: 'CZ: Post-secondary education with diploma: Higher vocational school (DiS), 5th and 6th year of conservatory (discharge)', 20310.0: "CZ: Bachelor's degree", 20311.0: "CZ: Master's degree education (Mgr., Ing., Ing., MUDr., MDDr., MVDr., …)", 20312.0: 'CZ: Doctoral studies, postgraduate education (Ph.D., Th.D., CSc, ...)', 20801.0: ' DK: municipal primary and lower secondary school: 5th grade or lower', 20802.0: ' DK: municipal primary and lower secondary school: 6th grade to 8th grade', 20803.0: ' DK: municipal primary and lower secondary school: 9th grade to 10th grade', 20804.0: " DK: '(Upper) Secondary Education: Gymnasium, Higher Preparatory Examination (HF), Higher Commercial Examination Program", 20805.0: ' DK: Semiskilled worker courses and short vocational education (less than 2 years)', 20806.0: ' DK: Vocational and technical (upper) secondary education', 20807.0: ' DK: Short-cycle higher non-university programmes (2-3 years)', 20808.0: ' DK: medium-cycle university and non-university programmes, proffesional bachelor degree (3-4 years)', 20809.0: ' DK: long-cycle university programmes, 1 part - university bachelor degree', 20810.0: ' DK: long-cycle university programmes, 2. part Kandidat degree (Masters) (5-6 years)', 20811.0: ' DK: Licentiat (closed research education program)', 20812.0: ' DK: Research Education, PhD., Doctor', 20813.0: ' DK: other', 23301.0: 'EE: Without primary education (less than 4 grades)', 23302.0: 'EE: Completed primary education (4-6 grades)', 23303.0: 'EE: Vocational education without completed primary education', 23304.0: 'EE: Completed basic education (7-9 grades)', 23305.0: 'EE: Vocational secondary education after basic education (training programme under 2 years)', 23306.0: 'EE: Completed secondary education', 23307.0: 'EE: Vocational secondary education after basic education (training programme 2 years or more)', 23308.0: 'EE: Vocational secondary education with secondary education or vocational secondary education (tehnical education) afte', 23309.0: 'EE: Vocational-secondary education (tehnical education) after secondary education', 23310.0: 'EE: Vocational university after secondary education diploma (up to 2 years trainings, but not Bachelor of Arts)', 23311.0: 'EE: Higher vocational education diploma or Bachelor of Arts degree (3-4 years of trainings)', 23312.0: 'EE: University Bachelor of Arts degree (3-4 years of trainings', 23313.0: 'EE: Master of Arts from Vocational university', 23314.0: 'EE: Master of Arts\xa0(3+2, 4+2 or by 5+4 system, incl. integrated BA and MA), higher education started before 1992 (speci', 23315.0: "EE: Doctor's degree", 24601.0: ' FI: Less than primary school (grades 1-6) or less than comprehensive school', 24602.0: " FI: Grades 1-6 of comprehensive school, or primary school or people's school", 24603.0: ' FI: Grades 7-9 of comprehensive school, or middle school', 24604.0: ' FI: General upper secondary school, matriculation examination', 24605.0: ' FI: Vocational upper secondary qualification, further vocational qualification', 24606.0: ' FI: Both matriculation examination and vocational qualification', 24607.0: ' FI: Specialist vocational qualification', 24608.0: ' FI: Post-secondary level vocational qualification (old)', 24609.0: " FI: Bachelors' degree in universities of applied sciences (polytechnic)", 24610.0: " FI: Bachelors' degree in university", 24611.0: " FI: Master's degree in universities of applied sciences (polytechnic)", 24612.0: " FI: Master's degree in university", 24613.0: ' FI: Licentiate degree', 24614.0: ' FI: Doctoral degree', 24615.0: ' FI: other', 25001.0: ' FR: no schooling or not completed primary education', 25002.0: " FR: Completed primary education, without the final certificate (Certificat d'études primaires=an old qualification off", 25003.0: " FR: Completed primary education, with the Certificat d'études primaires", 25004.0: " FR: Secondary education 1st cycle, without the final certificate (Brevet élémentaire, Brevet d'étude du premier cycl", 25005.0: " FR: Secondary education 1st cycle, with the final certificate (Brevet élémentaire, Brevet d'étude du premier cycle,", 25006.0: ' FR: Secondary education 2nd cycle, without the final exam (Baccalauréat)', 25007.0: " FR: Certificate of vocational ability : Certificat d'Aptitude Professionnelle (CAP) or Brevet d'Etudes Professionnelles", 25008.0: ' FR: Vocational qualification in care (home care, children care, health care)', 25009.0: ' FR: Vocational Baccalaureat', 25010.0: ' FR: Technological Baccalaureat or equivalent diploma', 25011.0: ' FR: General Baccalaureat or equivalent diploma', 25012.0: ' FR: Certificate of ability in Law, Diploma allowing admission in tertiary education', 25013.0: ' FR: Qualifications of vocational secondary education in health and care institutions (like Moniteur éducateur and equi', 25014.0: ' FR: University education, 1st cycle Diploma in general tertiary education (2 years), classes for competitive entrance', 25015.0: ' FR: Diploma in Technological Studies , Diploma of Advanced Technician', 25016.0: ' FR: Qualification in paramedical and social sectors (social assistant, etc,)', 25017.0: ' FR: Vocational Bachelor (University education, 1st graduation and apprenticeship)', 25018.0: ' FR: Bachelor (University education, 2nd cycle, 1st year and apprenticeship)', 25019.0: ' FR: Engineer Degree', 25020.0: ' FR: Vocational Master Degree', 25021.0: ' FR: Intermediate Master degree (university education, 2nd cycle, 2nd year), qualification of primary and secondary educ', 25022.0: ' FR: Research Master Degree, qualification of university education teachers', 25023.0: ' FR: Qualifications of architects, veterinaries, notaries', 25024.0: ' FR: Diplôma from selective “grandes écoles”', 25025.0: ' FR: Doctorate in medecine, odontology, pharmacy', 25026.0: ' FR: Doctorate', 26801.0: 'GE: No education', 26802.0: 'GE: Less than 6 years primary education', 26803.0: 'GE: Primary education completed, 6 years', 26804.0: 'GE: Basic/ lower general education certificate, 9 years', 26805.0: 'GE: Upper secondary general education certificate', 26806.0: 'GE: Professional diploma level 1, 2, or 3 (Vocational Education certificate, before 2010)', 26807.0: 'GE: Professional diploma level 4/5 (Secondary Vocational Educ. Certificate pre-2010, Certified Diploma 2008-2010)', 26808.0: 'GE: Interim qualification, short cycle tertiary education', 26809.0: 'GE: Bachelor degree', 26810.0: 'GE: Master degree', 26811.0: 'GE: Specialist studies', 26812.0: 'GE: Doctoral degree', 34801.0: 'HU: Never attented school; 1-3 years of primary school or equivalent', 34802.0: 'HU: 4-7 years of primary school or equivalent', 34803.0: 'HU: Completed primary school or equivalent', 34804.0: 'HU: Vocational school', 34805.0: 'HU: Vocational training after the 10. grade', 34806.0: 'HU: Completed secondary vocational education with school-leaving exam', 34807.0: 'HU: Completed secondary general education (Gymnasium) with school-leaving exam', 34808.0: 'HU: Post-secondary, mon-tertiary vocational education and training (E.g. technical school)', 34809.0: 'HU: Short-cycle tertiary level vocational education and training (VET)', 34810.0: "HU: College degree or college bachelor's degree - BA/BSc", 34811.0: "HU: University bachelor's degree - BA/BSc", 34812.0: "HU: College master's degree - MA/MSc", 34813.0: "HU: University degree or university master's degree - MA/MSc", 34814.0: 'HU: Doctoral degree', 35201.0: 'IS: Less than primary education', 35202.0: 'IS: Primary education', 35203.0: 'IS: Elementary school, 14–15 years of age', 35204.0: 'IS: National test', 35205.0: 'IS: Elementary school, 15–16 years of age (compulsory education)', 35206.0: 'IS: Elementary school, 16–17 years of age', 35207.0: 'IS: Vocational education at the lower secondary level', 35208.0: 'IS: Post-secondary non-tertiary education', 35209.0: 'IS: Other post-secondary, non-tertiary vocational education', 35210.0: 'IS: Upper secondary art education, 2+ years', 35211.0: 'IS: Certified trades, school diploma', 35212.0: 'IS: Matriculation examination', 35213.0: 'IS: Upper secondary education', 35214.0: 'IS: Master of a certified trade', 35215.0: 'IS: Tertiary general education, 2–3 years', 35216.0: 'IS: Tertiary vocational education, 2–3 years', 35217.0: 'IS: Bachelor’s or equivalent level', 35218.0: 'IS: Master’s or equivalent level', 35219.0: 'IS: Doctoral or equivalent level', 38001.0: ' IT: No title', 38002.0: ' IT: Elementary school diploma', 38003.0: ' IT: Vocational training', 38004.0: ' IT: Middle school diploma', 38005.0: ' IT: Post-obligation vocational regional qualification', 38006.0: " IT: Vocational institute diploma (2 or 3 years), including art teacher diploma, teacher's training school diploma and n", 38007.0: ' IT: High school diploma (5 years), technical or vocational institute, including art institute five years diploma', 38008.0: ' IT: High school diploma (4 or 5 years), classical, scientific, linguistic or psyco-pedagogical, musical lyceum, includi', 38009.0: ' IT: Post-(high school) diploma specialization, post-(high school) diploma vocational regional qualification, certificat', 38010.0: ' IT: Former system academic diploma (2 or 3 years), including higher institute of physical education, social service and', 38011.0: ' IT: Tertiary education not academic diploma: Music Conservatory, Academy of Fine Arts and similar', 38012.0: ' IT: Three years or first level degree (bachelor)', 38013.0: ' IT: First level specialization degree', 38014.0: " IT: Former system degree, master's degree, single cycle degree", 38015.0: ' IT: Second level specialization degree', 38016.0: ' IT: Post-graduate specialization (1 or 2 years)', 38017.0: ' IT: Post-graduate specialization (3 or 4 years), including medical specialization', 38018.0: ' IT: Doctoral degree', 42801.0: ' LV: Has not attended school', 42802.0: ' LV: Incompete primary education ( 6 grades)', 42803.0: ' LV: General primary education', 42804.0: ' LV: Basic vocational education', 42805.0: ' LV: Vocational education (without secondary) (1 year)', 42806.0: ' LV: Vocational education (without secondary) (3 years)', 42807.0: ' LV: General secondary education', 42808.0: ' LV: Vocational secondary education', 42809.0: ' LV: Vocational (not higher) education after general secondary education', 42810.0: ' LV: 1st level professional/ vocational higher education (college)', 42811.0: " LV: Bachelor's degree (professional)", 42812.0: " LV: Bachelor's degree (academic)", 42813.0: " LV: Master's degree (professional)", 42814.0: " LV: Master's degree (academic)", 42815.0: ' LV: Higher education obtained during the Soviet era', 42816.0: ' LV: Doctoral degree', 44000.0: ' LT: Less than primary', 44001.0: ' LT: Primary', 44002.0: ' LT: Lower general vocational', 44003.0: ' LT: General/ lower secondary', 44004.0: ' LT: General vocational', 44005.0: ' LT: Lower vocational after general', 44006.0: ' LT: Vocational after general', 44007.0: ' LT: Secondary', 44008.0: ' LT: Vocational with secondary', 44009.0: ' LT: Vocational after secondary', 44010.0: ' LT: Upper general and secondary vocational', 44011.0: ' LT: Upper secondary vocational', 44012.0: ' LT: Higher non university', 44013.0: ' LT: Higher university education (BA)', 44014.0: ' LT: Higher university education (MA)', 44015.0: ' LT: Higher university integrated education (I and II stages - 6 years)', 44016.0: ' LT: Doctoral studies and diploma', 49901.0: ' ME: No education', 49902.0: ' ME: Completed 4 years of primary education', 49903.0: ' ME: Completed lower secondary education', 49904.0: ' ME: 1-2 years vocational training', 49905.0: ' ME: Completed high school lasting three years', 49906.0: ' ME: Certificate of upper secondary vocational education (4 years)', 49907.0: ' ME: Secondary general (grammar schools, 4 years)', 49908.0: ' ME: Vocational exam', 49909.0: ' ME: Primary level diploma (after two or three years of bachelor level pre-Bologne reform)', 49910.0: ' ME: Completed two years of higher education that provides basic certificate but not entrance to MA studies', 49911.0: ' ME: Vocational studies (in duration of three years)', 49912.0: ' ME: Bachelor studies in duration of 3 years', 49913.0: ' ME: Specialization studies or four year studies in pre-Bologne system', 49914.0: ' ME: Master studies in duration of one year; Integrated studies (five year studies); Specialization studies', 49915.0: ' ME: Magister studies, Studies of medicine (in duration of 6 years, in old system), Specialisation', 49916.0: ' ME: PhD', 52801.0: 'NL: No completed primary education', 52802.0: 'NL: Primary education', 52803.0: "NL: Pre-vocational program ('vmbo level 1, 2 or 3' or 'lbo') or comparable", 52804.0: "NL: Preparatory secondary vocational education ('mavo' (previous 'vmbo') or 'vmbo level 4') or comparable", 52805.0: 'NL: Intermediate vocational educational, level 1 (duration 2 year)', 52806.0: "NL: Senior general secondary school ('havo') or comparable", 52807.0: "NL: Pre-university education ('vwo' or gymnasium) or comparable", 52808.0: "NL: kmbo', 'meao', 'mts' or comparable (duration 2-3 years)", 52809.0: 'NL: Intermediate vocational education , levels 2 and 3 (duration 2-3 years)', 52810.0: 'NL: Intermediate vocational education , level 4 (duration 4 years)', 52811.0: "NL: Intermediate vocational education plus (for people that completed 'havo')", 52812.0: 'NL: First year of university, Bachelor (propaedeutics)', 52813.0: 'NL: Shorter higher vocational education, shorter University of Applied Sciences (duration 2 or 3 years)', 52814.0: "NL: Higher vocational education / University of Applied Sciences, Bachelor's degree or comparable", 52815.0: "NL: University, Bachelor's degree", 52816.0: "NL: Higher vocational education / University of Applied Sciences, Master's degree or comparable", 52817.0: "NL: University, Master's degree or comparable", 52818.0: 'NL: University, doctoral degree (promotion)', 57801.0: ' NO: No education and/or preschool education', 57802.0: ' NO: Primary school (Elementary school. First part of compulsory education)\xa0', 57803.0: ' NO: Lower secondary school (Elementary school. Second part of compulsory education)', 57804.0: ' NO: Upper secondary school basic (including vk1, folkehøgskole, realskole)', 57805.0: ' NO: Upper secondary, complete graduation (including vk2, vk3, gymnas)', 57806.0: ' NO: Supplementary program for general university admissions certification', 57807.0: ' NO: University and university college, lower degree (including B.A., cand.mag)', 57808.0: ' NO: University and university college, higher degree (including M.A.)', 57809.0: ' NO: Scientific degree (Doctoral degree, Ph.D.)', 57810.0: ' NO: Primary education', 57824.0: ' NO: Lower secondary education - general', 57825.0: ' NO: Lower secondary education - vocational', 57834.0: ' NO: Upper secondary education - general', 57835.0: ' NO: Upper secondary education - vocational', 57844.0: ' NO: Post-secondary non-tertiary education - general', 57845.0: ' NO: Post-secondary non-tertiary education - vocational', 57854.0: ' NO: Short-cycle tertiary education - general', 57855.0: ' NO: Short-cycle tertiary education - vocational', 57856.0: ' NO: Short-cycle tertiary education - orientation unspecified', 57864.0: ' NO: Bachelor’s or equivalent level - academic', 57865.0: ' NO: Bachelor’s or equivalent level - professional', 57866.0: ' NO: Bachelor’s or equivalent level - orientation unspecified', 57874.0: ' NO: Master’s or equivalent level - academic', 57875.0: ' NO: Master’s or equivalent level - professional', 57876.0: ' NO: Master’s or equivalent level - orientation unspecified', 57884.0: ' NO: Doctoral or equivalent level - academic', 57885.0: ' NO: Doctoral or equivalent level - professional', 57886.0: ' NO: Doctoral or equivalent level - orientation unspecified', 61601.0: 'PL: primary education not completed', 61602.0: 'PL: primary education (6 grades or 4 grades)', 61603.0: 'PL: primary education', 61604.0: 'PL: lower secondary', 61605.0: 'PL: basic vocational education in agriculture', 61606.0: 'PL: basic vocational education', 61607.0: 'PL: basic vocational education', 61608.0: 'PL: upper secondary education without A-level diploma', 61609.0: 'PL: upper secondary education with A-level diploma', 61610.0: 'PL: upper secondary vocational or technical education', 61611.0: 'PL: upper secondary vocational or technical education with A-level diploma', 61612.0: 'PL: diploma of technician or post secondary education', 61613.0: "PL: post secondary education in teachers' college", 61614.0: "PL: BA or engineer's diploma", 61615.0: "PL: MA or doctor's diploma", 61616.0: "PL: PhD, assistant professor, professor's title", 61617.0: 'PL: other', 62001.0: ' PT: None', 62002.0: ' PT: Basic Level 1 (until 4th grade, primary education)', 62003.0: ' PT: Basic level 2 (until 6th grade, 1st cycle of former comercial or industrial high school)', 62004.0: ' PT: Type 1 education and formation courses. Attribution of level 1 professional qualification diploma', 62005.0: ' PT: Basic Level 3 (Certificate of conclusion of one of the following grades: 9th grade; former 5th grade of high school', 62006.0: ' PT: Type 2 education and formation courses. Attribution of level 2 professional qualification diploma', 62007.0: ' PT: Types 3 and 4 education and formation courses. Attribution of level 2 professional qualification diploma', 62008.0: ' PT: Secondary Level - Scientific-Humanistic studies (certificate of conclusion of one of the following grades: 12th gra', 62009.0: ' PT: Secondary Level -technologic and artistic studies (visual and audiovisual arts, dance and music), professional cour', 62010.0: ' PT: Technologic specialisation course. Attribution of Thecnologic specialisation diploma', 62011.0: ' PT: Polytechnic superior level:3 years bacalaureat (primary school teachers, social service, agricultural manager); f', 62012.0: ' PT: Polytechnic superior level:3-4 years graduation', 62013.0: ' PT: University superior level: 3-4 years graduation: 4 years two-stage graduation', 62014.0: ' PT: Post-graduation: post-graduation specialisation without degree atribution, MBA', 62015.0: ' PT: University superior level: gradution with more than 4 years: 5 years two-stage graduation', 62016.0: ' PT: Master degree (inc. integrated master)', 62017.0: ' PT: PhD', 64200.0: ' RO: No school at all', 64201.0: ' RO: Unfinished primary school', 64202.0: ' RO: Finished primary school', 64203.0: ' RO: Unfinished gymnasia', 64204.0: ' RO: Graduated gymnasia', 64205.0: ' RO: Apprentice (complementary) school', 64206.0: ' RO: School of Arts and Crafts', 64207.0: ' RO: School of Arts and Crafts - year of completion', 64208.0: ' RO: Professional school, less than 2 years', 64209.0: ' RO: Professional school, 2-4 years', 64210.0: ' RO: Unfinished High School', 64211.0: ' RO: Completed Theoretical High School (Baccalaureate Diploma)', 64212.0: ' RO: Completed Technological / Industrial High School (Baccalaureate Diploma)', 64213.0: ' RO: Foreman school without Baccalaureate', 64214.0: ' RO: Foreman school with Baccalaureate', 64215.0: ' RO: Post-highschool without Baccalaureate', 64216.0: ' RO: Post-highschool with Baccalaureate', 64217.0: ' RO: Unfinished university studies', 64218.0: ' RO: Sub-engineers or College', 64219.0: ' RO: BA (graduated university): 3 years', 64220.0: ' RO: BA (graduated university): 4 years', 64221.0: ' RO: BA (graduated university): 5 years', 64222.0: ' RO: BA (graduated university): 6 years', 64223.0: ' RO: Master', 64224.0: ' RO: PhD', 64301.0: 'RU: Did not study in school or completed 1-2 grades on school only (incomplete primary school)', 64302.0: 'RU: Graduated 3-7 grades of secondary school without attestat on basic general education', 64303.0: 'RU: Attestat of basic general educ.–complete 7 grade (pre 1958); 8 gr.(1960-1980); 9 gr.(modern system); no profes. ed', 64304.0: 'RU: Completed general secondary educ. (10 grade by old system,11 grade by new), got attestat; no professional educ.', 64305.0: 'RU: Got primary professional educ.–grad. PTU,FSU,FSO, profes.-techn. lyceum; no attestat of compl. sec. gen. educ.(<2y', 64306.0: 'RU: Got primary professional educ.– grad. PTU, professional-techn. lyceum, with attestat of completed sec. educ.(1-3 y', 64307.0: 'RU: Got secondary professional education – graduated technikum, uchilishe, college (2-4 years of study)', 64308.0: 'RU: Got diploma of bachelor in college after 4 years of education on two-stage new education system', 64309.0: 'RU: Got diploma of master in college after additional 2 years of education on two-stage new education system', 64310.0: 'RU: Completed high education by 5-6-years system (diploma of specialist)', 64311.0: 'RU: Scientific degree (candidate, doctor of sciences)', 68801.0: ' RS: No education (under 3rd grade)', 68802.0: ' RS: Primary education lower cycle (4th – 7th grade)', 68803.0: ' RS: Primary education upper cycle (8th grade)', 68804.0: ' RS: Secondary vocational 3 yrs', 68805.0: ' RS: Secondary vocational 4 yrs', 68806.0: ' RS: Secondary general (grammar schools)', 68807.0: ' RS: 1-2 years vocational training', 68808.0: ' RS: Higher schools', 68809.0: ' RS: University: Graduate studies', 68810.0: ' RS: Postgraduate studies: Specialist studies', 68811.0: ' RS: Postgraduate studies: Magister studies (outdated)', 68812.0: ' RS: Postgraduate studies: Master studies', 68813.0: ' RS: PhD studies/ Doctor’s Degree', 70301.0: 'SK: Not completed first stage of elementary school (not completed primary level of education)', 70302.0: 'SK: Not completed second stage of elementary school (not completed lower secondary level of education)', 70303.0: 'SK: Completed lower secondary educ.(completed 8/9 y. of elementary school or courses to complete lower secondary educ.)', 70304.0: ' SK: Practical (girl) school, two-years training programmes, requalification courses without vocational certificate', 70305.0: 'SK: secondary vocational school or sec. voc. training institution or sec. voc. school without leaving exam (no maturita)', 70306.0: 'SK: Secondary vocational school with leaving exam (with maturita), secondary vocat. training institution (with maturita)', 70307.0: 'SK: Eight year grammar school - 4 year grammar school', 70308.0: 'SK: Complementary study', 70309.0: "SK: Complementary 'postgraduate' pedagogical study", 70310.0: "SK: 'postgraduate' vocational study", 70311.0: 'SK: Postgraduate specialised study with leaving certificate, Dance conservatory', 70312.0: 'SK: Secondary vocational schoool with leaving examination (maturita) - six year study', 70313.0: 'SK: Bachelors degree', 70314.0: "SK: Master degree (engineering study, 'small' doctorate)", 70315.0: 'SK: postgraduate pedagogical study, teaching certificate for university graduates', 70316.0: 'SK: PhD study, doctoral degree', 70501.0: 'SI: Without school education', 70502.0: 'SI: Incomplete primary education', 70503.0: 'SI: Primary education', 70504.0: 'SI: Lower or upper secondary vocational education', 70505.0: 'SI: Secondary vocational education', 70506.0: 'SI: Secondary general education', 70507.0: 'SI: Higher vocational education, post-secondary education (2 years)', 70508.0: 'SI: Higher vocational education - 1. Bologna level.', 70509.0: 'SI: Higher education university education', 70510.0: "SI: Bologna master's degree", 70511.0: 'SI: Specialization', 70512.0: "SI: Master's degree", 70513.0: 'SI: phD', 72401.0: 'ES: No studies', 72402.0: 'ES: Not completed primary studies', 72403.0: 'ES: Old Primary School (Before 1970)', 72404.0: 'ES: Up to the 5th course of EGB (Basic General Education). From 70s to 90s', 72405.0: 'ES: Up to the 6th course of Primary School', 72406.0: 'ES: Primary School in Music and Dance', 72407.0: 'ES: Elementary High School (Before 1970)', 72408.0: 'ES: Up to the 8th course of EGB (Basic General Education). From 70s to 90s', 72409.0: 'ES: Compulsory High School', 72410.0: 'ES: High School (From 70s to 90s)', 72411.0: 'ES: Pre-University Studies (before 90s)', 72412.0: 'ES: Not compulsory High School', 72413.0: 'ES: Vocational training (initiation)', 72414.0: 'ES: Vocational training (initiation) (old Law)', 72415.0: 'ES: Vocational training (first grade) (old Law)', 72416.0: 'ES: Vocational training (first grade)', 72417.0: 'ES: Vocational training (medium grade, technical in various professions such as administrative or mechanic )', 72418.0: 'ES: Vocational training (medium grade, arts and design)', 72419.0: 'ES: Vocational training (medium grade, music and dance)', 72420.0: 'ES: Vocational training (high grade, industrial) (old Law)', 72421.0: 'ES: Vocational training (high grade, general) (old Law)', 72422.0: 'ES: Vocational training (high grade, general)', 72423.0: 'ES: Vocational training (high grade in arts)', 72424.0: 'ES: Medium Tertiary studies (nursery, school teacher, social worker)', 72425.0: 'ES: Medium Tertiary studies ( building engineer, industrial or business expert)', 72426.0: 'ES: Long tertiary studies', 72427.0: 'ES: Phd', 72428.0: 'ES: Other (specify)', 75201.0: ' SE: not completed ISCED level 1', 75202.0: ' SE: ISCED 1, completed primary education', 75203.0: ' SE: Qualification from general ISCED 2A programmes, access to ISCED 3A general or all 3', 75204.0: ' SE: Old version of vocational high school', 75205.0: ' SE: Old and current version of high school education that gives access to higher education', 75206.0: ' SE: Old version of mainly vocational high school with limited access to higher education', 75207.0: ' SE: Vocational high school', 75208.0: ' SE: Technical high school, formerly 4 years at high school, currently 3 years at high school and 4th year at university', 75209.0: ' SE: Degree from one year of studies at university college.', 75210.0: ' SE: Vocational degree, qualification obtained after high school graduation, from vocational training institutes', 75211.0: ' SE: Vocational degree, obtained at university or university college, e.g. old professional nursing programmes', 75212.0: ' SE: Vocational degree, obtained after high school graduation, longer programme 2-3 years from vocational training insti', 75213.0: " SE: Bachelor's degree or professional degree from a university college", 75214.0: " SE: Bachelor's degree or professional degree from a university", 75215.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75216.0: ' SE: Masters degree from university college', 75217.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75218.0: ' SE: Masters degree from university', 75219.0: ' SE: Research degree, licantiate degree, a sort of half-way doctoral degree, old degree that is not often used', 75220.0: ' SE: Research degree, phd', 75601.0: 'CH: Incompleted primary school', 75602.0: 'CH: Primary school', 75603.0: 'CH: Secondary education (first stage)', 75604.0: 'CH: Additional year of secondary education, preparation for vocational training', 75605.0: 'CH: General training school (2-3 years)', 75606.0: 'CH: Baccalaureate preparing for university', 75607.0: 'CH: Baccalaureate for adults or apprenticeship after Baccalaureate', 75608.0: 'CH: Diploma for teaching in primary school or preprimary school', 75609.0: 'CH: Vocational baccalaureate', 75610.0: 'CH: Vocational baccalaureate for adults', 75611.0: 'CH: Elementary vocational training (enterprise and school, 1-2 year)', 75612.0: 'CH: Apprenticeship (vocational training, dual system, 3-4 years)', 75613.0: 'CH: Second vocational training (or apprenticeship as second education)', 75614.0: 'CH: Advanced vocational qualification (specialization exam, federal certif. or dipl. of advanced vocational training)', 75615.0: 'CH: Higher vocational training (high school diploma - technical, administration, health, social work, applied arts)', 75616.0: 'CH:Higher vocational training (diploma of some specific high schools withrecognition of tertiary level)', 75617.0: 'CH: University of applied science and pedagogical university (Bachelor)', 75618.0: 'CH: University of applied science and pedagogical university (Master)', 75619.0: 'CH: University diploma (intermediary level)', 75620.0: 'CH: University diploma and post-graduate (including technical) (Bachelor and short university degree)', 75621.0: 'CH: University diploma and post-graduate (including technical) (degree requiring more than 4 years)', 75622.0: 'CH: University diploma and post-graduate (including technical) (Master)', 75623.0: 'CH: Doctoral degree', 75624.0: 'CH: other', 80400.0: ' UA: Incomplete primary secondary education (less than 4 classes)', 80401.0: ' UA: Primary secondary education (1- 3(4) classes)', 80402.0: ' UA: Basic secondary education (certificate for 8(9) class)', 80403.0: ' UA: Technical-vocational education based on basic secondary education (no certificate of complete secondary education)', 80404.0: ' UA: Technical-vocational education based on of basic general secondary (certificate of general secondary education and', 80405.0: ' UA: Complete secondary education (certificate of general secondary education of 10(11) classes)', 80406.0: ' UA: Additional training training on the basis of general secondary education (vocational and general educational course', 80407.0: ' UA: Technical-vocational education on the basis of general secondary education', 80408.0: ' UA: Professional pre-higher education (diploma of junior specialist)', 80409.0: " UA: Basic higher education (bachelor's degree)", 80410.0: ' UA: Full higher education (specialist)', 80411.0: ' UA: Full higher education (Master or equivalent)', 80412.0: ' UA: Academic degree (candidate, doctor, PhD)', 80701.0: 'MK: Without education', 80702.0: 'MK: Completed primary education (4 years of primary school)', 80703.0: 'MK: Completed primary and lower secondary education', 80704.0: 'MK: Completed upper secondary education, vocational training - 2 years', 80705.0: 'MK: Completed upper secondary education, vocational training - 3 years', 80706.0: 'MK: Completed upper secondary education, vocational training - 4 years', 80707.0: 'MK: Completed upper secondary education, general (high school) - 4 years', 80708.0: 'MK: Completed further education - short cycle university education - 2 years', 80709.0: 'MK: Bachelor degree - 3 years', 80710.0: 'MK: Bachelor degree - 4 years', 80711.0: 'MK: Completed postgraduate studies, specialist studies - 1 year', 80712.0: 'MK: Completed postgraduate studies, specialist studies - 2 years', 80713.0: 'MK: Completed postgraduate studies, master studies - 1 year', 80714.0: 'MK: Completed postgraduate studies, master studies - 2 years', 80715.0: 'MK: Doctoral degree, completed doctorate - 5 years', 80716.0: 'MK: Doctoral degree, completed doctorate (PhD studies) - 3 years', 80788.0: 'MK: don’t know (spontaneous)', 80799.0: 'MK: no answer (spontaneous)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 33460 | 521 |
v252_cs_DE1 | 380 | v252_cs_DE1 | numeric | DE COUNTRY-SPECIFIC: educational level spouse/partner (Q89), SCHOOL | {-10.0: 'multiple answers Mail', 27601.0: 'Primary school not completed', 27602.0: 'Primary school completed, secondary school not (yet) completed', 27603.0: 'Lower secondary schools (no access to general) or polytechnic secondary school (8th or 9th grade)', 27604.0: 'Lower secondary schools (access to general) or polytechnic secondary school (10th grade)', 27605.0: 'University of Applied Science entrance qualification (specialised vocational high schools etc.)', 27606.0: 'Grammar school (University entrance qualification)', 27607.0: 'Other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1471 | 7 |
v252_cs_DE2 | 381 | v252_cs_DE2 | numeric | DE COUNTRY-SPECIFIC: educational level spouse/partner (Q89), STUDIES | {-10.0: 'multiple answers Mail', 27601.0: 'No degree', 27602.0: 'Preliminary examination, preliminary diploma', 27603.0: 'College of public administration or University of Applied Sciences (diploma)', 27604.0: 'Vocational academy (diploma)', 27605.0: 'College of public administration or University of Applied Sciences (Bachelor)', 27606.0: 'Vocational academy (Bachelor)', 27607.0: 'University (Bachelor)', 27608.0: 'College of public administration or University of Applied Sciences (Master)', 27609.0: 'Vocational academy (Master)', 27610.0: 'University (diploma, Magister, state examaniation)', 27611.0: 'University (Master or postgraduate studies)', 27612.0: 'Doctoral studies', 27613.0: 'other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1390 | 13 |
v252_cs_DE3 | 382 | v252_cs_DE3 | numeric | DE COUNTRY-SPECIFIC: educational level spouse/partner (Q89), VOCATIONAL | {-10.0: 'multiple answers Mail', 27601.0: 'No degree', 27602.0: 'Pre-vocational training, qualification of short health sector programmes', 27603.0: 'Vocational training with diploma, but no Dual System (second cycle)', 27604.0: 'Medical assistant, nurse (qualification of health sector schools (2 and 3 years)', 27605.0: 'Qualification of civil servants for the medium level', 27606.0: 'Qualification in the Dual System (second cycle), industrial or agricultural sector', 27607.0: 'Qualification in the Dual System (second cycle), sales sector', 27608.0: 'Qualification of a vocational full-time school', 27609.0: 'Occupational qualification (second cycle) for students with occupational qualification or with university entrace qualif', 27610.0: "Master craftsmen's or Technician's qualification (or equal)", 27611.0: 'other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1379 | 11 |
v252_cs_GB1 | 383 | v252_cs_GB1 | numeric | GB COUNTRY-SPECIFIC:educational level spouse/partner: Up to 2 or more A-levels or equivalent (Q89a) | {82601.0: '2 or more A-levels or equivalent', 82602.0: ' GNVQ or GSVQ Intermediate', 82603.0: ' Vocational GCSE or equivalent', 82604.0: ' 5 or more GCSEs A*-C or equivalent', 82605.0: ' 1-4 GCSEs A*-C or equivalent', 82606.0: ' Skills for life', 82607.0: ' None of these'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 869 | 7 |
v252_cs_GB2 | 384 | v252_cs_GB2 | numeric | GB COUNTRY-SPECIFIC:educational level spouse/partner: Up to Ph.D or equivalent (Q89b) | {82601.0: ' Ph.D, D.Phil or equivalent', 82602.0: ' Masters Degree, M.Phil, Post-Graduate Diplomas and Certificates', 82603.0: ' 5 year University/CNAA first Degree (MB, BDS, BV etc)', 82604.0: ' 3-4 year University/CNAA first Degree (BA, BSc., BEd., BEng. Etc)', 82605.0: ' Nursing certificate, Teacher training, HE Diploma, Edexcel/BTEC/BEC/TEC/HND, City and Guilds, NVQ/SVQ - Leve', 82606.0: ' Foundation Degree (FdA, FdSc etc)', 82607.0: ' Edexcel/BTEC/BEC/TEC - Higher National Certificate (HNC) or equivalent', 82608.0: ' HE Access', 82609.0: ' Vocational A-level (AVCE), GCE Applied A-level, NVQ/SVQ Level 3, GNVQ/GSVQ Advanced, Edexcel/BTEC/BEC/TEC (G', 82610.0: ' (Modern) Apprenticeship, Advanced (Modern) Apprenticeship, SVQ/NVQ/Key Skills Level 1 and 2, City and Guild', 82611.0: ' None of these', 82612.0: ' Any other qualifications (specify)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 862 | 12 |
v253 | 385 | v253 | numeric | paid employment/no paid employment spouse/partner (Q90) | {1.0: '30h a week or more', 2.0: 'less then 30h a week', 3.0: 'self employed', 4.0: 'military service', 5.0: 'retired/pensioned', 6.0: 'homemaker not otherwise employed', 7.0: 'student', 8.0: 'unemployed', 9.0: 'disabled', 10.0: 'other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35752 | 10 |
v254 | 386 | v254 | numeric | employment/self-employment (spouse/partner): last job (Q91) | {1.0: 'employed', 2.0: 'self-employed'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'never had a paid job'} | True | 11543 | 2 |
v255_ISCO_2 | 387 | v255_ISCO_2 | numeric | kind of job spouse/partner - 2 digit ISCO08 code (Q92a) | {0.0: 'Armed Forces Occupations', 1.0: 'Commissioned Armed Forces Officers', 2.0: 'Non-commissioned Armed Forces Officers', 3.0: 'Armed Forces Occupations, Other Ranks', 10.0: 'ISCO 1-digit: Managers', 11.0: 'Chief Executives, Senior Officials and Legislators', 12.0: 'Administrative and Commercial Managers', 13.0: 'Production and Specialized Services Managers', 14.0: 'Hospitality, Retail and Other Services Managers', 20.0: 'ISCO 1-digit: Professionals', 21.0: 'Science and Engineering Professionals', 22.0: 'Health Professionals', 23.0: 'Teaching Professionals', 24.0: 'Business and Administration Professionals', 25.0: 'Information and Communications Technology Professionals', 26.0: 'Legal, Social and Cultural Professionals', 30.0: 'ISCO 1-digit: Technicians and Associate Professionals', 31.0: 'Science and Engineering Associate Professionals', 32.0: 'Health Associate Professionals', 33.0: 'Business and Administration Associate Professionals', 34.0: 'Legal, Social, Cultural and Related Associate Professionals', 35.0: 'Information and Communications Technicians', 40.0: 'ISCO 1-digit: Clerical Support Workers', 41.0: 'General and Keyboard Clerks', 42.0: 'Customer Services Clerks', 43.0: 'Numerical and Material Recording Clerks', 44.0: 'Other Clerical Support Workers', 50.0: 'ISCO 1-digit: Services and Sales Workers', 51.0: 'Personal Services Workers', 52.0: 'Sales Workers', 53.0: 'Personal Care Workers', 54.0: 'Protective Services Workers', 60.0: 'ISCO 1-digit: Skilled Agricultural, Forestry and Fishery Workers', 61.0: 'Market-oriented Skilled Agricultural Workers', 62.0: 'Market-oriented Skilled Forestry, Fishery and Hunting Workers', 63.0: 'Subsistence Farmers, Fishers, Hunters and Gatherers', 70.0: 'ISCO 1-digit: Craft and Related Trades Workers', 71.0: 'Building and Related Trades Workers (excluding Electricians)', 72.0: 'Metal, Machinery and Related Trades Workers', 73.0: 'Handicraft and Printing Workers', 74.0: 'Electrical and Electronics Trades Workers', 75.0: 'Food Processing, Woodworking, Garment and Other Craft and Related Trades Workers', 80.0: 'ISCO 1-digit: Plant and Machine Operators and Assemblers', 81.0: 'Stationary Plant and Machine Operators', 82.0: 'Assemblers', 83.0: 'Drivers and Mobile Plant Operators', 90.0: 'ISCO 1-digit: Elementary Occupations', 91.0: 'Cleaners and Helpers', 92.0: 'Agricultural, Forestry and Fishery Labourers', 93.0: 'Labourers in Mining, Construction, Manufacturing and Transport', 94.0: 'Food Preparation Assistants', 95.0: 'Street and Related Sales and Services Workers', 96.0: 'Refuse Workers and Other Elementary Workers', 99.0: 'ISCO could not be applied to response given'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 30950 | 47 |
v255_SIOPS | 388 | v255_SIOPS | numeric | kind of job spouse/partner -SIOPS08 code (Q92a) | {} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 30809 | 63 |
v255_ISEI | 389 | v255_ISEI | numeric | kind of job spouse/partner - ISEI08 code (Q92a) | {} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 30809 | 77 |
v255_ESeC | 390 | v255_ESeC | nominal | kind of job spouse/partner - ESEC08 code (Q92a) | {1.0: 'Large employers, higher mgrs/professionals', 2.0: 'Lower mgrs/professionals, higher supervisory/technicians', 3.0: 'Intermediate occupations', 4.0: 'Small employers and self-employed (non-agriculture)', 5.0: 'Small employers and self-employed (agriculture)', 6.0: 'Lower supervisors and technicians', 7.0: 'Lower sales and service', 8.0: 'Lower technical', 9.0: 'Routine', 99.0: 'ISCO could not be applied to response given'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey beak-off)', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 30809 | 9 |
v255_egp | 391 | v255_egp | numeric | kind of job spouse/partner - EGP11 (Q92a) | {1.0: 'I :Higher Controllers', 2.0: 'II :Lower Controllers', 3.0: 'IIIa:Routine Nonmanual', 4.0: 'IIIb:Lower Sales-Service', 5.0: 'IVa:Selfempl with empl', 6.0: 'IVb:Selfempl no empl', 7.0: 'V :Manual Supervisors', 8.0: 'VI :Skilled Worker', 9.0: 'VIIa:Unskilled Worker', 10.0: 'VIIb:Farm Labor', 11.0: 'IVc:Selfempl Farmer', 99999.0: 'ISCO could not be applied to response given'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 30796 | 11 |
v256 | 392 | v256 | numeric | spouse/partner has/had how many employees (Q93) | {1.0: 'none', 2.0: '1-9', 3.0: '10-24', 4.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3522 | 4 |
v257 | 393 | v257 | numeric | does/did spouse/partner supervise someone (Q94) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27871 | 2 |
v258 | 394 | v258 | numeric | how many people does she/he supervise (Q95) | {1.0: '1-9', 2.0: '10-24', 3.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 6562 | 3 |
v259 | 395 | v259 | numeric | respondent experienced unemployment longer than 3 months (Q96) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58024 | 2 |
v260 | 396 | v260 | numeric | dependency on social security during last 5 years respondent (Q97) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58561 | 2 |
v261 | 397 | v261 | nominal | households total net income (Q98) (standardized) | {1.0: 'A - 1st decile', 2.0: 'B - 2nd decile', 3.0: 'C - 3rd decile', 4.0: 'D - 4th decile', 5.0: 'E - 5th decile', 6.0: 'F - 6th decile', 7.0: 'G - 7th decile', 8.0: 'H - 8th decile', 9.0: 'I - 9th decile', 10.0: 'J - 10th decile'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50698 | 10 |
v261_ppp | 398 | v261_ppp | numeric | household monthly net income (x1000), corrected for ppp in euros (Q98) | {} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50698 | 348 |
v261_r | 399 | v261_r | numeric | household total net income (Q98) (income level) | {1.0: ' Low', 2.0: 'Middle', 3.0: 'High'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50698 | 3 |
v262_edulvlb | 400 | v262_edulvlb | nominal | educational level father: ESS-edulvlb coding (Q99) | {0.0: 'Not completed ISCED 1', 113.0: 'Completed ISCED 1', 129.0: 'short vocational ISCED 2', 212.0: 'General/pre-vocational ISCED 2 with access to 32x', 213.0: 'General ISCED 2 with access to all ISCED 3', 221.0: 'Vocational ISCED 2 without access to ISCED 3', 222.0: 'Vocational ISCED 2 with access to 32x only', 223.0: 'Vocational ISCED 2 with access to all ISCED 3', 229.0: 'short vocational ISCED 3', 311.0: 'General ISCED 3 without access to tertiary', 312.0: 'General ISCED 3 with access to non-university tertiary only', 313.0: 'General ISCED 3 with access to university', 321.0: 'Vocational ISCED 3 without access to tertiary', 322.0: 'Vocational ISCED 3 with access to non-university tertiary only', 323.0: 'Vocational ISCED 3 with access to university', 412.0: 'General ISCED 4, with access to non-university tertiary only', 413.0: 'General ISCED 4 with access to all first degrees at ISCED 6/7', 421.0: 'Vocational ISCED 4 without access to tertiary', 422.0: 'Vocational ISCED 4, with access to non-university tertiary only', 423.0: 'Vocational ISCED 4, with access to university', 510.0: "University qualification below bachelor's degree", 520.0: "Non-university qualification below bachelor's degree", 610.0: "Non-university bachelor's degree", 620.0: "University bachelor's degree", 710.0: "Non-university master's degree", 720.0: "University master's degree", 800.0: 'Doctoral degree', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53940 | 28 |
v262_edulvlb_2 | 401 | v262_edulvlb_2 | nominal | educational level father: ESS-edulvlb coding two digits (Q99) | {0.0: 'Less than primary', 11.0: 'Primary: general education', 12.0: 'Primary: vocational programmes', 21.0: 'Lower secondary: general education', 22.0: 'Lower secondary: vocational programmes', 31.0: 'Upper secondary: general education', 32.0: 'Upper secondary: vocational programmes', 41.0: 'Post-secondary non tertiary: general education', 42.0: 'Post-secondary non tertiary: vocational programmes', 51.0: 'Short-cycle tertiary: general education', 52.0: 'Short-cycle tertiary: vocational programmes', 61.0: 'Bachelor or equivalent: general education', 62.0: 'Bachelor or equivalent: vocational programmes', 71.0: 'Master or equivalent: general education', 72.0: 'Master or equivalent: vocational programmes', 80.0: 'Doctoral or equivalent', 666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53940 | 17 |
v262_edulvlb_1 | 402 | v262_edulvlb_1 | nominal | educational level father: ESS-edulvlb coding one digit (Q99) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53940 | 10 |
v262_ISCED_3 | 403 | v262_ISCED_3 | numeric | educational level father: ISCED-code three digit (Q99) | {0.0: 'Less than primary', 100.0: 'Primary', 244.0: 'General lower secondary with direct access to upper secondary', 253.0: 'Vocational lower secondary without direct access to upper secondary', 254.0: 'Vocational lower secondary with direct access to upper secondary', 343.0: 'General upper secondary without direct access to first tertiary programmes', 344.0: 'General upper secondary with direct access to first tertiary programmes', 353.0: 'Vocational upper secondary without direct access to first tertiary programmes', 354.0: 'Vocational upper secondary with direct access to first tertiary programmes', 444.0: 'General post-secondary non-tertiary with direct access to first tertiary programmes', 453.0: 'Vocational post-secondary non-tertiary without direct access to first tertiary programmes', 454.0: 'Vocational post-secondary non-tertiary with direct access to first tertiary programmes', 540.0: 'General short-cycle tertiary', 550.0: 'Vocational short-cycle tertiary', 640.0: 'Academic Bachelor’s or equivalent level', 650.0: 'Professional Bachelor’s or equivalent level', 740.0: 'Academic Master’s or equivalent level', 750.0: 'Professional Master’s or equivalent level', 800.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53940 | 20 |
v262_ISCED_2 | 404 | v262_ISCED_2 | numeric | educational level father: ISCED-code two digit (Q99) | {0.0: 'Pre-primary education', 10.0: 'Primary education', 24.0: 'Lower secondary general education', 25.0: 'Lower secondary vocational education', 34.0: 'Upper secondary general education', 35.0: 'Upper secondary vocational education', 44.0: 'Post-secondary non-tertiary general education', 45.0: 'Post-secondary non-tertiary vocational education', 54.0: 'Short-cycle tertiary general education', 55.0: 'Short-cycle tertiary vocational education', 64.0: 'Bachelor’s or equivalent level, academic', 65.0: 'Bachelor’s or equivalent level, professional', 74.0: 'Master’s or equivalent level, academic', 75.0: 'Master’s or equivalent level, professional', 80.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53940 | 16 |
v262_ISCED_2b | 405 | v262_ISCED_2b | nominal | educational level father: ISCED-code two digit, second option (Q99) | {0.0: 'Less than primary', 10.0: 'Primary', 23.0: 'Lower secondary, no access to upper secondary education', 24.0: 'Lower secondary, access to upper secondary education', 33.0: 'Upper secondary, no access to upper secondary education', 34.0: 'Upper secondary, access to upper secondary education', 43.0: 'Post-secondary non-tertiary, no access to upper secondary education', 44.0: 'Post-secondary non-tertiary, access to upper secondary education', 50.0: 'Short-cycle tertiary education', 60.0: 'Bachelor’s or equivalent level', 70.0: 'Master or equivalent level', 80.0: 'Doctorate or equivalent level', 6666.0: 'Other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 53940 | 13 |
v262_ISCED_1 | 406 | v262_ISCED_1 | nominal | educational level father: ISCED‐code one digit (Q99) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55017 | 10 |
v262_EISCED | 407 | v262_EISCED | numeric | educational level father: ES-ISCED coding (Q99) | {0.0: 'No formal or less than primary education', 1.0: 'I - Primary education', 2.0: 'II - Lower secondary (including vocational training that is not considered as completion of upper secondary education)', 3.0: 'IIIb - Upper secondary without access to higher education', 4.0: 'IIIa - Upper secondary with access to higher education', 5.0: 'IV - Post-secondary/advanced vocational education below bachelor’s degree level', 6.0: "V1 - Bachelor's level", 7.0: "V2 - Master's and higher level", 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53940 | 9 |
v262_ISCED97 | 408 | v262_ISCED97 | numeric | educational level father: ISCED97-code one digit (Q99) | {0.0: ' 0 : Pre-primary education or none education', 1.0: ' 1 : Primary education or first stage of basic education', 2.0: ' 2 : Lower secondary or second stage of basic education', 3.0: ' 3 : (Upper) secondary education', 4.0: ' 4 : Post-secondary non-tertiary education', 5.0: ' 5 : First stage of tertiary education', 6.0: ' 6 : Second stage of tertiary education'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 53940 | 8 |
v262_8cat | 409 | v262_8cat | numeric | educational level father: 8 categories (Q99) | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', 1.0: ' Inadequately completed elementary education', 2.0: ' Completed (compulsory) elementary education', 3.0: ' Incomplete secondary school: technical/vocational type', 4.0: ' Complete secondary school: technical/vocational type/secondary', 5.0: ' Incomplete secondary: university-preparatory type/secondary', 6.0: ' Complete secondary: university-preparatory type/full secondary', 7.0: ' Some university without degree/higher education - lower-level tertiary', 8.0: ' University with degree/higher education - upper-level tertiary'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 53940 | 9 |
v262_r | 410 | v262_r | nominal | educational level father: recoded (Q99) | {1.0: 'lower', 2.0: 'medium', 3.0: 'higher', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55017 | 4 |
v262_cs | 411 | v262_cs | numeric | educational level father: country-specific, ISO 3166-1 (Q99) | {801.0: ' AL: Without school', 802.0: ' AL: Primary education', 803.0: ' AL: Lower secondary (Leaving certificate)', 804.0: ' AL: Upper secondary general (Maturity diploma)', 805.0: ' AL: Upper secondary vocational (2 years, certificate of high technical or vocational education)', 806.0: ' AL: Upper secondary vocational (over 2 years, Maturity diplome)', 807.0: ' AL: Post-secondary non tertiary', 808.0: ' AL: University diploma/Bachelor', 809.0: ' AL: Vocational/Professional Master', 810.0: ' AL: Post-University', 811.0: ' AL: Master of Arts', 812.0: ' AL: Master of Science', 813.0: ' AL: Long term specialization', 814.0: ' AL: Doctoral Degree', 877.0: ' AL: not applicable', 888.0: ' AL: don’t know (spontaneous)', 899.0: ' AL: no answer (spontaneous)', 3101.0: ' AZ: None', 3102.0: ' AZ: Primary education (1-4 years)', 3103.0: ' AZ: Incomplete general secondary (5-8 grades)', 3104.0: ' AZ: General secondary education (9 grades)', 3105.0: ' AZ: Complete secondary education (11 grades)', 3106.0: ' AZ: Primary vocational-professional education (Vocational school/lyceum) (1-3 years)', 3107.0: ' AZ: Secondary special education (Technical school/College) (2-4 years)', 3108.0: ' AZ: Bachelor degree (4 years)', 3109.0: ' AZ: Master degree (2 years)', 3110.0: ' AZ: Science degree (former Aspirantura/PhD): Doctor of Philosophy, Doctor of Science', 3188.0: ' AZ: don’t know (spontaneous)', 3199.0: ' AZ: no answer (spontaneous)', 4001.0: 'AT: Not completed', 4002.0: 'AT: Completed primary education', 4003.0: 'AT: Completed Lower Secundary Education, New Secundary School', 4004.0: 'AT: Completed Polytechnic School, or one-year Middle School', 4005.0: 'AT: Completed Academic Secondary School (AHS), elementary level', 4006.0: 'AT: Completed apprenticeship (final apprenticeship examination)', 4007.0: 'AT: Completed Vocational Middle School (at least 2 years, e.g. commercial school, professional school)', 4008.0: 'AT: Completed Higher School Certificate (matriculation, general qualification for university entrance)', 4009.0: 'AT: Completed degree in health or nursing care', 4010.0: 'AT: Completed Higher School Certificate in Vocational Higher School', 4011.0: 'AT: Completed advanced vocational training', 4012.0: 'AT: Completed pedagoical/educational/medical degree', 4013.0: "AT: Completed Bachelor's degree at a university of applied sciences", 4014.0: "AT: Completed Bachelor's degree at a university", 4015.0: "AT: Completed Master's degree at a university of applied sciences", 4016.0: "AT: Completed Master's degree at a university of applied sciences", 4017.0: 'AT: Completed post-graduate university courses (e.g. MBA)', 4018.0: 'AT: Completed doctorate/PhD', 4019.0: 'AT: other', 5101.0: 'AM: Never went to school', 5102.0: 'AM: Less than 4 years of education', 5103.0: 'AM: 4 years of primary education', 5104.0: 'AM: Certificate of lower secondary/ basic secondary education, 9 years', 5105.0: 'AM: Certificate of initial vocational education after lower /basic education', 5106.0: 'AM: Advanced vocational education after lower /basic education', 5107.0: 'AM: Certificate of upper secondary education, 12 years', 5108.0: 'AM: Certificate of initial vocational training after upper secondary education', 5109.0: 'AM: Advanced vocational education after upper secondary education', 5110.0: 'AM: Bachelor degree 4 years', 5111.0: 'AM: Specialist degree', 5112.0: 'AM: Master degree 2 years', 5113.0: 'AM: Phd and habilitation', 7001.0: 'BA: No school (up to three grades of elementary school)', 7002.0: 'BA: Unfinished elementary school (4 to 7 grades)', 7003.0: 'BA: Completed elementary school', 7004.0: 'BA: Secondary vocational school up to 1-2 years', 7005.0: 'BA: Secondary vocational school up to 3 years', 7006.0: 'BA: Technical and related secondary vocational schools up to 4 years or more', 7007.0: 'BA: High school', 7008.0: 'BA: Professional study up to 2-3 years', 7009.0: "BA: Undergraduate university study - for the academic title 'baccalaureus' (3-4 years); Art academy (undergraduate stud", 7010.0: 'BA: Specialist graduate professional study (4-5 years); Specialist in the profession', 7011.0: 'BA: Graduate university study (4-6 years) - old program', 7012.0: "BA: Master's degree (5 years); Art academy (graduate study)", 7013.0: "BA: Master's degree of Science or completed postgraduate specialist studies", 7014.0: 'BA: Postgraduate Doctorate of Science', 10001.0: 'BG: Incomplete primary education', 10002.0: 'BG: Primary education', 10003.0: 'BG: Basic education', 10004.0: 'BG: Secondary general education', 10005.0: 'BG: Secondary special education Language Schools, Mathematics and Natural Science', 10006.0: 'BG: Secondary special education Arts and Sports', 10007.0: 'BG: Secondary vocational education', 10008.0: 'BG: Semi-higher education', 10009.0: 'BG: College - Professional bachelor', 10010.0: 'BG: Higher education - Bachelor', 10011.0: 'BG: Higher education - Master', 10012.0: 'BG: Doctor of science', 11201.0: 'BY: No education', 11202.0: 'BY: Primary school', 11203.0: 'BY: Certificate of lower secondary general education', 11204.0: 'BY: Certificate of upper general secondary education', 11205.0: 'BY: 1-2 year vocational education after lower secondary education', 11206.0: 'BY: Vocational training with upper secondary general training in parallel', 11207.0: 'BY: Certificate of upper vocational education with upper secondary general education', 11208.0: "BY: Bachelor's Degree", 11209.0: "BY: Higher education, Specialist's Diploma", 11210.0: "BY: Master's degree", 11211.0: 'BY: PhD', 11212.0: 'BY: Habilitation', 19101.0: 'HR: No school (up to three grades of elementary school)', 19102.0: 'HR: Unfinished elementary school (4 to 7 grades)', 19103.0: 'HR: Completed elementary school', 19104.0: 'HR: Secondary vocational school up to 1-2 years', 19105.0: 'HR: Secondary vocational school up to 3 years', 19106.0: 'HR: Technical and related secondary vocational schools up to 4 years or more', 19107.0: 'HR: High school', 19108.0: 'HR: Professional study up to 2-3 years', 19109.0: "HR: Undergraduate university study; for academic title 'baccalaureus' (3-4 years); Art academy (undergraduate study)", 19110.0: 'HR: Specialist graduate professional study (4-5 years); Specialist in the profession', 19111.0: 'HR: Graduate university study (4-6 years) - old program', 19112.0: "HR: Master's degree (5 years); Art academy (graduate study)", 19113.0: "HR: Master's degree of Science or completed postgraduate specialist studies", 19114.0: 'HR: Postgraduate Doctorate of Science', 20301.0: 'CZ Incomplete elementary education, unfinished 1st level (less than 5 grades/years)', 20302.0: 'CZ: Incomplete basic education (5+ years of schooling, only primary school completed, SZŠ, ZZŠ, general school)', 20303.0: 'CZ: Basic education (bougeois school)', 20304.0: 'CZ: Secondary education with an apprenticeship certificate, Secondary education without school-leaving examination', 20305.0: 'CZ: Secondary education without leaving examination followed by further studies with final exam (teaching, retraining)', 20306.0: 'CZ: Education with GCSE, full secondary vocational education with GCSE', 20307.0: 'CZ: Secondary educ. with school-leaving exam followed by studies with final exam (post-gr., follow-up courses etc)', 20308.0: 'CZ: Secondary general education with school-leaving examination (grammar school)', 20309.0: 'CZ: Post-secondary education with diploma: Higher vocational school (DiS), 5th and 6th year of conservatory (discharge)', 20310.0: "CZ: Bachelor's degree", 20311.0: "CZ: Master's degree education (Mgr., Ing., Ing., MUDr., MDDr., MVDr., …)", 20312.0: 'CZ: Doctoral studies, postgraduate education (Ph.D., Th.D., CSc, ...)', 20801.0: ' DK: municipal primary and lower secondary school: 5th grade or lower', 20802.0: ' DK: municipal primary and lower secondary school: 6th grade to 8th grade', 20803.0: ' DK: municipal primary and lower secondary school: 9th grade to 10th grade', 20804.0: " DK: '(Upper) Secondary Education: Gymnasium, Higher Preparatory Examination (HF), Higher Commercial Examination Program", 20805.0: ' DK: Semiskilled worker courses and short vocational education (less than 2 years)', 20806.0: ' DK: Vocational and technical (upper) secondary education', 20807.0: ' DK: Short-cycle higher non-university programmes (2-3 years)', 20808.0: ' DK: medium-cycle university and non-university programmes, proffesional bachelor degree (3-4 years)', 20809.0: ' DK: long-cycle university programmes, 1 part - university bachelor degree', 20810.0: ' DK: long-cycle university programmes, 2. part Kandidat degree (Masters) (5-6 years)', 20811.0: ' DK: Licentiat (closed research education program)', 20812.0: ' DK: Research Education, PhD., Doctor', 20813.0: ' DK: other', 23301.0: 'EE: Without primary education (less than 4 grades)', 23302.0: 'EE: Completed primary education (4-6 grades)', 23303.0: 'EE: Vocational education without completed primary education', 23304.0: 'EE: Completed basic education (7-9 grades)', 23305.0: 'EE: Vocational secondary education after basic education (training programme under 2 years)', 23306.0: 'EE: Completed secondary education', 23307.0: 'EE: Vocational secondary education after basic education (training programme 2 years or more)', 23308.0: 'EE: Vocational secondary education with secondary education or vocational secondary education (tehnical education) afte', 23309.0: 'EE: Vocational-secondary education (tehnical education) after secondary education', 23310.0: 'EE: Vocational university after secondary education diploma (up to 2 years trainings, but not Bachelor of Arts)', 23311.0: 'EE: Higher vocational education diploma or Bachelor of Arts degree (3-4 years of trainings)', 23312.0: 'EE: University Bachelor of Arts degree (3-4 years of trainings', 23313.0: 'EE: Master of Arts from Vocational university', 23314.0: 'EE: Master of Arts\xa0(3+2, 4+2 or by 5+4 system, incl. integrated BA and MA), higher education started before 1992 (speci', 23315.0: "EE: Doctor's degree", 24601.0: ' FI: Less than primary school (grades 1-6) or less than comprehensive school', 24602.0: " FI: Grades 1-6 of comprehensive school, or primary school or people's school", 24603.0: ' FI: Grades 7-9 of comprehensive school, or middle school', 24604.0: ' FI: General upper secondary school, matriculation examination', 24605.0: ' FI: Vocational upper secondary qualification, further vocational qualification', 24606.0: ' FI: Both matriculation examination and vocational qualification', 24607.0: ' FI: Specialist vocational qualification', 24608.0: ' FI: Post-secondary level vocational qualification (old)', 24609.0: " FI: Bachelors' degree in universities of applied sciences (polytechnic)", 24610.0: " FI: Bachelors' degree in university", 24611.0: " FI: Master's degree in universities of applied sciences (polytechnic)", 24612.0: " FI: Master's degree in university", 24613.0: ' FI: Licentiate degree', 24614.0: ' FI: Doctoral degree', 24615.0: ' FI: other', 25001.0: ' FR: no schooling or not completed primary education', 25002.0: " FR: Completed primary education, without the final certificate (Certificat d'études primaires=an old qualification off", 25003.0: " FR: Completed primary education, with the Certificat d'études primaires", 25004.0: " FR: Secondary education 1st cycle, without the final certificate (Brevet élémentaire, Brevet d'étude du premier cycl", 25005.0: " FR: Secondary education 1st cycle, with the final certificate (Brevet élémentaire, Brevet d'étude du premier cycle,", 25006.0: ' FR: Secondary education 2nd cycle, without the final exam (Baccalauréat)', 25007.0: " FR: Certificate of vocational ability : Certificat d'Aptitude Professionnelle (CAP) or Brevet d'Etudes Professionnelles", 25008.0: ' FR: Vocational qualification in care (home care, children care, health care)', 25009.0: ' FR: Vocational Baccalaureat', 25010.0: ' FR: Technological Baccalaureat or equivalent diploma', 25011.0: ' FR: General Baccalaureat or equivalent diploma', 25012.0: ' FR: Certificate of ability in Law, Diploma allowing admission in tertiary education', 25013.0: ' FR: Qualifications of vocational secondary education in health and care institutions (like Moniteur éducateur and equi', 25014.0: ' FR: University education, 1st cycle Diploma in general tertiary education (2 years), classes for competitive entrance', 25015.0: ' FR: Diploma in Technological Studies , Diploma of Advanced Technician', 25016.0: ' FR: Qualification in paramedical and social sectors (social assistant, etc,)', 25017.0: ' FR: Vocational Bachelor (University education, 1st graduation and apprenticeship)', 25018.0: ' FR: Bachelor (University education, 2nd cycle, 1st year and apprenticeship)', 25019.0: ' FR: Engineer Degree', 25020.0: ' FR: Vocational Master Degree', 25021.0: ' FR: Intermediate Master degree (university education, 2nd cycle, 2nd year), qualification of primary and secondary educ', 25022.0: ' FR: Research Master Degree, qualification of university education teachers', 25023.0: ' FR: Qualifications of architects, veterinaries, notaries', 25024.0: ' FR: Diplôma from selective “grandes écoles”', 25025.0: ' FR: Doctorate in medecine, odontology, pharmacy', 25026.0: ' FR: Doctorate', 26801.0: 'GE: No education', 26802.0: 'GE: Less than 6 years primary education', 26803.0: 'GE: Primary education completed, 6 years', 26804.0: 'GE: Basic/ lower general education certificate, 9 years', 26805.0: 'GE: Upper secondary general education certificate', 26806.0: 'GE: Professional diploma level 1, 2, or 3 (Vocational Education certificate, before 2010)', 26807.0: 'GE: Professional diploma level 4/5 (Secondary Vocational Educ. Certificate pre-2010, Certified Diploma 2008-2010)', 26808.0: 'GE: Interim qualification, short cycle tertiary education', 26809.0: 'GE: Bachelor degree', 26810.0: 'GE: Master degree', 26811.0: 'GE: Specialist studies', 26812.0: 'GE: Doctoral degree', 34801.0: 'HU: Never attented school; 1-3 years of primary school or equivalent', 34802.0: 'HU: 4-7 years of primary school or equivalent', 34803.0: 'HU: Completed primary school or equivalent', 34804.0: 'HU: Vocational school', 34805.0: 'HU: Vocational training after the 10. grade', 34806.0: 'HU: Completed secondary vocational education with school-leaving exam', 34807.0: 'HU: Completed secondary general education (Gymnasium) with school-leaving exam', 34808.0: 'HU: Post-secondary, mon-tertiary vocational education and training (E.g. technical school)', 34809.0: 'HU: Short-cycle tertiary level vocational education and training (VET)', 34810.0: "HU: College degree or college bachelor's degree - BA/BSc", 34811.0: "HU: University bachelor's degree - BA/BSc", 34812.0: "HU: College master's degree - MA/MSc", 34813.0: "HU: University degree or university master's degree - MA/MSc", 34814.0: 'HU: Doctoral degree', 35201.0: 'IS: Less than primary education', 35202.0: 'IS: Primary education', 35203.0: 'IS: Elementary school, 14–15 years of age', 35204.0: 'IS: National test', 35205.0: 'IS: Elementary school, 15–16 years of age (compulsory education)', 35206.0: 'IS: Elementary school, 16–17 years of age', 35207.0: 'IS: Vocational education at the lower secondary level', 35208.0: 'IS: Post-secondary non-tertiary education', 35209.0: 'IS: Other post-secondary, non-tertiary vocational education', 35210.0: 'IS: Upper secondary art education, 2+ years', 35211.0: 'IS: Certified trades, school diploma', 35212.0: 'IS: Matriculation examination', 35213.0: 'IS: Upper secondary education', 35214.0: 'IS: Master of a certified trade', 35215.0: 'IS: Tertiary general education, 2–3 years', 35216.0: 'IS: Tertiary vocational education, 2–3 years', 35217.0: 'IS: Bachelor’s or equivalent level', 35218.0: 'IS: Master’s or equivalent level', 35219.0: 'IS: Doctoral or equivalent level', 38001.0: ' IT: No title', 38002.0: ' IT: Elementary school diploma', 38003.0: ' IT: Vocational training', 38004.0: ' IT: Middle school diploma', 38005.0: ' IT: Post-obligation vocational regional qualification', 38006.0: " IT: Vocational institute diploma (2 or 3 years), including art teacher diploma, teacher's training school diploma and n", 38007.0: ' IT: High school diploma (5 years), technical or vocational institute, including art institute five years diploma', 38008.0: ' IT: High school diploma (4 or 5 years), classical, scientific, linguistic or psyco-pedagogical, musical lyceum, includi', 38009.0: ' IT: Post-(high school) diploma specialization, post-(high school) diploma vocational regional qualification, certificat', 38010.0: ' IT: Former system academic diploma (2 or 3 years), including higher institute of physical education, social service and', 38011.0: ' IT: Tertiary education not academic diploma: Music Conservatory, Academy of Fine Arts and similar', 38012.0: ' IT: Three years or first level degree (bachelor)', 38013.0: ' IT: First level specialization degree', 38014.0: " IT: Former system degree, master's degree, single cycle degree", 38015.0: ' IT: Second level specialization degree', 38016.0: ' IT: Post-graduate specialization (1 or 2 years)', 38017.0: ' IT: Post-graduate specialization (3 or 4 years), including medical specialization', 38018.0: ' IT: Doctoral degree', 42801.0: ' LV: Has not attended school', 42802.0: ' LV: Incompete primary education ( 6 grades)', 42803.0: ' LV: General primary education', 42804.0: ' LV: Basic vocational education', 42805.0: ' LV: Vocational education (without secondary) (1 year)', 42806.0: ' LV: Vocational education (without secondary) (3 years)', 42807.0: ' LV: General secondary education', 42808.0: ' LV: Vocational secondary education', 42809.0: ' LV: Vocational (not higher) education after general secondary education', 42810.0: ' LV: 1st level professional/ vocational higher education (college)', 42811.0: " LV: Bachelor's degree (professional)", 42812.0: " LV: Bachelor's degree (academic)", 42813.0: " LV: Master's degree (professional)", 42814.0: " LV: Master's degree (academic)", 42815.0: ' LV: Higher education obtained during the Soviet era', 42816.0: ' LV: Doctoral degree', 44000.0: ' LT: Less than primary', 44001.0: ' LT: Primary', 44002.0: ' LT: Lower general vocational', 44003.0: ' LT: General/ lower secondary', 44004.0: ' LT: General vocational', 44005.0: ' LT: Lower vocational after general', 44006.0: ' LT: Vocational after general', 44007.0: ' LT: Secondary', 44008.0: ' LT: Vocational with secondary', 44009.0: ' LT: Vocational after secondary', 44010.0: ' LT: Upper general and secondary vocational', 44011.0: ' LT: Upper secondary vocational', 44012.0: ' LT: Higher non university', 44013.0: ' LT: Higher university education (BA)', 44014.0: ' LT: Higher university education (MA)', 44015.0: ' LT: Higher university integrated education (I and II stages - 6 years)', 44016.0: ' LT: Doctoral studies and diploma', 49901.0: ' ME: No education', 49902.0: ' ME: Completed 4 years of primary education', 49903.0: ' ME: Completed lower secondary education', 49904.0: ' ME: 1-2 years vocational training', 49905.0: ' ME: Completed high school lasting three years', 49906.0: ' ME: Certificate of upper secondary vocational education (4 years)', 49907.0: ' ME: Secondary general (grammar schools, 4 years)', 49908.0: ' ME: Vocational exam', 49909.0: ' ME: Primary level diploma (after two or three years of bachelor level pre-Bologne reform)', 49910.0: ' ME: Completed two years of higher education that provides basic certificate but not entrance to MA studies', 49911.0: ' ME: Vocational studies (in duration of three years)', 49912.0: ' ME: Bachelor studies in duration of 3 years', 49913.0: ' ME: Specialization studies or four year studies in pre-Bologne system', 49914.0: ' ME: Master studies in duration of one year; Integrated studies (five year studies); Specialization studies', 49915.0: ' ME: Magister studies, Studies of medicine (in duration of 6 years, in old system), Specialisation', 49916.0: ' ME: PhD', 52801.0: 'NL: No completed primary education', 52802.0: 'NL: Primary education', 52803.0: "NL: Pre-vocational program ('vmbo level 1, 2 or 3' or 'lbo') or comparable", 52804.0: "NL: Preparatory secondary vocational education ('mavo' (previous 'vmbo') or 'vmbo level 4') or comparable", 52805.0: 'NL: Intermediate vocational educational, level 1 (duration 2 year)', 52806.0: "NL: Senior general secondary school ('havo') or comparable", 52807.0: "NL: Pre-university education ('vwo' or gymnasium) or comparable", 52808.0: "NL: kmbo', 'meao', 'mts' or comparable (duration 2-3 years)", 52809.0: 'NL: Intermediate vocational education , levels 2 and 3 (duration 2-3 years)', 52810.0: 'NL: Intermediate vocational education , level 4 (duration 4 years)', 52811.0: "NL: Intermediate vocational education plus (for people that completed 'havo')", 52812.0: 'NL: First year of university, Bachelor (propaedeutics)', 52813.0: 'NL: Shorter higher vocational education, shorter University of Applied Sciences (duration 2 or 3 years)', 52814.0: "NL: Higher vocational education / University of Applied Sciences, Bachelor's degree or comparable", 52815.0: "NL: University, Bachelor's degree", 52816.0: "NL: Higher vocational education / University of Applied Sciences, Master's degree or comparable", 52817.0: "NL: University, Master's degree or comparable", 52818.0: 'NL: University, doctoral degree (promotion)', 57801.0: ' NO: No education and/or preschool education', 57802.0: ' NO: Primary school (Elementary school. First part of compulsory education)\xa0', 57803.0: ' NO: Lower secondary school (Elementary school. Second part of compulsory education)', 57804.0: ' NO: Upper secondary school basic (including vk1, folkehøgskole, realskole)', 57805.0: ' NO: Upper secondary, complete graduation (including vk2, vk3, gymnas)', 57806.0: ' NO: Supplementary program for general university admissions certification', 57807.0: ' NO: University and university college, lower degree (including B.A., cand.mag)', 57808.0: ' NO: University and university college, higher degree (including M.A.)', 57809.0: ' NO: Scientific degree (Doctoral degree, Ph.D.)', 57810.0: ' NO: Primary education', 57824.0: ' NO: Lower secondary education - general', 57825.0: ' NO: Lower secondary education - vocational', 57834.0: ' NO: Upper secondary education - general', 57835.0: ' NO: Upper secondary education - vocational', 57844.0: ' NO: Post-secondary non-tertiary education - general', 57845.0: ' NO: Post-secondary non-tertiary education - vocational', 57854.0: ' NO: Short-cycle tertiary education - general', 57855.0: ' NO: Short-cycle tertiary education - vocational', 57856.0: ' NO: Short-cycle tertiary education - orientation unspecified', 57864.0: ' NO: Bachelor’s or equivalent level - academic', 57865.0: ' NO: Bachelor’s or equivalent level - professional', 57866.0: ' NO: Bachelor’s or equivalent level - orientation unspecified', 57874.0: ' NO: Master’s or equivalent level - academic', 57875.0: ' NO: Master’s or equivalent level - professional', 57876.0: ' NO: Master’s or equivalent level - orientation unspecified', 57884.0: ' NO: Doctoral or equivalent level - academic', 57885.0: ' NO: Doctoral or equivalent level - professional', 57886.0: ' NO: Doctoral or equivalent level - orientation unspecified', 61601.0: 'PL: primary education not completed', 61602.0: 'PL: primary education (6 grades or 4 grades)', 61603.0: 'PL: primary education', 61604.0: 'PL: lower secondary', 61605.0: 'PL: basic vocational education in agriculture', 61606.0: 'PL: basic vocational education', 61607.0: 'PL: basic vocational education', 61608.0: 'PL: upper secondary education without A-level diploma', 61609.0: 'PL: upper secondary education with A-level diploma', 61610.0: 'PL: upper secondary vocational or technical education', 61611.0: 'PL: upper secondary vocational or technical education with A-level diploma', 61612.0: 'PL: diploma of technician or post secondary education', 61613.0: "PL: post secondary education in teachers' college", 61614.0: "PL: BA or engineer's diploma", 61615.0: "PL: MA or doctor's diploma", 61616.0: "PL: PhD, assistant professor, professor's title", 61617.0: 'PL: other', 62001.0: ' PT: None', 62002.0: ' PT: Basic Level 1 (until 4th grade, primary education)', 62003.0: ' PT: Basic level 2 (until 6th grade, 1st cycle of former comercial or industrial high school)', 62004.0: ' PT: Type 1 education and formation courses. Attribution of level 1 professional qualification diploma', 62005.0: ' PT: Basic Level 3 (Certificate of conclusion of one of the following grades: 9th grade; former 5th grade of high school', 62006.0: ' PT: Type 2 education and formation courses. Attribution of level 2 professional qualification diploma', 62007.0: ' PT: Types 3 and 4 education and formation courses. Attribution of level 2 professional qualification diploma', 62008.0: ' PT: Secondary Level - Scientific-Humanistic studies (certificate of conclusion of one of the following grades: 12th gra', 62009.0: ' PT: Secondary Level -technologic and artistic studies (visual and audiovisual arts, dance and music), professional cour', 62010.0: ' PT: Technologic specialisation course. Attribution of Thecnologic specialisation diploma', 62011.0: ' PT: Polytechnic superior level:3 years bacalaureat (primary school teachers, social service, agricultural manager); f', 62012.0: ' PT: Polytechnic superior level:3-4 years graduation', 62013.0: ' PT: University superior level: 3-4 years graduation: 4 years two-stage graduation', 62014.0: ' PT: Post-graduation: post-graduation specialisation without degree atribution, MBA', 62015.0: ' PT: University superior level: gradution with more than 4 years: 5 years two-stage graduation', 62016.0: ' PT: Master degree (inc. integrated master)', 62017.0: ' PT: PhD', 64200.0: ' RO: No school at all', 64201.0: ' RO: Unfinished primary school', 64202.0: ' RO: Finished primary school', 64203.0: ' RO: Unfinished gymnasia', 64204.0: ' RO: Graduated gymnasia', 64205.0: ' RO: Apprentice (complementary) school', 64206.0: ' RO: School of Arts and Crafts', 64207.0: ' RO: School of Arts and Crafts - year of completion', 64208.0: ' RO: Professional school, less than 2 years', 64209.0: ' RO: Professional school, 2-4 years', 64210.0: ' RO: Unfinished High School', 64211.0: ' RO: Completed Theoretical High School (Baccalaureate Diploma)', 64212.0: ' RO: Completed Technological / Industrial High School (Baccalaureate Diploma)', 64213.0: ' RO: Foreman school without Baccalaureate', 64214.0: ' RO: Foreman school with Baccalaureate', 64215.0: ' RO: Post-highschool without Baccalaureate', 64216.0: ' RO: Post-highschool with Baccalaureate', 64217.0: ' RO: Unfinished university studies', 64218.0: ' RO: Sub-engineers or College', 64219.0: ' RO: BA (graduated university): 3 years', 64220.0: ' RO: BA (graduated university): 4 years', 64221.0: ' RO: BA (graduated university): 5 years', 64222.0: ' RO: BA (graduated university): 6 years', 64223.0: ' RO: Master', 64224.0: ' RO: PhD', 64301.0: 'RU: Did not study in school or completed 1-2 grades on school only (incomplete primary school)', 64302.0: 'RU: Graduated 3-7 grades of secondary school without attestat on basic general education', 64303.0: 'RU: Attestat of basic general educ.–complete 7 grade (pre 1958); 8 gr.(1960-1980); 9 gr.(modern system); no profes. ed', 64304.0: 'RU: Completed general secondary educ. (10 grade by old system,11 grade by new), got attestat; no professional educ.', 64305.0: 'RU: Got primary professional educ.–grad. PTU,FSU,FSO, profes.-techn. lyceum; no attestat of compl. sec. gen. educ.(<2y', 64306.0: 'RU: Got primary professional educ.– grad. PTU, professional-techn. lyceum, with attestat of completed sec. educ.(1-3 y', 64307.0: 'RU: Got secondary professional education – graduated technikum, uchilishe, college (2-4 years of study)', 64308.0: 'RU: Got diploma of bachelor in college after 4 years of education on two-stage new education system', 64309.0: 'RU: Got diploma of master in college after additional 2 years of education on two-stage new education system', 64310.0: 'RU: Completed high education by 5-6-years system (diploma of specialist)', 64311.0: 'RU: Scientific degree (candidate, doctor of sciences)', 68801.0: ' RS: No education (under 3rd grade)', 68802.0: ' RS: Primary education lower cycle (4th – 7th grade)', 68803.0: ' RS: Primary education upper cycle (8th grade)', 68804.0: ' RS: Secondary vocational 3 yrs', 68805.0: ' RS: Secondary vocational 4 yrs', 68806.0: ' RS: Secondary general (grammar schools)', 68807.0: ' RS: 1-2 years vocational training', 68808.0: ' RS: Higher schools', 68809.0: ' RS: University: Graduate studies', 68810.0: ' RS: Postgraduate studies: Specialist studies', 68811.0: ' RS: Postgraduate studies: Magister studies (outdated)', 68812.0: ' RS: Postgraduate studies: Master studies', 68813.0: ' RS: PhD studies/ Doctor’s Degree', 70301.0: 'SK: Not completed first stage of elementary school (not completed primary level of education)', 70302.0: 'SK: Not completed second stage of elementary school (not completed lower secondary level of education)', 70303.0: 'SK: Completed lower secondary educ.(completed 8/9 y. of elementary school or courses to complete lower secondary educ.)', 70304.0: ' SK: Practical (girl) school, two-years training programmes, requalification courses without vocational certificate', 70305.0: 'SK: secondary vocational school or sec. voc. training institution or sec. voc. school without leaving exam (no maturita)', 70306.0: 'SK: Secondary vocational school with leaving exam (with maturita), secondary vocat. training institution (with maturita)', 70307.0: 'SK: Eight year grammar school - 4 year grammar school', 70308.0: 'SK: Complementary study', 70309.0: "SK: Complementary 'postgraduate' pedagogical study", 70310.0: "SK: 'postgraduate' vocational study", 70311.0: 'SK: Postgraduate specialised study with leaving certificate, Dance conservatory', 70312.0: 'SK: Secondary vocational schoool with leaving examination (maturita) - six year study', 70313.0: 'SK: Bachelors degree', 70314.0: "SK: Master degree (engineering study, 'small' doctorate)", 70315.0: 'SK: postgraduate pedagogical study, teaching certificate for university graduates', 70316.0: 'SK: PhD study, doctoral degree', 70501.0: 'SI: Without school education', 70502.0: 'SI: Incomplete primary education', 70503.0: 'SI: Primary education', 70504.0: 'SI: Lower or upper secondary vocational education', 70505.0: 'SI: Secondary vocational education', 70506.0: 'SI: Secondary general education', 70507.0: 'SI: Higher vocational education, post-secondary education (2 years)', 70508.0: 'SI: Higher vocational education - 1. Bologna level.', 70509.0: 'SI: Higher education university education', 70510.0: "SI: Bologna master's degree", 70511.0: 'SI: Specialization', 70512.0: "SI: Master's degree", 70513.0: 'SI: phD', 72401.0: 'ES: No studies', 72402.0: 'ES: Not completed primary studies', 72403.0: 'ES: Old Primary School (Before 1970)', 72404.0: 'ES: Up to the 5th course of EGB (Basic General Education). From 70s to 90s', 72405.0: 'ES: Up to the 6th course of Primary School', 72406.0: 'ES: Primary School in Music and Dance', 72407.0: 'ES: Elementary High School (Before 1970)', 72408.0: 'ES: Up to the 8th course of EGB (Basic General Education). From 70s to 90s', 72409.0: 'ES: Compulsory High School', 72410.0: 'ES: High School (From 70s to 90s)', 72411.0: 'ES: Pre-University Studies (before 90s)', 72412.0: 'ES: Not compulsory High School', 72413.0: 'ES: Vocational training (initiation)', 72414.0: 'ES: Vocational training (initiation) (old Law)', 72415.0: 'ES: Vocational training (first grade) (old Law)', 72416.0: 'ES: Vocational training (first grade)', 72417.0: 'ES: Vocational training (medium grade, technical in various professions such as administrative or mechanic )', 72418.0: 'ES: Vocational training (medium grade, arts and design)', 72419.0: 'ES: Vocational training (medium grade, music and dance)', 72420.0: 'ES: Vocational training (high grade, industrial) (old Law)', 72421.0: 'ES: Vocational training (high grade, general) (old Law)', 72422.0: 'ES: Vocational training (high grade, general)', 72423.0: 'ES: Vocational training (high grade in arts)', 72424.0: 'ES: Medium Tertiary studies (nursery, school teacher, social worker)', 72425.0: 'ES: Medium Tertiary studies ( building engineer, industrial or business expert)', 72426.0: 'ES: Long tertiary studies', 72427.0: 'ES: Phd', 72428.0: 'ES: Other (specify)', 75201.0: ' SE: not completed ISCED level 1', 75202.0: ' SE: ISCED 1, completed primary education', 75203.0: ' SE: Qualification from general ISCED 2A programmes, access to ISCED 3A general or all 3', 75204.0: ' SE: Old version of vocational high school', 75205.0: ' SE: Old and current version of high school education that gives access to higher education', 75206.0: ' SE: Old version of mainly vocational high school with limited access to higher education', 75207.0: ' SE: Vocational high school', 75208.0: ' SE: Technical high school, formerly 4 years at high school, currently 3 years at high school and 4th year at university', 75209.0: ' SE: Degree from one year of studies at university college.', 75210.0: ' SE: Vocational degree, qualification obtained after high school graduation, from vocational training institutes', 75211.0: ' SE: Vocational degree, obtained at university or university college, e.g. old professional nursing programmes', 75212.0: ' SE: Vocational degree, obtained after high school graduation, longer programme 2-3 years from vocational training insti', 75213.0: " SE: Bachelor's degree or professional degree from a university college", 75214.0: " SE: Bachelor's degree or professional degree from a university", 75215.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75216.0: ' SE: Masters degree from university college', 75217.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75218.0: ' SE: Masters degree from university', 75219.0: ' SE: Research degree, licantiate degree, a sort of half-way doctoral degree, old degree that is not often used', 75220.0: ' SE: Research degree, phd', 75601.0: 'CH: Incompleted primary school', 75602.0: 'CH: Primary school', 75603.0: 'CH: Secondary education (first stage)', 75604.0: 'CH: Additional year of secondary education, preparation for vocational training', 75605.0: 'CH: General training school (2-3 years)', 75606.0: 'CH: Baccalaureate preparing for university', 75607.0: 'CH: Baccalaureate for adults or apprenticeship after Baccalaureate', 75608.0: 'CH: Diploma for teaching in primary school or preprimary school', 75609.0: 'CH: Vocational baccalaureate', 75610.0: 'CH: Vocational baccalaureate for adults', 75611.0: 'CH: Elementary vocational training (enterprise and school, 1-2 year)', 75612.0: 'CH: Apprenticeship (vocational training, dual system, 3-4 years)', 75613.0: 'CH: Second vocational training (or apprenticeship as second education)', 75614.0: 'CH: Advanced vocational qualification (specialization exam, federal certif. or dipl. of advanced vocational training)', 75615.0: 'CH: Higher vocational training (high school diploma - technical, administration, health, social work, applied arts)', 75616.0: 'CH:Higher vocational training (diploma of some specific high schools withrecognition of tertiary level)', 75617.0: 'CH: University of applied science and pedagogical university (Bachelor)', 75618.0: 'CH: University of applied science and pedagogical university (Master)', 75619.0: 'CH: University diploma (intermediary level)', 75620.0: 'CH: University diploma and post-graduate (including technical) (Bachelor and short university degree)', 75621.0: 'CH: University diploma and post-graduate (including technical) (degree requiring more than 4 years)', 75622.0: 'CH: University diploma and post-graduate (including technical) (Master)', 75623.0: 'CH: Doctoral degree', 75624.0: 'CH: other', 80400.0: ' UA: Incomplete primary secondary education (less than 4 classes)', 80401.0: ' UA: Primary secondary education (1- 3(4) classes)', 80402.0: ' UA: Basic secondary education (certificate for 8(9) class)', 80403.0: ' UA: Technical-vocational education based on basic secondary education (no certificate of complete secondary education)', 80404.0: ' UA: Technical-vocational education based on of basic general secondary (certificate of general secondary education and', 80405.0: ' UA: Complete secondary education (certificate of general secondary education of 10(11) classes)', 80406.0: ' UA: Additional training training on the basis of general secondary education (vocational and general educational course', 80407.0: ' UA: Technical-vocational education on the basis of general secondary education', 80408.0: ' UA: Professional pre-higher education (diploma of junior specialist)', 80409.0: " UA: Basic higher education (bachelor's degree)", 80410.0: ' UA: Full higher education (specialist)', 80411.0: ' UA: Full higher education (Master or equivalent)', 80412.0: ' UA: Academic degree (candidate, doctor, PhD)', 80701.0: 'MK: Without education', 80702.0: 'MK: Completed primary education (4 years of primary school)', 80703.0: 'MK: Completed primary and lower secondary education', 80704.0: 'MK: Completed upper secondary education, vocational training - 2 years', 80705.0: 'MK: Completed upper secondary education, vocational training - 3 years', 80706.0: 'MK: Completed upper secondary education, vocational training - 4 years', 80707.0: 'MK: Completed upper secondary education, general (high school) - 4 years', 80708.0: 'MK: Completed further education - short cycle university education - 2 years', 80709.0: 'MK: Bachelor degree - 3 years', 80710.0: 'MK: Bachelor degree - 4 years', 80711.0: 'MK: Completed postgraduate studies, specialist studies - 1 year', 80712.0: 'MK: Completed postgraduate studies, specialist studies - 2 years', 80713.0: 'MK: Completed postgraduate studies, master studies - 1 year', 80714.0: 'MK: Completed postgraduate studies, master studies - 2 years', 80715.0: 'MK: Doctoral degree, completed doctorate - 5 years', 80716.0: 'MK: Doctoral degree, completed doctorate (PhD studies) - 3 years', 80788.0: 'MK: don’t know (spontaneous)', 80799.0: 'MK: no answer (spontaneous)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51560 | 525 |
v262_cs_DE1 | 412 | v262_cs_DE1 | numeric | DE COUNTRY-SPECIFIC: educational level father (Q99), SCHOOL | {-10.0: 'multiple answers Mail', 27601.0: 'Primary school not completed', 27602.0: 'Primary school completed, secondary school not (yet) completed', 27603.0: 'Lower secondary schools (no access to general) or polytechnic secondary school (8th or 9th grade)', 27604.0: 'Lower secondary schools (access to general) or polytechnic secondary school (10th grade)', 27605.0: 'University of Applied Science entrance qualification (specialised vocational high schools etc.)', 27606.0: 'Grammar school (University entrance qualification)', 27607.0: 'Other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1835 | 7 |
v262_cs_DE2 | 413 | v262_cs_DE2 | numeric | DE COUNTRY-SPECIFIC: educational level father (Q99), STUDIES | {-10.0: 'multiple answers Mail', 27601.0: 'No degree', 27602.0: 'Preliminary examination, preliminary diploma', 27603.0: 'College of public administration or University of Applied Sciences (diploma)', 27604.0: 'Vocational academy (diploma)', 27605.0: 'College of public administration or University of Applied Sciences (Bachelor)', 27606.0: 'Vocational academy (Bachelor)', 27607.0: 'University (Bachelor)', 27608.0: 'College of public administration or University of Applied Sciences (Master)', 27609.0: 'Vocational academy (Master)', 27610.0: 'University (diploma, Magister, state examaniation)', 27611.0: 'University (Master or postgraduate studies)', 27612.0: 'Doctoral studies', 27613.0: 'other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1822 | 13 |
v262_cs_DE3 | 414 | v262_cs_DE3 | numeric | DE COUNTRY-SPECIFIC: educational level father (Q99), VOCATIONAL | {-10.0: 'multiple answers Mail', 27601.0: 'No degree', 27602.0: 'Pre-vocational training, qualification of short health sector programmes', 27603.0: 'Vocational training with diploma, but no Dual System (second cycle)', 27604.0: 'Medical assistant, nurse (qualification of health sector schools (2 and 3 years)', 27605.0: 'Qualification of civil servants for the medium level', 27606.0: 'Qualification in the Dual System (second cycle), industrial or agricultural sector', 27607.0: 'Qualification in the Dual System (second cycle), sales sector', 27608.0: 'Qualification of a vocational full-time school', 27609.0: 'Occupational qualification (second cycle) for students with occupational qualification or with university entrace qualif', 27610.0: "Master craftsmen's or Technician's qualification (or equal)", 27611.0: 'other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1737 | 11 |
v262_cs_GB1 | 415 | v262_cs_GB1 | numeric | GB COUNTRY-SPECIFIC:educational level father: Up to 2 or more A-levels or equivalent (Q99a) | {82601.0: '2 or more A-levels or equivalent', 82602.0: ' GNVQ or GSVQ Intermediate', 82603.0: ' Vocational GCSE or equivalent', 82604.0: ' 5 or more GCSEs A*-C or equivalent', 82605.0: ' 1-4 GCSEs A*-C or equivalent', 82606.0: ' Skills for life', 82607.0: ' None of these'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1449 | 7 |
v262_cs_GB2 | 416 | v262_cs_GB2 | numeric | GB COUNTRY-SPECIFIC:educational level father: Up to Ph.D or equivalent (Q99b) | {82601.0: ' Ph.D, D.Phil or equivalent', 82602.0: ' Masters Degree, M.Phil, Post-Graduate Diplomas and Certificates', 82603.0: ' 5 year University/CNAA first Degree (MB, BDS, BV etc)', 82604.0: ' 3-4 year University/CNAA first Degree (BA, BSc., BEd., BEng. Etc)', 82605.0: ' Nursing certificate, Teacher training, HE Diploma, Edexcel/BTEC/BEC/TEC/HND, City and Guilds, NVQ/SVQ - Leve', 82606.0: ' Foundation Degree (FdA, FdSc etc)', 82607.0: ' Edexcel/BTEC/BEC/TEC - Higher National Certificate (HNC) or equivalent', 82608.0: ' HE Access', 82609.0: ' Vocational A-level (AVCE), GCE Applied A-level, NVQ/SVQ Level 3, GNVQ/GSVQ Advanced, Edexcel/BTEC/BEC/TEC (G', 82610.0: ' (Modern) Apprenticeship, Advanced (Modern) Apprenticeship, SVQ/NVQ/Key Skills Level 1 and 2, City and Guild', 82611.0: ' None of these', 82612.0: ' Any other qualifications (specify)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1456 | 12 |
v263_edulvlb | 417 | v263_edulvlb | nominal | educational level mother: ESS-edulvlb coding (Q100) | {0.0: 'Not completed ISCED 1', 113.0: 'Completed ISCED 1', 129.0: 'short vocational ISCED 2', 212.0: 'General/pre-vocational ISCED 2 with access to 32x', 213.0: 'General ISCED 2 with access to all ISCED 3', 221.0: 'Vocational ISCED 2 without access to ISCED 3', 222.0: 'Vocational ISCED 2 with access to 32x only', 223.0: 'Vocational ISCED 2 with access to all ISCED 3', 229.0: 'short vocational ISCED 3', 311.0: 'General ISCED 3 without access to tertiary', 312.0: 'General ISCED 3 with access to non-university tertiary only', 313.0: 'General ISCED 3 with access to university', 321.0: 'Vocational ISCED 3 without access to tertiary', 322.0: 'Vocational ISCED 3 with access to non-university tertiary only', 323.0: 'Vocational ISCED 3 with access to university', 412.0: 'General ISCED 4, with access to non-university tertiary only', 413.0: 'General ISCED 4 with access to all first degrees at ISCED 6/7', 421.0: 'Vocational ISCED 4 without access to tertiary', 422.0: 'Vocational ISCED 4, with access to non-university tertiary only', 423.0: 'Vocational ISCED 4, with access to university', 510.0: "University qualification below bachelor's degree", 520.0: "Non-university qualification below bachelor's degree", 610.0: "Non-university bachelor's degree", 620.0: "University bachelor's degree", 710.0: "Non-university master's degree", 720.0: "University master's degree", 800.0: 'Doctoral degree', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55028 | 28 |
v263_edulvlb_2 | 418 | v263_edulvlb_2 | nominal | educational level mother: ESS-edulvlb coding two digits (Q100) | {0.0: 'Less than primary', 11.0: 'Primary: general education', 12.0: 'Primary: vocational programmes', 21.0: 'Lower secondary: general education', 22.0: 'Lower secondary: vocational programmes', 31.0: 'Upper secondary: general education', 32.0: 'Upper secondary: vocational programmes', 41.0: 'Post-secondary non tertiary: general education', 42.0: 'Post-secondary non tertiary: vocational programmes', 51.0: 'Short-cycle tertiary: general education', 52.0: 'Short-cycle tertiary: vocational programmes', 61.0: 'Bachelor or equivalent: general education', 62.0: 'Bachelor or equivalent: vocational programmes', 71.0: 'Master or equivalent: general education', 72.0: 'Master or equivalent: vocational programmes', 80.0: 'Doctoral or equivalent', 666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55028 | 17 |
v263_edulvlb_1 | 419 | v263_edulvlb_1 | nominal | educational level mother: ESS-edulvlb coding one digit (Q100) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55028 | 10 |
v263_ISCED_3 | 420 | v263_ISCED_3 | numeric | educational level mother: ISCED-code three digit (Q100) | {0.0: 'Less than primary', 100.0: 'Primary', 244.0: 'General lower secondary with direct access to upper secondary', 253.0: 'Vocational lower secondary without direct access to upper secondary', 254.0: 'Vocational lower secondary with direct access to upper secondary', 343.0: 'General upper secondary without direct access to first tertiary programmes', 344.0: 'General upper secondary with direct access to first tertiary programmes', 353.0: 'Vocational upper secondary without direct access to first tertiary programmes', 354.0: 'Vocational upper secondary with direct access to first tertiary programmes', 444.0: 'General post-secondary non-tertiary with direct access to first tertiary programmes', 453.0: 'Vocational post-secondary non-tertiary without direct access to first tertiary programmes', 454.0: 'Vocational post-secondary non-tertiary with direct access to first tertiary programmes', 540.0: 'General short-cycle tertiary', 550.0: 'Vocational short-cycle tertiary', 640.0: 'Academic Bachelor’s or equivalent level', 650.0: 'Professional Bachelor’s or equivalent level', 740.0: 'Academic Master’s or equivalent level', 750.0: 'Professional Master’s or equivalent level', 800.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55028 | 20 |
v263_ISCED_2 | 421 | v263_ISCED_2 | numeric | educational level mother: ISCED-code two digit (Q100) | {0.0: 'Pre-primary education', 10.0: 'Primary education', 24.0: 'Lower secondary general education', 25.0: 'Lower secondary vocational education', 34.0: 'Upper secondary general education', 35.0: 'Upper secondary vocational education', 44.0: 'Post-secondary non-tertiary general education', 45.0: 'Post-secondary non-tertiary vocational education', 54.0: 'Short-cycle tertiary general education', 55.0: 'Short-cycle tertiary vocational education', 64.0: 'Bachelor’s or equivalent level, academic', 65.0: 'Bachelor’s or equivalent level, professional', 74.0: 'Master’s or equivalent level, academic', 75.0: 'Master’s or equivalent level, professional', 80.0: 'Doctoral or equivalent level', 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55028 | 16 |
v263_ISCED_2b | 422 | v263_ISCED_2b | nominal | educational level mother: ISCED-code two digit, second option (Q100) | {0.0: 'Less than primary', 10.0: 'Primary', 23.0: 'Lower secondary, no access to upper secondary education', 24.0: 'Lower secondary, access to upper secondary education', 33.0: 'Upper secondary, no access to upper secondary education', 34.0: 'Upper secondary, access to upper secondary education', 43.0: 'Post-secondary non-tertiary, no access to upper secondary education', 44.0: 'Post-secondary non-tertiary, access to upper secondary education', 50.0: 'Short-cycle tertiary education', 60.0: 'Bachelor’s or equivalent level', 70.0: 'Master or equivalent level', 80.0: 'Doctorate or equivalent level', 6666.0: 'Other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 55028 | 13 |
v263_ISCED_1 | 423 | v263_ISCED_1 | nominal | educational level mother: ISCED-code one digit (Q100) | {0.0: 'Less than primary', 1.0: 'Primary', 2.0: 'Lower secondary', 3.0: 'Upper secondary', 4.0: 'Post-secondary non tertiary', 5.0: 'Short-cycle tertiary', 6.0: 'Bachelor or equivalent', 7.0: 'Master or equivalent', 8.0: 'Doctoral or equivalent', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56130 | 10 |
v263_EISCED | 424 | v263_EISCED | numeric | educational level mother: ES-ISCED coding (Q100) | {0.0: 'No formal or less than primary education', 1.0: 'I - Primary education', 2.0: 'II - Lower secondary (including vocational training that is not considered as completion of upper secondary education)', 3.0: 'IIIb - Upper secondary without access to higher education', 4.0: 'IIIa - Upper secondary with access to higher education', 5.0: 'IV - Post-secondary/advanced vocational education below bachelor’s degree level', 6.0: "V1 - Bachelor's level", 7.0: "V2 - Master's and higher level", 6666.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow up non response', -7.0: 'matrix not applied', -6.0: 'survey break-off', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55028 | 9 |
v263_ISCED97 | 425 | v263_ISCED97 | numeric | educational level mother: ISCED97-code one digit (Q99) | {0.0: ' 0 : Pre-primary education or none education', 1.0: ' 1 : Primary education or first stage of basic education', 2.0: ' 2 : Lower secondary or second stage of basic education', 3.0: ' 3 : (Upper) secondary education', 4.0: ' 4 : Post-secondary non-tertiary education', 5.0: ' 5 : First stage of tertiary education', 6.0: ' 6 : Second stage of tertiary education'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 55028 | 8 |
v263_8cat | 426 | v263_8cat | numeric | educational level mother: 8 categories (Q100) | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', 1.0: ' Inadequately completed elementary education', 2.0: ' Completed (compulsory) elementary education', 3.0: ' Incomplete secondary school: technical/vocational type', 4.0: ' Complete secondary school: technical/vocational type/secondary', 5.0: ' Incomplete secondary: university-preparatory type/secondary', 6.0: ' Complete secondary: university-preparatory type/full secondary', 7.0: ' Some university without degree/higher education - lower-level tertiary', 8.0: ' University with degree/higher education - upper-level tertiary'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 55028 | 9 |
v263_r | 427 | v263_r | nominal | educational level mother: recoded (Q100) | {1.0: 'lower', 2.0: 'medium', 3.0: 'higher', 66.0: 'other'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56130 | 4 |
v263_cs | 428 | v263_cs | numeric | educational level mother: country-specific, ISO 3166-1 (Q100) | {801.0: ' AL: Without school', 802.0: ' AL: Primary education', 803.0: ' AL: Lower secondary (Leaving certificate)', 804.0: ' AL: Upper secondary general (Maturity diploma)', 805.0: ' AL: Upper secondary vocational (2 years, certificate of high technical or vocational education)', 806.0: ' AL: Upper secondary vocational (over 2 years, Maturity diplome)', 807.0: ' AL: Post-secondary non tertiary', 808.0: ' AL: University diploma/Bachelor', 809.0: ' AL: Vocational/Professional Master', 810.0: ' AL: Post-University', 811.0: ' AL: Master of Arts', 812.0: ' AL: Master of Science', 813.0: ' AL: Long term specialization', 814.0: ' AL: Doctoral Degree', 877.0: ' AL: not applicable', 888.0: ' AL: don’t know (spontaneous)', 899.0: ' AL: no answer (spontaneous)', 3101.0: ' AZ: None', 3102.0: ' AZ: Primary education (1-4 years)', 3103.0: ' AZ: Incomplete general secondary (5-8 grades)', 3104.0: ' AZ: General secondary education (9 grades)', 3105.0: ' AZ: Complete secondary education (11 grades)', 3106.0: ' AZ: Primary vocational-professional education (Vocational school/lyceum) (1-3 years)', 3107.0: ' AZ: Secondary special education (Technical school/College) (2-4 years)', 3108.0: ' AZ: Bachelor degree (4 years)', 3109.0: ' AZ: Master degree (2 years)', 3110.0: ' AZ: Science degree (former Aspirantura/PhD): Doctor of Philosophy, Doctor of Science', 3188.0: ' AZ: don’t know (spontaneous)', 3199.0: ' AZ: no answer (spontaneous)', 4001.0: 'AT: Not completed', 4002.0: 'AT: Completed primary education', 4003.0: 'AT: Completed Lower Secundary Education, New Secundary School', 4004.0: 'AT: Completed Polytechnic School, or one-year Middle School', 4005.0: 'AT: Completed Academic Secondary School (AHS), elementary level', 4006.0: 'AT: Completed apprenticeship (final apprenticeship examination)', 4007.0: 'AT: Completed Vocational Middle School (at least 2 years, e.g. commercial school, professional school)', 4008.0: 'AT: Completed Higher School Certificate (matriculation, general qualification for university entrance)', 4009.0: 'AT: Completed degree in health or nursing care', 4010.0: 'AT: Completed Higher School Certificate in Vocational Higher School', 4011.0: 'AT: Completed advanced vocational training', 4012.0: 'AT: Completed pedagoical/educational/medical degree', 4013.0: "AT: Completed Bachelor's degree at a university of applied sciences", 4014.0: "AT: Completed Bachelor's degree at a university", 4015.0: "AT: Completed Master's degree at a university of applied sciences", 4016.0: "AT: Completed Master's degree at a university of applied sciences", 4017.0: 'AT: Completed post-graduate university courses (e.g. MBA)', 4018.0: 'AT: Completed doctorate/PhD', 4019.0: 'AT: other', 5101.0: 'AM: Never went to school', 5102.0: 'AM: Less than 4 years of education', 5103.0: 'AM: 4 years of primary education', 5104.0: 'AM: Certificate of lower secondary/ basic secondary education, 9 years', 5105.0: 'AM: Certificate of initial vocational education after lower /basic education', 5106.0: 'AM: Advanced vocational education after lower /basic education', 5107.0: 'AM: Certificate of upper secondary education, 12 years', 5108.0: 'AM: Certificate of initial vocational training after upper secondary education', 5109.0: 'AM: Advanced vocational education after upper secondary education', 5110.0: 'AM: Bachelor degree 4 years', 5111.0: 'AM: Specialist degree', 5112.0: 'AM: Master degree 2 years', 5113.0: 'AM: Phd and habilitation', 7001.0: 'BA: No school (up to three grades of elementary school)', 7002.0: 'BA: Unfinished elementary school (4 to 7 grades)', 7003.0: 'BA: Completed elementary school', 7004.0: 'BA: Secondary vocational school up to 1-2 years', 7005.0: 'BA: Secondary vocational school up to 3 years', 7006.0: 'BA: Technical and related secondary vocational schools up to 4 years or more', 7007.0: 'BA: High school', 7008.0: 'BA: Professional study up to 2-3 years', 7009.0: "BA: Undergraduate university study - for the academic title 'baccalaureus' (3-4 years); Art academy (undergraduate stud", 7010.0: 'BA: Specialist graduate professional study (4-5 years); Specialist in the profession', 7011.0: 'BA: Graduate university study (4-6 years) - old program', 7012.0: "BA: Master's degree (5 years); Art academy (graduate study)", 7013.0: "BA: Master's degree of Science or completed postgraduate specialist studies", 7014.0: 'BA: Postgraduate Doctorate of Science', 10001.0: 'BG: Incomplete primary education', 10002.0: 'BG: Primary education', 10003.0: 'BG: Basic education', 10004.0: 'BG: Secondary general education', 10005.0: 'BG: Secondary special education Language Schools, Mathematics and Natural Science', 10006.0: 'BG: Secondary special education Arts and Sports', 10007.0: 'BG: Secondary vocational education', 10008.0: 'BG: Semi-higher education', 10009.0: 'BG: College - Professional bachelor', 10010.0: 'BG: Higher education - Bachelor', 10011.0: 'BG: Higher education - Master', 10012.0: 'BG: Doctor of science', 11201.0: 'BY: No education', 11202.0: 'BY: Primary school', 11203.0: 'BY: Certificate of lower secondary general education', 11204.0: 'BY: Certificate of upper general secondary education', 11205.0: 'BY: 1-2 year vocational education after lower secondary education', 11206.0: 'BY: Vocational training with upper secondary general training in parallel', 11207.0: 'BY: Certificate of upper vocational education with upper secondary general education', 11208.0: "BY: Bachelor's Degree", 11209.0: "BY: Higher education, Specialist's Diploma", 11210.0: "BY: Master's degree", 11211.0: 'BY: PhD', 11212.0: 'BY: Habilitation', 19101.0: 'HR: No school (up to three grades of elementary school)', 19102.0: 'HR: Unfinished elementary school (4 to 7 grades)', 19103.0: 'HR: Completed elementary school', 19104.0: 'HR: Secondary vocational school up to 1-2 years', 19105.0: 'HR: Secondary vocational school up to 3 years', 19106.0: 'HR: Technical and related secondary vocational schools up to 4 years or more', 19107.0: 'HR: High school', 19108.0: 'HR: Professional study up to 2-3 years', 19109.0: "HR: Undergraduate university study; for academic title 'baccalaureus' (3-4 years); Art academy (undergraduate study)", 19110.0: 'HR: Specialist graduate professional study (4-5 years); Specialist in the profession', 19111.0: 'HR: Graduate university study (4-6 years) - old program', 19112.0: "HR: Master's degree (5 years); Art academy (graduate study)", 19113.0: "HR: Master's degree of Science or completed postgraduate specialist studies", 19114.0: 'HR: Postgraduate Doctorate of Science', 20301.0: 'CZ Incomplete elementary education, unfinished 1st level (less than 5 grades/years)', 20302.0: 'CZ: Incomplete basic education (5+ years of schooling, only primary school completed, SZŠ, ZZŠ, general school)', 20303.0: 'CZ: Basic education (bougeois school)', 20304.0: 'CZ: Secondary education with an apprenticeship certificate, Secondary education without school-leaving examination', 20305.0: 'CZ: Secondary education without leaving examination followed by further studies with final exam (teaching, retraining)', 20306.0: 'CZ: Education with GCSE, full secondary vocational education with GCSE', 20307.0: 'CZ: Secondary educ. with school-leaving exam followed by studies with final exam (post-gr., follow-up courses etc)', 20308.0: 'CZ: Secondary general education with school-leaving examination (grammar school)', 20309.0: 'CZ: Post-secondary education with diploma: Higher vocational school (DiS), 5th and 6th year of conservatory (discharge)', 20310.0: "CZ: Bachelor's degree", 20311.0: "CZ: Master's degree education (Mgr., Ing., Ing., MUDr., MDDr., MVDr., …)", 20312.0: 'CZ: Doctoral studies, postgraduate education (Ph.D., Th.D., CSc, ...)', 20801.0: ' DK: municipal primary and lower secondary school: 5th grade or lower', 20802.0: ' DK: municipal primary and lower secondary school: 6th grade to 8th grade', 20803.0: ' DK: municipal primary and lower secondary school: 9th grade to 10th grade', 20804.0: " DK: '(Upper) Secondary Education: Gymnasium, Higher Preparatory Examination (HF), Higher Commercial Examination Program", 20805.0: ' DK: Semiskilled worker courses and short vocational education (less than 2 years)', 20806.0: ' DK: Vocational and technical (upper) secondary education', 20807.0: ' DK: Short-cycle higher non-university programmes (2-3 years)', 20808.0: ' DK: medium-cycle university and non-university programmes, proffesional bachelor degree (3-4 years)', 20809.0: ' DK: long-cycle university programmes, 1 part - university bachelor degree', 20810.0: ' DK: long-cycle university programmes, 2. part Kandidat degree (Masters) (5-6 years)', 20811.0: ' DK: Licentiat (closed research education program)', 20812.0: ' DK: Research Education, PhD., Doctor', 20813.0: ' DK: other', 23301.0: 'EE: Without primary education (less than 4 grades)', 23302.0: 'EE: Completed primary education (4-6 grades)', 23303.0: 'EE: Vocational education without completed primary education', 23304.0: 'EE: Completed basic education (7-9 grades)', 23305.0: 'EE: Vocational secondary education after basic education (training programme under 2 years)', 23306.0: 'EE: Completed secondary education', 23307.0: 'EE: Vocational secondary education after basic education (training programme 2 years or more)', 23308.0: 'EE: Vocational secondary education with secondary education or vocational secondary education (tehnical education) afte', 23309.0: 'EE: Vocational-secondary education (tehnical education) after secondary education', 23310.0: 'EE: Vocational university after secondary education diploma (up to 2 years trainings, but not Bachelor of Arts)', 23311.0: 'EE: Higher vocational education diploma or Bachelor of Arts degree (3-4 years of trainings)', 23312.0: 'EE: University Bachelor of Arts degree (3-4 years of trainings', 23313.0: 'EE: Master of Arts from Vocational university', 23314.0: 'EE: Master of Arts\xa0(3+2, 4+2 or by 5+4 system, incl. integrated BA and MA), higher education started before 1992 (speci', 23315.0: "EE: Doctor's degree", 24601.0: ' FI: Less than primary school (grades 1-6) or less than comprehensive school', 24602.0: " FI: Grades 1-6 of comprehensive school, or primary school or people's school", 24603.0: ' FI: Grades 7-9 of comprehensive school, or middle school', 24604.0: ' FI: General upper secondary school, matriculation examination', 24605.0: ' FI: Vocational upper secondary qualification, further vocational qualification', 24606.0: ' FI: Both matriculation examination and vocational qualification', 24607.0: ' FI: Specialist vocational qualification', 24608.0: ' FI: Post-secondary level vocational qualification (old)', 24609.0: " FI: Bachelors' degree in universities of applied sciences (polytechnic)", 24610.0: " FI: Bachelors' degree in university", 24611.0: " FI: Master's degree in universities of applied sciences (polytechnic)", 24612.0: " FI: Master's degree in university", 24613.0: ' FI: Licentiate degree', 24614.0: ' FI: Doctoral degree', 24615.0: ' FI: other', 25001.0: ' FR: no schooling or not completed primary education', 25002.0: " FR: Completed primary education, without the final certificate (Certificat d'études primaires=an old qualification off", 25003.0: " FR: Completed primary education, with the Certificat d'études primaires", 25004.0: " FR: Secondary education 1st cycle, without the final certificate (Brevet élémentaire, Brevet d'étude du premier cycl", 25005.0: " FR: Secondary education 1st cycle, with the final certificate (Brevet élémentaire, Brevet d'étude du premier cycle,", 25006.0: ' FR: Secondary education 2nd cycle, without the final exam (Baccalauréat)', 25007.0: " FR: Certificate of vocational ability : Certificat d'Aptitude Professionnelle (CAP) or Brevet d'Etudes Professionnelles", 25008.0: ' FR: Vocational qualification in care (home care, children care, health care)', 25009.0: ' FR: Vocational Baccalaureat', 25010.0: ' FR: Technological Baccalaureat or equivalent diploma', 25011.0: ' FR: General Baccalaureat or equivalent diploma', 25012.0: ' FR: Certificate of ability in Law, Diploma allowing admission in tertiary education', 25013.0: ' FR: Qualifications of vocational secondary education in health and care institutions (like Moniteur éducateur and equi', 25014.0: ' FR: University education, 1st cycle Diploma in general tertiary education (2 years), classes for competitive entrance', 25015.0: ' FR: Diploma in Technological Studies , Diploma of Advanced Technician', 25016.0: ' FR: Qualification in paramedical and social sectors (social assistant, etc,)', 25017.0: ' FR: Vocational Bachelor (University education, 1st graduation and apprenticeship)', 25018.0: ' FR: Bachelor (University education, 2nd cycle, 1st year and apprenticeship)', 25019.0: ' FR: Engineer Degree', 25020.0: ' FR: Vocational Master Degree', 25021.0: ' FR: Intermediate Master degree (university education, 2nd cycle, 2nd year), qualification of primary and secondary educ', 25022.0: ' FR: Research Master Degree, qualification of university education teachers', 25023.0: ' FR: Qualifications of architects, veterinaries, notaries', 25024.0: ' FR: Diplôma from selective “grandes écoles”', 25025.0: ' FR: Doctorate in medecine, odontology, pharmacy', 25026.0: ' FR: Doctorate', 26801.0: 'GE: No education', 26802.0: 'GE: Less than 6 years primary education', 26803.0: 'GE: Primary education completed, 6 years', 26804.0: 'GE: Basic/ lower general education certificate, 9 years', 26805.0: 'GE: Upper secondary general education certificate', 26806.0: 'GE: Professional diploma level 1, 2, or 3 (Vocational Education certificate, before 2010)', 26807.0: 'GE: Professional diploma level 4/5 (Secondary Vocational Educ. Certificate pre-2010, Certified Diploma 2008-2010)', 26808.0: 'GE: Interim qualification, short cycle tertiary education', 26809.0: 'GE: Bachelor degree', 26810.0: 'GE: Master degree', 26811.0: 'GE: Specialist studies', 26812.0: 'GE: Doctoral degree', 34801.0: 'HU: Never attented school; 1-3 years of primary school or equivalent', 34802.0: 'HU: 4-7 years of primary school or equivalent', 34803.0: 'HU: Completed primary school or equivalent', 34804.0: 'HU: Vocational school', 34805.0: 'HU: Vocational training after the 10. grade', 34806.0: 'HU: Completed secondary vocational education with school-leaving exam', 34807.0: 'HU: Completed secondary general education (Gymnasium) with school-leaving exam', 34808.0: 'HU: Post-secondary, mon-tertiary vocational education and training (E.g. technical school)', 34809.0: 'HU: Short-cycle tertiary level vocational education and training (VET)', 34810.0: "HU: College degree or college bachelor's degree - BA/BSc", 34811.0: "HU: University bachelor's degree - BA/BSc", 34812.0: "HU: College master's degree - MA/MSc", 34813.0: "HU: University degree or university master's degree - MA/MSc", 34814.0: 'HU: Doctoral degree', 35201.0: 'IS: Less than primary education', 35202.0: 'IS: Primary education', 35203.0: 'IS: Elementary school, 14–15 years of age', 35204.0: 'IS: National test', 35205.0: 'IS: Elementary school, 15–16 years of age (compulsory education)', 35206.0: 'IS: Elementary school, 16–17 years of age', 35207.0: 'IS: Vocational education at the lower secondary level', 35208.0: 'IS: Post-secondary non-tertiary education', 35209.0: 'IS: Other post-secondary, non-tertiary vocational education', 35210.0: 'IS: Upper secondary art education, 2+ years', 35211.0: 'IS: Certified trades, school diploma', 35212.0: 'IS: Matriculation examination', 35213.0: 'IS: Upper secondary education', 35214.0: 'IS: Master of a certified trade', 35215.0: 'IS: Tertiary general education, 2–3 years', 35216.0: 'IS: Tertiary vocational education, 2–3 years', 35217.0: 'IS: Bachelor’s or equivalent level', 35218.0: 'IS: Master’s or equivalent level', 35219.0: 'IS: Doctoral or equivalent level', 38001.0: ' IT: No title', 38002.0: ' IT: Elementary school diploma', 38003.0: ' IT: Vocational training', 38004.0: ' IT: Middle school diploma', 38005.0: ' IT: Post-obligation vocational regional qualification', 38006.0: " IT: Vocational institute diploma (2 or 3 years), including art teacher diploma, teacher's training school diploma and n", 38007.0: ' IT: High school diploma (5 years), technical or vocational institute, including art institute five years diploma', 38008.0: ' IT: High school diploma (4 or 5 years), classical, scientific, linguistic or psyco-pedagogical, musical lyceum, includi', 38009.0: ' IT: Post-(high school) diploma specialization, post-(high school) diploma vocational regional qualification, certificat', 38010.0: ' IT: Former system academic diploma (2 or 3 years), including higher institute of physical education, social service and', 38011.0: ' IT: Tertiary education not academic diploma: Music Conservatory, Academy of Fine Arts and similar', 38012.0: ' IT: Three years or first level degree (bachelor)', 38013.0: ' IT: First level specialization degree', 38014.0: " IT: Former system degree, master's degree, single cycle degree", 38015.0: ' IT: Second level specialization degree', 38016.0: ' IT: Post-graduate specialization (1 or 2 years)', 38017.0: ' IT: Post-graduate specialization (3 or 4 years), including medical specialization', 38018.0: ' IT: Doctoral degree', 42801.0: ' LV: Has not attended school', 42802.0: ' LV: Incompete primary education ( 6 grades)', 42803.0: ' LV: General primary education', 42804.0: ' LV: Basic vocational education', 42805.0: ' LV: Vocational education (without secondary) (1 year)', 42806.0: ' LV: Vocational education (without secondary) (3 years)', 42807.0: ' LV: General secondary education', 42808.0: ' LV: Vocational secondary education', 42809.0: ' LV: Vocational (not higher) education after general secondary education', 42810.0: ' LV: 1st level professional/ vocational higher education (college)', 42811.0: " LV: Bachelor's degree (professional)", 42812.0: " LV: Bachelor's degree (academic)", 42813.0: " LV: Master's degree (professional)", 42814.0: " LV: Master's degree (academic)", 42815.0: ' LV: Higher education obtained during the Soviet era', 42816.0: ' LV: Doctoral degree', 44000.0: ' LT: Less than primary', 44001.0: ' LT: Primary', 44002.0: ' LT: Lower general vocational', 44003.0: ' LT: General/ lower secondary', 44004.0: ' LT: General vocational', 44005.0: ' LT: Lower vocational after general', 44006.0: ' LT: Vocational after general', 44007.0: ' LT: Secondary', 44008.0: ' LT: Vocational with secondary', 44009.0: ' LT: Vocational after secondary', 44010.0: ' LT: Upper general and secondary vocational', 44011.0: ' LT: Upper secondary vocational', 44012.0: ' LT: Higher non university', 44013.0: ' LT: Higher university education (BA)', 44014.0: ' LT: Higher university education (MA)', 44015.0: ' LT: Higher university integrated education (I and II stages - 6 years)', 44016.0: ' LT: Doctoral studies and diploma', 49901.0: ' ME: No education', 49902.0: ' ME: Completed 4 years of primary education', 49903.0: ' ME: Completed lower secondary education', 49904.0: ' ME: 1-2 years vocational training', 49905.0: ' ME: Completed high school lasting three years', 49906.0: ' ME: Certificate of upper secondary vocational education (4 years)', 49907.0: ' ME: Secondary general (grammar schools, 4 years)', 49908.0: ' ME: Vocational exam', 49909.0: ' ME: Primary level diploma (after two or three years of bachelor level pre-Bologne reform)', 49910.0: ' ME: Completed two years of higher education that provides basic certificate but not entrance to MA studies', 49911.0: ' ME: Vocational studies (in duration of three years)', 49912.0: ' ME: Bachelor studies in duration of 3 years', 49913.0: ' ME: Specialization studies or four year studies in pre-Bologne system', 49914.0: ' ME: Master studies in duration of one year; Integrated studies (five year studies); Specialization studies', 49915.0: ' ME: Magister studies, Studies of medicine (in duration of 6 years, in old system), Specialisation', 49916.0: ' ME: PhD', 52801.0: 'NL: No completed primary education', 52802.0: 'NL: Primary education', 52803.0: "NL: Pre-vocational program ('vmbo level 1, 2 or 3' or 'lbo') or comparable", 52804.0: "NL: Preparatory secondary vocational education ('mavo' (previous 'vmbo') or 'vmbo level 4') or comparable", 52805.0: 'NL: Intermediate vocational educational, level 1 (duration 2 year)', 52806.0: "NL: Senior general secondary school ('havo') or comparable", 52807.0: "NL: Pre-university education ('vwo' or gymnasium) or comparable", 52808.0: "NL: kmbo', 'meao', 'mts' or comparable (duration 2-3 years)", 52809.0: 'NL: Intermediate vocational education , levels 2 and 3 (duration 2-3 years)', 52810.0: 'NL: Intermediate vocational education , level 4 (duration 4 years)', 52811.0: "NL: Intermediate vocational education plus (for people that completed 'havo')", 52812.0: 'NL: First year of university, Bachelor (propaedeutics)', 52813.0: 'NL: Shorter higher vocational education, shorter University of Applied Sciences (duration 2 or 3 years)', 52814.0: "NL: Higher vocational education / University of Applied Sciences, Bachelor's degree or comparable", 52815.0: "NL: University, Bachelor's degree", 52816.0: "NL: Higher vocational education / University of Applied Sciences, Master's degree or comparable", 52817.0: "NL: University, Master's degree or comparable", 52818.0: 'NL: University, doctoral degree (promotion)', 57801.0: ' NO: No education and/or preschool education', 57802.0: ' NO: Primary school (Elementary school. First part of compulsory education)\xa0', 57803.0: ' NO: Lower secondary school (Elementary school. Second part of compulsory education)', 57804.0: ' NO: Upper secondary school basic (including vk1, folkehøgskole, realskole)', 57805.0: ' NO: Upper secondary, complete graduation (including vk2, vk3, gymnas)', 57806.0: ' NO: Supplementary program for general university admissions certification', 57807.0: ' NO: University and university college, lower degree (including B.A., cand.mag)', 57808.0: ' NO: University and university college, higher degree (including M.A.)', 57809.0: ' NO: Scientific degree (Doctoral degree, Ph.D.)', 57810.0: ' NO: Primary education', 57824.0: ' NO: Lower secondary education - general', 57825.0: ' NO: Lower secondary education - vocational', 57834.0: ' NO: Upper secondary education - general', 57835.0: ' NO: Upper secondary education - vocational', 57844.0: ' NO: Post-secondary non-tertiary education - general', 57845.0: ' NO: Post-secondary non-tertiary education - vocational', 57854.0: ' NO: Short-cycle tertiary education - general', 57855.0: ' NO: Short-cycle tertiary education - vocational', 57856.0: ' NO: Short-cycle tertiary education - orientation unspecified', 57864.0: ' NO: Bachelor’s or equivalent level - academic', 57865.0: ' NO: Bachelor’s or equivalent level - professional', 57866.0: ' NO: Bachelor’s or equivalent level - orientation unspecified', 57874.0: ' NO: Master’s or equivalent level - academic', 57875.0: ' NO: Master’s or equivalent level - professional', 57876.0: ' NO: Master’s or equivalent level - orientation unspecified', 57884.0: ' NO: Doctoral or equivalent level - academic', 57885.0: ' NO: Doctoral or equivalent level - professional', 57886.0: ' NO: Doctoral or equivalent level - orientation unspecified', 61601.0: 'PL: primary education not completed', 61602.0: 'PL: primary education (6 grades or 4 grades)', 61603.0: 'PL: primary education', 61604.0: 'PL: lower secondary', 61605.0: 'PL: basic vocational education in agriculture', 61606.0: 'PL: basic vocational education', 61607.0: 'PL: basic vocational education', 61608.0: 'PL: upper secondary education without A-level diploma', 61609.0: 'PL: upper secondary education with A-level diploma', 61610.0: 'PL: upper secondary vocational or technical education', 61611.0: 'PL: upper secondary vocational or technical education with A-level diploma', 61612.0: 'PL: diploma of technician or post secondary education', 61613.0: "PL: post secondary education in teachers' college", 61614.0: "PL: BA or engineer's diploma", 61615.0: "PL: MA or doctor's diploma", 61616.0: "PL: PhD, assistant professor, professor's title", 61617.0: 'PL: other', 62001.0: ' PT: None', 62002.0: ' PT: Basic Level 1 (until 4th grade, primary education)', 62003.0: ' PT: Basic level 2 (until 6th grade, 1st cycle of former comercial or industrial high school)', 62004.0: ' PT: Type 1 education and formation courses. Attribution of level 1 professional qualification diploma', 62005.0: ' PT: Basic Level 3 (Certificate of conclusion of one of the following grades: 9th grade; former 5th grade of high school', 62006.0: ' PT: Type 2 education and formation courses. Attribution of level 2 professional qualification diploma', 62007.0: ' PT: Types 3 and 4 education and formation courses. Attribution of level 2 professional qualification diploma', 62008.0: ' PT: Secondary Level - Scientific-Humanistic studies (certificate of conclusion of one of the following grades: 12th gra', 62009.0: ' PT: Secondary Level -technologic and artistic studies (visual and audiovisual arts, dance and music), professional cour', 62010.0: ' PT: Technologic specialisation course. Attribution of Thecnologic specialisation diploma', 62011.0: ' PT: Polytechnic superior level:3 years bacalaureat (primary school teachers, social service, agricultural manager); f', 62012.0: ' PT: Polytechnic superior level:3-4 years graduation', 62013.0: ' PT: University superior level: 3-4 years graduation: 4 years two-stage graduation', 62014.0: ' PT: Post-graduation: post-graduation specialisation without degree atribution, MBA', 62015.0: ' PT: University superior level: gradution with more than 4 years: 5 years two-stage graduation', 62016.0: ' PT: Master degree (inc. integrated master)', 62017.0: ' PT: PhD', 64200.0: ' RO: No school at all', 64201.0: ' RO: Unfinished primary school', 64202.0: ' RO: Finished primary school', 64203.0: ' RO: Unfinished gymnasia', 64204.0: ' RO: Graduated gymnasia', 64205.0: ' RO: Apprentice (complementary) school', 64206.0: ' RO: School of Arts and Crafts', 64207.0: ' RO: School of Arts and Crafts - year of completion', 64208.0: ' RO: Professional school, less than 2 years', 64209.0: ' RO: Professional school, 2-4 years', 64210.0: ' RO: Unfinished High School', 64211.0: ' RO: Completed Theoretical High School (Baccalaureate Diploma)', 64212.0: ' RO: Completed Technological / Industrial High School (Baccalaureate Diploma)', 64213.0: ' RO: Foreman school without Baccalaureate', 64214.0: ' RO: Foreman school with Baccalaureate', 64215.0: ' RO: Post-highschool without Baccalaureate', 64216.0: ' RO: Post-highschool with Baccalaureate', 64217.0: ' RO: Unfinished university studies', 64218.0: ' RO: Sub-engineers or College', 64219.0: ' RO: BA (graduated university): 3 years', 64220.0: ' RO: BA (graduated university): 4 years', 64221.0: ' RO: BA (graduated university): 5 years', 64222.0: ' RO: BA (graduated university): 6 years', 64223.0: ' RO: Master', 64224.0: ' RO: PhD', 64301.0: 'RU: Did not study in school or completed 1-2 grades on school only (incomplete primary school)', 64302.0: 'RU: Graduated 3-7 grades of secondary school without attestat on basic general education', 64303.0: 'RU: Attestat of basic general educ.–complete 7 grade (pre 1958); 8 gr.(1960-1980); 9 gr.(modern system); no profes. ed', 64304.0: 'RU: Completed general secondary educ. (10 grade by old system,11 grade by new), got attestat; no professional educ.', 64305.0: 'RU: Got primary professional educ.–grad. PTU,FSU,FSO, profes.-techn. lyceum; no attestat of compl. sec. gen. educ.(<2y', 64306.0: 'RU: Got primary professional educ.– grad. PTU, professional-techn. lyceum, with attestat of completed sec. educ.(1-3 y', 64307.0: 'RU: Got secondary professional education – graduated technikum, uchilishe, college (2-4 years of study)', 64308.0: 'RU: Got diploma of bachelor in college after 4 years of education on two-stage new education system', 64309.0: 'RU: Got diploma of master in college after additional 2 years of education on two-stage new education system', 64310.0: 'RU: Completed high education by 5-6-years system (diploma of specialist)', 64311.0: 'RU: Scientific degree (candidate, doctor of sciences)', 68801.0: ' RS: No education (under 3rd grade)', 68802.0: ' RS: Primary education lower cycle (4th – 7th grade)', 68803.0: ' RS: Primary education upper cycle (8th grade)', 68804.0: ' RS: Secondary vocational 3 yrs', 68805.0: ' RS: Secondary vocational 4 yrs', 68806.0: ' RS: Secondary general (grammar schools)', 68807.0: ' RS: 1-2 years vocational training', 68808.0: ' RS: Higher schools', 68809.0: ' RS: University: Graduate studies', 68810.0: ' RS: Postgraduate studies: Specialist studies', 68811.0: ' RS: Postgraduate studies: Magister studies (outdated)', 68812.0: ' RS: Postgraduate studies: Master studies', 68813.0: ' RS: PhD studies/ Doctor’s Degree', 70301.0: 'SK: Not completed first stage of elementary school (not completed primary level of education)', 70302.0: 'SK: Not completed second stage of elementary school (not completed lower secondary level of education)', 70303.0: 'SK: Completed lower secondary educ.(completed 8/9 y. of elementary school or courses to complete lower secondary educ.)', 70304.0: ' SK: Practical (girl) school, two-years training programmes, requalification courses without vocational certificate', 70305.0: 'SK: secondary vocational school or sec. voc. training institution or sec. voc. school without leaving exam (no maturita)', 70306.0: 'SK: Secondary vocational school with leaving exam (with maturita), secondary vocat. training institution (with maturita)', 70307.0: 'SK: Eight year grammar school - 4 year grammar school', 70308.0: 'SK: Complementary study', 70309.0: "SK: Complementary 'postgraduate' pedagogical study", 70310.0: "SK: 'postgraduate' vocational study", 70311.0: 'SK: Postgraduate specialised study with leaving certificate, Dance conservatory', 70312.0: 'SK: Secondary vocational schoool with leaving examination (maturita) - six year study', 70313.0: 'SK: Bachelors degree', 70314.0: "SK: Master degree (engineering study, 'small' doctorate)", 70315.0: 'SK: postgraduate pedagogical study, teaching certificate for university graduates', 70316.0: 'SK: PhD study, doctoral degree', 70501.0: 'SI: Without school education', 70502.0: 'SI: Incomplete primary education', 70503.0: 'SI: Primary education', 70504.0: 'SI: Lower or upper secondary vocational education', 70505.0: 'SI: Secondary vocational education', 70506.0: 'SI: Secondary general education', 70507.0: 'SI: Higher vocational education, post-secondary education (2 years)', 70508.0: 'SI: Higher vocational education - 1. Bologna level.', 70509.0: 'SI: Higher education university education', 70510.0: "SI: Bologna master's degree", 70511.0: 'SI: Specialization', 70512.0: "SI: Master's degree", 70513.0: 'SI: phD', 72401.0: 'ES: No studies', 72402.0: 'ES: Not completed primary studies', 72403.0: 'ES: Old Primary School (Before 1970)', 72404.0: 'ES: Up to the 5th course of EGB (Basic General Education). From 70s to 90s', 72405.0: 'ES: Up to the 6th course of Primary School', 72406.0: 'ES: Primary School in Music and Dance', 72407.0: 'ES: Elementary High School (Before 1970)', 72408.0: 'ES: Up to the 8th course of EGB (Basic General Education). From 70s to 90s', 72409.0: 'ES: Compulsory High School', 72410.0: 'ES: High School (From 70s to 90s)', 72411.0: 'ES: Pre-University Studies (before 90s)', 72412.0: 'ES: Not compulsory High School', 72413.0: 'ES: Vocational training (initiation)', 72414.0: 'ES: Vocational training (initiation) (old Law)', 72415.0: 'ES: Vocational training (first grade) (old Law)', 72416.0: 'ES: Vocational training (first grade)', 72417.0: 'ES: Vocational training (medium grade, technical in various professions such as administrative or mechanic )', 72418.0: 'ES: Vocational training (medium grade, arts and design)', 72419.0: 'ES: Vocational training (medium grade, music and dance)', 72420.0: 'ES: Vocational training (high grade, industrial) (old Law)', 72421.0: 'ES: Vocational training (high grade, general) (old Law)', 72422.0: 'ES: Vocational training (high grade, general)', 72423.0: 'ES: Vocational training (high grade in arts)', 72424.0: 'ES: Medium Tertiary studies (nursery, school teacher, social worker)', 72425.0: 'ES: Medium Tertiary studies ( building engineer, industrial or business expert)', 72426.0: 'ES: Long tertiary studies', 72427.0: 'ES: Phd', 72428.0: 'ES: Other (specify)', 75201.0: ' SE: not completed ISCED level 1', 75202.0: ' SE: ISCED 1, completed primary education', 75203.0: ' SE: Qualification from general ISCED 2A programmes, access to ISCED 3A general or all 3', 75204.0: ' SE: Old version of vocational high school', 75205.0: ' SE: Old and current version of high school education that gives access to higher education', 75206.0: ' SE: Old version of mainly vocational high school with limited access to higher education', 75207.0: ' SE: Vocational high school', 75208.0: ' SE: Technical high school, formerly 4 years at high school, currently 3 years at high school and 4th year at university', 75209.0: ' SE: Degree from one year of studies at university college.', 75210.0: ' SE: Vocational degree, qualification obtained after high school graduation, from vocational training institutes', 75211.0: ' SE: Vocational degree, obtained at university or university college, e.g. old professional nursing programmes', 75212.0: ' SE: Vocational degree, obtained after high school graduation, longer programme 2-3 years from vocational training insti', 75213.0: " SE: Bachelor's degree or professional degree from a university college", 75214.0: " SE: Bachelor's degree or professional degree from a university", 75215.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75216.0: ' SE: Masters degree from university college', 75217.0: ' SE: One year masters degree or old one semester master degree, equivalent of at least 4 years or more of studies at uni', 75218.0: ' SE: Masters degree from university', 75219.0: ' SE: Research degree, licantiate degree, a sort of half-way doctoral degree, old degree that is not often used', 75220.0: ' SE: Research degree, phd', 75601.0: 'CH: Incompleted primary school', 75602.0: 'CH: Primary school', 75603.0: 'CH: Secondary education (first stage)', 75604.0: 'CH: Additional year of secondary education, preparation for vocational training', 75605.0: 'CH: General training school (2-3 years)', 75606.0: 'CH: Baccalaureate preparing for university', 75607.0: 'CH: Baccalaureate for adults or apprenticeship after Baccalaureate', 75608.0: 'CH: Diploma for teaching in primary school or preprimary school', 75609.0: 'CH: Vocational baccalaureate', 75610.0: 'CH: Vocational baccalaureate for adults', 75611.0: 'CH: Elementary vocational training (enterprise and school, 1-2 year)', 75612.0: 'CH: Apprenticeship (vocational training, dual system, 3-4 years)', 75613.0: 'CH: Second vocational training (or apprenticeship as second education)', 75614.0: 'CH: Advanced vocational qualification (specialization exam, federal certif. or dipl. of advanced vocational training)', 75615.0: 'CH: Higher vocational training (high school diploma - technical, administration, health, social work, applied arts)', 75616.0: 'CH:Higher vocational training (diploma of some specific high schools withrecognition of tertiary level)', 75617.0: 'CH: University of applied science and pedagogical university (Bachelor)', 75618.0: 'CH: University of applied science and pedagogical university (Master)', 75619.0: 'CH: University diploma (intermediary level)', 75620.0: 'CH: University diploma and post-graduate (including technical) (Bachelor and short university degree)', 75621.0: 'CH: University diploma and post-graduate (including technical) (degree requiring more than 4 years)', 75622.0: 'CH: University diploma and post-graduate (including technical) (Master)', 75623.0: 'CH: Doctoral degree', 75624.0: 'CH: other', 80400.0: ' UA: Incomplete primary secondary education (less than 4 classes)', 80401.0: ' UA: Primary secondary education (1- 3(4) classes)', 80402.0: ' UA: Basic secondary education (certificate for 8(9) class)', 80403.0: ' UA: Technical-vocational education based on basic secondary education (no certificate of complete secondary education)', 80404.0: ' UA: Technical-vocational education based on of basic general secondary (certificate of general secondary education and', 80405.0: ' UA: Complete secondary education (certificate of general secondary education of 10(11) classes)', 80406.0: ' UA: Additional training training on the basis of general secondary education (vocational and general educational course', 80407.0: ' UA: Technical-vocational education on the basis of general secondary education', 80408.0: ' UA: Professional pre-higher education (diploma of junior specialist)', 80409.0: " UA: Basic higher education (bachelor's degree)", 80410.0: ' UA: Full higher education (specialist)', 80411.0: ' UA: Full higher education (Master or equivalent)', 80412.0: ' UA: Academic degree (candidate, doctor, PhD)', 80701.0: 'MK: Without education', 80702.0: 'MK: Completed primary education (4 years of primary school)', 80703.0: 'MK: Completed primary and lower secondary education', 80704.0: 'MK: Completed upper secondary education, vocational training - 2 years', 80705.0: 'MK: Completed upper secondary education, vocational training - 3 years', 80706.0: 'MK: Completed upper secondary education, vocational training - 4 years', 80707.0: 'MK: Completed upper secondary education, general (high school) - 4 years', 80708.0: 'MK: Completed further education - short cycle university education - 2 years', 80709.0: 'MK: Bachelor degree - 3 years', 80710.0: 'MK: Bachelor degree - 4 years', 80711.0: 'MK: Completed postgraduate studies, specialist studies - 1 year', 80712.0: 'MK: Completed postgraduate studies, specialist studies - 2 years', 80713.0: 'MK: Completed postgraduate studies, master studies - 1 year', 80714.0: 'MK: Completed postgraduate studies, master studies - 2 years', 80715.0: 'MK: Doctoral degree, completed doctorate - 5 years', 80716.0: 'MK: Doctoral degree, completed doctorate (PhD studies) - 3 years', 80788.0: 'MK: don’t know (spontaneous)', 80799.0: 'MK: no answer (spontaneous)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52602 | 516 |
v263_cs_DE1 | 429 | v263_cs_DE1 | numeric | DE COUNTRY-SPECIFIC: educational level mother (Q100), SCHOOL | {-10.0: 'multiple answers Mail', 27601.0: 'Primary school not completed', 27602.0: 'Primary school completed, secondary school not (yet) completed', 27603.0: 'Lower secondary schools (no access to general) or polytechnic secondary school (8th or 9th grade)', 27604.0: 'Lower secondary schools (access to general) or polytechnic secondary school (10th grade)', 27605.0: 'University of Applied Science entrance qualification (specialised vocational high schools etc.)', 27606.0: 'Grammar school (University entrance qualification)', 27607.0: 'Other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1918 | 7 |
v263_cs_DE2 | 430 | v263_cs_DE2 | numeric | DE COUNTRY-SPECIFIC: educational level mother (Q100), STUDIES | {-10.0: 'multiple answers Mail', 27601.0: 'No degree', 27602.0: 'Preliminary examination, preliminary diploma', 27603.0: 'College of public administration or University of Applied Sciences (diploma)', 27604.0: 'Vocational academy (diploma)', 27605.0: 'College of public administration or University of Applied Sciences (Bachelor)', 27606.0: 'Vocational academy (Bachelor)', 27607.0: 'University (Bachelor)', 27608.0: 'College of public administration or University of Applied Sciences (Master)', 27609.0: 'Vocational academy (Master)', 27610.0: 'University (diploma, Magister, state examaniation)', 27611.0: 'University (Master or postgraduate studies)', 27612.0: 'Doctoral studies', 27613.0: 'other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1865 | 13 |
v263_cs_DE3 | 431 | v263_cs_DE3 | numeric | DE COUNTRY-SPECIFIC: educational level mother (Q100), VOCATIONAL | {-10.0: 'multiple answers Mail', 27601.0: 'No degree', 27602.0: 'Pre-vocational training, qualification of short health sector programmes', 27603.0: 'Vocational training with diploma, but no Dual System (second cycle)', 27604.0: 'Medical assistant, nurse (qualification of health sector schools (2 and 3 years)', 27605.0: 'Qualification of civil servants for the medium level', 27606.0: 'Qualification in the Dual System (second cycle), industrial or agricultural sector', 27607.0: 'Qualification in the Dual System (second cycle), sales sector', 27608.0: 'Qualification of a vocational full-time school', 27609.0: 'Occupational qualification (second cycle) for students with occupational qualification or with university entrace qualif', 27610.0: "Master craftsmen's or Technician's qualification (or equal)", 27611.0: 'other, please specify: (write in)'} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1781 | 11 |
v263_cs_GB1 | 432 | v263_cs_GB1 | numeric | GB COUNTRY-SPECIFIC:educational level mother: Up to 2 or more A-levels or equivalent (Q100a) | {82601.0: '2 or more A-levels or equivalent', 82602.0: ' GNVQ or GSVQ Intermediate', 82603.0: ' Vocational GCSE or equivalent', 82604.0: ' 5 or more GCSEs A*-C or equivalent', 82605.0: ' 1-4 GCSEs A*-C or equivalent', 82606.0: ' Skills for life', 82607.0: ' None of these'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1504 | 7 |
v263_cs_GB2 | 433 | v263_cs_GB2 | numeric | GB COUNTRY-SPECIFIC:educational level mother: Up to Ph.D or equivalent (Q100a) | {82601.0: ' Ph.D, D.Phil or equivalent', 82602.0: ' Masters Degree, M.Phil, Post-Graduate Diplomas and Certificates', 82603.0: ' 5 year University/CNAA first Degree (MB, BDS, BV etc)', 82604.0: ' 3-4 year University/CNAA first Degree (BA, BSc., BEd., BEng. Etc)', 82605.0: ' Nursing certificate, Teacher training, HE Diploma, Edexcel/BTEC/BEC/TEC/HND, City and Guilds, NVQ/SVQ - Leve', 82606.0: ' Foundation Degree (FdA, FdSc etc)', 82607.0: ' Edexcel/BTEC/BEC/TEC - Higher National Certificate (HNC) or equivalent', 82608.0: ' HE Access', 82609.0: ' Vocational A-level (AVCE), GCE Applied A-level, NVQ/SVQ Level 3, GNVQ/GSVQ Advanced, Edexcel/BTEC/BEC/TEC (G', 82610.0: ' (Modern) Apprenticeship, Advanced (Modern) Apprenticeship, SVQ/NVQ/Key Skills Level 1 and 2, City and Guild', 82611.0: ' None of these', 82612.0: ' Any other qualifications (specify)'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 1508 | 11 |
v264 | 434 | v264 | numeric | at age 14, was father employed (Q101) | {1.0: 'yes employed', 2.0: 'yes self employed', 3.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55049 | 3 |
v265 | 435 | v265 | numeric | at age 14, was mother employed (Q102) | {1.0: 'yes employed', 2.0: 'yes self employed', 3.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56942 | 3 |
v266 | 436 | v266 | numeric | at age 14, occupational group of your parent (main earner) (Q103) | {1.0: 'professional and technical (for example: doctor, teacher, engineer, artist, accountant, nurse)', 2.0: 'higher administrative (for example: banker, executive in big business, high government official, union official)', 3.0: 'clerical (for example: secretary, clerk, office manager, civil servant, bookkeeper)', 4.0: 'sales (for example: sales manager, shop owner, shop assistant, insurance agent, buyer)', 5.0: 'service (for example: restaurant owner, police officer, waitress, barber, caretaker)', 6.0: 'skilled worker (for example: foreman, motor mechanic, printer, seamstress, tool and die maker, electrician)', 7.0: 'semi-skilled worker (for example: bricklayer, bus driver, cannery worker, carpenter, sheet metal worker, baker)', 8.0: 'unskilled worker (for example: laborer, porter, unskilled factory worker, cleaner)', 9.0: 'farm worker (for example: farm laborer, tractor driver)', 10.0: 'farm proprietor, farm manager'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 66.0: 'does not apply to me (spontaneous)'} | True | 55344 | 10 |
v267 | 437 | v267 | numeric | at age 14, mother liked to read books (Q104A) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56398 | 4 |
v268 | 438 | v268 | numeric | at age 14, discussed politics with mother (Q104B) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 57142 | 4 |
v269 | 439 | v269 | numeric | at age 14, mother liked to follow the news (Q104C) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56312 | 4 |
v270 | 440 | v270 | numeric | at age 14, parent(s) had problems making ends meet (Q104D) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56977 | 4 |
v271 | 441 | v271 | numeric | at age 14, father liked to read books (Q104E) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 54376 | 4 |
v272 | 442 | v272 | numeric | at age 14, discussed politics with father (Q104F) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55219 | 4 |
v273 | 443 | v273 | numeric | at age 14, father liked to follow the news (Q104G) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 54635 | 4 |
v274 | 444 | v274 | numeric | at age 14, parent(s) had problems replacing broken things (Q104H) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55792 | 4 |
v275b_N2 | 445 | v275b_N2 | nominal | region where interview was conducted: NUTS-2 code (Q105) | {'AL01': 'AL: Albania - Northern Albania', 'AL02': 'AL: Albania - Central Albania', 'AL03': 'AL: Albania - Southern Albania', 'ALZZ': 'AL: Extra-region - Extra-region', 'AM01': 'AM: Armenia - Yerevan', 'AM02': 'AM: Armenia - II Region', 'AM03': 'AM: Armenia - III Region', 'AM04': 'AM: Armenia - IV Region', 'AMZZ': 'AM: Extra-region - Extra-region', 'AT11': ' AT: OSTÖSTERREICH - Burgenland', 'AT12': ' AT: OSTÖSTERREICH - Niederösterreich', 'AT13': ' AT: OSTÖSTERREICH - Wien', 'AT21': ' AT: SÜDÖSTERREICH - Kärnten', 'AT22': ' AT: SÜDÖSTERREICH - Steiermark', 'AT31': ' AT: WESTÖSTERREICH - Oberösterreich', 'AT32': ' AT: WESTÖSTERREICH - Salzburg', 'AT33': ' AT: WESTÖSTERREICH - Tirol', 'AT34': ' AT: WESTÖSTERREICH - Vorarlberg', 'ATZZ': ' AT: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'AZ-ABS': ' AZ: Absheron', 'AZ-AGA': ' AZ: Agstafa', 'AZ-AGC': ' AZ: Agdzhebedinsky', 'AZ-AGS': ' AZ: Agdash', 'AZ-AGU': ' AZ: Agsu', 'AZ-AST': ' AZ: Astara', 'AZ-BA': ' AZ: Baku city', 'AZ-BAL': ' AZ: Belakansky', 'AZ-BAR': ' AZ: Barda', 'AZ-BEY': ' AZ: Beylagan', 'AZ-BIL': ' AZ: Bilasuvar', 'AZ-CAL': ' AZ: Jalilabad', 'AZ-DAS': ' AZ: Dashkesan', 'AZ-GA': ' AZ: Ganja city', 'AZ-GAD': ' AZ: Kedabegsky', 'AZ-GOR': ' AZ: Goranboy', 'AZ-GOY': ' AZ: Goychay', 'AZ-GYG': ' AZ: Gay-Gelsky', 'AZ-HAC': ' AZ: Hajikabul', 'AZ-IMI': ' AZ: Imishli', 'AZ-ISM': ' AZ: Ismaili', 'AZ-KUR': ' AZ: Kurdamir', 'AZ-LAN': ' AZ: Lankaran', 'AZ-LER': ' AZ: Lerik', 'AZ-MAS': ' AZ: Masalli', 'AZ-MI': ' AZ: Mingechaur city', 'AZ-NA': ' AZ: Naftalan city', 'AZ-NEF': ' AZ: Neftchala', 'AZ-OGU': ' AZ: Oguz', 'AZ-QAB': ' AZ: Gabala region', 'AZ-QAX': ' AZ: Gakh', 'AZ-QAZ': ' AZ: Gazakh', 'AZ-QBA': ' AZ: Gubinsky', 'AZ-QOB': ' AZ: Gobustan', 'AZ-QUS': ' AZ: Gusar', 'AZ-SAB': ' AZ: Sabirabad', 'AZ-SAK': ' AZ: Sheki', 'AZ-SAL': ' AZ: Salyan', 'AZ-SAT': ' AZ: Saatly', 'AZ-SBN': ' AZ: Shabran', 'AZ-SIY': ' AZ: Siyazan', 'AZ-SKR': ' AZ: Shamkir', 'AZ-SM': ' AZ: Sumgait city', 'AZ-SMI': ' AZ: Shemakha', 'AZ-SMX': ' AZ: Samukh', 'AZ-SR': ' AZ: Shirvan city', 'AZ-TOV': ' AZ: Tovuz', 'AZ-UCA': ' AZ: Ujar', 'AZ-XAC': ' AZ: Khachmaz', 'AZ-XIZ': ' AZ: Khyzy', 'AZ-YAR': ' AZ: Yardimli', 'AZ-YEV': ' AZ: Yevlakh', 'AZ-ZAQ': ' AZ: Zakatala', 'AZ-ZAR': ' AZ: Zardob', 'BABIH': 'BA: Bosnia and Herzegovina - Federation of Bosnia i Herzegovina', 'BABRC': 'BA: Bosnia and Herzegovina - Brčko DISTRICT', 'BASRP': 'BA: Bosnia and Herzegovina - Republica Srpska', 'BG31': ' BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Severozapaden', 'BG32': ' BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Severen tsentralen', 'BG33': ' BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Severoiztochen', 'BG34': ' BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Yugoiztochen', 'BG41': ' BG: YUGOZAPADNA I YUZHNA TSENTRALNA BULGARIA - Yugozapaden', 'BG42': ' BG: YUGOZAPADNA I YUZHNA TSENTRALNA BULGARIA - Yuzhen tsentralen', 'BGZZ': ' BG: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'BY01': 'BY: Belarus - Belarus - Brest region', 'BY02': 'BY: Belarus - Belarus - Vitebsk region', 'BY03': 'BY: Belarus - Belarus - Gomel region', 'BY04': 'BY: Belarus - Belarus - Grodno region', 'BY05': 'BY: Belarus - Belarus - Minsk (capital city)', 'BY06': 'BY: Belarus - Belarus - Minsk region', 'BY07': 'BY: Belarus - Belarus - Mogilev region', 'BYZZ': 'BY: Extra-regio - Extra-regio - Extra-regio', 'CH01': 'CH: Switzerland - Region Lemanique', 'CH02': 'CH: Switzerland - Espace Mittelland', 'CH03': 'CH: Switzerland - Nordwestschweiz', 'CH04': 'CH: Switzerland - Zürich', 'CH05': 'CH: Switzerland - Ostschweiz', 'CH06': 'CH: Switzerland - Zentralschweiz', 'CH07': 'CH: Switzerland - Ticino', 'CHZZ': 'CH: Extra-region- Extra-region', 'CZ01': ' CZ: ČESKÁ REPUBLIKA - Praha', 'CZ02': ' CZ: ČESKÁ REPUBLIKA - Střední Čechy', 'CZ03': ' CZ: ČESKÁ REPUBLIKA - Jihozápad', 'CZ04': ' CZ: ČESKÁ REPUBLIKA - Severozápad', 'CZ05': ' CZ: ČESKÁ REPUBLIKA - Severovýchod', 'CZ06': ' CZ: ČESKÁ REPUBLIKA - Jihovýchod', 'CZ07': ' CZ: ČESKÁ REPUBLIKA - Střední Morava', 'CZ08': ' CZ: ČESKÁ REPUBLIKA - Moravskoslezsko', 'CZZZ': ' CZ: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'DE11': ' DE: BADEN-WÜRTTEMBERG - Stuttgart', 'DE12': ' DE: BADEN-WÜRTTEMBERG - Karlsruhe', 'DE13': ' DE: BADEN-WÜRTTEMBERG - Freiburg', 'DE14': ' DE: BADEN-WÜRTTEMBERG - Tübingen', 'DE21': ' DE: BAYERN - Oberbayern', 'DE22': ' DE: BAYERN - Niederbayern', 'DE23': ' DE: BAYERN - Oberpfalz', 'DE24': ' DE: BAYERN - Oberfranken', 'DE25': ' DE: BAYERN - Mittelfranken', 'DE26': ' DE: BAYERN - Unterfranken', 'DE27': ' DE: BAYERN - Schwaben', 'DE30': ' DE: BERLIN - Berlin', 'DE40': ' DE: BRANDENBURG - Brandenburg', 'DE50': ' DE: BREMEN - Brandenburg', 'DE60': ' DE: HAMBURG - Hamburg', 'DE71': ' DE: HESSEN - Darmstadt', 'DE72': ' DE: HESSEN - Gießen', 'DE73': ' DE: HESSEN - Kassel', 'DE80': ' DE: MECKLENBURG-VORPOMMERN - Mecklenburg-Vorpommern', 'DE91': ' DE: NIEDERSACHSEN - Braunschweig', 'DE92': ' DE: NIEDERSACHSEN - Hannover', 'DE93': ' DE: NIEDERSACHSEN - Lüneburg', 'DE94': ' DE: NIEDERSACHSEN - Weser-Ems', 'DEA1': ' DE: NORDRHEIN-WESTFALEN - Düsseldorf', 'DEA2': ' DE: NORDRHEIN-WESTFALEN - Köln', 'DEA3': ' DE: NORDRHEIN-WESTFALEN - Münster', 'DEA4': ' DE: NORDRHEIN-WESTFALEN - Detmold', 'DEA5': ' DE: NORDRHEIN-WESTFALEN - Arnsberg', 'DEB1': ' DE: RHEINLAND-PFALZ - Koblenz', 'DEB2': ' DE: RHEINLAND-PFALZ - Trier', 'DEB3': ' DE: RHEINLAND-PFALZ - Rheinhessen-Pfalz', 'DEC0': ' DE: SAARLAND - Saarland', 'DED2': ' DE: SACHSEN - Dresden', 'DED4': ' DE: SACHSEN - Chemnitz', 'DED5': ' DE: SACHSEN - Leipzig', 'DEE0': ' DE: SACHSEN-ANHALT - Sachsen-Anhalt', 'DEF0': ' DE: SCHLESWIG-HOLSTEIN - Schleswig-Holstein', 'DEG0': ' DE: THÜRINGEN - Thüringen', 'DEZZ': ' DE: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'DK01': ' DK: DANMARK - Hovedstaden', 'DK02': ' DK: DANMARK - Sjælland', 'DK03': ' DK: DANMARK - Syddanmark', 'DK04': ' DK: DANMARK - Midtjylland', 'DK05': ' DK: DANMARK - Nordjylland', 'DKZZ': ' DK: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'EE00': ' EE: EESTI - Esti', 'EEZZ': ' EE: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'EL30': 'GR: ATTICA (ATTIKI) - Attica', 'EL41': 'GR: NISSIA AIGAIOU-KRITI - North Aegean (Previous Voreio Aigaio)', 'EL42': 'GR: NISSIA AIGAIOU-KRITI - South Aegean (Previous Notio Aigaio)', 'EL43': 'GR: NISSIA AIGAIOU-KRITI - Crete (Previous Kriti)', 'EL51': 'GR: VOREIA ELLADA - Eastern Macedonia And Thrace', 'EL52': 'GR: VOREIA ELLADA - Central Macedonia', 'EL53': 'GR: VOREIA ELLADA - Western Macedonia', 'EL54': 'GR: VOREIA ELLADA - Epirus', 'EL61': 'GR: KENTRIKI ELLADA - Thessaly', 'EL62': 'GR: KENTRIKI ELLADA - Ionian Islands', 'EL63': 'GR: KENTRIKI ELLADA - Western Greece', 'EL64': 'GR: KENTRIKI ELLADA - Central Greece (Previous Sterea Ellada)', 'EL65': 'GR: KENTRIKI ELLADA - Peloponnese', 'ES11': ' ES: NOROESTE - Galicia', 'ES12': ' ES: NOROESTE - Principado de Asturias', 'ES13': ' ES: NOROESTE - Cantabria', 'ES21': ' ES: NORESTE - País Vasco', 'ES22': ' ES: NORESTE - Comunidad Foral de Navarra', 'ES23': ' ES: NORESTE - La Rioja', 'ES24': ' ES: NORESTE - Aragón', 'ES30': ' ES: COMUNIDAD DE MADRID - Comunidad de Madrid', 'ES41': ' ES: CENTRO (ES) - Castilla y León', 'ES42': ' ES: CENTRO (ES) - Castilla-La Mancha', 'ES43': ' ES: CENTRO (ES) - Extremadura', 'ES51': ' ES: ESTE - Cataluña', 'ES52': ' ES: ESTE - Comunidad Valenciana', 'ES53': ' ES: ESTE - Illes Balears', 'ES61': ' ES: SUR - Andalucía', 'ES62': ' ES: SUR - Región de Murcia', 'ES63': ' ES: SUR - Ciudad Autónoma de Ceuta', 'ES64': ' ES: SUR - Ciudad Autónoma de Melilla', 'ES70': ' ES: CANARIAS - Canarias', 'ESZZ': ' ES: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'FI19': ' FI: MANNER-SUOMI - Länsi-Suomi', 'FI1B': ' FI: MANNER-SUOMI - Helsinki-Uusimaa', 'FI1C': ' FI: MANNER-SUOMI - Etelä-Suomi', 'FI1D': ' FI: MANNER-SUOMI - Pohjois- ja Itä-Suomi', 'FI20': ' FI: ÅLAND - ÅLAND', 'FIZZ': ' FI: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'FR10': ' FR: ÎLE-DE-FRANCE - Île-de-France', 'FRB0': ' FR: CENTRE-VAL DE LOIRE - Centre-Val de Loire', 'FRC1': ' FR: BOURGOGNE-FRANCHE-COMTÉ - Bourgogne', 'FRC2': ' FR: BOURGOGNE-FRANCHE-COMTÉ - Franche-Comté', 'FRD1': ' FR: NORMANDIE - Basse-Normandie', 'FRD2': ' FR: NORMANDIE - Haute-Normandie', 'FRE1': ' FR: HAUTS-DE-FRANCE - Nord-Pas-de-Calais', 'FRE2': ' FR: HAUTS-DE-FRANCE - Picardie', 'FRF1': ' FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE - Alsace', 'FRF2': ' FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE - Champagne-Ardenne', 'FRF3': ' FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE - Lorraine', 'FRG0': ' FR: PAYS-DE-LA-LOIRE - Pays-de-la-Loire', 'FRH0': ' FR: BRETAGNE - Bretagne', 'FRI1': ' FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES - Aquitaine', 'FRI2': ' FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES - Limousin', 'FRI3': ' FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES - Poitou-Charentes', 'FRJ1': ' FR: LANGUEDOC-ROUSSILLON-MIDI-PYRÉNÉES - Languedoc-Roussillon', 'FRJ2': ' FR: LANGUEDOC-ROUSSILLON-MIDI-PYRÉNÉES - Midi-Pyrénées', 'FRK1': ' FR: AUVERGNE-RHÔNE-ALPES - Auvergne', 'FRK2': ' FR: AUVERGNE-RHÔNE-ALPES - Rhône-Alpes', 'FRL0': ' FR: PROVENCE-ALPES-CÔTE D’AZUR - Provence-Alpes-Côte d’Azur', 'FRM0': ' FR: CORSE - Corse', 'FRY1': ' FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Guadeloupe', 'FRY2': ' FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Martinique', 'FRY3': ' FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Guyane', 'FRY4': ' FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - La Réunion', 'FRY5': ' FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Mayotte', 'FRZZ': ' FR: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'GE01': 'GE: Georgia - Capital', 'GE02': 'GE: Georgia - Western Georgia', 'GE03': 'GE: Georgia - Eastern Georgia', 'GEZZ': 'GE: Extra-regio - Extra-regio', 'GRZZ': 'GR: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'HR03': ' HR: HRVATSKA - Jadranska Hrvatska', 'HR04': ' HR: HRVATSKA - Kontinentalna Hrvatska', 'HRZZ': ' HR: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'HU11': ' HU: KÖZÉP-MAGYARORSZÁG - Budapest', 'HU12': ' HU: KÖZÉP-MAGYARORSZÁG - Pest', 'HU21': ' HU: DUNÁNTÚL - Közép-Dunántúl', 'HU22': ' HU: DUNÁNTÚL - Nyugat-Dunántúl', 'HU23': ' HU: DUNÁNTÚL - Dél-Dunántúl', 'HU31': ' HU: ALFÖLD ÉS ÉSZAK - Észak-Magyarország', 'HU32': ' HU: ALFÖLD ÉS ÉSZAK - Észak-Alföld', 'HU33': ' HU: ALFÖLD ÉS ÉSZAK - Dél-Alföld', 'HUZZ': ' HU: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'IS00': 'IS: Ísland - Ísland', 'ISZZ': 'IS: Extra-regio - Extra-regio', 'ITC1': ' IT: NORD-OVEST - Piemonte', 'ITC2': ' IT: NORD-OVEST - Valle d’Aosta/Vallée d’Aoste', 'ITC3': ' IT: NORD-OVEST - Liguria', 'ITC4': ' IT: NORD-OVEST - Lombardia', 'ITF1': ' IT: SUD - Abruzzo', 'ITF2': ' IT: SUD - Molise', 'ITF3': ' IT: SUD - Campania', 'ITF4': ' IT: SUD - Puglia', 'ITF5': ' IT: SUD - Basilicata', 'ITF6': ' IT: SUD - Calabria', 'ITG1': ' IT: ISOLE - Sicilia', 'ITG2': ' IT: ISOLE - Sardegna', 'ITH1': ' IT: NORD-EST - Provincia Autonoma di Bolzano/Bozen\xa0', 'ITH2': ' IT: NORD-EST - Provincia Autonoma di Trento', 'ITH3': ' IT: NORD-EST - Veneto', 'ITH4': ' IT: NORD-EST - Friuli-Venezia Giulia', 'ITH5': ' IT: NORD-EST - Emilia-Romagna', 'ITI1': ' IT: CENTRO (IT) - Toscana', 'ITI2': ' IT: CENTRO (IT) - Umbria', 'ITI3': ' IT: CENTRO (IT) - Marche', 'ITI4': ' IT: CENTRO (IT) - Lazio', 'ITZZ': ' IT: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'LT01': ' LT: LIETUVA - Sostinės regionas', 'LT02': ' LT: LIETUVA - Vidurio ir vakarų Lietuvos regionas', 'LTZZ': ' LT: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'LV00': ' LV: LATVIJA - Latvija', 'LVZZ': ' LV: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'ME00': 'ME:Montenegro - Montenegro', 'MK00': 'MK: MACEDONIA - Macedonia', 'MKZZ': 'MK: MACEDONIA - extra-regio', 'NL11': ' NL: NOORD-NEDERLAND - Groningen', 'NL12': ' NL: NOORD-NEDERLAND - Friesland (NL)', 'NL13': ' NL: NOORD-NEDERLAND - Drenthe', 'NL21': ' NL: OOST-NEDERLAND - Overijssel', 'NL22': ' NL: OOST-NEDERLAND - Gelderland', 'NL23': ' NL: OOST-NEDERLAND - Flevoland', 'NL31': ' NL: WEST-NEDERLAND - Utrecht', 'NL32': ' NL: WEST-NEDERLAND - Noord-Holland', 'NL33': ' NL: WEST-NEDERLAND - Zuid-Holland', 'NL34': ' NL: WEST-NEDERLAND - Zeeland', 'NL41': ' NL: ZUID-NEDERLAND - Noord-Brabant', 'NL42': ' NL: ZUID-NEDERLAND - Limburg (NL)', 'NLZZ': ' NL: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'NO01': 'NO: Norway - Oslo og Akershus', 'NO02': 'NO: Norway - Hedmark og Oppland', 'NO03': 'NO: Norway - Sør-Østlandet', 'NO04': 'NO: Norway - Agder og Rogaland', 'NO05': 'NO: Norway - Vestlandet', 'NO06': 'NO: Norway - Trøndelag', 'NO07': 'NO: Norway - Nord- Norge', 'NOZZ': 'NO: Extra-regio - Extra-regio', 'PL21': ' PL: MAKROREGION POŁUDNIOWY - Małopolskie', 'PL22': ' PL: MAKROREGION POŁUDNIOWY - Śląskie', 'PL41': ' PL: MAKROREGION PÓŁNOCNO-ZACHODNI - Wielkopolskie', 'PL42': ' PL: MAKROREGION PÓŁNOCNO-ZACHODNI - Zachodniopomorskie', 'PL43': ' PL: MAKROREGION PÓŁNOCNO-ZACHODNI - Lubuskie', 'PL51': ' PL: MAKROREGION POŁUDNIOWO-ZACHODNI - Dolnośląskie', 'PL52': ' PL: MAKROREGION POŁUDNIOWO-ZACHODNI - Opolskie', 'PL61': ' PL: MAKROREGION PÓŁNOCNY - Kujawsko-pomorskie', 'PL62': ' PL: MAKROREGION PÓŁNOCNY - Warmińsko-mazurskie', 'PL63': ' PL: MAKROREGION PÓŁNOCNY - Pomorskie', 'PL71': ' PL: MAKROREGION CENTRALNY - Łódzkie', 'PL72': ' PL: MAKROREGION CENTRALNY - Świętokrzyskie', 'PL81': ' PL: MAKROREGION WSCHODNI - Lubelskie', 'PL82': ' PL: MAKROREGION WSCHODNI - Podkarpackie', 'PL84': ' PL: MAKROREGION WSCHODNI - Podlaskie', 'PL91': ' PL: MAKROREGION WOJEWÓDZTWO MAZOWIECKIE - Warszawski stołeczny', 'PL92': ' PL: MAKROREGION WOJEWÓDZTWO MAZOWIECKIE - Mazowiecki regionalny', 'PLZZ': ' PL: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'PT11': 'PT: Continente - Norte', 'PT15': 'PT: Continente - Algarve', 'PT16': 'PT: Continente - Centro', 'PT17': 'PT: Continente - Área Metropolitana de Lisboa', 'PT18': 'PT: Continente - Alentejo', 'PT20': 'PT: Região Autónoma dos Açores - Região Autónoma dos Açores', 'PT30': 'PT: Região Autónoma da Madeira - Região Autónoma da Madeira', 'PTZZ': 'PT: Extra-regio - Extra-regio', 'RO11': ' RO: MACROREGIUNEA UNU - Nord-Vest', 'RO12': ' RO: MACROREGIUNEA UNU - Centru', 'RO21': ' RO: MACROREGIUNEA DOI - Nord-Est', 'RO22': ' RO: MACROREGIUNEA DOI - Sud-Est', 'RO31': ' RO: MACROREGIUNEA TREI - Sud-Muntenia', 'RO32': ' RO: MACROREGIUNEA TREI - București-Ilfov', 'RO41': ' RO: MACROREGIUNEA PATRU - Sud-Vest Oltenia', 'RO42': ' RO: MACROREGIUNEA PATRU - Vest', 'ROZZ': ' RO: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'RS11': 'RS: Sever - Grad Beograd', 'RS12': 'RS: Sever - Vojvodina', 'RS21': 'RS: Jug - Šumadija i zapadna Srbija', 'RS22': 'RS: Jug - Južna i istočna Srbija', 'RSZZ': 'RS: extra-regio - extra-regio', 'RU11': 'RU: Central Federal District - Central Federal District', 'RU21': 'RU: North West Federal District - North West Federal District', 'RU31': 'RU: South Federal District - South Federal District', 'RU41': 'RU: Privolzhsky Federal District - Privolzhsky Federal District', 'RU51': 'RU: Urals Federal District - Urals Federal District', 'RU61': 'RU: Siberian Federal District - Siberian Federal District', 'RU71': 'RU: Far East Federal District - Far East Federal District', 'RU81': 'RU: North Caucasian federal district - North Caucasian federal district', 'SE11': ' SE: ÖSTRA SVERIGE - Stockholm', 'SE12': ' SE: ÖSTRA SVERIGE - Östra Mellansverige', 'SE21': ' SE: SÖDRA SVERIGE - Småland med öarna', 'SE22': ' SE: SÖDRA SVERIGE - Sydsverige', 'SE23': ' SE: SÖDRA SVERIGE - Västsverige', 'SE31': ' SE: NORRA SVERIGE - Norra Mellansverige', 'SE32': ' SE: NORRA SVERIGE - Mellersta Norrland', 'SE33': ' SE: NORRA SVERIGE - Övre Norrland', 'SEZZ': ' SE: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'SI03': ' SI: SLOVENIJA - Vzhodna Slovenija', 'SI04': ' SI: SLOVENIJA - Zahodna Slovenija', 'SIZZ': ' SI: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'SK01': ' SK: SLOVENSKO - Bratislavský kraj', 'SK02': ' SK: SLOVENSKO - Západné Slovensko', 'SK03': ' SK: SLOVENSKO - Stredné Slovensko', 'SK04': ' SK: SLOVENSKO - Východné Slovensko', 'SKZZ': ' SK: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 'UA11': 'UA: WEST 1 - Volyn region', 'UA12': 'UA: WEST 1 - Zakarpattya region', 'UA13': 'UA: WEST 1 - Ivano-Frankivsk region', 'UA14': 'UA: WEST 1 - Lviv region', 'UA21': 'UA: WEST 2 - Rivne region', 'UA22': 'UA: WEST 2 - Ternopil region', 'UA23': 'UA: WEST 2 - Khmelnytsky region', 'UA24': 'UA: WEST 2 - Chernivtsi region', 'UA31': 'UA: THE CITY OF KYIV - The city of Kyiv', 'UA41': 'UA: SOUTH - Mykolaiv region', 'UA42': 'UA: SOUTH - Odesa region', 'UA43': 'UA: SOUTH - Kherson region', 'UA51': 'UA: NORTH - Zhytomyr region', 'UA52': 'UA: NORTH - Kyiv region', 'UA53': 'UA: NORTH - Chernihiv region', 'UA61': 'UA: EAST 1 - Dnipropetrovsk region', 'UA62': 'UA: EAST 1 - Sumy region', 'UA63': 'UA: EAST 1 - Kharkiv region', 'UA71': 'UA: EAST 2 - Donetsk region', 'UA72': 'UA: EAST 2 - Zaporizhya region', 'UA73': 'UA: EAST 2 - Luhansk region', 'UA81': 'UA: CENTER - Vinnytsia region', 'UA82': 'UA: CENTER - Kirovograd region', 'UA83': 'UA: CENTER - Poltava region', 'UA84': 'UA: CENTER - Cherkasy region', 'UKC1': ' UK - GB-GBN: North East (England) - Tees Valley and Durham', 'UKC2': ' UK - GB-GBN: North East (England) - Northumberland and Tyne and Wear', 'UKD1': ' UK - GB-GBN: North West (England) - Cumbria', 'UKD3': ' UK - GB-GBN: North West (England) - Greater Manchester', 'UKD4': ' UK - GB-GBN: North West (England) - Lancashire', 'UKD6': ' UK - GB-GBN: North West (England) - Cheshire', 'UKD7': ' UK - GB-GBN: North West (England) - Merseyside', 'UKE1': ' UK - GB-GBN: Yorkshire and the Humber - East Yorkshire and Northern Lincolnshire', 'UKE2': ' UK - GB-GBN: Yorkshire and the Humber - North Yorkshire', 'UKE3': ' UK - GB-GBN: Yorkshire and the Humber - South Yorkshire', 'UKE4': ' UK - GB-GBN: Yorkshire and the Humber - West Yorkshire', 'UKF1': ' UK - GB-GBN: East Midlands (England) - Derbyshire and Nottinghamshire', 'UKF2': ' UK - GB-GBN: East Midlands (England) - Leicestershire, Rutland and Northamptonshire', 'UKF3': ' UK - GB-GBN: East Midlands (England) - Lincolnshire', 'UKG1': ' UK - GB-GBN: West Midlands (England) - Herefordshire, Worcestershire and Warwickshire', 'UKG2': ' UK - GB-GBN: West Midlands (England) - Shropshire and Staffordshire', 'UKG3': ' UK - GB-GBN: West Midlands (England) - West Midlands', 'UKH1': ' UK - GB-GBN: East of England - East Anglia', 'UKH2': ' UK - GB-GBN: East of England - Bedfordshire and Hertfordshire', 'UKH3': ' UK - GB-GBN: East of England - Essex', 'UKI3': ' UK - GB-GBN: London - Inner London West', 'UKI4': ' UK - GB-GBN: London - Inner London East', 'UKI5': ' UK - GB-GBN: London - Outer London - East and North East', 'UKI6': ' UK - GB-GBN: London - Outer London - South', 'UKI7': ' UK - GB-GBN: London - Outer London - West and North West', 'UKJ1': ' UK - GB-GBN: South East (England) - Berkshire, Buckinghamshire and Oxfordshire', 'UKJ2': ' UK - GB-GBN: South East (England) - Surrey, East and West Sussex', 'UKJ3': ' UK - GB-GBN: South East (England) - Hampshire and Isle of Wight', 'UKJ4': ' UK - GB-GBN: South East (England) - Kent', 'UKK1': ' UK - GB-GBN: South West (England) - Gloucestershire, Wiltshire and Bristol/Bath area', 'UKK2': ' UK - GB-GBN: South West (England) - Dorset and Somerset', 'UKK3': ' UK - GB-GBN: South West (England) - Cornwall and Isles of Scilly', 'UKK4': ' UK - GB-GBN: South West (England) - Devon', 'UKL1': ' UK - GB-GBN: Wales - West Wales and The Valleys', 'UKL2': ' UK - GB-GBN: Wales - East Wales', 'UKM5': ' UK - GB-GBN: Scotland - North Eastern Scotland', 'UKM6': ' UK - GB-GBN: Scotland - Highlands and Islands', 'UKM7': ' UK - GB-GBN: Scotland - Eastern Scotland', 'UKM8': ' UK - GB-GBN: Scotland - West Central Scotland', 'UKM9': ' UK - GB-GBN: Scotland - Southern Scotland', 'UKN0': ' UK - GB-NIR: Northern Ireland - Northern Ireland', 'UKZZ': ' UK - EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2'} | {'-1': "don't know/no answer", '-4': 'item not included', '-5': 'other missing'} | True | 57248 | 312 |
v275b_N1 | 446 | v275b_N1 | nominal | region where interview was conducted: NUTS-1 code (Q105) | {' GRZ': 'GR: EXTRA-REGIO NUTS 1', 'AL0': 'AL: Albania', 'ALZ': 'AL: Extra-region', 'AM0': 'AM: Armenia', 'AMZ': 'AM: Extra-region', 'AT1': ' AT: OSTÖSTERREICH', 'AT2': ' AT: SÜDÖSTERREICH', 'AT3': ' AT: WESTÖSTERREICH', 'ATZ': ' AT: EXTRA-REGIO NUTS 1', 'AZ1': 'AZ: BAKU CITY', 'AZ2': 'AZ: APSHERON', 'AZ3': 'AZ:KUBA-KHACHMAZ', 'AZ4': 'AZ: ARAN', 'AZ5': 'AZ: LENKARAN', 'AZ6': 'AZ:MOUNTAINOUS SHIRVAN', 'AZ7': 'AZ: GANJA-KAZAKH', 'AZ8': 'AZ: SHEKI-ZAKATALA', 'BA': 'BA: Bosnia and Herzegovina', 'BG0': ' BG: BULGARIA', 'BG3': ' BG: SEVERNA I YUGOIZTOCHNA BULGARIA', 'BG4': ' BG: YUGOZAPADNA I YUZHNA TSENTRALNA BULGARIA', 'BGZ': ' BG: EXTRA-REGIO NUTS 1', 'BY0': 'BY: Belarus', 'BYZ': 'BY: Extra-region', 'CH0': 'CH: Switzerland', 'CHZ': 'CH: Extra-regio', 'CZ0': ' CZ: ČESKÁ REPUBLIKA', 'CZZ': ' CZ: EXTRA-REGIO NUTS 1', 'DE1': ' DE: BADEN-WÜRTTEMBERG', 'DE2': ' DE: BAYERN', 'DE3': ' DE: BERLIN', 'DE4': ' DE: BRANDENBURG', 'DE5': ' DE: BREMEN', 'DE6': ' DE: HAMBURG', 'DE7': ' DE: HESSEN', 'DE8': ' DE: MECKLENBURG-VORPOMMERN', 'DE9': ' DE: NIEDERSACHSEN', 'DEA': ' DE: NORDRHEIN-WESTFALEN', 'DEB': ' DE: RHEINLAND-PFALZ', 'DEC': ' DE: SAARLAND', 'DED': ' DE: SACHSEN', 'DEE': ' DE: SACHSEN-ANHALT', 'DEF': ' DE: SCHLESWIG-HOLSTEIN', 'DEG': ' DE: THÜRINGEN', 'DEZ': ' DE: EXTRA-REGIO NUTS 1', 'DK0': ' DK: DANMARK', 'DKZ': ' DK: EXTRA-REGIO NUTS 1', 'EE0': ' EE: EESTI', 'EEZ': ' EE: EXTRA-REGIO NUTS 1', 'EL3': 'GR: ATTICA (ATTIKI)', 'EL4': 'GR: NISSIA AIGAIOU-KRITI', 'EL5': 'GR: VOREIA ELLADA', 'EL6': 'GR: KENTRIKI ELLADA', 'ES1': ' ES: NOROESTE', 'ES2': ' ES: NORESTE', 'ES3': ' ES: COMUNIDAD DE MADRID', 'ES4': ' ES: CENTRO (ES)', 'ES5': ' ES: ESTE', 'ES6': ' ES: SUR', 'ES7': ' ES: CANARIAS', 'ESZ': ' ES: EXTRA-REGIO NUTS 1', 'FI1': ' FI: MANNER-SUOMI', 'FI2': ' FI: ÅLAND', 'FIZ': ' FI: EXTRA-REGIO NUTS 1', 'FR1': ' FR: ÎLE-DE-FRANCE', 'FRB': ' FR: CENTRE-VAL DE LOIRE', 'FRC': ' FR: BOURGOGNE-FRANCHE-COMTÉ', 'FRD': ' FR: NORMANDIE', 'FRE': ' FR: HAUTS-DE-FRANCE', 'FRF': ' FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE', 'FRG': ' FR: PAYS-DE-LA-LOIRE', 'FRH': ' FR: BRETAGNE', 'FRI': ' FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES', 'FRJ': ' FR: LANGUEDOC-ROUSSILLON-MIDI-PYRÉNÉES', 'FRK': ' FR: AUVERGNE-RHÔNE-ALPES', 'FRL': ' FR: PROVENCE-ALPES-CÔTE D’AZUR', 'FRM': ' FR: CORSE', 'FRY': ' FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES', 'FRZ': ' FR: EXTRA-REGIO NUTS 1', 'GE0': 'GE: Georgia', 'GEZ': 'GE: Extra-regio', 'HR0': ' HR: HRVATSKA', 'HRZ': ' HR: EXTRA-REGIO NUTS 1', 'HU1': ' HU - KÖZÉP-MAGYARORSZÁG', 'HU2': ' HU - DUNÁNTÚL', 'HU3': ' HU - ALFÖLD ÉS ÉSZAK', 'HUZ': ' HU - EXTRA-REGIO NUTS 1', 'IS0': 'IS: ÍSLAND', 'ISZ': 'IS: Extra-regio', 'ITC': ' IT: NORD-OVEST', 'ITF': ' IT: SUD', 'ITG': ' IT: ISOLE', 'ITH': ' IT: NORD-EST', 'ITI': ' IT: CENTRO (IT)', 'ITZ': ' IT: EXTRA-REGIO NUTS 1', 'LT0': ' LT: LIETUVA', 'LTZ': ' LT: EXTRA-REGIO NUTS 1', 'LV0': 'LV: LATVIJA', 'LVZ': 'LV: EXTRA-REGIO NUTS 1', 'ME0': 'ME: Montenegro', 'MK0': 'MK: MACEDONIA', 'MKZ': 'MK: EXTRA REGIO NUTS 1', 'NL1': ' NL: NOORD-NEDERLAND', 'NL2': ' NL: OOST-NEDERLAND', 'NL3': ' NL: WEST-NEDERLAND', 'NL4': ' NL: ZUID-NEDERLAND', 'NLZ': ' NL: EXTRA-REGIO NUTS 1', 'NO0': 'NO: Norway', 'NOZ': 'NO: Extra-regio', 'PL2': ' PL: MAKROREGION POŁUDNIOWY', 'PL4': ' PL: MAKROREGION PÓŁNOCNO-ZACHODNI', 'PL5': ' PL: MAKROREGION POŁUDNIOWO-ZACHODNI', 'PL6': ' PL: MAKROREGION PÓŁNOCNY', 'PL7': ' PL: MAKROREGION CENTRALNY', 'PL8': ' PL: MAKROREGION WSCHODNI', 'PL9': ' PL: MAKROREGION WOJEWÓDZTWO MAZOWIECKIE', 'PLZ': ' PL: EXTRA-REGIO NUTS 1', 'PT1': 'PT: Continente', 'PT2': 'PT: Região Autónoma dos Açores', 'PT3': 'PT: Região Autónoma da Madeira', 'PTZ': 'PT: Extra-regio', 'RO1': ' RO: MACROREGIUNEA UNU', 'RO2': ' RO: MACROREGIUNEA DOI', 'RO3': ' RO: MACROREGIUNEA TREI', 'RO4': ' RO: MACROREGIUNEA PATRU', 'ROZ': ' RO: EXTRA-REGIO NUTS 1', 'RS1': 'RS: Sever', 'RS2': 'RS: Jug', 'RSZ': 'RS: Extra-region', 'RU1': 'RU: Central Federal District', 'RU2': 'RU: North West federal district', 'RU3': 'RU: South Federal district', 'RU4': 'RU: Privolzhsky federal district', 'RU5': 'RU: Urals federal district', 'RU6': 'RU: Siberian federal district', 'RU7': 'RU: Far East federal district', 'RU8': 'RU: North Caucasian federal district', 'SE1': ' SE: ÖSTRA SVERIGE', 'SE2': ' SE: SÖDRA SVERIGE', 'SE3': ' SE: NORRA SVERIGE', 'SEZ': ' SE: EXTRA-REGIO NUTS 1', 'SI0': ' SI: SLOVENIJA', 'SIZ': ' SI: EXTRA-REGIO NUTS 1', 'SK0': ' SK: SLOVENSKO', 'SKZ': ' SK: EXTRA-REGIO NUTS 1', 'UA1': ' UA: WEST 1', 'UA2': ' UA: WEST 2', 'UA3': ' UA: THE CITY OF KYIV', 'UA4': ' UA: SOUTH', 'UA5': ' UA: NORTH', 'UA6': ' UA: EAST 1', 'UA7': ' UA: EAST 2', 'UA8': ' UA: CENTER', 'UKC': ' UK - GB-GBN: NORTH EAST (ENGLAND)', 'UKD': ' UK - GB-GBN: NORTH WEST (ENGLAND)', 'UKE': ' UK - GB-GBN: YORKSHIRE AND THE HUMBER', 'UKF': ' UK - GB-GBN: EAST MIDLANDS (ENGLAND)', 'UKG': ' UK - GB-GBN: WEST MIDLANDS (ENGLAND)', 'UKH': ' UK - GB-GBN: EAST OF ENGLAND', 'UKI': ' UK - GB-GBN: LONDON', 'UKJ': ' UK - GB-GBN: SOUTH EAST (ENGLAND)', 'UKK': ' UK - GB-GBN: SOUTH WEST (ENGLAND)', 'UKL': ' UK - GB-GBN: WALES', 'UKM': ' UK - GB-GBN: SCOTLAND', 'UKN': ' UK - GB-NIR: NORTHERN IRELAND', 'UKZ': ' UK - EXTRA-REGIO NUTS 1'} | {'-1': "don't know/no answer", '-4': 'item not included', '-5': 'other missing'} | True | 59418 | 123 |
v275c_N2 | 447 | v275c_N2 | nominal | region where interview was conducted: EVS numeric NUTS-2 code (Q105) | {80001.0: 'AL: ALBANIA - Northern Albania', 80102.0: 'AL: ALBANIA - Central Albania', 80203.0: 'AL: ALBANIA - Southern Albania', 80335.0: 'AL: EXTRA-REGIO - Extra-regio', 310100.0: 'AZ: BAKU CITY -Baku city', 310201.0: 'AZ: APSHERON - Absheron', 310202.0: 'AZ: APSHERON - Khyzy', 310203.0: 'AZ: APSHERON - Sumgait city', 310300.0: 'AZ: KUBA-KHACHMAZ - Gubinsky', 310301.0: 'AZ: KUBA-KHACHMAZ - Gusar', 310302.0: 'AZ: KUBA-KHACHMAZ - Khachmaz', 310303.0: 'AZ: KUBA-KHACHMAZ - Shabran', 310304.0: 'AZ: KUBA-KHACHMAZ - Siyazan', 310400.0: 'AZ: ARAN - Agdash', 310401.0: 'AZ: ARAN - Agdzhebedinsky', 310402.0: 'AZ: ARAN - Barda', 310403.0: 'AZ: ARAN - Beylagan', 310404.0: 'AZ: ARAN - Bilasuvar', 310405.0: 'AZ: ARAN - Goychay', 310406.0: 'AZ: ARAN - Hajikabul', 310407.0: 'AZ: ARAN - Imishli', 310408.0: 'AZ: ARAN - Kurdamir', 310409.0: 'AZ: ARAN - Mingechaur city', 310410.0: 'AZ: ARAN - Neftchala', 310411.0: 'AZ: ARAN - Saatly', 310412.0: 'AZ: ARAN - Sabirabad', 310413.0: 'AZ: ARAN - Salyan', 310414.0: 'AZ: ARAN - Shirvan city', 310415.0: 'AZ: ARAN - Ujar', 310416.0: 'AZ: ARAN - Yevlakh', 310417.0: 'AZ: ARAN - Zardob', 310500.0: 'AZ: LENKARAN - Astara', 310501.0: 'AZ: LENKARAN - Jalilabad', 310502.0: 'AZ: LENKARAN - Lankaran', 310503.0: 'AZ: LENKARAN - Lerik', 310504.0: 'AZ: LENKARAN - Masalli', 310505.0: 'AZ: LENKARAN - Yardimli', 310600.0: 'AZ: MOUNTAINOUS SHIRVAN - Agsu', 310601.0: 'AZ: MOUNTAINOUS SHIRVAN - Gobustan', 310602.0: 'AZ: MOUNTAINOUS SHIRVAN - Ismaili', 310603.0: 'AZ: MOUNTAINOUS SHIRVAN - Shemakha', 310700.0: 'AZ: GANJA-KAZAKH - Agstafa', 310701.0: 'AZ: GANJA-KAZAKH - Dashkesan', 310702.0: 'AZ: GANJA-KAZAKH - Ganja city', 310703.0: 'AZ: GANJA-KAZAKH - Gay-Gelsky', 310704.0: 'AZ: GANJA-KAZAKH - Gazakh', 310705.0: 'AZ: GANJA-KAZAKH - Goranboy', 310706.0: 'AZ: GANJA-KAZAKH - Kedabegsky', 310707.0: 'AZ: GANJA-KAZAKH - Naftalan city', 310708.0: 'AZ: GANJA-KAZAKH - Samukh', 310709.0: 'AZ: GANJA-KAZAKH - Shamkir', 310710.0: 'AZ: GANJA-KAZAKH - Tovuz', 310800.0: 'AZ: SHEKI-ZAKATALA - Belakansky', 310801.0: 'AZ: SHEKI-ZAKATALA - Gabala region', 310802.0: 'AZ: SHEKI-ZAKATALA - Gakh', 310803.0: 'AZ: SHEKI-ZAKATALA - Oguz', 310804.0: 'AZ: SHEKI-ZAKATALA - Sheki', 310805.0: 'AZ: SHEKI-ZAKATALA - Zakatala', 313535.0: 'AZ: EXTRA-REGIO - extra-regio', 400101.0: 'AT: OSTÖSTERREICH - Burgenland', 400102.0: 'AT: OSTÖSTERREICH - Niederösterreich', 400103.0: 'AT: OSTÖSTERREICH - Wien', 400201.0: 'AT: SÜDÖSTERREICH - Kärnten', 400202.0: 'AT: SÜDÖSTERREICH - Steiermark', 400301.0: 'AT: WESTÖSTERREICH - Oberösterreich', 400302.0: 'AT: WESTÖSTERREICH - Salzburg', 400303.0: 'AT: WESTÖSTERREICH - Tirol', 400304.0: 'AT: WESTÖSTERREICH - Vorarlberg', 403535.0: 'AT: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 510001.0: 'AM: ARMENIA - Yerevan', 510002.0: 'AM: ARMENIA - II Region', 510003.0: 'AM: ARMENIA - III Region', 510004.0: 'AM: ARMENIA - IV Region', 513535.0: 'AM: EXTRA-REGIO - extra-regio', 700001.0: 'BA: BOSNIA AND HERZEGOVINA - Federation of Bosnia i Herzegovina', 700002.0: 'BA: BOSNIA AND HERZEGOVINA - Republica Srpska', 700003.0: 'BA: BOSNIA AND HERZEGOVINA - Brčko DISTRICT', 1000301.0: 'BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Severozapaden', 1000302.0: 'BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Severen tsentralen', 1000303.0: 'BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Severoiztochen', 1000304.0: 'BG: SEVERNA I YUGOIZTOCHNA BULGARIA - Yugoiztochen', 1000401.0: 'BG: YUGOZAPADNA I YUZHNA TSENTRALNA BULGARIA - Yugozapaden', 1000402.0: 'BG: YUGOZAPADNA I YUZHNA TSENTRALNA BULGARIA - Yuzhen tsentralen', 1003535.0: 'BG: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 1120000.0: 'BY: BELARUS - Belarus', 1120001.0: 'BY: BELARUS - Brest region', 1120002.0: 'BY: BELARUS - Vitebsk region', 1120003.0: 'BY: BELARUS - Gomel region', 1120004.0: 'BY: BELARUS - Grodno region', 1120005.0: 'BY: BELARUS - Minsk (capital city)', 1120006.0: 'BY: BELARUS - Minsk region', 1120007.0: 'BY: BELARUS - Mogilev region', 1123535.0: 'BY: EXTRA-REGIO - extra-regio', 1910003.0: 'HR: HRVATSKA - Jadranska Hrvatska', 1910004.0: 'HR: HRVATSKA - Kontinentalna Hrvatska', 1913535.0: 'HR: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 2030001.0: 'CZ: ČESKÁ REPUBLIKA - Praha', 2030002.0: 'CZ: ČESKÁ REPUBLIKA - Střední Čechy', 2030003.0: 'CZ: ČESKÁ REPUBLIKA - Jihozápad', 2030004.0: 'CZ: ČESKÁ REPUBLIKA - Severozápad', 2030005.0: 'CZ: ČESKÁ REPUBLIKA - Severovýchod', 2030006.0: 'CZ: ČESKÁ REPUBLIKA - Jihovýchod', 2030007.0: 'CZ: ČESKÁ REPUBLIKA - Střední Morava', 2030008.0: 'CZ: ČESKÁ REPUBLIKA - Moravskoslezsko', 2033535.0: 'CZ: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 2080001.0: 'DK: DANMARK - Hovedstaden', 2080002.0: 'DK: DANMARK - Sjælland', 2080003.0: 'DK: DANMARK - Syddanmark', 2080004.0: 'DK: DANMARK - Midtjylland', 2080005.0: 'DK: DANMARK - Nordjylland', 2083535.0: 'DK: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 2330000.0: 'EE: EESTI - Esti', 2333535.0: 'EE: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 2460109.0: 'FI: MANNER-SUOMI - Länsi-Suomi', 2460111.0: 'FI: MANNER-SUOMI - Helsinki-Uusimaa', 2460112.0: 'FI: MANNER-SUOMI - Etelä-Suomi', 2460113.0: 'FI: MANNER-SUOMI - Pohjois- ja Itä-Suomi', 2460200.0: 'FI: ÅLAND - ÅLAND', 2463535.0: 'FI: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 2500100.0: 'FR: ÎLE-DE-FRANCE - Île-de-France', 2501100.0: 'FR: CENTRE-VAL DE LOIRE - Centre-Val de Loire', 2501201.0: 'FR: BOURGOGNE-FRANCHE-COMTÉ - Bourgogne', 2501202.0: 'FR: BOURGOGNE-FRANCHE-COMTÉ - Franche-Comté', 2501301.0: 'FR: NORMANDIE - Basse-Normandie', 2501302.0: 'FR: NORMANDIE - Haute-Normandie', 2501401.0: 'FR: HAUTS-DE-FRANCE - Nord-Pas-de-Calais', 2501402.0: 'FR: HAUTS-DE-FRANCE - Picardie', 2501501.0: 'FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE - Alsace', 2501502.0: 'FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE - Champagne-Ardenne', 2501503.0: 'FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE - Lorraine', 2501600.0: 'FR: PAYS-DE-LA-LOIRE - Pays-de-la-Loire', 2501700.0: 'FR: BRETAGNE - Bretagne', 2501801.0: 'FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES - Aquitaine', 2501802.0: 'FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES - Limousin', 2501803.0: 'FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES - Poitou-Charentes', 2501901.0: 'FR: LANGUEDOC-ROUSSILLON-MIDI-PYRÉNÉES - Languedoc-Roussillon', 2501902.0: 'FR: LANGUEDOC-ROUSSILLON-MIDI-PYRÉNÉES - Midi-Pyrénées', 2502001.0: 'FR: AUVERGNE-RHÔNE-ALPES - Auvergne', 2502002.0: 'FR: AUVERGNE-RHÔNE-ALPES - Rhône-Alpes', 2502100.0: 'FR: PROVENCE-ALPES-CÔTE D’AZUR - Provence-Alpes-Côte d’Azur', 2502200.0: 'FR: CORSE - Corse', 2503401.0: 'FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Guadeloupe', 2503402.0: 'FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Martinique', 2503403.0: 'FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Guyane', 2503404.0: 'FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - La Réunion', 2503405.0: 'FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES - Mayotte', 2503535.0: 'FR: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 2680001.0: 'GE: GEORGIA - Capital', 2680002.0: 'GE: GEORGIA - Western Georgia', 2680003.0: 'GE: GEORGIA - Eastern Georgia', 2683535.0: 'GE: EXTRA-REGIO - Extra-regio', 2760101.0: 'DE: BADEN-WÜRTTEMBERG - Stuttgart', 2760102.0: 'DE: BADEN-WÜRTTEMBERG - Karlsruhe', 2760103.0: 'DE: BADEN-WÜRTTEMBERG - Freiburg', 2760104.0: 'DE: BADEN-WÜRTTEMBERG - Tübingen', 2760201.0: 'DE: BAYERN - Oberbayern', 2760202.0: 'DE: BAYERN - Niederbayern', 2760203.0: 'DE: BAYERN - Oberpfalz', 2760204.0: 'DE: BAYERN - Oberfranken', 2760205.0: 'DE: BAYERN - Mittelfranken', 2760206.0: 'DE: BAYERN - Unterfranken', 2760207.0: 'DE: BAYERN - Schwaben', 2760300.0: 'DE: BERLIN - Berlin', 2760400.0: 'DE: BRANDENBURG - Brandenburg', 2760500.0: 'DE: BREMEN - Brandenburg', 2760600.0: 'DE: HAMBURG - Hamburg', 2760701.0: 'DE: HESSEN - Darmstadt', 2760702.0: 'DE: HESSEN - Gießen', 2760703.0: 'DE: HESSEN - Kassel', 2760800.0: 'DE: MECKLENBURG-VORPOMMERN - Mecklenburg-Vorpommern', 2760901.0: 'DE: NIEDERSACHSEN - Braunschweig', 2760902.0: 'DE: NIEDERSACHSEN - Hannover', 2760903.0: 'DE: NIEDERSACHSEN - Lüneburg', 2760904.0: 'DE: NIEDERSACHSEN - Weser-Ems', 2761001.0: 'DE: NORDRHEIN-WESTFALEN - Düsseldorf', 2761002.0: 'DE: NORDRHEIN-WESTFALEN - Köln', 2761003.0: 'DE: NORDRHEIN-WESTFALEN - Münster', 2761004.0: 'DE: NORDRHEIN-WESTFALEN - Detmold', 2761005.0: 'DE: NORDRHEIN-WESTFALEN - Arnsberg', 2761101.0: 'DE: RHEINLAND-PFALZ - Koblenz', 2761102.0: 'DE: RHEINLAND-PFALZ - Trier', 2761103.0: 'DE: RHEINLAND-PFALZ - Rheinhessen-Pfalz', 2761200.0: 'DE: SAARLAND - Saarland', 2761302.0: 'DE: SACHSEN - Dresden', 2761304.0: 'DE: SACHSEN - Chemnitz', 2761305.0: 'DE: SACHSEN - Leipzig', 2761400.0: 'DE: SACHSEN-ANHALT - Sachsen-Anhalt', 2761500.0: 'DE: SCHLESWIG-HOLSTEIN - Schleswig-Holstein', 2761600.0: 'DE: THÜRINGEN - Thüringen', 2763535.0: 'DE: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 3000300.0: 'GR: ATTICA (ATTIKI) - Attica', 3000401.0: 'GR: NISSIA AIGAIOU-KRITI - North Aegean (Previous Voreio Aigaio)', 3000402.0: 'GR: NISSIA AIGAIOU-KRITI - South Aegean (Previous Notio Aigaio)', 3000403.0: 'GR: NISSIA AIGAIOU-KRITI - Crete (Previous Kriti)', 3000501.0: 'GR: VOREIA ELLADA - Eastern Macedonia And Thrace', 3000502.0: 'GR: VOREIA ELLADA - Central Macedonia', 3000503.0: 'GR: VOREIA ELLADA - Western Macedonia', 3000504.0: 'GR: VOREIA ELLADA - Epirus', 3000601.0: 'GR: KENTRIKI ELLADA - Thessaly', 3000602.0: 'GR: KENTRIKI ELLADA - Ionian Islands', 3000603.0: 'GR: KENTRIKI ELLADA - Western Greece', 3000604.0: 'GR: KENTRIKI ELLADA - Central Greece (Previous Sterea Ellada)', 3000605.0: 'GR: KENTRIKI ELLADA - Peloponnese', 3003535.0: 'GR: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 3480101.0: 'HU: KÖZÉP-MAGYARORSZÁG - Budapest', 3480102.0: 'HU: KÖZÉP-MAGYARORSZÁG - Pest', 3480201.0: 'HU: DUNÁNTÚL - Közép-Dunántúl', 3480202.0: 'HU: DUNÁNTÚL - Nyugat-Dunántúl', 3480203.0: 'HU: DUNÁNTÚL - Dél-Dunántúl', 3480301.0: 'HU: ALFÖLD ÉS ÉSZAK - Észak-Magyarország', 3480302.0: 'HU: ALFÖLD ÉS ÉSZAK - Észak-Alföld', 3480303.0: 'HU: ALFÖLD ÉS ÉSZAK - Dél-Alföld', 3483535.0: 'HU: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 3520000.0: 'IS: ÍSLAND - Ísland', 3523535.0: 'IS: EXTRA-REGIO - Extra-regio', 3801201.0: 'IT: NORD-OVEST - Piemonte', 3801202.0: 'IT: NORD-OVEST - Valle d’Aosta/Vallée d’Aoste', 3801203.0: 'IT: NORD-OVEST - Liguria', 3801204.0: 'IT: NORD-OVEST - Lombardia', 3801501.0: 'IT: SUD - Abruzzo', 3801502.0: 'IT: SUD - Molise', 3801503.0: 'IT: SUD - Campania', 3801504.0: 'IT: SUD - Puglia', 3801505.0: 'IT: SUD - Basilicata', 3801506.0: 'IT: SUD - Calabria', 3801601.0: 'IT: ISOLE - Sicilia', 3801602.0: 'IT: ISOLE - Sardegna', 3801701.0: 'IT: NORD-EST - Provincia Autonoma di Bolzano/Bozen\xa0', 3801702.0: 'IT: NORD-EST - Provincia Autonoma di Trento', 3801703.0: 'IT: NORD-EST - Veneto', 3801704.0: 'IT: NORD-EST - Friuli-Venezia Giulia', 3801705.0: 'IT: NORD-EST - Emilia-Romagna', 3801801.0: 'IT: CENTRO (IT) - Toscana', 3801802.0: 'IT: CENTRO (IT) - Umbria', 3801803.0: 'IT: CENTRO (IT) - Marche', 3801804.0: 'IT: CENTRO (IT) - Lazio', 3803535.0: 'IT: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 4280000.0: 'LV: LATVIJA - Latvija', 4283535.0: ' LV: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 4400001.0: 'LT: LIETUVA - Sostinės regionas', 4400002.0: 'LT: LIETUVA - Vidurio ir vakarų Lietuvos regionas', 4403535.0: 'LT: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 4990000.0: 'ME: MONTENEGRO - Montenegro', 5280101.0: 'NL: NOORD-NEDERLAND - Groningen', 5280102.0: 'NL: NOORD-NEDERLAND - Friesland (NL)', 5280103.0: 'NL: NOORD-NEDERLAND - Drenthe', 5280201.0: 'NL: OOST-NEDERLAND - Overijssel', 5280202.0: 'NL: OOST-NEDERLAND - Gelderland', 5280203.0: 'NL: OOST-NEDERLAND - Flevoland', 5280301.0: 'NL: WEST-NEDERLAND - Utrecht', 5280302.0: 'NL: WEST-NEDERLAND - Noord-Holland', 5280303.0: 'NL: WEST-NEDERLAND - Zuid-Holland', 5280304.0: 'NL: WEST-NEDERLAND - Zeeland', 5280401.0: 'NL: ZUID-NEDERLAND - Noord-Brabant', 5280402.0: 'NL: ZUID-NEDERLAND - Limburg (NL)', 5283535.0: 'NL: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 5780001.0: 'NO: NORWAY - Oslo og Akershus', 5780002.0: 'NO: NORWAY - Hedmark og Oppland', 5780003.0: 'NO: NORWAY - Sør-Østlandet', 5780004.0: 'NO: NORWAY - Agder og Rogaland', 5780005.0: 'NO: NORWAY - Vestlandet', 5780006.0: 'NO: NORWAY - Trøndelag', 5780007.0: 'NO: NORWAY - Nord- Norge', 5783535.0: 'NO: EXTRA-REGIO - Extra-regio', 6160201.0: 'PL: MAKROREGION POŁUDNIOWY - Małopolskie', 6160202.0: 'PL: MAKROREGION POŁUDNIOWY - Śląskie', 6160401.0: 'PL: MAKROREGION PÓŁNOCNO-ZACHODNI - Wielkopolskie', 6160402.0: 'PL: MAKROREGION PÓŁNOCNO-ZACHODNI - Zachodniopomorskie', 6160403.0: 'PL: MAKROREGION PÓŁNOCNO-ZACHODNI - Lubuskie', 6160501.0: 'PL: MAKROREGION POŁUDNIOWO-ZACHODNI - Dolnośląskie', 6160502.0: 'PL: MAKROREGION POŁUDNIOWO-ZACHODNI - Opolskie', 6160601.0: 'PL: MAKROREGION PÓŁNOCNY - Kujawsko-pomorskie', 6160602.0: 'PL: MAKROREGION PÓŁNOCNY - Warmińsko-mazurskie', 6160603.0: 'PL: MAKROREGION PÓŁNOCNY - Pomorskie', 6160701.0: 'PL: MAKROREGION CENTRALNY - Łódzkie', 6160702.0: 'PL: MAKROREGION CENTRALNY - Świętokrzyskie', 6160801.0: 'PL: MAKROREGION WSCHODNI - Lubelskie', 6160802.0: 'PL: MAKROREGION WSCHODNI - Podkarpackie', 6160804.0: 'PL: MAKROREGION WSCHODNI - Podlaskie', 6160901.0: 'PL: MAKROREGION WOJEWÓDZTWO MAZOWIECKIE - Warszawski stołeczny', 6160902.0: 'PL: MAKROREGION WOJEWÓDZTWO MAZOWIECKIE - Mazowiecki regionalny', 6163535.0: 'PL: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 6200101.0: 'PT: Continente - Norte', 6200105.0: 'PT: Continente - Algarve', 6200106.0: 'PT: Continente - Centro', 6200107.0: 'PT: Continente - Área Metropolitana de Lisboa', 6200108.0: 'PT: Continente - Alentejo', 6200200.0: 'PT: Região Autónoma dos Açores - Região Autónoma dos Açores', 6200300.0: 'PT: Região Autónoma da Madeira - Região Autónoma da Madeira', 6203535.0: 'PT: Extra-regio - Extra-regio', 6420101.0: 'RO: MACROREGIUNEA UNU - Nord-Vest', 6420102.0: 'RO: MACROREGIUNEA UNU - Centru', 6420201.0: 'RO: MACROREGIUNEA DOI - Nord-Est', 6420202.0: 'RO: MACROREGIUNEA DOI - Sud-Est', 6420301.0: 'RO: MACROREGIUNEA TREI - Sud-Muntenia', 6420302.0: 'RO: MACROREGIUNEA TREI - București-Ilfov', 6420401.0: 'RO: MACROREGIUNEA PATRU - Sud-Vest Oltenia', 6420402.0: 'RO: MACROREGIUNEA PATRU - Vest', 6423535.0: 'RO: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 6430101.0: 'RU: CENTRAL FEDERAL DISTRICT - Central Federal District', 6430201.0: 'RU: NORTH WEST FEDERAL DISTRICT - North West Federal District', 6430301.0: 'RU: SOUTH FEDERAL DISTRICT - South Federal District', 6430401.0: 'RU: PRIVOLZHSKY FEDERAL DISTRICT - Privolzhsky Federal District', 6430501.0: 'RU: URALS FEDERAL DISTRICT - Urals Federal District', 6430601.0: 'RU: SIBERIAN FEDERAL DISTRICT - Siberian Federal District', 6430701.0: 'RU: FAR EAST FEDERAL DISTRICT - Far East Federal District', 6430801.0: 'RU: NORTH CAUCASIAN FEDERAL DISTRICT - North Caucasian federal district', 6880101.0: 'RS: SEVER - Beogradski region', 6880102.0: 'RS: SEVER - Region Vojvodine', 6880201.0: 'RS: JUG - Region Šumadije i Zapadne Srbije', 6880202.0: 'RS: JUG - Region Južne i Istočne Srbije', 6883535.0: 'RS: EXTRA-REGIO - Extra-regio', 7030001.0: 'SK: SLOVENSKO - Bratislavský kraj', 7030002.0: 'SK: SLOVENSKO - Západné Slovensko', 7030003.0: 'SK: SLOVENSKO - Stredné Slovensko', 7030004.0: 'SK: SLOVENSKO - Východné Slovensko', 7033535.0: 'SK: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 7050003.0: 'SI: SLOVENIJA - Vzhodna Slovenija', 7050004.0: 'SI: SLOVENIJA - Zahodna Slovenija', 7053535.0: 'SI: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 7240101.0: 'ES: NOROESTE - Galicia', 7240102.0: 'ES: NOROESTE - Principado de Asturias', 7240103.0: 'ES: NOROESTE - Cantabria', 7240201.0: 'ES: NORESTE - País Vasco', 7240202.0: 'ES: NORESTE - Comunidad Foral de Navarra', 7240203.0: 'ES: NORESTE - La Rioja', 7240204.0: 'ES: NORESTE - Aragón', 7240300.0: 'ES: COMUNIDAD DE MADRID - Comunidad de Madrid', 7240401.0: 'ES: CENTRO (ES) - Castilla y León', 7240402.0: 'ES: CENTRO (ES) - Castilla-La Mancha', 7240403.0: 'ES: CENTRO (ES) - Extremadura', 7240501.0: 'ES: ESTE - Cataluña', 7240502.0: 'ES: ESTE - Comunidad Valenciana', 7240503.0: 'ES: ESTE - Illes Balears', 7240601.0: 'ES: SUR - Andalucía', 7240602.0: 'ES: SUR - Región de Murcia', 7240603.0: 'ES: SUR - Ciudad Autónoma de Ceuta', 7240604.0: 'ES: SUR - Ciudad Autónoma de Melilla', 7240700.0: 'ES: CANARIAS - Canarias', 7243535.0: 'ES: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 7520101.0: 'SE: ÖSTRA SVERIGE - Stockholm', 7520102.0: 'SE: ÖSTRA SVERIGE - Östra Mellansverige', 7520201.0: 'SE: SÖDRA SVERIGE - Småland med öarna', 7520202.0: 'SE: SÖDRA SVERIGE - Sydsverige', 7520203.0: 'SE: SÖDRA SVERIGE - Västsverige', 7520301.0: 'SE: NORRA SVERIGE - Norra Mellansverige', 7520302.0: 'SE: NORRA SVERIGE - Mellersta Norrland', 7520303.0: 'SE: NORRA SVERIGE - Övre Norrland', 7523535.0: 'SE: EXTRA-REGIO NUTS 1 - Extra-Regio NUTS 2', 7560001.0: 'CH: SWITZERLAND - Region Lemanique', 7560002.0: 'CH: SWITZERLAND - Espace Mittelland', 7560003.0: 'CH: SWITZERLAND - Nordwestschweiz', 7560004.0: 'CH: SWITZERLAND - Zürich', 7560005.0: 'CH: SWITZERLAND - Ostschweiz', 7560006.0: 'CH: SWITZERLAND - Zentralschweiz', 7560007.0: 'CH: SWITZERLAND - Ticino', 7563535.0: 'CH: EXTRA-REGIO - Extra-regio', 8040101.0: 'UA: WEST 1 - Volyn region', 8040102.0: 'UA: WEST 1 - Zakarpattya region', 8040103.0: 'UA: WEST 1 - Ivano-Frankivsk region', 8040104.0: 'UA: WEST 1 - Lviv region', 8040201.0: 'UA: WEST 2 - Rivne region', 8040202.0: 'UA: WEST 2 - Ternopil region', 8040203.0: 'UA: WEST 2 - Khmelnytsky region', 8040204.0: 'UA: WEST 2 - Chernivtsi region', 8040301.0: 'UA: THE CITY OF KYIV - The city of Kyiv', 8040401.0: 'UA: SOUTH - Mykolaiv region', 8040402.0: 'UA: SOUTH - Odesa region', 8040403.0: 'UA: SOUTH - Kherson region', 8040501.0: 'UA: NORTH - Zhytomyr region', 8040502.0: 'UA: NORTH - Kyiv region', 8040503.0: 'UA: NORTH - Chernihiv region', 8040601.0: 'UA: EAST 1 - Dnipropetrovsk region', 8040602.0: 'UA: EAST 1 - Sumy region', 8040603.0: 'UA: EAST 1 - Kharkiv region', 8040701.0: 'UA: EAST 2 - Donetsk region', 8040702.0: 'UA: EAST 2 - Zaporizhya region', 8040703.0: 'UA: EAST 2 - Luhansk region', 8040801.0: 'UA: CENTER - Vinnytsia region', 8040802.0: 'UA: CENTER - Kirovograd region', 8040803.0: 'UA: CENTER - Poltava region', 8040804.0: 'UA: CENTER - Cherkasy region', 8070000.0: 'MK: MACEDONIA - Macedonia', 8073535.0: 'MK: MACEDONIA - extra-regio', 8261201.0: 'UK - GB-GBN: NORTH EAST (ENGLAND) - Tees Valley and Durham', 8261202.0: 'UK - GB-GBN: NORTH EAST (ENGLAND) - Northumberland and Tyne and Wear', 8261301.0: 'UK - GB-GBN: NORTH WEST (ENGLAND) - Cumbria', 8261303.0: 'UK - GB-GBN: NORTH WEST (ENGLAND) - Greater Manchester', 8261304.0: 'UK - GB-GBN: NORTH WEST (ENGLAND) - Lancashire', 8261306.0: 'UK - GB-GBN: NORTH WEST (ENGLAND) - Cheshire', 8261307.0: 'UK - GB-GBN: NORTH WEST (ENGLAND) - Merseyside', 8261401.0: 'UK - GB-GBN: YORKSHIRE AND THE HUMBER - East Yorkshire and Northern Lincolnshire', 8261402.0: 'UK - GB-GBN: YORKSHIRE AND THE HUMBER - North Yorkshire', 8261403.0: 'UK - GB-GBN: YORKSHIRE AND THE HUMBER - South Yorkshire', 8261404.0: 'UK - GB-GBN: YORKSHIRE AND THE HUMBER - West Yorkshire', 8261501.0: 'UK - GB-GBN: EAST MIDLANDS (ENGLAND) - Derbyshire and Nottinghamshire', 8261502.0: 'UK - GB-GBN: EAST MIDLANDS (ENGLAND) - Leicestershire, Rutland and Northamptonshire', 8261503.0: 'UK - GB-GBN: EAST MIDLANDS (ENGLAND) - Lincolnshire', 8261601.0: 'UK - GB-GBN: WEST MIDLANDS (ENGLAND) - Herefordshire, Worcestershire and Warwickshire', 8261602.0: 'UK - GB-GBN: WEST MIDLANDS (ENGLAND) - Shropshire and Staffordshire', 8261603.0: 'UK - GB-GBN: WEST MIDLANDS (ENGLAND) - West Midlands', 8261701.0: 'UK - GB-GBN: EAST OF ENGLAND - East Anglia', 8261702.0: 'UK - GB-GBN: EAST OF ENGLAND - Bedfordshire and Hertfordshire', 8261703.0: 'UK - GB-GBN: EAST OF ENGLAND - Essex', 8261803.0: 'UK - GB-GBN: LONDON - Inner London West', 8261804.0: 'UK - GB-GBN: LONDON - Inner London East', 8261805.0: 'UK - GB-GBN: LONDON - Outer London - East and North East', 8261806.0: 'UK - GB-GBN: LONDON - Outer London - South', 8261807.0: 'UK - GB-GBN: LONDON - Outer London - West and North West', 8261901.0: 'UK - GB-GBN: SOUTH EAST (ENGLAND) - Berkshire, Buckinghamshire and Oxfordshire', 8261902.0: 'UK - GB-GBN: SOUTH EAST (ENGLAND) - Surrey, East and West Sussex', 8261903.0: 'UK - GB-GBN: SOUTH EAST (ENGLAND) - Hampshire and Isle of Wight', 8261904.0: 'UK - GB-GBN: SOUTH EAST (ENGLAND) - Kent', 8262001.0: 'UK - GB-GBN: SOUTH WEST (ENGLAND) - Gloucestershire, Wiltshire and Bristol/Bath area', 8262002.0: 'UK - GB-GBN: SOUTH WEST (ENGLAND) - Dorset and Somerset', 8262003.0: 'UK - GB-GBN: SOUTH WEST (ENGLAND) - Cornwall and Isles of Scilly', 8262004.0: 'UK - GB-GBN: SOUTH WEST (ENGLAND) - Devon', 8262101.0: 'UK - GB-GBN: WALES - West Wales and The Valleys', 8262102.0: 'UK - GB-GBN: WALES - East Wales', 8262205.0: 'UK - GB-GBN: SCOTLAND - North Eastern Scotland', 8262206.0: 'UK - GB-GBN: SCOTLAND - Highlands and Islands', 8262207.0: 'UK - GB-GBN: SCOTLAND - Eastern Scotland', 8262208.0: 'UK - GB-GBN: SCOTLAND - West Central Scotland', 8262209.0: 'UK - GB-GBN: SCOTLAND - Southern Scotland', 8263535.0: 'UK - EXTRA-REGIO - EXTRA-REGIO', 9092300.0: 'UK - GB-NIR: NORTHERN IRELAND - Northern Ireland'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 57248 | 312 |
v275c_N1 | 448 | v275c_N1 | nominal | region where interview was conducted: EVS numeric NUTS-1 code (Q105) | {800.0: 'AL: ALBANIA', 835.0: 'AL: EXTRA-REGION', 3101.0: 'AZ: BAKU CITY', 3102.0: 'AZ: APSHERON', 3103.0: 'AZ: KUBA-KHACHMAZ', 3104.0: 'AZ: ARAN', 3105.0: 'AZ: LENKARAN', 3106.0: 'AZ: MOUNTAINOUS SHIRVAN', 3107.0: 'AZ: GANJA-KAZAKH', 3108.0: 'AZ: SHEKI-ZAKATALA', 3135.0: 'AZ: EXTRA-REGIO', 4001.0: 'AT: OSTÖSTERREICH', 4002.0: 'AT: SÜDÖSTERREICH', 4003.0: 'AT: WESTÖSTERREICH', 4035.0: 'AT: EXTRA-REGIO NUTS 1', 5100.0: 'AM: ARMENIA', 5135.0: 'AM: EXTRA-REGIO', 7000.0: 'BA: BOSNIA AND HERZEGOVINA', 10000.0: 'BG: BULGARIA', 10003.0: 'BG: SEVERNA I YUGOIZTOCHNA BULGARIA', 10004.0: 'BG: YUGOZAPADNA I YUZHNA TSENTRALNA BULGARIA', 10035.0: 'BG: EXTRA-REGIO NUTS 1', 11200.0: 'BY: BELARUS', 11235.0: 'BY: EXTRA-REGIO', 19100.0: 'HR: HRVATSKA', 19135.0: 'HR: EXTRA-REGIO NUTS 1', 20300.0: 'CZ: ČESKÁ REPUBLIKA', 20335.0: 'CZ: EXTRA-REGIO NUTS 1', 20800.0: 'DK: DANMARK', 20835.0: 'DK: EXTRA-REGIO NUTS 1', 23300.0: 'EE: EESTI', 23335.0: 'EE: EXTRA-REGIO NUTS 1', 24601.0: 'FI: MANNER-SUOMI', 24602.0: 'FI: ÅLAND', 24635.0: 'FI: EXTRA-REGIO NUTS 1', 25001.0: 'FR: ÎLE-DE-FRANCE', 25011.0: 'FR: CENTRE-VAL DE LOIRE', 25012.0: 'FR: BOURGOGNE-FRANCHE-COMTÉ', 25013.0: 'FR: NORMANDIE', 25014.0: 'FR: HAUTS-DE-FRANCE', 25015.0: 'FR: ALSACE-CHAMPAGNE-ARDENNE-LORRAINE', 25016.0: 'FR: PAYS-DE-LA-LOIRE', 25017.0: 'FR: BRETAGNE', 25018.0: 'FR: AQUITAINE-LIMOUSIN-POITOU-CHARENTES', 25019.0: 'FR: LANGUEDOC-ROUSSILLON-MIDI-PYRÉNÉES', 25020.0: 'FR: AUVERGNE-RHÔNE-ALPES', 25021.0: 'FR: PROVENCE-ALPES-CÔTE D’AZUR', 25022.0: 'FR: CORSE', 25034.0: 'FR: RUP FR — RÉGIONS ULTRAPÉRIPHÉRIQUES FRANÇAISES', 25035.0: 'FR: EXTRA-REGIO NUTS 1', 26800.0: 'GE: GEORGIA', 26835.0: 'GE: EXTRA-REGIO', 27601.0: 'DE: BADEN-WÜRTTEMBERG', 27602.0: 'DE: BAYERN', 27603.0: 'DE: BERLIN', 27604.0: 'DE: BRANDENBURG', 27605.0: 'DE: BREMEN', 27606.0: 'DE: HAMBURG', 27607.0: 'DE: HESSEN', 27608.0: 'DE: MECKLENBURG-VORPOMMERN', 27609.0: 'DE: NIEDERSACHSEN', 27610.0: 'DE: NORDRHEIN-WESTFALEN', 27611.0: 'DE: RHEINLAND-PFALZ', 27612.0: 'DE: SAARLAND', 27613.0: 'DE: SACHSEN', 27614.0: 'DE: SACHSEN-ANHALT', 27615.0: 'DE: SCHLESWIG-HOLSTEIN', 27616.0: 'DE: THÜRINGEN', 27635.0: 'DE: EXTRA-REGIO NUTS 1', 30003.0: 'GR: ATTICA (ATTIKI)', 30004.0: 'GR: NISSIA AIGAIOU-KRITI', 30005.0: 'GR: VOREIA ELLADA', 30006.0: 'GR: KENTRIKI ELLADA', 30035.0: 'GR: EXTRA-REGIO NUTS 1', 34801.0: 'HU: KÖZÉP-MAGYARORSZÁG', 34802.0: 'HU: DUNÁNTÚL', 34803.0: 'HU: ALFÖLD ÉS ÉSZAK', 34835.0: 'HU: EXTRA-REGIO NUTS 1', 35200.0: 'IS: ÍSLAND', 35235.0: 'IS: EXTRA-REGIO', 38012.0: 'IT: NORD-OVEST', 38015.0: 'IT: SUD', 38016.0: 'IT: ISOLE', 38017.0: 'IT: NORD-EST', 38018.0: 'IT: CENTRO (IT)', 38035.0: 'IT: EXTRA-REGIO NUTS 1', 42800.0: 'LV: LATVIJA', 42835.0: 'LV: EXTRA-REGIO NUTS 1', 44000.0: 'LT: LIETUVA', 44035.0: 'LT: EXTRA-REGIO NUTS 1', 49900.0: 'ME: MONTENEGRO', 52801.0: 'NL: NOORD-NEDERLAND', 52802.0: 'NL: OOST-NEDERLAND', 52803.0: 'NL: WEST-NEDERLAND', 52804.0: 'NL: ZUID-NEDERLAND', 52835.0: 'NL: EXTRA-REGIO NUTS 1', 57800.0: 'NO: NORWAY', 57835.0: 'NO: EXTRA-REGIO', 61602.0: 'PL: MAKROREGION POŁUDNIOWY', 61604.0: 'PL: MAKROREGION PÓŁNOCNO-ZACHODNI', 61605.0: 'PL: MAKROREGION POŁUDNIOWO-ZACHODNI', 61606.0: 'PL: MAKROREGION PÓŁNOCNY', 61607.0: 'PL: MAKROREGION CENTRALNY', 61608.0: 'PL: MAKROREGION WSCHODNI', 61609.0: 'PL: MAKROREGION WOJEWÓDZTWO MAZOWIECKIE', 61635.0: 'PL: EXTRA-REGIO NUTS 1', 62001.0: 'PT: Continente', 62002.0: 'PT: Região Autónoma dos Açores', 62003.0: 'PT: Região Autónoma da Madeira', 62035.0: 'PT: Extra-regio', 64201.0: 'RO: MACROREGIUNEA UNU', 64202.0: 'RO: MACROREGIUNEA DOI', 64203.0: 'RO: MACROREGIUNEA TREI', 64204.0: 'RO: MACROREGIUNEA PATRU', 64235.0: 'RO: EXTRA-REGIO NUTS 1', 64301.0: 'RU: CENTRAL FEDERAL DISTRICT', 64302.0: 'RU: NORTH WEST FEDERAL DISTRICT', 64303.0: 'RU: SOUTH FEDERAL DISTRICT', 64304.0: 'RU: PRIVOLZHSKY FEDERAL DISTRICT', 64305.0: 'RU: URALS FEDERAL DISTRICT', 64306.0: 'RU: SIBERIAN FEDERAL DISTRICT', 64307.0: 'RU: FAR EAST FEDERAL DISTRICT', 64308.0: 'RU: NORTH CAUCASIAN FEDERAL DISTRICT', 68801.0: 'RS: SEVER', 68802.0: 'RS: JUG', 68835.0: 'RS: EXTRA-REGION', 70300.0: 'SK: SLOVENSKO', 70335.0: 'SK: EXTRA-REGIO NUTS 1', 70500.0: 'SI: SLOVENIJA', 70535.0: 'SI: EXTRA-REGIO NUTS 1', 72401.0: 'ES: NOROESTE', 72402.0: 'ES: NORESTE', 72403.0: 'ES: COMUNIDAD DE MADRID', 72404.0: 'ES: CENTRO (ES)', 72405.0: 'ES: ESTE', 72406.0: 'ES: SUR', 72407.0: 'ES: CANARIAS', 72435.0: 'ES: EXTRA-REGIO NUTS 1', 75201.0: 'SE: ÖSTRA SVERIGE', 75202.0: 'SE: SÖDRA SVERIGE', 75203.0: 'SE: NORRA SVERIGE', 75235.0: 'SE: EXTRA-REGIO NUTS 1', 75600.0: 'CH: SWITZERLAND', 75635.0: 'CH: EXTRA-REGIO', 80401.0: 'UA: WEST 1', 80402.0: 'UA: WEST 2', 80403.0: 'UA: THE CITY OF KYIV', 80404.0: 'UA: SOUTH', 80405.0: 'UA: NORTH', 80406.0: 'UA: EAST 1', 80407.0: 'UA: EAST 2', 80408.0: 'UA: CENTER', 80700.0: 'MK: MACEDONIA', 80735.0: 'MK: EXTRA-REGION', 82612.0: 'UK - GB-GBN: NORTH EAST (ENGLAND)', 82613.0: 'UK - GB-GBN: NORTH WEST (ENGLAND)', 82614.0: 'UK - GB-GBN: YORKSHIRE AND THE HUMBER', 82615.0: 'UK - GB-GBN: EAST MIDLANDS (ENGLAND)', 82616.0: 'UK - GB-GBN: WEST MIDLANDS (ENGLAND)', 82617.0: 'UK - GB-GBN: EAST OF ENGLAND', 82618.0: 'UK - GB-GBN: LONDON', 82619.0: 'UK - GB-GBN: SOUTH EAST (ENGLAND)', 82620.0: 'UK - GB-GBN: SOUTH WEST (ENGLAND)', 82621.0: 'UK - GB-GBN: WALES', 82622.0: 'UK - GB-GBN: SCOTLAND', 82635.0: 'UK - EXTRA-REGIO', 90923.0: 'UK - GB-NIR: NORTHERN IRELAND'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59418 | 123 |
v276_r | 449 | v276_r | numeric | size of town where interview was conducted Q106 (5 categories) | {1.0: 'under 5000', 2.0: '5000-20000', 3.0: '20000-100000', 4.0: '100000-500000', 5.0: '500000 and more'} | {-4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 53444 | 5 |
v277 | 450 | v277 | numeric | date of interview (Q107) | {} | {-4.0: 'item not included', -2.0: 'no answer'} | True | 57588 | 951 |
mm_v277_fu | 451 | mm_v277_fu | numeric | MATRIX DESIGN EXPERIMENT: date of interview (Q107) (follow-up) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer'} | True | 3376 | 120 |
v278a | 452 | v278a | nominal | time of interview: start hour (Q108) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56217 | 24 |
v278b | 453 | v278b | nominal | time of interview: start minute (Q108) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56217 | 60 |
v278c_r | 454 | v278c_r | numeric | time of interview: start (constructed) (Q108) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -2.0: 'no answer'} | True | 56217 | 1278 |
v279a | 455 | v279a | nominal | time of interview: end hour (Q108) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56138 | 25 |
v279b | 456 | v279b | nominal | time of interview: end minute (Q108) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56138 | 60 |
v279c_r | 457 | v279c_r | numeric | time of interview: end (constructed) (Q108) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -2.0: 'no answer'} | True | 56138 | 1295 |
v279d_r | 458 | v279d_r | numeric | time of interview: duration in minutes (constructed) (Q109) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -6.0: 'na (survey break-off)', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55336 | 502 |
mm_v278a_fu | 459 | mm_v278a_fu | nominal | MATRIX DESIGN EXPERIMENT: time of interview: start hour (Q108) (follow-up) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable'} | False | 2855 | 24 |
mm_v278b_fu | 460 | mm_v278b_fu | nominal | MATRIX DESIGN EXPERIMENT: time of interview: start minute (Q108) (follow-up) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable'} | False | 2855 | 60 |
mm_v279a_fu | 461 | mm_v279a_fu | nominal | MATRIX DESIGN EXPERIMENT: time of interview: end hour (Q108) (follow-up) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer'} | False | 2823 | 24 |
mm_v279b_fu | 462 | mm_v279b_fu | nominal | MATRIX DESIGN EXPERIMENT: time of interview: end minute (Q108) (follow-up) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer'} | False | 2823 | 60 |
v280 | 463 | v280 | numeric | interest of respondent during interview (Q109) | {1.0: 'very interested', 2.0: 'somewhat interested', 3.0: 'not very interested'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54488 | 3 |
v281a | 464 | v281a | nominal | language of interview (ISO 639-1 Alpha code) (Q110) | {'aa': 'Afar', 'ab': 'Abkhazian', 'ae': 'Avestan', 'af': 'Afrikaans', 'ak': 'Akan', 'am': 'Amharic', 'an': 'Aragonese', 'ar': 'Arabic', 'as': 'Assamese', 'av': 'Avaric', 'ay': 'Aymara', 'az': 'Azerbaijani', 'ba': 'Bashkir', 'be': 'Belarusian', 'bg': 'Bulgarian', 'bh': 'Bihari', 'bi': 'Bislama', 'bm': 'Bambara', 'bn': 'Bengali', 'bo': 'Tibetan', 'br': 'Breton', 'bs': 'Bosnian', 'ca': 'Catalan', 'ce': 'Chechen', 'ch': 'Chamorro', 'cnr': 'Montenegrin (ISO 639-2 Alpha code)', 'co': 'Corsican', 'cr': 'Cree', 'cs': 'Czech', 'cu': 'Church Slavic', 'cv': 'Chuvash', 'cy': 'Welsh', 'da': 'Danish', 'de': 'German', 'dv': 'Divehi', 'dz': 'Dzongkha', 'ee': 'Ewe', 'el': 'Greek', 'en': 'English', 'eo': 'Esperanto', 'es': 'Spanish', 'et': 'Estonian', 'eu': 'Basque', 'fa': 'Persian', 'ff': 'Fulah', 'fi': 'Finnish', 'fj': 'Fijian', 'fo': 'Faroese', 'fr': 'French', 'fy': 'Western Frisian', 'ga': 'Irish', 'gd': 'Scottish Gaelic', 'gl': 'Galician', 'gn': 'Guaraní', 'gu': 'Gujarati', 'gv': 'Manx', 'ha': 'Hausa', 'he': 'Hebrew', 'hi': 'Hindi', 'ho': 'Hiri Motu', 'hr': 'Croatian', 'ht': 'Haitian', 'hu': 'Hungarian', 'hy': 'Armenian', 'hz': 'Herero', 'ia': 'Interlingua (International Auxiliary Language Association)', 'id': 'Indonesian', 'ie': 'Interlingue', 'ig': 'Igbo', 'ii': 'Sichuan Yi', 'ik': 'Inupiaq', 'io': 'Ido', 'is': 'Icelandic', 'it': 'Italian', 'iu': 'Inuktitut', 'ja': 'Japanese', 'jv': 'Javanese', 'ka': 'Georgian', 'kg': 'Kongo', 'ki': 'Kikuyu', 'kj': 'Kwanyama', 'kk': 'Kazakh', 'kl': 'Kalaallisut', 'km': 'Khmer', 'kn': 'Kannada', 'ko': 'Korean', 'kr': 'Kanuri', 'ks': 'Kashmiri', 'ku': 'Kurdish', 'kv': 'Komi', 'kw': 'Cornish', 'ky': 'Kirghiz', 'la': 'Latin', 'lb': 'Luxembourgish', 'lg': 'Ganda', 'li': 'Limburgish', 'ln': 'Lingala', 'lo': 'Lao', 'lt': 'Lithuanian', 'lu': 'Luba‐Katanga', 'lv': 'Latvian', 'mg': 'Malagasy', 'mh': 'Marshallese', 'mi': 'Māori', 'mk': 'Macedonian', 'ml': 'Malayalam', 'mn': 'Mongolian', 'mo': 'Moldavian', 'mr': 'Marathi', 'ms': 'Malay', 'mt': 'Maltese', 'my': 'Burmese', 'na': 'Nauru', 'nb': 'Norwegian Bokmål', 'nd': 'North Ndebele', 'ne': 'Nepali', 'ng': 'Ndonga', 'nl': 'Dutch', 'nn': 'Norwegian Nynorsk', 'no': 'Norwegian', 'nr': 'South Ndebele', 'nv': 'Navajo', 'ny': 'Chichewa', 'oc': 'Occitan', 'oj': 'Ojibwa', 'om': 'Oromo', 'or': 'Oriya', 'os': 'Ossetian', 'pa': 'Panjabi', 'pi': 'Pāli', 'pl': 'Polish', 'ps': 'Pashto', 'pt': 'Portuguese', 'qu': 'Quechua', 'rm': 'Raeto‐Romance', 'rn': 'Kirundi', 'ro': 'Romanian', 'ru': 'Russian', 'rw': 'Kinyarwanda', 'sa': 'Sanskrit', 'sc': 'Sardinian', 'sd': 'Sindhi', 'se': 'Northern Sami', 'sg': 'Sango', 'sh': 'Serbo‐Croatian', 'si': 'Sinhalese', 'sk': 'Slovak', 'sl': 'Slovenian', 'sm': 'Samoan', 'sn': 'Shona', 'so': 'Somali', 'sq': 'Albanian', 'sr': 'Serbian', 'ss': 'Swati', 'st': 'Sotho', 'su': 'Sundanese', 'sv': 'Swedish', 'sw': 'Swahili', 'ta': 'Tamil', 'te': 'Telugu', 'tg': 'Tajik', 'th': 'Thai', 'ti': 'Tigrinya', 'tk': 'Turkmen', 'tl': 'Tagalog', 'tn': 'Tswana', 'to': 'Tonga', 'tr': 'Turkish', 'ts': 'Tsonga', 'tt': 'Tatar', 'tw': 'Twi', 'ty': 'Tahitian', 'ug': 'Uighur', 'uk': 'Ukrainian', 'ur': 'Urdu', 'uz': 'Uzbek', 've': 'Venda', 'vi': 'Vietnamese', 'vo': 'Volapük', 'wa': 'Walloon', 'wo': 'Wolof', 'xh': 'Xhosa', 'yi': 'Yiddish', 'yo': 'Yoruba', 'za': 'Zhuang', 'zh': 'Chinese', 'zu': 'Zulu'} | {'-1': 'dont know or no answer', '-3': 'not applicable'} | True | 59430 | 36 |
v281a_r | 465 | v281a_r | numeric | language of interview (WVS/EVS numeric 3-digit) (Q110) | {2.0: 'Basque', 3.0: 'Galician', 13.0: 'Albanian', 25.0: 'Armenian', 32.0: 'Danish', 40.0: 'Azerbaijani', 65.0: 'Bosnian', 69.0: 'Bulgarian', 72.0: 'Icelandic', 75.0: 'Catalan', 90.0: 'Croatian', 98.0: 'Lithuanian', 101.0: 'Chinese', 120.0: 'Dutch', 128.0: 'English', 132.0: 'Estonian', 141.0: 'Finnish', 144.0: 'French', 157.0: 'Georgian', 158.0: 'German', 166.0: 'Greek', 184.0: 'Hungarian', 186.0: 'Montenegrin', 200.0: 'Norwegian', 207.0: 'Italian', 256.0: 'Latvian', 279.0: 'Macedonian', 363.0: 'Polish', 364.0: 'Portuguese', 377.0: 'Romanian', 380.0: 'Russian', 397.0: 'Serbian', 415.0: 'Slovak', 416.0: 'Slovenian', 426.0: 'Spanish', 435.0: 'Swedish', 475.0: 'Ukrainian', 600.0: 'Czech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non respons', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59430 | 36 |
v282 | 466 | v282 | numeric | interviewer number (Q111) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -5.0: 'other missing', -4.0: 'item not included'} | True | 51092 | 1989 |
gweight | 467 | gweight | numeric | Calibration weights | {} | {} | True | 59438 | 6924 |
gweight_no_edu | 468 | gweight_no_edu | numeric | Calibration weights: without educational level | {} | {} | True | 59438 | 2849 |
dweight | 469 | dweight | numeric | Design Weight | {} | {-4.0: 'item not included'} | True | 10246 | 2423 |
pweight | 470 | pweight | numeric | Population size weight | {} | {} | True | 59438 | 36 |
age_r3_weight | 471 | age_r3_weight | numeric | CALIBRATION variable: Age in categories | {1.0: '18-24', 2.0: '25-34', 3.0: '35-44', 4.0: '45-54', 5.0: '55-64', 6.0: '65-74', 7.0: '75+'} | {} | True | 59438 | 7 |
v225_weight | 472 | v225_weight | numeric | CALIBRATION variable: Gender | {1.0: 'Male', 2.0: 'Female'} | {} | True | 59438 | 2 |
v243_r_weight | 473 | v243_r_weight | numeric | CALIBRATION variable: Educational level | {1.0: 'lower', 2.0: 'medium', 3.0: 'higher'} | {} | True | 59438 | 3 |
We can see some important information about the 474 variables contained in this survey. Every variable has an id
, a name
, a type
and lists of valid
and invalid values
.
The column is_usable
indicates if the variable can be used for an automatic analysis and the calculation of an interpretable result. Depending on a variable’s type, there are different criteria that make a variable usable or unusable for the automatic system:
Numeric variables are always usable. Nominal and ordinal variables need to provide information about all valid and invalid values.
Variables of unknown type are not usable, as well as variables that take less than two different values on the whole dataset.
(Please note: If you use slightly lower level functionality of the library, you can use all variables and do whatever you want. But you have to “manually” take care of the feature creation, and you’re on your own to assemble all information to have an interpretable result.)
The loaded data contains a lot of administrative variables that are not interesting for a tangle analysis. We are mainly interested in the questions the respondents were actually asked. Nearly all variables corresponding to the ‘real’ questions have a name starting with a prefix “v” followed by a number. We want to extract only these columns.
Please note that there are exceptions to this rule and our simple heuristic will remove some special variables and some derived variables. For the purpose of this notebook, we just don’t care…
We can extract a subset of the columns using the function select_questions
. There are various ways to specify the selected columns, please consult the documentation for a detailed description. In the following cell, we use a small lambda
expression to extract variables with a name satisfying the heuristic criterion described above:
questions = complete_survey.select_questions(lambda x:x.name[0] == 'v' and x.name[1:].isnumeric(), suppress_check_var_warning=True)
Let’s look at the variable info, again:
styled_df(questions.variable_info())
id | name | type | label | valid_values | invalid_values | is_usable | num_valid_answers | num_unique_answers | |
---|---|---|---|---|---|---|---|---|---|
v1 | 0 | v1 | numeric | how important in your life: work (Q1A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58727 | 4 |
v2 | 1 | v2 | numeric | how important in your life: family (Q1B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59221 | 4 |
v3 | 2 | v3 | numeric | how important in your life: friends and acquaintances (Q1C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59215 | 4 |
v4 | 3 | v4 | numeric | how important in your life: leisure time (Q1D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59032 | 4 |
v5 | 4 | v5 | numeric | how important in your life: politics (Q1E) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58764 | 4 |
v6 | 5 | v6 | numeric | how important in your life: religion (Q1F) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58622 | 4 |
v7 | 6 | v7 | numeric | taking all things together how happy are you (Q2) | {1.0: 'very happy', 2.0: 'quite happy', 3.0: 'not very happy', 4.0: 'not at all happy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58707 | 4 |
v8 | 7 | v8 | numeric | describe your state of health these days (Q3) | {1.0: 'very good', 2.0: 'good', 3.0: 'fair', 4.0: 'poor', 5.0: 'very poor'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59304 | 5 |
v9 | 8 | v9 | numeric | do you belong to: religious organization (Q4A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58333 | 2 |
v10 | 9 | v10 | numeric | do you belong to: cultural activities (Q4B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58284 | 2 |
v11 | 10 | v11 | numeric | do you belong to: trade unions (Q4C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58269 | 2 |
v12 | 11 | v12 | numeric | do you belong to: political parties/groups (Q4D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58243 | 2 |
v13 | 12 | v13 | numeric | do you belong to: environment, ecology, animal rights (Q4E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58271 | 2 |
v14 | 13 | v14 | numeric | do you belong to: professional associations (Q4F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58223 | 2 |
v15 | 14 | v15 | numeric | do you belong to: sports/recreation (Q4G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58374 | 2 |
v16 | 15 | v16 | numeric | do you belong to: charitable/humanitarian organization (Q4H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58259 | 2 |
v17 | 16 | v17 | numeric | do you belong to: consumer organization (Q4I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58181 | 2 |
v18 | 17 | v18 | numeric | do you belong to: self-help group, mutual aid group (Q4J) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58176 | 2 |
v19 | 18 | v19 | numeric | do you belong to: other groups (Q4K) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57728 | 2 |
v20 | 19 | v20 | numeric | do you belong to: none (spontaneous) (Q4) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53185 | 2 |
v21 | 20 | v21 | numeric | did you do voluntary work in the last 6 months (Q5) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58986 | 2 |
v22 | 21 | v22 | numeric | dont like as neighbours: people of different race (Q6A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57084 | 2 |
v23 | 22 | v23 | numeric | dont like as neighbours: heavy drinkers (Q6B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58075 | 2 |
v24 | 23 | v24 | numeric | dont like as neighbours: immigrants/foreign workers (Q6C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56520 | 2 |
v25 | 24 | v25 | numeric | dont like as neighbours: drug addicts (Q6D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58247 | 2 |
v26 | 25 | v26 | numeric | dont like as neighbours: homosexuals (Q6E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57513 | 2 |
v27 | 26 | v27 | numeric | dont like as neighbours: Christians (optional in countries with Christian majority) (Q6F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49003 | 2 |
v28 | 27 | v28 | numeric | dont like as neighbours: Muslims (optional in countries with Muslim majority) (Q6G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55587 | 2 |
v29 | 28 | v29 | numeric | dont like as neighbours: Jews (optional) (Q6H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57061 | 2 |
v30 | 29 | v30 | numeric | dont like as neighbours: Gypsies (optional) (Q6I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57288 | 2 |
v31 | 30 | v31 | numeric | people can be trusted/can't be too careful (Q7) | {1.0: 'most people can be trusted', 2.0: 'can not be too careful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58083 | 2 |
v32 | 31 | v32 | numeric | how much you trust: your family (Q8A) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59178 | 4 |
v33 | 32 | v33 | numeric | how much you trust: people in your neighborhood (Q8B) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58616 | 4 |
v34 | 33 | v34 | numeric | how much you trust: people you know personally (Q8C) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58981 | 4 |
v35 | 34 | v35 | numeric | how much you trust: people you meet for the first time (Q8D) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57969 | 4 |
v36 | 35 | v36 | numeric | how much you trust: people of another religion (Q8E) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54785 | 4 |
v37 | 36 | v37 | numeric | how much you trust: people of another nationality (Q8F) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55087 | 4 |
v38 | 37 | v38 | numeric | how much control over your life (Q9) | {1.0: 'none at all', 10.0: 'a great deal'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58550 | 10 |
v39 | 38 | v39 | numeric | how satisfied are you with your life (Q10) | {1.0: 'dissatisfied', 10.0: 'satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59122 | 10 |
v40 | 39 | v40 | numeric | important in a job: good pay (Q11A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58740 | 2 |
v41 | 40 | v41 | numeric | important in a job: good hours (Q11B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58538 | 2 |
v42 | 41 | v42 | numeric | important in a job: opportunity to use initiative (Q11C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57902 | 2 |
v43 | 42 | v43 | numeric | important in a job: generous holidays (Q11D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58007 | 2 |
v44 | 43 | v44 | numeric | important in a job: achieving something (Q11E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58218 | 2 |
v45 | 44 | v45 | numeric | important in a job: responsible job (Q11F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57965 | 2 |
v46 | 45 | v46 | numeric | job needed to develop talents (Q12A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58777 | 5 |
v47 | 46 | v47 | numeric | humiliating receiving money without working (Q12B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58515 | 5 |
v48 | 47 | v48 | numeric | people turn lazy not working (Q12C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58687 | 5 |
v49 | 48 | v49 | numeric | work is a duty towards society (Q12D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58714 | 5 |
v50 | 49 | v50 | numeric | work comes always first (Q12E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58820 | 5 |
v51 | 50 | v51 | numeric | do you belong to a religious denomination (Q13) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58984 | 2 |
v52 | 51 | v52 | nominal | which religious denomination do you belong to (Q13a) (harmonized) | {1.0: 'Roman catholic', 2.0: 'Protestant', 3.0: 'Free church/Non-conformist/Evangelical', 4.0: 'Jew', 5.0: 'Muslim', 6.0: 'Hindu', 7.0: 'Buddhist', 8.0: 'Orthodox', 9.0: 'Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 41037 | 9 |
v53 | 52 | v53 | numeric | did you belong to a religious denomination (Q14) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 18919 | 2 |
v54 | 53 | v54 | numeric | how often attend religious services (Q15) | {1.0: 'more than once week', 2.0: 'once a week', 3.0: 'once a month', 4.0: 'only on specific holy days', 5.0: 'once a year', 6.0: 'less often', 7.0: 'never, practically never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58851 | 7 |
v55 | 54 | v55 | numeric | how often attend religious services 12 years old (Q16) | {1.0: 'more than once week', 2.0: 'once a week', 3.0: 'once a month', 4.0: 'only on specific holy days', 5.0: 'once a year', 6.0: 'less often', 7.0: 'never, practically never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57551 | 7 |
v56 | 55 | v56 | numeric | are you a religious person (Q17) | {1.0: 'a religious person', 2.0: 'not a religious person', 3.0: 'a convinced atheist'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57082 | 3 |
v57 | 56 | v57 | numeric | do you believe in: God (Q18A) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55894 | 2 |
v58 | 57 | v58 | numeric | do you believe in: life after death (Q18B) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51186 | 2 |
v59 | 58 | v59 | numeric | do you believe in: hell (Q18C) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51868 | 2 |
v60 | 59 | v60 | numeric | do you believe in: heaven (Q18D) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51899 | 2 |
v61 | 60 | v61 | numeric | do you believe in: re-incarnation (Q19) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52299 | 2 |
v62 | 61 | v62 | numeric | which statement closest to your beliefs (Q20) | {1.0: 'personal God', 2.0: 'spirit or life force', 3.0: "don't know what to think", 4.0: 'no spirit, God or life force'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57497 | 4 |
v63 | 62 | v63 | numeric | how important is God in your life (Q21) | {1.0: 'not at all important', 10.0: 'very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57947 | 10 |
v64 | 63 | v64 | numeric | how often do you pray outside religious services (Q22) | {1.0: 'every day', 2.0: 'more than once week', 3.0: 'once a week', 4.0: 'at least once a month', 5.0: 'several times a year', 6.0: 'less often', 7.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57882 | 7 |
v65 | 64 | v65 | numeric | important in marriage: faithfulness (Q23A) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59138 | 3 |
v66 | 65 | v66 | numeric | important in marriage: adequate income (Q23B) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59078 | 3 |
v67 | 66 | v67 | numeric | important in marriage: good housing (Q23C) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59121 | 3 |
v68 | 67 | v68 | numeric | important in marriage: share household chores (Q23D) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58887 | 3 |
v69 | 68 | v69 | numeric | important in marriage: children (Q23E) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58676 | 3 |
v70 | 69 | v70 | numeric | important in marriage: time for friends and personal hobbies (Q23F) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58814 | 3 |
v71 | 70 | v71 | numeric | marriage is outdated (Q24) | {1.0: 'agree', 2.0: 'disagree'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57349 | 2 |
v72 | 71 | v72 | numeric | child suffers with working mother (Q25A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58080 | 4 |
v73 | 72 | v73 | numeric | women really want home and children (Q25B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57241 | 4 |
v74 | 73 | v74 | numeric | family life suffers when woman has full-time job (Q25C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58055 | 4 |
v75 | 74 | v75 | numeric | man's job is to earn money; woman's job is to look after home and family (Q25D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58466 | 4 |
v76 | 75 | v76 | numeric | men make better political leaders than women (Q25E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56994 | 4 |
v77 | 76 | v77 | numeric | university education more important for a boy than for a girl (Q25F) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58183 | 4 |
v78 | 77 | v78 | numeric | men make better business executives than women (Q25G) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57158 | 4 |
v79 | 78 | v79 | numeric | one of main goals in life has been to make my parents proud (Q25H) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56622 | 4 |
v80 | 79 | v80 | numeric | jobs are scarce: giving...(nation) priority (Q26A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58633 | 5 |
v81 | 80 | v81 | numeric | jobs are scarce: giving men priority (Q26B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58695 | 5 |
v82 | 81 | v82 | numeric | homosexual couples - as good parents as other couples (Q27A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54764 | 5 |
v83 | 82 | v83 | numeric | duty towards society to have children (Q27B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58491 | 5 |
v84 | 83 | v84 | numeric | It is childs duty to provide long-term care for parents (Q27C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58678 | 5 |
v85 | 84 | v85 | numeric | learn children at home: good manners (Q28A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58798 | 2 |
v86 | 85 | v86 | numeric | learn children at home: independence (Q28B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58627 | 2 |
v87 | 86 | v87 | numeric | learn children at home: hard work (Q28C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58708 | 2 |
v88 | 87 | v88 | numeric | learn children at home: feeling of responsibility (Q28D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58729 | 2 |
v89 | 88 | v89 | numeric | learn children at home: imagination (Q28E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58160 | 2 |
v90 | 89 | v90 | numeric | learn children at home: tolerance and respect (Q28F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58560 | 2 |
v91 | 90 | v91 | numeric | learn children at home: thrift, saving money and things (Q28G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58432 | 2 |
v92 | 91 | v92 | numeric | learn children at home: determination, perseverance (Q28H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58376 | 2 |
v93 | 92 | v93 | numeric | learn children at home: religious faith (Q28I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58124 | 2 |
v94 | 93 | v94 | numeric | learn children at home: unselfishness (Q28J) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58190 | 2 |
v95 | 94 | v95 | numeric | learn children at home: obedience (Q28K) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58189 | 2 |
v96 | 95 | v96 | numeric | learn children at home: none (spontaneous) (Q28) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53671 | 2 |
v97 | 96 | v97 | numeric | how interested are you in politics (Q29) | {1.0: 'very interested', 2.0: 'somewhat interested', 3.0: 'not very interested', 4.0: 'not at all interested'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59132 | 4 |
v98 | 97 | v98 | numeric | political action: signing a petition (Q30A) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57002 | 3 |
v99 | 98 | v99 | numeric | political action: joining in boycotts (Q30B) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55875 | 3 |
v100 | 99 | v100 | numeric | political action: attending lawful demonstrations (Q30C) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56622 | 3 |
v101 | 100 | v101 | numeric | political action: joining unofficial strikes (Q30D) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55474 | 3 |
v102 | 101 | v102 | numeric | political view: left-right (Q31) | {1.0: 'the left', 10.0: 'the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47723 | 10 |
v103 | 102 | v103 | numeric | individual vs. state responsibility for providing (Q32A) | {1.0: 'individual responsibility', 10.0: 'state responsibility'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58214 | 10 |
v104 | 103 | v104 | numeric | take any job vs. right to refuse job when unemployed (Q32B) | {1.0: 'unemployed take any job', 10.0: 'unemployed right to refuse a job'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58066 | 10 |
v105 | 104 | v105 | numeric | competition is good vs. harmful (Q32C) | {1.0: 'competition good', 10.0: 'competition harmful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57516 | 10 |
v106 | 105 | v106 | numeric | equalize incomes vs. incentives for individual effort (Q32D) | {1.0: 'incomes more equal', 10.0: 'incentives to individual efforts'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58103 | 10 |
v107 | 106 | v107 | numeric | private vs. government ownership business (Q32E) | {1.0: 'private ownership increased', 10.0: 'government ownership increased'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54991 | 10 |
v108 | 107 | v108 | numeric | aims of this country: most important (Q33) | {1.0: 'high level of economic growth', 2.0: 'strong defense forces', 3.0: 'more say at their jobs/ in communities', 4.0: 'make cities/countryside more beautiful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57503 | 4 |
v109 | 108 | v109 | numeric | aims of this country: 2nd most important (Q33a) | {1.0: 'high level of economic growth', 2.0: 'strong defense forces', 3.0: 'more say at their jobs/ in communities', 4.0: 'make cities/countryside more beautiful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56342 | 4 |
v110 | 109 | v110 | numeric | aims of respondent: most important (Q34) | {1.0: 'maintaining order in nation', 2.0: 'more say in important government decisions', 3.0: 'fighting rising prices', 4.0: 'protect freedom of speech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58066 | 4 |
v111 | 110 | v111 | numeric | aims of respondent: 2nd most important (Q35) | {1.0: 'maintaining order in nation', 2.0: 'more say in important government decisions', 3.0: 'fighting rising prices', 4.0: 'protect freedom of speech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57178 | 4 |
v112 | 111 | v112 | numeric | are you willing to fight for country (Q36) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52718 | 2 |
v113 | 112 | v113 | numeric | good/bad: decrease work importance (Q37A) | {1.0: 'good', 2.0: 'bad', 3.0: "don't mind"} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56028 | 3 |
v114 | 113 | v114 | numeric | good/bad: greater respect for authority (Q37B) | {1.0: 'good', 2.0: 'bad', 3.0: "don't mind"} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55280 | 3 |
v115 | 114 | v115 | numeric | how much confidence in: church (Q38A) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57455 | 4 |
v116 | 115 | v116 | numeric | how much confidence in: armed forces (Q38B) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56006 | 4 |
v117 | 116 | v117 | numeric | how much confidence in: education system (Q38C) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58159 | 4 |
v118 | 117 | v118 | numeric | how much confidence in: the press (Q38D) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58072 | 4 |
v119 | 118 | v119 | numeric | how much confidence in: trade unions (Q38E) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54191 | 4 |
v120 | 119 | v120 | numeric | how much confidence in: the police (Q38F) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58495 | 4 |
v121 | 120 | v121 | numeric | how much confidence in: parliament (Q38G) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57366 | 4 |
v122 | 121 | v122 | numeric | how much confidence in: civil service (Q38H) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57228 | 4 |
v123 | 122 | v123 | numeric | how much confidence in: social security system (Q38I) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57294 | 4 |
v124 | 123 | v124 | numeric | how much confidence in: European Union (Q38J) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55705 | 4 |
v125 | 124 | v125 | numeric | how much confidence in: United Nations Organization (Q38K) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54168 | 4 |
v126 | 125 | v126 | numeric | how much confidence in: health care system (Q38L) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58585 | 4 |
v127 | 126 | v127 | numeric | how much confidence in: justice system (Q38M) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57092 | 4 |
v128 | 127 | v128 | numeric | how much confidence in: major companies (Q38N) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54645 | 4 |
v129 | 128 | v129 | numeric | how much confidence in: environmental organizations (Q38O) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55582 | 4 |
v130 | 129 | v130 | numeric | how much confidence in: political parties (Q38P) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56884 | 4 |
v131 | 130 | v131 | numeric | how much confidence in: government (Q38Q) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57506 | 4 |
v132 | 131 | v132 | numeric | how much confidence in: social media (Q38R) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54341 | 4 |
v133 | 132 | v133 | numeric | democracy: governments tax the rich and subsidize the poor (Q39A) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52980 | 11 |
v134 | 133 | v134 | numeric | democracy: religious authorities interpret the laws (Q39B) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51892 | 11 |
v135 | 134 | v135 | numeric | democracy: people choose their leaders in free elections (Q39C) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54015 | 11 |
v136 | 135 | v136 | numeric | democracy: people receive state aid for unemployment (Q39D) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53977 | 11 |
v137 | 136 | v137 | numeric | democracy: the army takes over when government is incompetent (Q39E) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51896 | 11 |
v138 | 137 | v138 | numeric | democracy: civil rights protect people from state oppression (Q39F) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52504 | 11 |
v139 | 138 | v139 | numeric | democracy: the state makes people’s incomes equal (Q39G) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53050 | 11 |
v140 | 139 | v140 | numeric | democracy: people obey their rulers (Q39H) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53068 | 11 |
v141 | 140 | v141 | numeric | democracy: women have the same rights as men (Q39I) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54426 | 11 |
v142 | 141 | v142 | numeric | importance of democracy (Q40) | {1.0: 'not at all important', 10.0: 'absolutely important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58125 | 10 |
v143 | 142 | v143 | numeric | democracy in own country (Q41) | {1.0: 'not at all democratic', 10.0: 'completely democratic'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57214 | 10 |
v144 | 143 | v144 | numeric | satisfaction political system (Q42) | {1.0: 'not satisfied at all', 10.0: 'completely satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57530 | 10 |
v145 | 144 | v145 | numeric | political system: strong leader (Q43A) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55374 | 4 |
v146 | 145 | v146 | numeric | political system: experts making decisions (Q43B) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54313 | 4 |
v147 | 146 | v147 | numeric | political system: the army ruling (Q43C) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55409 | 4 |
v148 | 147 | v148 | numeric | political system: democratic (Q43D) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56245 | 4 |
v149 | 148 | v149 | numeric | do you justify: claiming state benefits (Q44A) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58240 | 10 |
v150 | 149 | v150 | numeric | do you justify: cheating on tax (Q44B) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58511 | 10 |
v151 | 150 | v151 | numeric | do you justify: taking soft drugs (Q44C) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58442 | 10 |
v152 | 151 | v152 | numeric | do you justify: accepting a bribe (Q44D) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58723 | 10 |
v153 | 152 | v153 | numeric | do you justify: homosexuality (Q44E) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56952 | 10 |
v154 | 153 | v154 | numeric | do you justify: abortion (Q44F) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57277 | 10 |
v155 | 154 | v155 | numeric | do you justify: divorce (Q44G) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57903 | 10 |
v156 | 155 | v156 | numeric | do you justify: euthanasia (Q44H) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56185 | 10 |
v157 | 156 | v157 | numeric | do you justify: suicide (Q44I) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56705 | 10 |
v158 | 157 | v158 | numeric | do you justify: having casual sex (Q44J) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57033 | 10 |
v159 | 158 | v159 | numeric | do you justify: avoiding a fare on public transport (Q44K) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58559 | 10 |
v160 | 159 | v160 | numeric | do you justify: prostitution (Q44L) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57709 | 10 |
v161 | 160 | v161 | numeric | do you justify: artificial insemination or in-vitro fertilization (Q44M) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56800 | 10 |
v162 | 161 | v162 | numeric | do you justify: political violence (Q44N) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58009 | 10 |
v163 | 162 | v163 | numeric | do you justify: death penalty (Q44O) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57123 | 10 |
v164 | 163 | v164 | numeric | how close do you feel: to own town/city (Q45A) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58942 | 4 |
v165 | 164 | v165 | numeric | how close do you feel: to your [county, region, district] (Q45B) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58786 | 4 |
v166 | 165 | v166 | numeric | how close do you feel: to [country] (Q45C) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58857 | 4 |
v167 | 166 | v167 | numeric | how close do you feel: to [continent] (Q45D) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58110 | 4 |
v168 | 167 | v168 | numeric | how close do you feel: to world (Q45E) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57393 | 4 |
v169 | 168 | v169 | numeric | do you have... [country's] nationality (Q46) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59318 | 2 |
v170 | 169 | v170 | numeric | how proud are you to be a ... [country] citizen (Q47) | {1.0: 'very proud', 2.0: 'quite proud', 3.0: 'not very proud', 4.0: 'not at all proud'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55885 | 4 |
v171 | 170 | v171 | numeric | vote in elections: local level (Q48A) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 57525 | 3 |
v172 | 171 | v172 | numeric | vote in elections: national level (Q48B) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 56952 | 3 |
v173 | 172 | v173 | numeric | vote in elections: European level (Q48C) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 34242 | 3 |
v176 | 173 | v176 | numeric | how often in country's elections: votes are counted fairly (Q50A) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52270 | 4 |
v177 | 174 | v177 | numeric | how often in country's elections: opposition candidates are prevented from running (Q50B) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47740 | 4 |
v178 | 175 | v178 | numeric | how often in country's elections: TV news favors the governing party (Q50C) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51081 | 4 |
v179 | 176 | v179 | numeric | how often in country's elections: voters are bribed (Q50D) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49897 | 4 |
v180 | 177 | v180 | numeric | how often in country's elections: journalists provide fair coverage of elections (Q50E) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51685 | 4 |
v181 | 178 | v181 | numeric | how often in country's elections: election officials are fair (Q50F) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50664 | 4 |
v182 | 179 | v182 | numeric | how often in country's elections: rich people buy elections (Q50G) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 48126 | 4 |
v183 | 180 | v183 | numeric | how often in country's elections: voters are threatened with violence at the polls (Q50H) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49341 | 4 |
v184 | 181 | v184 | numeric | immigrants: impact on the development of [your country] (Q51) | {1.0: 'very bad', 2.0: 'quite bad', 3.0: 'neither good, nor bad', 4.0: 'quite good', 5.0: 'very good'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56958 | 5 |
v185 | 182 | v185 | numeric | immigrants take away jobs from [nationality] (Q52A) | {1.0: 'take away', 10.0: 'do not take away'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57389 | 10 |
v186 | 183 | v186 | numeric | immigrants increase crime problems (Q52B) | {1.0: 'make it worse', 10.0: 'do not make it worse'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56679 | 10 |
v187 | 184 | v187 | numeric | immigrants are a strain on welfare system (Q52C) | {1.0: 'are a strain', 10.0: 'are not a strain'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56578 | 10 |
v188 | 185 | v188 | numeric | better if immigrants maintain/not maintain own customs (Q52D) | {1.0: 'maintain distinct customs and traditions', 10.0: 'do not maintain distinct customs and traditions'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55706 | 10 |
v189 | 186 | v189 | numeric | important: to have been born in [country] (Q53A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58850 | 4 |
v190 | 187 | v190 | numeric | important: to respect [country nationality] political institutions and laws (Q53B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58850 | 4 |
v191 | 188 | v191 | numeric | important: to have [country nationality] ancestry (Q53C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58728 | 4 |
v192 | 189 | v192 | numeric | important: to be able to speak [national language] (Q53D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59034 | 4 |
v193 | 190 | v193 | numeric | important: to share [national] culture (Q53E) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58811 | 4 |
v194 | 191 | v194 | numeric | important: to be born in Europe (Q54A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57741 | 4 |
v195 | 192 | v195 | numeric | important: to have European ancestry (Q54B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57562 | 4 |
v196 | 193 | v196 | numeric | important: to be a Christian (Q54C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57302 | 4 |
v197 | 194 | v197 | numeric | important: to share European culture (Q54D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57614 | 4 |
v198 | 195 | v198 | numeric | European Union enlargement (Q55) | {1.0: 'should go further', 10.0: 'has gone too far'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54139 | 10 |
v199 | 196 | v199 | numeric | environment: giving part of income (Q56A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57664 | 5 |
v200 | 197 | v200 | numeric | environment: too difficult for me to do much (Q56B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58012 | 5 |
v201 | 198 | v201 | numeric | environment: there are more important things to do (Q56C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58091 | 5 |
v202 | 199 | v202 | numeric | environment: no point unless others do the same (Q56D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58042 | 5 |
v203 | 200 | v203 | numeric | environment: environmental threats are exaggerated (Q56E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56348 | 5 |
v204 | 201 | v204 | numeric | protecting environment vs. economic growth (Q57) | {1.0: 'protecting the environment priority, even if slower economic growth and loss of jobs', 2.0: 'economic growth and creating jobs priority, even if environment suffers'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 3.0: 'other answer (code if volunteered only!)'} | True | 52224 | 2 |
v205 | 202 | v205 | numeric | government: public area under video surveillance (Q58A) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57923 | 4 |
v206 | 203 | v206 | numeric | government: monitor all information exchanged on the internet (Q58B) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57022 | 4 |
v207 | 204 | v207 | numeric | government: collect information about anyone without their knowledge (Q58C) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57470 | 4 |
v208 | 205 | v208 | numeric | how often do you follow politics: on television (Q59A) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59128 | 5 |
v209 | 206 | v209 | numeric | how often do you follow politics: on the radio (Q59B) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58924 | 5 |
v210 | 207 | v210 | numeric | how often do you follow politics: in the daily papers (Q59C) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58929 | 5 |
v211 | 208 | v211 | numeric | how often do you follow politics: on social media (Q59D) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58647 | 5 |
v212 | 209 | v212 | numeric | are you concerned with: people neighbourhood (Q60A) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58883 | 5 |
v213 | 210 | v213 | numeric | are you concerned with: people own region (Q60B) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58781 | 5 |
v214 | 211 | v214 | numeric | are you concerned with: fellow countrymen (Q60C) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58704 | 5 |
v215 | 212 | v215 | numeric | are you concerned with: Europeans (Q60D) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58212 | 5 |
v216 | 213 | v216 | numeric | are you concerned with: humankind (Q60E) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58144 | 5 |
v217 | 214 | v217 | numeric | are you concerned with: elderly people (Q61A) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59034 | 5 |
v218 | 215 | v218 | numeric | are you concerned with: unemployed people (Q61B) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58797 | 5 |
v219 | 216 | v219 | numeric | are you concerned with: immigrants (Q61C) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58379 | 5 |
v220 | 217 | v220 | numeric | are you concerned with: sick and disabled (Q61D) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58912 | 5 |
v221 | 218 | v221 | numeric | important: eliminating income inequalities (Q62A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57320 | 4 |
v222 | 219 | v222 | numeric | important: basic needs for all (Q62B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58247 | 4 |
v223 | 220 | v223 | numeric | important: recognizing people on merits (Q62C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57866 | 4 |
v224 | 221 | v224 | numeric | important: protecting against terrorism (Q62D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58215 | 4 |
v225 | 222 | v225 | numeric | sex respondent (Q63) | {1.0: 'male', 2.0: 'female'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59412 | 2 |
v226 | 223 | v226 | numeric | year of birth respondent (Q64) | {1937.0: '1937 and before'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 67 |
v227 | 224 | v227 | numeric | respondent born in [country] (Q65) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59367 | 2 |
v229 | 225 | v229 | numeric | year in which respondent came to live in [country] (Q67) | {1941.0: '1941 and before'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 4116 | 79 |
v230 | 226 | v230 | numeric | father born in [country] (Q68) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59042 | 2 |
v232 | 227 | v232 | numeric | mother born in [country] (Q70) | {1.0: 'yes', 2.0: 'no'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59218 | 2 |
v234 | 228 | v234 | numeric | current legal marital status respondent (Q72) | {1.0: 'married', 2.0: 'registered partnership', 3.0: 'widowed', 4.0: 'divorced', 5.0: 'separated', 6.0: 'never married and never registered partnership'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59020 | 6 |
v235 | 229 | v235 | numeric | lived with partner before marriage (Q73) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 43987 | 2 |
v236 | 230 | v236 | numeric | living with partner (Q74) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27301 | 2 |
v237 | 231 | v237 | numeric | having steady relationship (Q75) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 19888 | 2 |
v238 | 232 | v238 | numeric | do you live with your parents/parents in law (Q76) | {1.0: 'no', 2.0: 'yes, own parent(s)', 3.0: 'yes, parent(s) in law', 4.0: 'yes, both own parent(s) and parent(s) in law'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59001 | 4 |
v240 | 233 | v240 | numeric | number of people in household (Q78) | {1.0: 'I live alone', 6.0: '6 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58691 | 6 |
v241 | 234 | v241 | numeric | age of youngest person in household (Q79) | {80.0: '80 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 45983 | 81 |
v242 | 235 | v242 | numeric | age completed education respondent (Q80) | {7.0: '7 and younger', 70.0: '70 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 0.0: 'no formal education'} | True | 55834 | 64 |
v244 | 236 | v244 | numeric | paid employment/no paid employment (Q82) | {1.0: '30h a week or more', 2.0: 'less then 30h a week', 3.0: 'self employed', 4.0: 'military service', 5.0: 'retired/pensioned', 6.0: 'homemaker not otherwise employed', 7.0: 'student', 8.0: 'unemployed', 9.0: 'disabled', 10.0: 'other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59027 | 10 |
v245 | 237 | v245 | numeric | employment/self-employment: last job (Q83) | {1.0: 'employed', 2.0: 'self-employed'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'never had a paid job'} | True | 21903 | 2 |
v247 | 238 | v247 | numeric | respondent has/had how many employees (Q85) | {1.0: 'none', 2.0: '1-9', 3.0: '10-24', 4.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 5467 | 4 |
v248 | 239 | v248 | numeric | do/did you supervise someone (Q86) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 46098 | 2 |
v249 | 240 | v249 | numeric | employment sector you work/-ed for (Q87) | {1.0: 'government or public institution', 2.0: 'private business or industry', 3.0: 'private non-profit organization'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 43442 | 3 |
v250 | 241 | v250 | numeric | partner/spouse born in [country] (Q88) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35914 | 2 |
v253 | 242 | v253 | numeric | paid employment/no paid employment spouse/partner (Q90) | {1.0: '30h a week or more', 2.0: 'less then 30h a week', 3.0: 'self employed', 4.0: 'military service', 5.0: 'retired/pensioned', 6.0: 'homemaker not otherwise employed', 7.0: 'student', 8.0: 'unemployed', 9.0: 'disabled', 10.0: 'other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35752 | 10 |
v254 | 243 | v254 | numeric | employment/self-employment (spouse/partner): last job (Q91) | {1.0: 'employed', 2.0: 'self-employed'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'never had a paid job'} | True | 11543 | 2 |
v256 | 244 | v256 | numeric | spouse/partner has/had how many employees (Q93) | {1.0: 'none', 2.0: '1-9', 3.0: '10-24', 4.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3522 | 4 |
v257 | 245 | v257 | numeric | does/did spouse/partner supervise someone (Q94) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27871 | 2 |
v258 | 246 | v258 | numeric | how many people does she/he supervise (Q95) | {1.0: '1-9', 2.0: '10-24', 3.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 6562 | 3 |
v259 | 247 | v259 | numeric | respondent experienced unemployment longer than 3 months (Q96) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58024 | 2 |
v260 | 248 | v260 | numeric | dependency on social security during last 5 years respondent (Q97) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58561 | 2 |
v261 | 249 | v261 | nominal | households total net income (Q98) (standardized) | {1.0: 'A - 1st decile', 2.0: 'B - 2nd decile', 3.0: 'C - 3rd decile', 4.0: 'D - 4th decile', 5.0: 'E - 5th decile', 6.0: 'F - 6th decile', 7.0: 'G - 7th decile', 8.0: 'H - 8th decile', 9.0: 'I - 9th decile', 10.0: 'J - 10th decile'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50698 | 10 |
v264 | 250 | v264 | numeric | at age 14, was father employed (Q101) | {1.0: 'yes employed', 2.0: 'yes self employed', 3.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55049 | 3 |
v265 | 251 | v265 | numeric | at age 14, was mother employed (Q102) | {1.0: 'yes employed', 2.0: 'yes self employed', 3.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56942 | 3 |
v266 | 252 | v266 | numeric | at age 14, occupational group of your parent (main earner) (Q103) | {1.0: 'professional and technical (for example: doctor, teacher, engineer, artist, accountant, nurse)', 2.0: 'higher administrative (for example: banker, executive in big business, high government official, union official)', 3.0: 'clerical (for example: secretary, clerk, office manager, civil servant, bookkeeper)', 4.0: 'sales (for example: sales manager, shop owner, shop assistant, insurance agent, buyer)', 5.0: 'service (for example: restaurant owner, police officer, waitress, barber, caretaker)', 6.0: 'skilled worker (for example: foreman, motor mechanic, printer, seamstress, tool and die maker, electrician)', 7.0: 'semi-skilled worker (for example: bricklayer, bus driver, cannery worker, carpenter, sheet metal worker, baker)', 8.0: 'unskilled worker (for example: laborer, porter, unskilled factory worker, cleaner)', 9.0: 'farm worker (for example: farm laborer, tractor driver)', 10.0: 'farm proprietor, farm manager'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 66.0: 'does not apply to me (spontaneous)'} | True | 55344 | 10 |
v267 | 253 | v267 | numeric | at age 14, mother liked to read books (Q104A) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56398 | 4 |
v268 | 254 | v268 | numeric | at age 14, discussed politics with mother (Q104B) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 57142 | 4 |
v269 | 255 | v269 | numeric | at age 14, mother liked to follow the news (Q104C) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56312 | 4 |
v270 | 256 | v270 | numeric | at age 14, parent(s) had problems making ends meet (Q104D) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56977 | 4 |
v271 | 257 | v271 | numeric | at age 14, father liked to read books (Q104E) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 54376 | 4 |
v272 | 258 | v272 | numeric | at age 14, discussed politics with father (Q104F) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55219 | 4 |
v273 | 259 | v273 | numeric | at age 14, father liked to follow the news (Q104G) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 54635 | 4 |
v274 | 260 | v274 | numeric | at age 14, parent(s) had problems replacing broken things (Q104H) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55792 | 4 |
v277 | 261 | v277 | numeric | date of interview (Q107) | {} | {-4.0: 'item not included', -2.0: 'no answer'} | True | 57588 | 951 |
v280 | 262 | v280 | numeric | interest of respondent during interview (Q109) | {1.0: 'very interested', 2.0: 'somewhat interested', 3.0: 'not very interested'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54488 | 3 |
v282 | 263 | v282 | numeric | interviewer number (Q111) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -5.0: 'other missing', -4.0: 'item not included'} | True | 51092 | 1989 |
We see that the subset of the complete survey does not contain any administrative columns anymore, just as desired. The type of most variables is numeric
. The reason is that a lot of SPSS users often label ordinal variables as scale
type variables, because it is the type providing most freedom in SPSS. From a statistical point of view, this might often be correct. The tangle library interprets scale variables as numeric - that’s the best we can do without further knowledge about the variables.
If one investigates the variables in more detail, one can see that nearly all variables labeled as numeric are actually of ordinal type. Therefore, we change all numeric types to 'ordinal'
and correct the few exceptions afterward:
questions.set_variable_types('ordinal', lambda x: x.type=='numeric' and len(x.valid_values)>0)
questions.set_variable_types('numeric', ['v226', 'v229', 'v241', 'v242'])
questions.set_variable_types('nominal', [f'v{i}' for i in range(108,115)] + ['v234','v266','v253','v249','v244','v232'])
Internally, the variable info is checked for usability every time we change something.
Let’s see how the variable info looks now. We only show variables that are still marked as not usable.
Like above, this is done by specifying a ColumnSelection
, again by a small lambda
expression:
styled_df(questions.variable_info(lambda v: not v.is_usable))
id | name | type | label | valid_values | invalid_values | is_usable | num_valid_answers | num_unique_answers | |
---|---|---|---|---|---|---|---|---|---|
v38 | 0 | v38 | ordinal | how much control over your life (Q9) | {1.0: 'none at all', 10.0: 'a great deal'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58550 | 10 |
v39 | 1 | v39 | ordinal | how satisfied are you with your life (Q10) | {1.0: 'dissatisfied', 10.0: 'satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 59122 | 10 |
v63 | 2 | v63 | ordinal | how important is God in your life (Q21) | {1.0: 'not at all important', 10.0: 'very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57947 | 10 |
v102 | 3 | v102 | ordinal | political view: left-right (Q31) | {1.0: 'the left', 10.0: 'the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 47723 | 10 |
v103 | 4 | v103 | ordinal | individual vs. state responsibility for providing (Q32A) | {1.0: 'individual responsibility', 10.0: 'state responsibility'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58214 | 10 |
v104 | 5 | v104 | ordinal | take any job vs. right to refuse job when unemployed (Q32B) | {1.0: 'unemployed take any job', 10.0: 'unemployed right to refuse a job'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58066 | 10 |
v105 | 6 | v105 | ordinal | competition is good vs. harmful (Q32C) | {1.0: 'competition good', 10.0: 'competition harmful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57516 | 10 |
v106 | 7 | v106 | ordinal | equalize incomes vs. incentives for individual effort (Q32D) | {1.0: 'incomes more equal', 10.0: 'incentives to individual efforts'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58103 | 10 |
v107 | 8 | v107 | ordinal | private vs. government ownership business (Q32E) | {1.0: 'private ownership increased', 10.0: 'government ownership increased'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 54991 | 10 |
v133 | 9 | v133 | ordinal | democracy: governments tax the rich and subsidize the poor (Q39A) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 52980 | 11 |
v134 | 10 | v134 | ordinal | democracy: religious authorities interpret the laws (Q39B) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 51892 | 11 |
v135 | 11 | v135 | ordinal | democracy: people choose their leaders in free elections (Q39C) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 54015 | 11 |
v136 | 12 | v136 | ordinal | democracy: people receive state aid for unemployment (Q39D) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 53977 | 11 |
v137 | 13 | v137 | ordinal | democracy: the army takes over when government is incompetent (Q39E) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 51896 | 11 |
v138 | 14 | v138 | ordinal | democracy: civil rights protect people from state oppression (Q39F) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 52504 | 11 |
v139 | 15 | v139 | ordinal | democracy: the state makes people’s incomes equal (Q39G) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 53050 | 11 |
v140 | 16 | v140 | ordinal | democracy: people obey their rulers (Q39H) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 53068 | 11 |
v141 | 17 | v141 | ordinal | democracy: women have the same rights as men (Q39I) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 54426 | 11 |
v142 | 18 | v142 | ordinal | importance of democracy (Q40) | {1.0: 'not at all important', 10.0: 'absolutely important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58125 | 10 |
v143 | 19 | v143 | ordinal | democracy in own country (Q41) | {1.0: 'not at all democratic', 10.0: 'completely democratic'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57214 | 10 |
v144 | 20 | v144 | ordinal | satisfaction political system (Q42) | {1.0: 'not satisfied at all', 10.0: 'completely satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57530 | 10 |
v149 | 21 | v149 | ordinal | do you justify: claiming state benefits (Q44A) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58240 | 10 |
v150 | 22 | v150 | ordinal | do you justify: cheating on tax (Q44B) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58511 | 10 |
v151 | 23 | v151 | ordinal | do you justify: taking soft drugs (Q44C) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58442 | 10 |
v152 | 24 | v152 | ordinal | do you justify: accepting a bribe (Q44D) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58723 | 10 |
v153 | 25 | v153 | ordinal | do you justify: homosexuality (Q44E) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56952 | 10 |
v154 | 26 | v154 | ordinal | do you justify: abortion (Q44F) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57277 | 10 |
v155 | 27 | v155 | ordinal | do you justify: divorce (Q44G) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57903 | 10 |
v156 | 28 | v156 | ordinal | do you justify: euthanasia (Q44H) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56185 | 10 |
v157 | 29 | v157 | ordinal | do you justify: suicide (Q44I) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56705 | 10 |
v158 | 30 | v158 | ordinal | do you justify: having casual sex (Q44J) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57033 | 10 |
v159 | 31 | v159 | ordinal | do you justify: avoiding a fare on public transport (Q44K) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58559 | 10 |
v160 | 32 | v160 | ordinal | do you justify: prostitution (Q44L) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57709 | 10 |
v161 | 33 | v161 | ordinal | do you justify: artificial insemination or in-vitro fertilization (Q44M) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56800 | 10 |
v162 | 34 | v162 | ordinal | do you justify: political violence (Q44N) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58009 | 10 |
v163 | 35 | v163 | ordinal | do you justify: death penalty (Q44O) | {1.0: 'never', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57123 | 10 |
v185 | 36 | v185 | ordinal | immigrants take away jobs from [nationality] (Q52A) | {1.0: 'take away', 10.0: 'do not take away'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 57389 | 10 |
v186 | 37 | v186 | ordinal | immigrants increase crime problems (Q52B) | {1.0: 'make it worse', 10.0: 'do not make it worse'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56679 | 10 |
v187 | 38 | v187 | ordinal | immigrants are a strain on welfare system (Q52C) | {1.0: 'are a strain', 10.0: 'are not a strain'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 56578 | 10 |
v188 | 39 | v188 | ordinal | better if immigrants maintain/not maintain own customs (Q52D) | {1.0: 'maintain distinct customs and traditions', 10.0: 'do not maintain distinct customs and traditions'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 55706 | 10 |
v198 | 40 | v198 | ordinal | European Union enlargement (Q55) | {1.0: 'should go further', 10.0: 'has gone too far'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 54139 | 10 |
v240 | 41 | v240 | ordinal | number of people in household (Q78) | {1.0: 'I live alone', 6.0: '6 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | False | 58691 | 6 |
We observe another small problem seen often in the metadata of survey data: Many ordinal variables only have labels for the minimal and the maximal answer. This is perfectly fine for a manual survey analysis, but the tangle analysis likes to know the complete list of possible values in these cases. Fortunately, this can be corrected very easily: We simply infer the lists from the data:
questions.guess_variable_value_lists(lambda v: not v.is_usable)
Our survey contains a bunch of binary variables. Binary variables are a special case: obviously they are nominal variables, but sometimes it makes sense to interpret them as ordinal variables. For aesthetic reasons, let’s change their types to ‘binary’ (internally it does not make a difference if they are typed as nominal, ordinal or binary):
questions.set_variable_types('binary', lambda v: len(v.valid_values)==2)
Ok, let’s have a final look at the survey’s fully configured variable info:
styled_df(questions.variable_info())
id | name | type | label | valid_values | invalid_values | is_usable | num_valid_answers | num_unique_answers | |
---|---|---|---|---|---|---|---|---|---|
v1 | 0 | v1 | ordinal | how important in your life: work (Q1A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58727 | 4 |
v2 | 1 | v2 | ordinal | how important in your life: family (Q1B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59221 | 4 |
v3 | 2 | v3 | ordinal | how important in your life: friends and acquaintances (Q1C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59215 | 4 |
v4 | 3 | v4 | ordinal | how important in your life: leisure time (Q1D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59032 | 4 |
v5 | 4 | v5 | ordinal | how important in your life: politics (Q1E) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58764 | 4 |
v6 | 5 | v6 | ordinal | how important in your life: religion (Q1F) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58622 | 4 |
v7 | 6 | v7 | ordinal | taking all things together how happy are you (Q2) | {1.0: 'very happy', 2.0: 'quite happy', 3.0: 'not very happy', 4.0: 'not at all happy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58707 | 4 |
v8 | 7 | v8 | ordinal | describe your state of health these days (Q3) | {1.0: 'very good', 2.0: 'good', 3.0: 'fair', 4.0: 'poor', 5.0: 'very poor'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59304 | 5 |
v9 | 8 | v9 | binary | do you belong to: religious organization (Q4A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58333 | 2 |
v10 | 9 | v10 | binary | do you belong to: cultural activities (Q4B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58284 | 2 |
v11 | 10 | v11 | binary | do you belong to: trade unions (Q4C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58269 | 2 |
v12 | 11 | v12 | binary | do you belong to: political parties/groups (Q4D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58243 | 2 |
v13 | 12 | v13 | binary | do you belong to: environment, ecology, animal rights (Q4E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58271 | 2 |
v14 | 13 | v14 | binary | do you belong to: professional associations (Q4F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58223 | 2 |
v15 | 14 | v15 | binary | do you belong to: sports/recreation (Q4G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58374 | 2 |
v16 | 15 | v16 | binary | do you belong to: charitable/humanitarian organization (Q4H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58259 | 2 |
v17 | 16 | v17 | binary | do you belong to: consumer organization (Q4I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58181 | 2 |
v18 | 17 | v18 | binary | do you belong to: self-help group, mutual aid group (Q4J) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58176 | 2 |
v19 | 18 | v19 | binary | do you belong to: other groups (Q4K) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57728 | 2 |
v20 | 19 | v20 | binary | do you belong to: none (spontaneous) (Q4) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53185 | 2 |
v21 | 20 | v21 | binary | did you do voluntary work in the last 6 months (Q5) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58986 | 2 |
v22 | 21 | v22 | binary | dont like as neighbours: people of different race (Q6A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57084 | 2 |
v23 | 22 | v23 | binary | dont like as neighbours: heavy drinkers (Q6B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58075 | 2 |
v24 | 23 | v24 | binary | dont like as neighbours: immigrants/foreign workers (Q6C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56520 | 2 |
v25 | 24 | v25 | binary | dont like as neighbours: drug addicts (Q6D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58247 | 2 |
v26 | 25 | v26 | binary | dont like as neighbours: homosexuals (Q6E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57513 | 2 |
v27 | 26 | v27 | binary | dont like as neighbours: Christians (optional in countries with Christian majority) (Q6F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49003 | 2 |
v28 | 27 | v28 | binary | dont like as neighbours: Muslims (optional in countries with Muslim majority) (Q6G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55587 | 2 |
v29 | 28 | v29 | binary | dont like as neighbours: Jews (optional) (Q6H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57061 | 2 |
v30 | 29 | v30 | binary | dont like as neighbours: Gypsies (optional) (Q6I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57288 | 2 |
v31 | 30 | v31 | binary | people can be trusted/can't be too careful (Q7) | {1.0: 'most people can be trusted', 2.0: 'can not be too careful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58083 | 2 |
v32 | 31 | v32 | ordinal | how much you trust: your family (Q8A) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59178 | 4 |
v33 | 32 | v33 | ordinal | how much you trust: people in your neighborhood (Q8B) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58616 | 4 |
v34 | 33 | v34 | ordinal | how much you trust: people you know personally (Q8C) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58981 | 4 |
v35 | 34 | v35 | ordinal | how much you trust: people you meet for the first time (Q8D) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57969 | 4 |
v36 | 35 | v36 | ordinal | how much you trust: people of another religion (Q8E) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54785 | 4 |
v37 | 36 | v37 | ordinal | how much you trust: people of another nationality (Q8F) | {1.0: 'trust completely', 2.0: 'trust somewhat', 3.0: 'do not trust very much', 4.0: 'do not trust at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55087 | 4 |
v38 | 37 | v38 | ordinal | how much control over your life (Q9) | {1.0: 'none at all', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'a great deal'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58550 | 10 |
v39 | 38 | v39 | ordinal | how satisfied are you with your life (Q10) | {1.0: 'dissatisfied', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59122 | 10 |
v40 | 39 | v40 | binary | important in a job: good pay (Q11A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58740 | 2 |
v41 | 40 | v41 | binary | important in a job: good hours (Q11B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58538 | 2 |
v42 | 41 | v42 | binary | important in a job: opportunity to use initiative (Q11C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57902 | 2 |
v43 | 42 | v43 | binary | important in a job: generous holidays (Q11D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58007 | 2 |
v44 | 43 | v44 | binary | important in a job: achieving something (Q11E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58218 | 2 |
v45 | 44 | v45 | binary | important in a job: responsible job (Q11F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57965 | 2 |
v46 | 45 | v46 | ordinal | job needed to develop talents (Q12A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58777 | 5 |
v47 | 46 | v47 | ordinal | humiliating receiving money without working (Q12B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58515 | 5 |
v48 | 47 | v48 | ordinal | people turn lazy not working (Q12C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58687 | 5 |
v49 | 48 | v49 | ordinal | work is a duty towards society (Q12D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58714 | 5 |
v50 | 49 | v50 | ordinal | work comes always first (Q12E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58820 | 5 |
v51 | 50 | v51 | binary | do you belong to a religious denomination (Q13) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58984 | 2 |
v52 | 51 | v52 | nominal | which religious denomination do you belong to (Q13a) (harmonized) | {1.0: 'Roman catholic', 2.0: 'Protestant', 3.0: 'Free church/Non-conformist/Evangelical', 4.0: 'Jew', 5.0: 'Muslim', 6.0: 'Hindu', 7.0: 'Buddhist', 8.0: 'Orthodox', 9.0: 'Other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 41037 | 9 |
v53 | 52 | v53 | binary | did you belong to a religious denomination (Q14) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 18919 | 2 |
v54 | 53 | v54 | ordinal | how often attend religious services (Q15) | {1.0: 'more than once week', 2.0: 'once a week', 3.0: 'once a month', 4.0: 'only on specific holy days', 5.0: 'once a year', 6.0: 'less often', 7.0: 'never, practically never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58851 | 7 |
v55 | 54 | v55 | ordinal | how often attend religious services 12 years old (Q16) | {1.0: 'more than once week', 2.0: 'once a week', 3.0: 'once a month', 4.0: 'only on specific holy days', 5.0: 'once a year', 6.0: 'less often', 7.0: 'never, practically never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57551 | 7 |
v56 | 55 | v56 | ordinal | are you a religious person (Q17) | {1.0: 'a religious person', 2.0: 'not a religious person', 3.0: 'a convinced atheist'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57082 | 3 |
v57 | 56 | v57 | binary | do you believe in: God (Q18A) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55894 | 2 |
v58 | 57 | v58 | binary | do you believe in: life after death (Q18B) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51186 | 2 |
v59 | 58 | v59 | binary | do you believe in: hell (Q18C) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51868 | 2 |
v60 | 59 | v60 | binary | do you believe in: heaven (Q18D) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51899 | 2 |
v61 | 60 | v61 | binary | do you believe in: re-incarnation (Q19) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52299 | 2 |
v62 | 61 | v62 | ordinal | which statement closest to your beliefs (Q20) | {1.0: 'personal God', 2.0: 'spirit or life force', 3.0: "don't know what to think", 4.0: 'no spirit, God or life force'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57497 | 4 |
v63 | 62 | v63 | ordinal | how important is God in your life (Q21) | {1.0: 'not at all important', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57947 | 10 |
v64 | 63 | v64 | ordinal | how often do you pray outside religious services (Q22) | {1.0: 'every day', 2.0: 'more than once week', 3.0: 'once a week', 4.0: 'at least once a month', 5.0: 'several times a year', 6.0: 'less often', 7.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57882 | 7 |
v65 | 64 | v65 | ordinal | important in marriage: faithfulness (Q23A) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59138 | 3 |
v66 | 65 | v66 | ordinal | important in marriage: adequate income (Q23B) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59078 | 3 |
v67 | 66 | v67 | ordinal | important in marriage: good housing (Q23C) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59121 | 3 |
v68 | 67 | v68 | ordinal | important in marriage: share household chores (Q23D) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58887 | 3 |
v69 | 68 | v69 | ordinal | important in marriage: children (Q23E) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58676 | 3 |
v70 | 69 | v70 | ordinal | important in marriage: time for friends and personal hobbies (Q23F) | {1.0: 'very important', 2.0: 'rather important', 3.0: 'not very important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58814 | 3 |
v71 | 70 | v71 | binary | marriage is outdated (Q24) | {1.0: 'agree', 2.0: 'disagree'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57349 | 2 |
v72 | 71 | v72 | ordinal | child suffers with working mother (Q25A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58080 | 4 |
v73 | 72 | v73 | ordinal | women really want home and children (Q25B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57241 | 4 |
v74 | 73 | v74 | ordinal | family life suffers when woman has full-time job (Q25C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58055 | 4 |
v75 | 74 | v75 | ordinal | man's job is to earn money; woman's job is to look after home and family (Q25D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58466 | 4 |
v76 | 75 | v76 | ordinal | men make better political leaders than women (Q25E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56994 | 4 |
v77 | 76 | v77 | ordinal | university education more important for a boy than for a girl (Q25F) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58183 | 4 |
v78 | 77 | v78 | ordinal | men make better business executives than women (Q25G) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57158 | 4 |
v79 | 78 | v79 | ordinal | one of main goals in life has been to make my parents proud (Q25H) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'disagree', 4.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56622 | 4 |
v80 | 79 | v80 | ordinal | jobs are scarce: giving...(nation) priority (Q26A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58633 | 5 |
v81 | 80 | v81 | ordinal | jobs are scarce: giving men priority (Q26B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58695 | 5 |
v82 | 81 | v82 | ordinal | homosexual couples - as good parents as other couples (Q27A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54764 | 5 |
v83 | 82 | v83 | ordinal | duty towards society to have children (Q27B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58491 | 5 |
v84 | 83 | v84 | ordinal | It is childs duty to provide long-term care for parents (Q27C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58678 | 5 |
v85 | 84 | v85 | binary | learn children at home: good manners (Q28A) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58798 | 2 |
v86 | 85 | v86 | binary | learn children at home: independence (Q28B) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58627 | 2 |
v87 | 86 | v87 | binary | learn children at home: hard work (Q28C) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58708 | 2 |
v88 | 87 | v88 | binary | learn children at home: feeling of responsibility (Q28D) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58729 | 2 |
v89 | 88 | v89 | binary | learn children at home: imagination (Q28E) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58160 | 2 |
v90 | 89 | v90 | binary | learn children at home: tolerance and respect (Q28F) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58560 | 2 |
v91 | 90 | v91 | binary | learn children at home: thrift, saving money and things (Q28G) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58432 | 2 |
v92 | 91 | v92 | binary | learn children at home: determination, perseverance (Q28H) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58376 | 2 |
v93 | 92 | v93 | binary | learn children at home: religious faith (Q28I) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58124 | 2 |
v94 | 93 | v94 | binary | learn children at home: unselfishness (Q28J) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58190 | 2 |
v95 | 94 | v95 | binary | learn children at home: obedience (Q28K) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58189 | 2 |
v96 | 95 | v96 | binary | learn children at home: none (spontaneous) (Q28) | {1.0: 'mentioned', 2.0: 'not mentioned'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53671 | 2 |
v97 | 96 | v97 | ordinal | how interested are you in politics (Q29) | {1.0: 'very interested', 2.0: 'somewhat interested', 3.0: 'not very interested', 4.0: 'not at all interested'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59132 | 4 |
v98 | 97 | v98 | ordinal | political action: signing a petition (Q30A) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57002 | 3 |
v99 | 98 | v99 | ordinal | political action: joining in boycotts (Q30B) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55875 | 3 |
v100 | 99 | v100 | ordinal | political action: attending lawful demonstrations (Q30C) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56622 | 3 |
v101 | 100 | v101 | ordinal | political action: joining unofficial strikes (Q30D) | {1.0: 'have done', 2.0: 'might do', 3.0: 'would never do'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55474 | 3 |
v102 | 101 | v102 | ordinal | political view: left-right (Q31) | {1.0: 'the left', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47723 | 10 |
v103 | 102 | v103 | ordinal | individual vs. state responsibility for providing (Q32A) | {1.0: 'individual responsibility', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'state responsibility'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58214 | 10 |
v104 | 103 | v104 | ordinal | take any job vs. right to refuse job when unemployed (Q32B) | {1.0: 'unemployed take any job', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'unemployed right to refuse a job'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58066 | 10 |
v105 | 104 | v105 | ordinal | competition is good vs. harmful (Q32C) | {1.0: 'competition good', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'competition harmful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57516 | 10 |
v106 | 105 | v106 | ordinal | equalize incomes vs. incentives for individual effort (Q32D) | {1.0: 'incomes more equal', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'incentives to individual efforts'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58103 | 10 |
v107 | 106 | v107 | ordinal | private vs. government ownership business (Q32E) | {1.0: 'private ownership increased', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'government ownership increased'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54991 | 10 |
v108 | 107 | v108 | nominal | aims of this country: most important (Q33) | {1.0: 'high level of economic growth', 2.0: 'strong defense forces', 3.0: 'more say at their jobs/ in communities', 4.0: 'make cities/countryside more beautiful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57503 | 4 |
v109 | 108 | v109 | nominal | aims of this country: 2nd most important (Q33a) | {1.0: 'high level of economic growth', 2.0: 'strong defense forces', 3.0: 'more say at their jobs/ in communities', 4.0: 'make cities/countryside more beautiful'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56342 | 4 |
v110 | 109 | v110 | nominal | aims of respondent: most important (Q34) | {1.0: 'maintaining order in nation', 2.0: 'more say in important government decisions', 3.0: 'fighting rising prices', 4.0: 'protect freedom of speech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58066 | 4 |
v111 | 110 | v111 | nominal | aims of respondent: 2nd most important (Q35) | {1.0: 'maintaining order in nation', 2.0: 'more say in important government decisions', 3.0: 'fighting rising prices', 4.0: 'protect freedom of speech'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57178 | 4 |
v112 | 111 | v112 | binary | are you willing to fight for country (Q36) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52718 | 2 |
v113 | 112 | v113 | nominal | good/bad: decrease work importance (Q37A) | {1.0: 'good', 2.0: 'bad', 3.0: "don't mind"} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56028 | 3 |
v114 | 113 | v114 | nominal | good/bad: greater respect for authority (Q37B) | {1.0: 'good', 2.0: 'bad', 3.0: "don't mind"} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55280 | 3 |
v115 | 114 | v115 | ordinal | how much confidence in: church (Q38A) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57455 | 4 |
v116 | 115 | v116 | ordinal | how much confidence in: armed forces (Q38B) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56006 | 4 |
v117 | 116 | v117 | ordinal | how much confidence in: education system (Q38C) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58159 | 4 |
v118 | 117 | v118 | ordinal | how much confidence in: the press (Q38D) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58072 | 4 |
v119 | 118 | v119 | ordinal | how much confidence in: trade unions (Q38E) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54191 | 4 |
v120 | 119 | v120 | ordinal | how much confidence in: the police (Q38F) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58495 | 4 |
v121 | 120 | v121 | ordinal | how much confidence in: parliament (Q38G) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57366 | 4 |
v122 | 121 | v122 | ordinal | how much confidence in: civil service (Q38H) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57228 | 4 |
v123 | 122 | v123 | ordinal | how much confidence in: social security system (Q38I) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57294 | 4 |
v124 | 123 | v124 | ordinal | how much confidence in: European Union (Q38J) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55705 | 4 |
v125 | 124 | v125 | ordinal | how much confidence in: United Nations Organization (Q38K) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54168 | 4 |
v126 | 125 | v126 | ordinal | how much confidence in: health care system (Q38L) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58585 | 4 |
v127 | 126 | v127 | ordinal | how much confidence in: justice system (Q38M) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57092 | 4 |
v128 | 127 | v128 | ordinal | how much confidence in: major companies (Q38N) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54645 | 4 |
v129 | 128 | v129 | ordinal | how much confidence in: environmental organizations (Q38O) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55582 | 4 |
v130 | 129 | v130 | ordinal | how much confidence in: political parties (Q38P) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56884 | 4 |
v131 | 130 | v131 | ordinal | how much confidence in: government (Q38Q) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57506 | 4 |
v132 | 131 | v132 | ordinal | how much confidence in: social media (Q38R) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54341 | 4 |
v133 | 132 | v133 | ordinal | democracy: governments tax the rich and subsidize the poor (Q39A) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52980 | 11 |
v134 | 133 | v134 | ordinal | democracy: religious authorities interpret the laws (Q39B) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51892 | 11 |
v135 | 134 | v135 | ordinal | democracy: people choose their leaders in free elections (Q39C) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54015 | 11 |
v136 | 135 | v136 | ordinal | democracy: people receive state aid for unemployment (Q39D) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53977 | 11 |
v137 | 136 | v137 | ordinal | democracy: the army takes over when government is incompetent (Q39E) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51896 | 11 |
v138 | 137 | v138 | ordinal | democracy: civil rights protect people from state oppression (Q39F) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52504 | 11 |
v139 | 138 | v139 | ordinal | democracy: the state makes people’s incomes equal (Q39G) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53050 | 11 |
v140 | 139 | v140 | ordinal | democracy: people obey their rulers (Q39H) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 53068 | 11 |
v141 | 140 | v141 | ordinal | democracy: women have the same rights as men (Q39I) | {0.0: 'it is against democracy [DO NOT READ OUT]', 1.0: 'not at all an essential characteristic of democracy', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'an essential characteristic of democracy'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54426 | 11 |
v142 | 141 | v142 | ordinal | importance of democracy (Q40) | {1.0: 'not at all important', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'absolutely important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58125 | 10 |
v143 | 142 | v143 | ordinal | democracy in own country (Q41) | {1.0: 'not at all democratic', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'completely democratic'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57214 | 10 |
v144 | 143 | v144 | ordinal | satisfaction political system (Q42) | {1.0: 'not satisfied at all', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'completely satisfied'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57530 | 10 |
v145 | 144 | v145 | ordinal | political system: strong leader (Q43A) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55374 | 4 |
v146 | 145 | v146 | ordinal | political system: experts making decisions (Q43B) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54313 | 4 |
v147 | 146 | v147 | ordinal | political system: the army ruling (Q43C) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55409 | 4 |
v148 | 147 | v148 | ordinal | political system: democratic (Q43D) | {1.0: 'very good', 2.0: 'fairly good', 3.0: 'fairly bad', 4.0: 'very bad'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56245 | 4 |
v149 | 148 | v149 | ordinal | do you justify: claiming state benefits (Q44A) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58240 | 10 |
v150 | 149 | v150 | ordinal | do you justify: cheating on tax (Q44B) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58511 | 10 |
v151 | 150 | v151 | ordinal | do you justify: taking soft drugs (Q44C) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58442 | 10 |
v152 | 151 | v152 | ordinal | do you justify: accepting a bribe (Q44D) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58723 | 10 |
v153 | 152 | v153 | ordinal | do you justify: homosexuality (Q44E) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56952 | 10 |
v154 | 153 | v154 | ordinal | do you justify: abortion (Q44F) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57277 | 10 |
v155 | 154 | v155 | ordinal | do you justify: divorce (Q44G) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57903 | 10 |
v156 | 155 | v156 | ordinal | do you justify: euthanasia (Q44H) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56185 | 10 |
v157 | 156 | v157 | ordinal | do you justify: suicide (Q44I) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56705 | 10 |
v158 | 157 | v158 | ordinal | do you justify: having casual sex (Q44J) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57033 | 10 |
v159 | 158 | v159 | ordinal | do you justify: avoiding a fare on public transport (Q44K) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58559 | 10 |
v160 | 159 | v160 | ordinal | do you justify: prostitution (Q44L) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57709 | 10 |
v161 | 160 | v161 | ordinal | do you justify: artificial insemination or in-vitro fertilization (Q44M) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56800 | 10 |
v162 | 161 | v162 | ordinal | do you justify: political violence (Q44N) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58009 | 10 |
v163 | 162 | v163 | ordinal | do you justify: death penalty (Q44O) | {1.0: 'never', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'always'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57123 | 10 |
v164 | 163 | v164 | ordinal | how close do you feel: to own town/city (Q45A) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58942 | 4 |
v165 | 164 | v165 | ordinal | how close do you feel: to your [county, region, district] (Q45B) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58786 | 4 |
v166 | 165 | v166 | ordinal | how close do you feel: to [country] (Q45C) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58857 | 4 |
v167 | 166 | v167 | ordinal | how close do you feel: to [continent] (Q45D) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58110 | 4 |
v168 | 167 | v168 | ordinal | how close do you feel: to world (Q45E) | {1.0: 'very close', 2.0: 'close', 3.0: 'not very close', 4.0: 'not close at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57393 | 4 |
v169 | 168 | v169 | binary | do you have... [country's] nationality (Q46) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59318 | 2 |
v170 | 169 | v170 | ordinal | how proud are you to be a ... [country] citizen (Q47) | {1.0: 'very proud', 2.0: 'quite proud', 3.0: 'not very proud', 4.0: 'not at all proud'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55885 | 4 |
v171 | 170 | v171 | ordinal | vote in elections: local level (Q48A) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 57525 | 3 |
v172 | 171 | v172 | ordinal | vote in elections: national level (Q48B) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 56952 | 3 |
v173 | 172 | v173 | ordinal | vote in elections: European level (Q48C) | {1.0: 'always', 2.0: 'usually', 3.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 7.0: 'not allowed to vote'} | True | 34242 | 3 |
v176 | 173 | v176 | ordinal | how often in country's elections: votes are counted fairly (Q50A) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 52270 | 4 |
v177 | 174 | v177 | ordinal | how often in country's elections: opposition candidates are prevented from running (Q50B) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 47740 | 4 |
v178 | 175 | v178 | ordinal | how often in country's elections: TV news favors the governing party (Q50C) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51081 | 4 |
v179 | 176 | v179 | ordinal | how often in country's elections: voters are bribed (Q50D) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49897 | 4 |
v180 | 177 | v180 | ordinal | how often in country's elections: journalists provide fair coverage of elections (Q50E) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 51685 | 4 |
v181 | 178 | v181 | ordinal | how often in country's elections: election officials are fair (Q50F) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50664 | 4 |
v182 | 179 | v182 | ordinal | how often in country's elections: rich people buy elections (Q50G) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 48126 | 4 |
v183 | 180 | v183 | ordinal | how often in country's elections: voters are threatened with violence at the polls (Q50H) | {1.0: 'very often', 2.0: 'fairly often', 3.0: 'not often', 4.0: 'not at all often'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 49341 | 4 |
v184 | 181 | v184 | ordinal | immigrants: impact on the development of [your country] (Q51) | {1.0: 'very bad', 2.0: 'quite bad', 3.0: 'neither good, nor bad', 4.0: 'quite good', 5.0: 'very good'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56958 | 5 |
v185 | 182 | v185 | ordinal | immigrants take away jobs from [nationality] (Q52A) | {1.0: 'take away', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'do not take away'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57389 | 10 |
v186 | 183 | v186 | ordinal | immigrants increase crime problems (Q52B) | {1.0: 'make it worse', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'do not make it worse'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56679 | 10 |
v187 | 184 | v187 | ordinal | immigrants are a strain on welfare system (Q52C) | {1.0: 'are a strain', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'are not a strain'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56578 | 10 |
v188 | 185 | v188 | ordinal | better if immigrants maintain/not maintain own customs (Q52D) | {1.0: 'maintain distinct customs and traditions', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'do not maintain distinct customs and traditions'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 55706 | 10 |
v189 | 186 | v189 | ordinal | important: to have been born in [country] (Q53A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58850 | 4 |
v190 | 187 | v190 | ordinal | important: to respect [country nationality] political institutions and laws (Q53B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58850 | 4 |
v191 | 188 | v191 | ordinal | important: to have [country nationality] ancestry (Q53C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58728 | 4 |
v192 | 189 | v192 | ordinal | important: to be able to speak [national language] (Q53D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59034 | 4 |
v193 | 190 | v193 | ordinal | important: to share [national] culture (Q53E) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58811 | 4 |
v194 | 191 | v194 | ordinal | important: to be born in Europe (Q54A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57741 | 4 |
v195 | 192 | v195 | ordinal | important: to have European ancestry (Q54B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57562 | 4 |
v196 | 193 | v196 | ordinal | important: to be a Christian (Q54C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57302 | 4 |
v197 | 194 | v197 | ordinal | important: to share European culture (Q54D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57614 | 4 |
v198 | 195 | v198 | ordinal | European Union enlargement (Q55) | {1.0: 'should go further', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6.0(*)', 7.0: '7.0(*)', 8.0: '8.0(*)', 9.0: '9.0(*)', 10.0: 'has gone too far'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54139 | 10 |
v199 | 196 | v199 | ordinal | environment: giving part of income (Q56A) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57664 | 5 |
v200 | 197 | v200 | ordinal | environment: too difficult for me to do much (Q56B) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58012 | 5 |
v201 | 198 | v201 | ordinal | environment: there are more important things to do (Q56C) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58091 | 5 |
v202 | 199 | v202 | ordinal | environment: no point unless others do the same (Q56D) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58042 | 5 |
v203 | 200 | v203 | ordinal | environment: environmental threats are exaggerated (Q56E) | {1.0: 'agree strongly', 2.0: 'agree', 3.0: 'neither agree nor disagree', 4.0: 'disagree', 5.0: 'disagree strongly'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 56348 | 5 |
v204 | 201 | v204 | binary | protecting environment vs. economic growth (Q57) | {1.0: 'protecting the environment priority, even if slower economic growth and loss of jobs', 2.0: 'economic growth and creating jobs priority, even if environment suffers'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 3.0: 'other answer (code if volunteered only!)'} | True | 52224 | 2 |
v205 | 202 | v205 | ordinal | government: public area under video surveillance (Q58A) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57923 | 4 |
v206 | 203 | v206 | ordinal | government: monitor all information exchanged on the internet (Q58B) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57022 | 4 |
v207 | 204 | v207 | ordinal | government: collect information about anyone without their knowledge (Q58C) | {1.0: 'definitely should have the right', 2.0: 'probably should have the right', 3.0: 'probably should not have the right', 4.0: 'definitely should not have the right'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57470 | 4 |
v208 | 205 | v208 | ordinal | how often do you follow politics: on television (Q59A) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59128 | 5 |
v209 | 206 | v209 | ordinal | how often do you follow politics: on the radio (Q59B) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58924 | 5 |
v210 | 207 | v210 | ordinal | how often do you follow politics: in the daily papers (Q59C) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58929 | 5 |
v211 | 208 | v211 | ordinal | how often do you follow politics: on social media (Q59D) | {1.0: 'every day', 2.0: 'several times a week', 3.0: 'once or twice a week', 4.0: 'less often', 5.0: 'never'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58647 | 5 |
v212 | 209 | v212 | ordinal | are you concerned with: people neighbourhood (Q60A) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58883 | 5 |
v213 | 210 | v213 | ordinal | are you concerned with: people own region (Q60B) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58781 | 5 |
v214 | 211 | v214 | ordinal | are you concerned with: fellow countrymen (Q60C) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58704 | 5 |
v215 | 212 | v215 | ordinal | are you concerned with: Europeans (Q60D) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58212 | 5 |
v216 | 213 | v216 | ordinal | are you concerned with: humankind (Q60E) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58144 | 5 |
v217 | 214 | v217 | ordinal | are you concerned with: elderly people (Q61A) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59034 | 5 |
v218 | 215 | v218 | ordinal | are you concerned with: unemployed people (Q61B) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58797 | 5 |
v219 | 216 | v219 | ordinal | are you concerned with: immigrants (Q61C) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58379 | 5 |
v220 | 217 | v220 | ordinal | are you concerned with: sick and disabled (Q61D) | {1.0: 'very much', 2.0: 'much', 3.0: 'to a certain extent', 4.0: 'not so much', 5.0: 'not at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58912 | 5 |
v221 | 218 | v221 | ordinal | important: eliminating income inequalities (Q62A) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57320 | 4 |
v222 | 219 | v222 | ordinal | important: basic needs for all (Q62B) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58247 | 4 |
v223 | 220 | v223 | ordinal | important: recognizing people on merits (Q62C) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 57866 | 4 |
v224 | 221 | v224 | ordinal | important: protecting against terrorism (Q62D) | {1.0: 'very important', 2.0: 'quite important', 3.0: 'not important', 4.0: 'not at all important'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58215 | 4 |
v225 | 222 | v225 | binary | sex respondent (Q63) | {1.0: 'male', 2.0: 'female'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59412 | 2 |
v226 | 223 | v226 | numeric | year of birth respondent (Q64) | {1937.0: '1937 and before'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59113 | 67 |
v227 | 224 | v227 | binary | respondent born in [country] (Q65) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59367 | 2 |
v229 | 225 | v229 | numeric | year in which respondent came to live in [country] (Q67) | {1941.0: '1941 and before'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 4116 | 79 |
v230 | 226 | v230 | binary | father born in [country] (Q68) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59042 | 2 |
v232 | 227 | v232 | binary | mother born in [country] (Q70) | {1.0: 'yes', 2.0: 'no'} | {-5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: "don't know"} | True | 59218 | 2 |
v234 | 228 | v234 | nominal | current legal marital status respondent (Q72) | {1.0: 'married', 2.0: 'registered partnership', 3.0: 'widowed', 4.0: 'divorced', 5.0: 'separated', 6.0: 'never married and never registered partnership'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59020 | 6 |
v235 | 229 | v235 | binary | lived with partner before marriage (Q73) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 43987 | 2 |
v236 | 230 | v236 | binary | living with partner (Q74) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27301 | 2 |
v237 | 231 | v237 | binary | having steady relationship (Q75) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 19888 | 2 |
v238 | 232 | v238 | ordinal | do you live with your parents/parents in law (Q76) | {1.0: 'no', 2.0: 'yes, own parent(s)', 3.0: 'yes, parent(s) in law', 4.0: 'yes, both own parent(s) and parent(s) in law'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59001 | 4 |
v240 | 233 | v240 | ordinal | number of people in household (Q78) | {1.0: 'I live alone', 2.0: '2.0(*)', 3.0: '3.0(*)', 4.0: '4.0(*)', 5.0: '5.0(*)', 6.0: '6 and more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58691 | 6 |
v241 | 234 | v241 | numeric | age of youngest person in household (Q79) | {80.0: '80 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 45983 | 81 |
v242 | 235 | v242 | binary | age completed education respondent (Q80) | {7.0: '7 and younger', 70.0: '70 and older'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 0.0: 'no formal education'} | False | 55834 | 64 |
v244 | 236 | v244 | nominal | paid employment/no paid employment (Q82) | {1.0: '30h a week or more', 2.0: 'less then 30h a week', 3.0: 'self employed', 4.0: 'military service', 5.0: 'retired/pensioned', 6.0: 'homemaker not otherwise employed', 7.0: 'student', 8.0: 'unemployed', 9.0: 'disabled', 10.0: 'other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 59027 | 10 |
v245 | 237 | v245 | binary | employment/self-employment: last job (Q83) | {1.0: 'employed', 2.0: 'self-employed'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'never had a paid job'} | True | 21903 | 2 |
v247 | 238 | v247 | ordinal | respondent has/had how many employees (Q85) | {1.0: 'none', 2.0: '1-9', 3.0: '10-24', 4.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 5467 | 4 |
v248 | 239 | v248 | binary | do/did you supervise someone (Q86) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 46098 | 2 |
v249 | 240 | v249 | nominal | employment sector you work/-ed for (Q87) | {1.0: 'government or public institution', 2.0: 'private business or industry', 3.0: 'private non-profit organization'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 43442 | 3 |
v250 | 241 | v250 | binary | partner/spouse born in [country] (Q88) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35914 | 2 |
v253 | 242 | v253 | nominal | paid employment/no paid employment spouse/partner (Q90) | {1.0: '30h a week or more', 2.0: 'less then 30h a week', 3.0: 'self employed', 4.0: 'military service', 5.0: 'retired/pensioned', 6.0: 'homemaker not otherwise employed', 7.0: 'student', 8.0: 'unemployed', 9.0: 'disabled', 10.0: 'other'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 35752 | 10 |
v254 | 243 | v254 | binary | employment/self-employment (spouse/partner): last job (Q91) | {1.0: 'employed', 2.0: 'self-employed'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'never had a paid job'} | True | 11543 | 2 |
v256 | 244 | v256 | ordinal | spouse/partner has/had how many employees (Q93) | {1.0: 'none', 2.0: '1-9', 3.0: '10-24', 4.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 3522 | 4 |
v257 | 245 | v257 | binary | does/did spouse/partner supervise someone (Q94) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 27871 | 2 |
v258 | 246 | v258 | ordinal | how many people does she/he supervise (Q95) | {1.0: '1-9', 2.0: '10-24', 3.0: '25 or more'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 6562 | 3 |
v259 | 247 | v259 | binary | respondent experienced unemployment longer than 3 months (Q96) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58024 | 2 |
v260 | 248 | v260 | binary | dependency on social security during last 5 years respondent (Q97) | {1.0: 'yes', 2.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 58561 | 2 |
v261 | 249 | v261 | nominal | households total net income (Q98) (standardized) | {1.0: 'A - 1st decile', 2.0: 'B - 2nd decile', 3.0: 'C - 3rd decile', 4.0: 'D - 4th decile', 5.0: 'E - 5th decile', 6.0: 'F - 6th decile', 7.0: 'G - 7th decile', 8.0: 'H - 8th decile', 9.0: 'I - 9th decile', 10.0: 'J - 10th decile'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 50698 | 10 |
v264 | 250 | v264 | ordinal | at age 14, was father employed (Q101) | {1.0: 'yes employed', 2.0: 'yes self employed', 3.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55049 | 3 |
v265 | 251 | v265 | ordinal | at age 14, was mother employed (Q102) | {1.0: 'yes employed', 2.0: 'yes self employed', 3.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56942 | 3 |
v266 | 252 | v266 | nominal | at age 14, occupational group of your parent (main earner) (Q103) | {1.0: 'professional and technical (for example: doctor, teacher, engineer, artist, accountant, nurse)', 2.0: 'higher administrative (for example: banker, executive in big business, high government official, union official)', 3.0: 'clerical (for example: secretary, clerk, office manager, civil servant, bookkeeper)', 4.0: 'sales (for example: sales manager, shop owner, shop assistant, insurance agent, buyer)', 5.0: 'service (for example: restaurant owner, police officer, waitress, barber, caretaker)', 6.0: 'skilled worker (for example: foreman, motor mechanic, printer, seamstress, tool and die maker, electrician)', 7.0: 'semi-skilled worker (for example: bricklayer, bus driver, cannery worker, carpenter, sheet metal worker, baker)', 8.0: 'unskilled worker (for example: laborer, porter, unskilled factory worker, cleaner)', 9.0: 'farm worker (for example: farm laborer, tractor driver)', 10.0: 'farm proprietor, farm manager'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 66.0: 'does not apply to me (spontaneous)'} | True | 55344 | 10 |
v267 | 253 | v267 | ordinal | at age 14, mother liked to read books (Q104A) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56398 | 4 |
v268 | 254 | v268 | ordinal | at age 14, discussed politics with mother (Q104B) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 57142 | 4 |
v269 | 255 | v269 | ordinal | at age 14, mother liked to follow the news (Q104C) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56312 | 4 |
v270 | 256 | v270 | ordinal | at age 14, parent(s) had problems making ends meet (Q104D) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 56977 | 4 |
v271 | 257 | v271 | ordinal | at age 14, father liked to read books (Q104E) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 54376 | 4 |
v272 | 258 | v272 | ordinal | at age 14, discussed politics with father (Q104F) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55219 | 4 |
v273 | 259 | v273 | ordinal | at age 14, father liked to follow the news (Q104G) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 54635 | 4 |
v274 | 260 | v274 | ordinal | at age 14, parent(s) had problems replacing broken things (Q104H) | {1.0: 'yes', 2.0: 'to some extent', 3.0: 'a little bit', 4.0: 'no'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know', 6.0: 'does not apply to me (spontaneous)'} | True | 55792 | 4 |
v277 | 261 | v277 | numeric | date of interview (Q107) | {} | {-4.0: 'item not included', -2.0: 'no answer'} | True | 57588 | 951 |
v280 | 262 | v280 | ordinal | interest of respondent during interview (Q109) | {1.0: 'very interested', 2.0: 'somewhat interested', 3.0: 'not very interested'} | {-10.0: 'multiple answers (Mail)', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 54488 | 3 |
v282 | 263 | v282 | numeric | interviewer number (Q111) | {} | {-9.0: 'no follow-up', -8.0: 'follow-up non response', -7.0: 'matrix not applied', -5.0: 'other missing', -4.0: 'item not included'} | True | 51092 | 1989 |
This looks nice! Soon we can search for tangles :-)
Please note: The configuration of a survey has to be done only once: A configured Survey
object can be saved to disk and loaded from disk using the functions save
and load
of the class Survey
. For other datasets the configuration might require more or less work than we have seen in this example. If you analyse data from your own study, it should be possible to set up the data in SPSS and use it directly in the tangle library with no or only a few modifications on the python side.
2. A simple tangle analysis#
Sometimes it makes sense to analyse tangles of features generated for the complete set of questions, but this can take quite some time and the results are not always easy to interpret. In this notebook, it is beneficial to extract a subset of the questions, i.e. a subset of the columns of the dataframe.
We select a subset of questions about the respondents’ level of trust in various institutions. These are questions that have their id
within the range from 114
to 131
, inclusive.
(Please note that the id
of a question is only valid inside a Survey
object: it is a local id
. If a subset of the questions is selected to build another instance of a Survey
, the local id
of a question might not be persistent.)
We can subset the survey, like above, with help of the function select_questions
. Afterward, we select a subset of the rows: We want to ignore all rows that contain missing or other invalid values. This is done in the same way as for the columns by the function select_rows
. After sub-setting the survey, we display the reduced variable info, again:
some_questions = questions.select_questions(range(114,132))
some_questions = some_questions.select_respondents(some_questions.complete_rows())
styled_df(some_questions.variable_info())
id | name | type | label | valid_values | invalid_values | is_usable | num_valid_answers | num_unique_answers | |
---|---|---|---|---|---|---|---|---|---|
v115 | 0 | v115 | ordinal | how much confidence in: church (Q38A) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v116 | 1 | v116 | ordinal | how much confidence in: armed forces (Q38B) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v117 | 2 | v117 | ordinal | how much confidence in: education system (Q38C) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v118 | 3 | v118 | ordinal | how much confidence in: the press (Q38D) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v119 | 4 | v119 | ordinal | how much confidence in: trade unions (Q38E) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v120 | 5 | v120 | ordinal | how much confidence in: the police (Q38F) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v121 | 6 | v121 | ordinal | how much confidence in: parliament (Q38G) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v122 | 7 | v122 | ordinal | how much confidence in: civil service (Q38H) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v123 | 8 | v123 | ordinal | how much confidence in: social security system (Q38I) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v124 | 9 | v124 | ordinal | how much confidence in: European Union (Q38J) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v125 | 10 | v125 | ordinal | how much confidence in: United Nations Organization (Q38K) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v126 | 11 | v126 | ordinal | how much confidence in: health care system (Q38L) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v127 | 12 | v127 | ordinal | how much confidence in: justice system (Q38M) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v128 | 13 | v128 | ordinal | how much confidence in: major companies (Q38N) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v129 | 14 | v129 | ordinal | how much confidence in: environmental organizations (Q38O) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v130 | 15 | v130 | ordinal | how much confidence in: political parties (Q38P) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v131 | 16 | v131 | ordinal | how much confidence in: government (Q38Q) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
v132 | 17 | v132 | ordinal | how much confidence in: social media (Q38R) | {1.0: 'a great deal', 2.0: 'quite a lot', 3.0: 'not very much', 4.0: 'none at all'} | {-10.0: 'multiple answers Mail', -9.0: 'no follow-up', -8.0: 'follow-up non response', -5.0: 'other missing', -4.0: 'item not included', -3.0: 'not applicable', -2.0: 'no answer', -1.0: 'dont know'} | True | 42710 | 4 |
We have 18 questions left, all are ordinal and take values from a great deal
(encoded as the numerical value 1
) to none at all
(encoded as the numerical value 4
). The numerical encoding is not like one would expect - a bigger number means less trust - but this comes from the raw SPSS data. This phenomenon can often be seen in survey data, so maybe it’s just a common way to encode ordinal categories in the social sciences. We could change it, but this is not really necessary. We just keep it in mind, so we are not confused by this later…
So, now it’s really time to try the tangle analysis!
The library provides a class SurveyTangles
that manages the tangle search and its results.
The most convenient way to start a tangle analysis is given by the static function SurveyTangles.search(..)
. This function sets up a SurveyTangles
object, initializes some important properties and starts a tangle search for a given agreement, i.e. the minimum intersection size allowed for all triples of a tangle’s specifications (please see the book for a detailed explanation of this parameter).
The function can be used in many different ways: you can find more information about the various parameters in the documentation.
There are two mandatory parameters: the Survey
of interest, and the agreement
.
Without knowing more about the data set, we first try setting the agreement parameter to 10% of the data size. We use another parameter to specify an order function. We want to order the features by the order function \((\text{O}2)\), a similarity based order function from the tangles book:
tangles = SurveyTangles.search(survey=some_questions, agreement=questions.num_respondents//10, order="O2", progress_callback=None)
That’s it! The result is stored in the SurveyTangles
object tangles
. Let’s inspect the result:
The library provides a function typical_answers()
that converts the specifications of each tangle back to typical answers of the survey questions. The function returns a table containing, in each row, the typical answers specified by the tangle corresponding to that row. For visual simplicity, we display the transpose of this table (so every column corresponds to a tangle):
styled_df(tangles.typical_answers().T, scrollable=False)
0 | |
---|---|
how much confidence in: church (Q38A)[v115] | [quite a lot; not very much] |
how much confidence in: armed forces (Q38B)[v116] | quite a lot |
how much confidence in: education system (Q38C)[v117] | quite a lot |
how much confidence in: the press (Q38D)[v118] | not very much |
how much confidence in: trade unions (Q38E)[v119] | not very much |
how much confidence in: the police (Q38F)[v120] | quite a lot |
how much confidence in: parliament (Q38G)[v121] | not very much |
how much confidence in: civil service (Q38H)[v122] | [quite a lot; not very much] |
how much confidence in: social security system (Q38I)[v123] | [quite a lot; not very much] |
how much confidence in: European Union (Q38J)[v124] | [quite a lot; not very much] |
how much confidence in: United Nations Organization (Q38K)[v125] | [quite a lot; not very much] |
how much confidence in: health care system (Q38L)[v126] | quite a lot |
how much confidence in: justice system (Q38M)[v127] | [quite a lot; not very much] |
how much confidence in: major companies (Q38N)[v128] | not very much |
how much confidence in: environmental organizations (Q38O)[v129] | [quite a lot; not very much] |
how much confidence in: political parties (Q38P)[v130] | not very much |
how much confidence in: government (Q38Q)[v131] | not very much |
how much confidence in: social media (Q38R)[v132] | not very much |
Ok, there seems to be only one typical way to answer these questions at this agreement level. It seems particularly typical to not trust political parties, the government, big companies, the press and some further institutions. Interestingly, the only positive answers are about trust in armed forces, education, the police and the health care system.
For some questions we don’t see a typical answer, but a range of typical answers. This happens, if not all features corresponding to a question could be specified for this agreement.
Let’s try to find more tangles by lowering the agreement parameter. We don’t have to start a new search from scratch, because the SurveyTangles
object still maintains a link to the algorithm (TangleSweep
) and a data structure (TangleSearchTree
) that allows to dynamically update the tangles.
The agreement can be changed by the function change_agreement
. If the agreement falls below the current lowest agreement, a tree update has to be done so that the algorithm is able to create valid tangle search results. Unfortunately, this can take a long time. To avoid triggering a time-consuming task by accident, the function change_agreement
contains a second parameter force_tree_update
, that has to be set to True
if the tree update should really be performed.
We choose a slightly lower agreement of questions.num_respondents//16
(using the ‘//’ operator for integer division):
tangles.change_agreement(questions.num_respondents//16, force_tree_update=True)
Let’s look at the results again. If we have more than just a few tangles, the situation can become quite confusing. Therefore, the function typical_answers
contains a few parameters to focus on interesting details.
For example, a helpful parameter is the flag extract_const_answers
. If it set to True
, the function returns a second dataframe containing all answers that are typical for all tangles and removes the corresponding columns in the typical answers dataframe.
Another helpful parameter, only_max_level_tangles
, allows us to focus only on complete tangles. We use both these options in the next cell:
answers,const_answers = tangles.typical_answers(only_max_level_tangles=True, extract_const_answers=True)
The answers shared by all tangles are:
styled_df(const_answers.T, scrollable=False)
0 | |
---|---|
how much confidence in: armed forces (Q38B)[v116] | quite a lot |
how much confidence in: education system (Q38C)[v117] | quite a lot |
how much confidence in: the press (Q38D)[v118] | not very much |
how much confidence in: trade unions (Q38E)[v119] | not very much |
how much confidence in: the police (Q38F)[v120] | quite a lot |
how much confidence in: parliament (Q38G)[v121] | not very much |
how much confidence in: health care system (Q38L)[v126] | quite a lot |
how much confidence in: major companies (Q38N)[v128] | not very much |
how much confidence in: political parties (Q38P)[v130] | not very much |
how much confidence in: government (Q38Q)[v131] | not very much |
how much confidence in: social media (Q38R)[v132] | not very much |
This means that, at this agreement level, the only typical way to answer these questions is as displayed in the table. The remaining typical answers given by each tangle are in the dataframe answers
. We display the transpose:
styled_df(answers.T, scrollable=False)
0 | 1 | 2 | |
---|---|---|---|
how much confidence in: church (Q38A)[v115] | quite a lot | not very much | not very much |
how much confidence in: civil service (Q38H)[v122] | quite a lot | quite a lot | not very much |
how much confidence in: social security system (Q38I)[v123] | quite a lot | quite a lot | not very much |
how much confidence in: European Union (Q38J)[v124] | quite a lot | quite a lot | not very much |
how much confidence in: United Nations Organization (Q38K)[v125] | quite a lot | quite a lot | not very much |
how much confidence in: justice system (Q38M)[v127] | quite a lot | quite a lot | not very much |
how much confidence in: environmental organizations (Q38O)[v129] | quite a lot | quite a lot | not very much |
There seem to be two typical ways to answer these questions: One that reflects some positive level of trust in all of these institutions and one that does not. Interestingly, in addition, the first tangle splits off a slight variation differing only in the trust in the institution of church. It seems to be a bit less common to trust the church than the other “trustful” institutions as there are no other ‘transition’-tangles between the extreme cases - at least at this agreement level and if we only consider full specifications.
The library provides a small convenience function to visualize tangle search results and show the inherent tree structure.
So, finally, let’s have a look at the Tangle Search Tree. In most cases it is not easy to visualise tangles. Therefore, the user has to provide a couple of callback functions to customize the visualization. We use two custom functions to translate the metadata of the edges to a more readable form:
from tangles.convenience.survey_tangles_visualization import plot_tangle_search_tree
ordered_metadata = tangles.ordered_metadata(insert_labels=False)
def level_label(level):
if level >= len(ordered_metadata):
return ""
var = ordered_metadata[level][0].info[0]
label = tangles.survey.variables[var].label
label = label[:(i:=label.index(":")+1)]+"\n" + label[i+1:label.index("(")] # + f"({var})"
return (label, 'top', -0.5)
def edge_label_func(var, op , val):
# to avoid confusion: we inverted the operation for a more natural interpretation (bigger numeric values mean lower trust)
if op == ">=":
return f"'{tangles.survey.variables[var].valid_values.get(val) or val}' or less"
else:
return f"more than '{tangles.survey.variables[var].valid_values.get(val) or val}'"
plt.figure(figsize=(10,5))
plot_tangle_search_tree(tangles, ax=plt.gca(), only_max_level_tangles=True, level_label_func=level_label,
draw_edge_labels=True, _include_splitting='nodes',
level_label_width=2, edge_label_func_for_meta_data=edge_label_func)
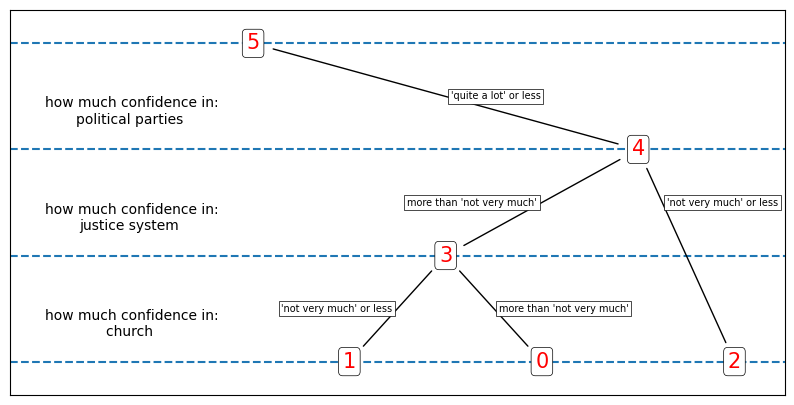
3. Summary#
We have seen an example tangle analysis of a part of the European Value Study. The purpose of this notebook was not primarily to generate meaningful insights into the data but to provide a quick overview of the steps needed to use tangles on survey data. Let’s quickly summarize some important aspects:
The survey data has to be converted to an internal data structure, called a
Survey
. This class provides all functionality needed to do a convenient tangle analysisThe
Survey
data structure can be set up with data created or loaded with external libraries - like, for example,pandas
orpyreadstat
.Usually, the meta information provided by
pyreadstat
can not be used ‘out of the box’ but has to be customized after setting up theSurvey
object.If the
Survey
object is set up correctly, the classSurveyTangles
provides simple-to-use functions for tangle analysis and the interpretation of results.
The scope of this notebook only allowed us to touch the surface of the functionality available in the survey interface and the tangle library in its entirety. Please consult the documentation for an in-depth overview of the full functionality.